Introduction to Touch Lamps
A touch lamp is a unique and innovative lighting solution that allows you to control the lamp by simply touching its base or any other conductive surface. Unlike traditional lamps that require a physical switch, touch lamps utilize a touch-sensitive circuit to detect human touch and toggle the light on or off accordingly. This article will provide an in-depth exploration of Touch Lamp Circuits, their working principles, components, and how to build your own touch lamp.
What is a Touch Lamp?
A touch lamp is a type of lamp that is activated or deactivated by human touch, rather than a conventional switch. When you touch the lamp’s base or any other designated conductive surface, the touch-sensitive circuit detects the change in capacitance caused by your body’s electrical field. This triggers the lamp to turn on or off, or even cycle through different brightness levels with each successive touch.
Advantages of Touch Lamps
Touch lamps offer several advantages over traditional lamps:
-
Convenience: With a touch lamp, you don’t need to fumble for a switch in the dark. A simple touch is all it takes to control the light.
-
Aesthetic Appeal: Touch lamps often have a sleek and modern design, as they lack visible switches or buttons. This can contribute to a clean and minimalist look in your home or office.
-
Durability: Since touch lamps don’t have mechanical switches, they are less prone to wear and tear over time. This can extend the lifespan of the lamp.
-
Versatility: Touch lamps can be made in various shapes, sizes, and styles to suit different decor preferences and lighting needs.
How Touch Lamps Work
The Capacitive Sensing Principle
Touch lamps operate on the principle of capacitive sensing. Capacitive sensing is a technology that detects the presence or absence of human touch by measuring the change in capacitance on a conductive surface.
In a touch lamp circuit, a conductive plate or surface acts as a capacitive sensor. When a person touches this surface, their body acts as a capacitor, storing a small electrical charge. This change in capacitance is detected by the touch lamp circuit, which then triggers the lamp to turn on or off.
Components of a Touch Lamp Circuit
A basic touch lamp circuit consists of the following components:
-
Microcontroller: The microcontroller is the brain of the touch lamp circuit. It processes the touch input and controls the lamp’s output accordingly. Common microcontrollers used in touch lamp circuits include Arduino, PIC, and ATtiny.
-
Capacitive Sensor: The capacitive sensor is the conductive surface that detects human touch. It can be a metal plate, a conductive pad, or even the lamp’s base itself.
-
Transistor: The transistor acts as a switch that controls the flow of current to the lamp. When the microcontroller detects a touch, it sends a signal to the transistor, which then allows current to flow to the lamp.
-
Resistors: Resistors are used to limit the current flow and protect the Circuit Components from damage.
-
Lamp: The lamp is the output device that provides illumination when the Touch Sensor is activated.
Circuit Diagram and Working Explanation
Here’s a simplified circuit diagram of a basic touch lamp:
+------+
| |
| MC |
| |
+---+--+
|
|
|
+---+--+
| |
| CS |
| |
+------+
|
|
|
+---+--+
| |
| T |
| |
+---+--+
|
|
|
+---+--+
| |
| L |
| |
+------+
- MC: Microcontroller
- CS: Capacitive Sensor
- T: Transistor
- L: Lamp
When a person touches the capacitive sensor (CS), it causes a change in capacitance. The microcontroller (MC) constantly monitors the capacitance level of the sensor. When it detects a significant change, it interprets it as a touch event.
Upon detecting a touch, the microcontroller sends a signal to the transistor (T). The transistor acts as a switch, allowing current to flow from the power source to the lamp (L). This turns the lamp on.
When the person removes their touch, the capacitance level returns to its baseline value. The microcontroller detects this change and sends a signal to the transistor to turn off the current flow, thereby turning the lamp off.
Building a Touch Lamp Circuit
Required Components
To build a basic touch lamp circuit, you will need the following components:
Component | Quantity |
---|---|
Arduino Uno | 1 |
Capacitive Sensor | 1 |
NPN Transistor | 1 |
220Ω Resistor | 1 |
10kΩ Resistor | 1 |
LED Lamp | 1 |
Jumper Wires | As needed |
Step-by-Step Guide
- Connect the capacitive sensor:
- Connect one end of the capacitive sensor to an analog input pin on the Arduino (e.g., A0).
-
Connect the other end of the sensor to a 10kΩ resistor, and then connect the other end of the resistor to ground.
-
Set up the transistor:
- Connect the collector of the NPN transistor to one lead of the LED lamp.
- Connect the other lead of the LED lamp to the positive power supply (e.g., 5V on Arduino).
-
Connect the emitter of the transistor to ground.
-
Configure the base resistor:
-
Connect a 220Ω resistor between a digital output pin on the Arduino (e.g., D2) and the base of the transistor.
-
Upload the code:
- Open the Arduino IDE and create a new sketch.
