Introduction to Touch Lamp Circuits
A touch lamp circuit is an electronic circuit that allows a lamp to be turned on and off by simply touching a conductive surface, such as a metal plate or a human hand. This type of circuit is becoming increasingly popular in modern lighting design due to its convenience, aesthetics, and energy efficiency. In this article, we will explore the fundamentals of touch lamp circuits, their components, and how they work.
What is a Touch Lamp Circuit?
A touch lamp circuit is an electronic circuit that uses the human body’s natural capacitance to detect when a person touches a conductive surface. When a person touches the surface, their body acts as a capacitor, which changes the circuit’s capacitance and triggers the lamp to turn on or off. This type of circuit is often used in touch lamps, where the user can simply touch the lamp’s base or a specific area to control the light.
Advantages of Touch Lamp Circuits
Touch lamp circuits offer several advantages over traditional lamp switches:
- Convenience: Users can easily turn the lamp on or off without having to fumble for a switch in the dark.
- Aesthetics: Touch lamps often have a sleek, modern design without visible switches, which can enhance the overall appearance of a room.
- Energy efficiency: Touch lamps can be programmed to turn off automatically after a certain period of inactivity, saving energy and reducing electricity costs.
- Durability: With no mechanical switches, touch lamps are less prone to wear and tear, increasing their lifespan.
Components of a Touch Lamp Circuit
A basic touch lamp circuit consists of several key components:
- Microcontroller: The brain of the circuit, responsible for processing the touch input and controlling the lamp’s output.
- Touch Sensor: A conductive surface, such as a metal plate or a capacitive sensor, that detects the user’s touch.
- Transistor: Used to switch the lamp on and off based on the microcontroller’s output.
- Resistors and capacitors: Passive components that help regulate the circuit’s voltage and current.
- Lamp: The light source connected to the circuit, typically an LED or incandescent bulb.
Microcontrollers for Touch Lamp Circuits
Microcontrollers are essential components in touch lamp circuits, as they process the touch input and control the lamp’s output. Some popular microcontrollers used in touch lamp circuits include:
- Arduino: An open-source platform that offers a wide range of microcontroller boards and a user-friendly programming environment.
- PIC microcontrollers: A family of microcontrollers manufactured by Microchip Technology, known for their low cost and ease of use.
- ATtiny microcontrollers: A series of compact, low-power microcontrollers produced by Atmel (now owned by Microchip Technology), ideal for small-scale projects like touch lamps.
When choosing a microcontroller for your touch lamp circuit, consider factors such as processing power, memory, number of input/output pins, and compatibility with your preferred programming language.
Touch Sensors for Touch Lamp Circuits
Touch sensors are the key components that detect the user’s touch and trigger the lamp to turn on or off. There are two main types of touch sensors used in touch lamp circuits:
-
Capacitive touch sensors: These sensors detect changes in capacitance when a user touches the sensor surface. They are highly sensitive and can detect touch through non-conductive materials like glass or plastic.
-
Conductive touch sensors: These sensors are simple metal plates that detect the user’s touch through direct contact. They are less sensitive than capacitive sensors but are often cheaper and easier to implement.
When selecting a touch sensor for your touch lamp circuit, consider factors such as sensitivity, size, and compatibility with your chosen microcontroller.
How Touch Lamp Circuits Work
Touch lamp circuits work by detecting changes in capacitance when a user touches the sensor surface. The human body acts as a capacitor, and when it comes into contact with the touch sensor, it changes the circuit’s capacitance. The microcontroller continuously monitors the touch sensor’s capacitance and detects when a touch event occurs.
Detecting Touch Events
To detect touch events, the microcontroller typically uses a technique called capacitive sensing. This involves measuring the time it takes to charge or discharge the touch sensor’s capacitance through a resistor. When a user touches the sensor, the capacitance increases, which changes the charging or discharging time. The microcontroller can then compare this time to a predefined threshold to determine whether a touch event has occurred.
Controlling the Lamp Output
Once a touch event is detected, the microcontroller processes the input and determines whether to turn the lamp on or off. This is usually achieved by sending a signal to a transistor, which acts as a switch to control the current flowing to the lamp.
For example, when the microcontroller detects a touch event, it can send a high signal to the transistor’s base, allowing current to flow from the power supply to the lamp, turning it on. When another touch event is detected, the microcontroller sends a low signal to the transistor’s base, cutting off the current flow and turning the lamp off.
Debouncing Touch Events
One challenge in implementing touch lamp circuits is dealing with false touch events caused by electrical noise or unintentional touches. To minimize these false events, microcontrollers often employ a technique called debouncing.
Debouncing involves setting a short delay between the detection of a touch event and the execution of the corresponding action (turning the lamp on or off). During this delay, the microcontroller continuously checks the touch sensor’s state to ensure that the touch event is stable and not a result of noise or accidental contact. If the touch event persists throughout the debounce period, the microcontroller proceeds with turning the lamp on or off.
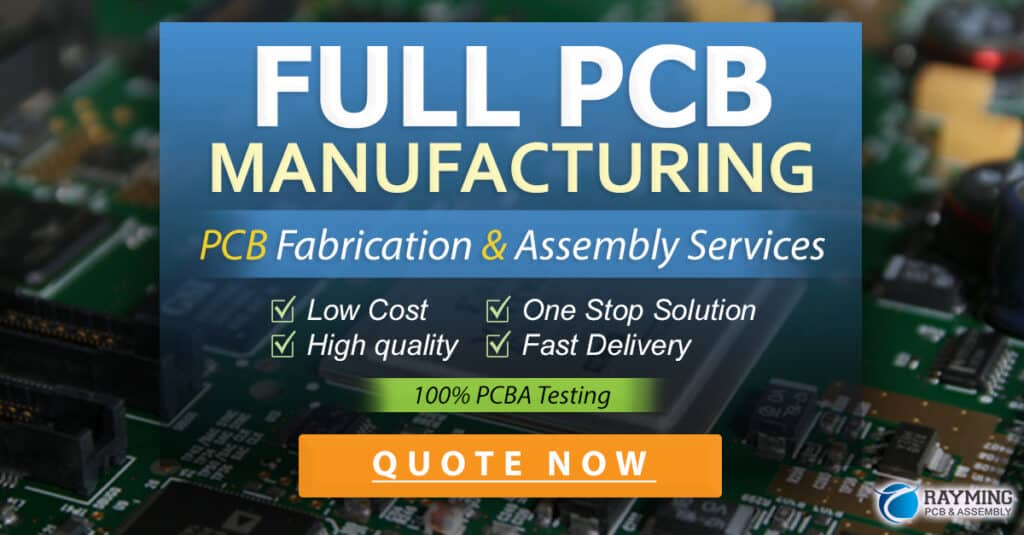
Building a Touch Lamp Circuit
Building a touch lamp circuit requires a basic understanding of electronic components and circuits. In this section, we will guide you through the process of creating a simple touch lamp circuit using an Arduino microcontroller.
Required Components
To build an Arduino-based touch lamp circuit, you will need the following components:
- Arduino Uno or compatible microcontroller board
- Capacitive touch sensor (e.g., AT42QT1010)
- NPN transistor (e.g., BC547)
- 1 kΩ resistor
- LED or incandescent lamp
- Breadboard and jumper wires
- Power supply (e.g., 9V battery or AC adapter)
Circuit Diagram
Here’s a simple circuit diagram for an Arduino-based touch lamp:
+-----+
| |
+---------|VCC |
| | |
| |GND |
| | |
| |SDA |
| | |
| |SCL |
| +-----+
| |
| |
| |
| +-+
| | |
| | |1kΩ
| | |
| +-+
| |
| |
| |
| +-+
| | |
| | |BC547
| | |
| +-+
| |
| |
| |
| +---+
| | |
| |LED|
| | |
| +---+
| |
| |
GND GND
In this circuit:
– The capacitive touch sensor (AT42QT1010) is connected to the Arduino’s VCC, GND, SDA, and SCL pins.
– A 1 kΩ resistor is connected between the touch sensor’s SCL pin and the base of the NPN transistor (BC547).
– The transistor’s collector is connected to the LED or lamp, and the emitter is connected to ground.
– The LED or lamp’s other terminal is connected to the power supply’s positive terminal.