- Write the code to read the capacitive sensor value and control the lamp based on the touch input.
-
Upload the code to the Arduino board.
-
Test the touch lamp:
- Power on the Arduino board.
- Touch the capacitive sensor and observe if the lamp turns on.
- Remove your touch and see if the lamp turns off.
Sample Arduino Code
Here’s a sample Arduino code for a basic touch lamp:
const int SENSOR_PIN = A0;
const int LAMP_PIN = 2;
const int THRESHOLD = 500;
void setup() {
pinMode(LAMP_PIN, OUTPUT);
}
void loop() {
int sensorValue = analogRead(SENSOR_PIN);
if (sensorValue > THRESHOLD) {
digitalWrite(LAMP_PIN, HIGH);
} else {
digitalWrite(LAMP_PIN, LOW);
}
delay(100);
}
This code reads the analog value from the capacitive sensor connected to pin A0. If the sensor value exceeds a predefined threshold (in this case, 500), it turns on the lamp by setting the digital output pin 2 to HIGH. Otherwise, it turns off the lamp by setting pin 2 to LOW.
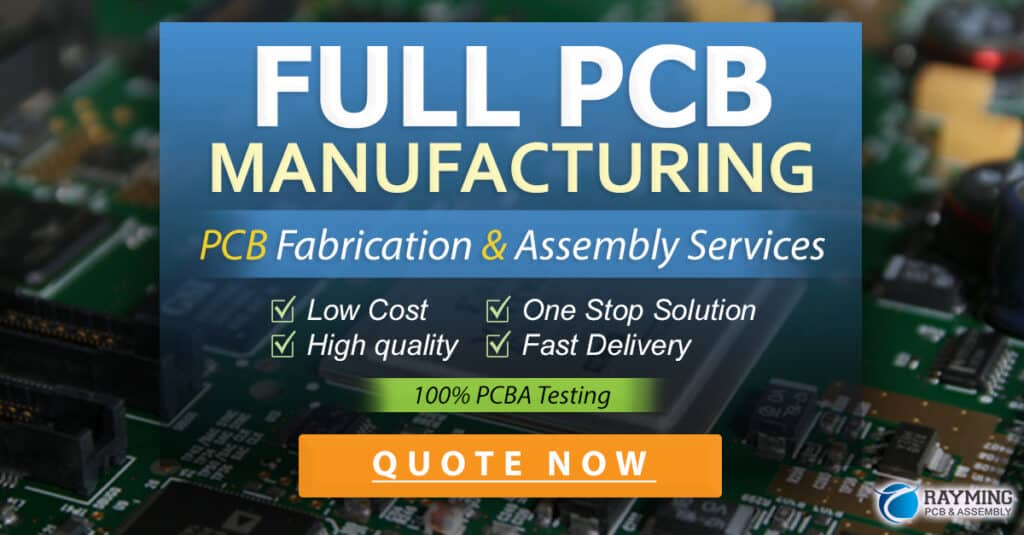
Advanced Touch Lamp Circuits
Multiple Brightness Levels
You can enhance your touch lamp circuit to support multiple brightness levels. Each successive touch can cycle through different brightness settings. This can be achieved by modifying the Arduino code to keep track of the number of touches and adjust the lamp’s brightness accordingly.
Here’s an example of how you can modify the code to support three brightness levels:
const int SENSOR_PIN = A0;
const int LAMP_PIN = 3;
const int THRESHOLD = 500;
const int MAX_LEVEL = 3;
int brightnessLevel = 0;
int lastTouchTime = 0;
void setup() {
pinMode(LAMP_PIN, OUTPUT);
}
void loop() {
int sensorValue = analogRead(SENSOR_PIN);
if (sensorValue > THRESHOLD) {
if (millis() - lastTouchTime > 500) {
brightnessLevel = (brightnessLevel + 1) % MAX_LEVEL;
lastTouchTime = millis();
}
}
analogWrite(LAMP_PIN, brightnessLevel * 85);
delay(100);
}
In this modified code:
– We define MAX_LEVEL
as the number of brightness levels (in this case, 3).
– We introduce a variable brightnessLevel
to keep track of the current brightness level.
– We use lastTouchTime
to debounce the touch input and avoid multiple touches being registered in quick succession.
– Inside the touch detection condition, we increment brightnessLevel
and wrap it around to 0 when it reaches MAX_LEVEL
.
– We use analogWrite()
to set the brightness of the lamp based on the current brightnessLevel
.
Proximity Sensing
Another advanced feature you can add to your touch lamp circuit is proximity sensing. Instead of requiring physical touch, the lamp can turn on when your hand is in close proximity to the sensor. This can be achieved using a capacitive Proximity Sensor or an infrared (IR) sensor.