Arduino Code
Here’s a sample Arduino code for the touch lamp circuit:
#include <Wire.h>
#include "AT42QT1010.h"
const int TOUCH_SENSOR_ADDR = 0x1B;
const int LED_PIN = 13;
const int DEBOUNCE_DELAY = 50;
AT42QT1010 touchSensor(TOUCH_SENSOR_ADDR);
bool ledState = false;
unsigned long lastTouchTime = 0;
void setup() {
Wire.begin();
touchSensor.begin();
pinMode(LED_PIN, OUTPUT);
}
void loop() {
if (touchSensor.isTouch() && millis() - lastTouchTime > DEBOUNCE_DELAY) {
lastTouchTime = millis();
ledState = !ledState;
digitalWrite(LED_PIN, ledState);
}
}
This code:
1. Includes the necessary libraries for the touch sensor and I2C communication.
2. Defines constants for the touch sensor’s I2C address, LED pin, and debounce delay.
3. Initializes the touch sensor and LED pin in the setup()
function.
4. Continuously checks for touch events in the loop()
function.
5. If a touch event is detected and the debounce delay has passed, it toggles the LED state and updates the lastTouchTime
.
Building and Testing the Circuit
To build and test the touch lamp circuit:
- Assemble the components on a breadboard according to the circuit diagram.
- Connect the Arduino to your computer using a USB cable.
- Open the Arduino IDE and upload the provided code to the Arduino board.
- Touch the capacitive sensor and observe the LED turning on or off.
If the LED responds correctly to your touch, congratulations! You have successfully built a basic touch lamp circuit.
Advanced Touch Lamp Circuits
While the basic touch lamp circuit is functional, there are several ways to enhance its features and performance. In this section, we will explore some advanced touch lamp circuit concepts and techniques.
Multi-Level Brightness Control
Instead of simply turning the lamp on or off, you can implement multi-level brightness control to allow users to adjust the lamp’s brightness by touching the sensor for different durations or in specific patterns.
To achieve this, you can:
1. Use pulse-width modulation (PWM) to control the lamp’s brightness. PWM involves rapidly switching the lamp on and off at different duty cycles to create the illusion of varying brightness levels.
2. Modify the Arduino code to detect the duration of touch events and adjust the PWM duty cycle accordingly. For example, you can set up the circuit to cycle through different brightness levels (e.g., 25%, 50%, 75%, 100%) each time the user touches and holds the sensor for a specific duration.
3. Implement a capacitive sensing library that supports multi-touch gestures, such as the Arduino CapacitiveSensor library, to detect different touch patterns and assign them to different brightness levels.
Proximity Sensing
Another advanced feature you can add to your touch lamp circuit is proximity sensing. This allows the lamp to turn on automatically when a user’s hand is near the sensor, without requiring direct contact.
To implement proximity sensing:
1. Choose a proximity sensor that is compatible with your microcontroller, such as the HC-SR04 ultrasonic sensor or the APDS-9960 proximity sensor.
2. Connect the proximity sensor to your microcontroller according to the sensor’s datasheet and the microcontroller’s pin configuration.
3. Modify the Arduino code to continuously read the proximity sensor’s output and turn the lamp on or off based on the user’s proximity to the sensor. You can set a threshold distance at which the lamp will be activated or deactivated.
4. Experiment with different threshold distances and sensor positions to find the optimal setup for your touch lamp circuit.
Gesture Control
Gesture control is another advanced feature that can enhance the user experience of your touch lamp circuit. By incorporating a gesture sensor, such as the APDS-9960, you can allow users to control the lamp using hand gestures like swiping or waving.
To add gesture control to your touch lamp circuit:
1. Connect the APDS-9960 gesture sensor to your microcontroller according to the sensor’s datasheet and the microcontroller’s pin configuration.
2. Install the necessary Arduino libraries for the APDS-9960 sensor, such as the SparkFun APDS-9960 RGB and Gesture Sensor library.
3. Modify the Arduino code to initialize the gesture sensor and continuously read its output. You can assign different gestures to different lamp functions, such as swiping left or right to adjust the brightness, or waving to turn the lamp on or off.
4. Test the gesture control functionality and adjust the sensor’s sensitivity and positioning as needed to ensure accurate gesture recognition.