Here’s an example of how you can modify the circuit and code to incorporate proximity sensing using an IR sensor:
- Connect the IR sensor:
- Connect the VCC pin of the IR sensor to the 5V pin on the Arduino.
- Connect the GND pin of the IR sensor to the GND pin on the Arduino.
-
Connect the OUT pin of the IR sensor to a digital input pin on the Arduino (e.g., D3).
-
Modify the Arduino code:
const int SENSOR_PIN = 3;
const int LAMP_PIN = 2;
void setup() {
pinMode(SENSOR_PIN, INPUT);
pinMode(LAMP_PIN, OUTPUT);
}
void loop() {
int sensorValue = digitalRead(SENSOR_PIN);
if (sensorValue == LOW) {
digitalWrite(LAMP_PIN, HIGH);
} else {
digitalWrite(LAMP_PIN, LOW);
}
delay(100);
}
In this modified code:
– We use a digital input pin (D3) to read the output from the IR sensor.
– When the IR sensor detects an object in close proximity, it pulls the output pin LOW. We check for this condition and turn on the lamp accordingly.
– When no object is detected, the IR sensor output remains HIGH, and we turn off the lamp.
Troubleshooting and Common Issues
False Triggering
One common issue with touch lamp circuits is false triggering, where the lamp turns on or off unintentionally. This can happen due to various reasons, such as:
- Electrical noise interference
- Nearby conductive objects or surfaces
- Humidity or moisture affecting the sensor
To mitigate false triggering, you can try the following:
- Adjust the sensitivity threshold in the code to require a stronger touch or proximity signal.
- Use shielded cables or wires to reduce electrical noise interference.
- Ensure proper grounding of the circuit components.
- Place the capacitive sensor or conductive surface away from other conductive objects or surfaces.
- Apply conformal coating or insulation to protect the sensor from moisture.
Inconsistent Behavior
Another issue you might encounter is inconsistent behavior, where the touch lamp doesn’t respond reliably to touches or proximity. This can be caused by:
- Loose or faulty connections in the circuit
- Damaged or malfunctioning components
- Insufficient power supply
To troubleshoot inconsistent behavior:
- Double-check all the connections in the circuit and ensure they are secure.
- Test the individual components (sensor, transistor, lamp) separately to identify any faulty parts.
- Ensure the power supply is providing sufficient voltage and current to the circuit.
- Use a multimeter to measure voltages and continuity at different points in the circuit.
Frequently Asked Questions (FAQ)
- Can I use any type of lamp with a touch lamp circuit?
-
Yes, you can use any lamp that is compatible with the voltage and current requirements of your touch lamp circuit. Common options include LED lamps, incandescent bulbs, or even small appliances like fans or motors.
-
How do I adjust the sensitivity of the touch sensor?
-
You can adjust the sensitivity of the touch sensor by modifying the threshold value in the Arduino code. Increase the threshold value to require a stronger touch or proximity signal, or decrease it to make the sensor more sensitive.
-
Can I control multiple lamps with a single touch lamp circuit?
-
Yes, you can control multiple lamps with a single touch lamp circuit by connecting them in parallel to the transistor’s collector. However, ensure that the total current drawn by the lamps does not exceed the maximum rating of the transistor and the power supply.
-
How can I make my touch lamp circuit more energy-efficient?
-
To make your touch lamp circuit more energy-efficient, you can use low-power components such as LEDs instead of incandescent bulbs. Additionally, you can implement features like auto-off timers or ambient Light Sensors to turn off the lamp when it’s not needed.
-
Can I integrate my touch lamp circuit with other smart home devices?
- Yes, you can integrate your touch lamp circuit with other smart home devices by adding wireless connectivity modules like Wi-Fi, Bluetooth, or Zigbee to your microcontroller. This allows you to control the touch lamp remotely or sync it with other smart home automation systems.
Conclusion
Touch lamp circuits offer a convenient and innovative way to control lighting in your home or office. By understanding the capacitive sensing principle and the basic components of a touch lamp circuit, you can build your own touch-sensitive lamps with ease.
Through this comprehensive guide, we’ve covered the working principles of touch lamps, the step-by-step process of building a basic touch lamp circuit, and advanced features like multiple brightness levels and proximity sensing. We’ve also discussed common issues and troubleshooting techniques to help you overcome any challenges you might face.
With the knowledge gained from this article, you can unleash your creativity and experiment with different touch lamp designs and functionalities. Whether you’re a hobbyist, a DIY enthusiast, or simply looking to add a touch of innovation to your lighting setup, touch lamp circuits provide a fun and rewarding project to explore.
So go ahead, gather your components, and start building your own touch lamp circuit today! Feel the magic of controlling light with a simple touch and enjoy the convenience and aesthetic appeal it brings to your space.
No responses yet