By incorporating these advanced features into your touch lamp circuit, you can create a more interactive and user-friendly lighting experience.
Troubleshooting Touch Lamp Circuits
Despite careful design and implementation, touch lamp circuits may sometimes experience issues. In this section, we will discuss common problems encountered in touch lamp circuits and provide troubleshooting tips to help you resolve them.
Lamp Not Responding to Touch
If your touch lamp is not responding to touch events, consider the following:
- Check the wiring: Ensure that all components are correctly connected according to the circuit diagram. Look for loose connections, broken wires, or short circuits.
- Verify the power supply: Make sure that the power supply is providing the correct voltage and current to the circuit. Use a multimeter to test the voltage at various points in the circuit.
- Test the touch sensor: Disconnect the touch sensor from the circuit and test it separately using a multimeter or oscilloscope. If the sensor is not functioning properly, replace it with a new one.
- Check the microcontroller: Ensure that the microcontroller is programmed correctly and that the code is uploaded successfully. Use the Arduino IDE’s serial monitor to print debug messages and verify that the touch events are being detected correctly.
False Touch Events
If your touch lamp is turning on or off without being touched, or if it is responding erratically to touch events, consider the following:
- Adjust the debounce delay: Increase the debounce delay in your Arduino code to allow more time for the touch sensor to stabilize before executing the corresponding action.
- Implement software filtering: Use software filtering techniques, such as averaging multiple touch sensor readings or setting a minimum touch duration, to reduce the impact of electrical noise and unintentional touches.
- Improve the circuit’s shielding: Ensure that the touch sensor and its connections are adequately shielded from external noise sources, such as power lines or other electronic devices. Use shielded cables and ground planes to minimize interference.
- Calibrate the touch sensor: Some touch sensors, like capacitive sensors, may require calibration to account for variations in the operating environment. Consult the sensor’s datasheet for calibration procedures and implement them in your Arduino code.
Lamp Flickering or Dimming
If your touch lamp is flickering or dimming unintentionally, consider the following:
- Check the power supply: Ensure that the power supply is providing a stable and sufficient voltage and current to the lamp. Use a voltage regulator or a capacitor to smooth out any ripples in the power supply.
- Verify the transistor’s rating: Make sure that the transistor used in your circuit is rated for the lamp’s current and voltage requirements. If the transistor is underrated, it may not be able to handle the current flow, causing the lamp to flicker or dim.
- Implement PWM correctly: If you are using PWM to control the lamp’s brightness, ensure that the PWM frequency is high enough to avoid visible flickering. Use the Arduino’s
analogWrite()
function to set the PWM duty cycle and adjust the frequency if necessary. - Check for loose connections: Inspect the circuit for loose or intermittent connections, especially at the lamp and transistor terminals. Resolder any suspicious joints and ensure that all connections are secure.
By following these troubleshooting tips, you should be able to identify and resolve most common issues encountered in touch lamp circuits. If the problem persists, consider seeking assistance from experienced electronics enthusiasts or professionals.
Frequently Asked Questions (FAQ)
-
Q: Can I use any type of lamp with a touch lamp circuit?
A: Most touch lamp circuits are designed to work with low-voltage lamps, such as LEDs or small incandescent bulbs. If you want to use a higher-voltage lamp, you may need to modify the circuit to include a relay or a high-power transistor to handle the increased current and voltage requirements. -
Q: How do I change the touch sensitivity of my touch lamp circuit?
A: The touch sensitivity of your touch lamp circuit can be adjusted by modifying the threshold value in your Arduino code or by changing the value of the resistor connected to the touch sensor. Increasing the threshold value or the resistor value will make the touch sensor less sensitive, while decreasing these values will make it more sensitive. -
Q: Can I use a touch lamp circuit with a battery-powered lamp?
A: Yes, you can use a touch lamp circuit with a battery-powered lamp, as long as the battery provides the correct voltage and current for the circuit components. Keep in mind that the battery life may be reduced due to the continuous power consumption of the microcontroller and touch sensor. -
Q: How can I make my touch lamp circuit more energy-efficient?
A: To improve the energy efficiency of your touch lamp circuit, you can implement sleep modes or power-saving features in your microcont
No responses yet