Understanding Motion Sensor Circuits
Before diving into the DIY projects, let’s first understand the basics of Motion Sensor Circuits. A motion sensor circuit typically consists of the following components:
- Motion Sensor: The primary component that detects movement in its field of view. Common types include Passive Infrared (PIR) sensors, microwave sensors, and Ultrasonic Sensors.
- Microcontroller: A programmable device that processes the sensor data and controls the circuit’s behavior.
- Power Supply: Provides the necessary power to the components in the circuit.
- Output Device: The component that responds to the detected motion, such as an LED, buzzer, or relay.
Types of Motion Sensors
Sensor Type | Description | Detection Range | Power Consumption |
---|---|---|---|
PIR Sensor | Detects changes in infrared radiation emitted by objects in its field of view. | 5-10 meters | Low |
Microwave Sensor | Emits microwave radiation and detects changes in the reflected signal caused by motion. | 10-30 meters | High |
Ultrasonic Sensor | Emits high-frequency sound waves and detects changes in the reflected signal caused by motion. | 5-10 meters | Medium |
Project 1: PIR Sensor-Based Motion Detector
In this project, we will build a simple motion detector using a PIR sensor and an Arduino Microcontroller.
Components Required
- PIR sensor (HC-SR501)
- Arduino UNO board
- Breadboard
- LED
- 220Ω resistor
- Jumper wires
Circuit Diagram
PIR Sensor Arduino
VCC --------- 5V
GND --------- GND
OUT --------- Digital Pin 2
LED Arduino
Anode ------- Digital Pin 13
Cathode ----- GND (through 220Ω resistor)
Code
int pirSensor = 2;
int led = 13;
void setup() {
pinMode(pirSensor, INPUT);
pinMode(led, OUTPUT);
}
void loop() {
int sensorValue = digitalRead(pirSensor);
if (sensorValue == HIGH) {
digitalWrite(led, HIGH);
delay(5000); // Keep the LED on for 5 seconds
} else {
digitalWrite(led, LOW);
}
}
Project 2: Microwave Sensor-Based Motion Detector
This project utilizes a microwave sensor to detect motion and trigger an alarm.
Components Required
- Microwave sensor (HB100)
- Arduino UNO board
- Breadboard
- Buzzer
- Jumper wires
Circuit Diagram
Microwave Sensor Arduino
VCC ---------------- 5V
GND ---------------- GND
OUT ---------------- Digital Pin 3
Buzzer Arduino
Positive ----------- Digital Pin 9
Negative ----------- GND
Code
int microwaveSensor = 3;
int buzzer = 9;
void setup() {
pinMode(microwaveSensor, INPUT);
pinMode(buzzer, OUTPUT);
}
void loop() {
int sensorValue = digitalRead(microwaveSensor);
if (sensorValue == HIGH) {
tone(buzzer, 1000); // Activate the buzzer with a frequency of 1kHz
delay(5000); // Keep the buzzer on for 5 seconds
noTone(buzzer); // Turn off the buzzer
}
}
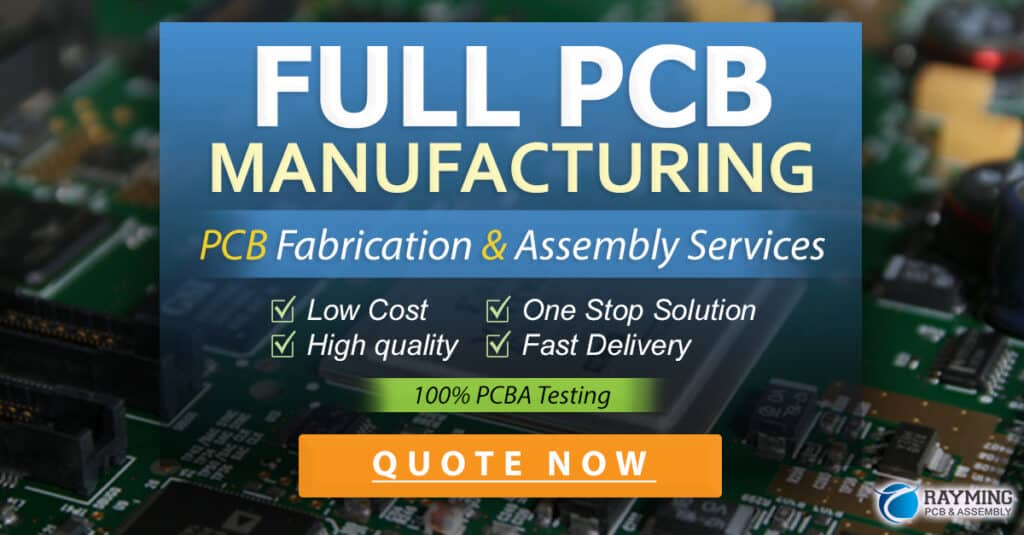
Project 3: Ultrasonic Sensor-Based Motion Detector
This project uses an ultrasonic sensor to detect motion and control an LED strip.
Components Required
- Ultrasonic sensor (HC-SR04)
- Arduino UNO board
- Breadboard
- LED strip (WS2812B)
- Jumper wires
Circuit Diagram
Ultrasonic Sensor Arduino
VCC ---------------- 5V
GND ---------------- GND
TRIG --------------- Digital Pin 4
ECHO --------------- Digital Pin 5
LED Strip Arduino
VCC ---------------- 5V
GND ---------------- GND
DIN ---------------- Digital Pin 6
Code
#include <FastLED.h>
#define LED_PIN 6
#define NUM_LEDS 30
CRGB leds[NUM_LEDS];
int trigPin = 4;
int echoPin = 5;
void setup() {
FastLED.addLeds<WS2812B, LED_PIN, GRB>(leds, NUM_LEDS);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
}
void loop() {
long duration, distance;
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration / 2) / 29.1;
if (distance < 100) { // Detect motion within 100 cm
fill_solid(leds, NUM_LEDS, CRGB::White);
FastLED.show();
delay(5000); // Keep the LED strip on for 5 seconds
} else {
fill_solid(leds, NUM_LEDS, CRGB::Black);
FastLED.show();
}
}
Project 4: Dual Sensor Motion Detector
This project combines a PIR sensor and an ultrasonic sensor to create a more reliable motion detector.
Components Required
- PIR sensor (HC-SR501)
- Ultrasonic sensor (HC-SR04)
- Arduino UNO board
- Breadboard
- LED
- 220Ω resistor
- Jumper wires
Circuit Diagram
PIR Sensor Arduino
VCC --------- 5V
GND --------- GND
OUT --------- Digital Pin 2
Ultrasonic Sensor Arduino
VCC ---------------- 5V
GND ---------------- GND
TRIG --------------- Digital Pin 4
ECHO --------------- Digital Pin 5
LED Arduino
Anode ------- Digital Pin 13
Cathode ----- GND (through 220Ω resistor)
Code
int pirSensor = 2;
int trigPin = 4;
int echoPin = 5;
int led = 13;
void setup() {
pinMode(pirSensor, INPUT);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(led, OUTPUT);
}
void loop() {
int pirValue = digitalRead(pirSensor);
long duration, distance;
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration / 2) / 29.1;
if (pirValue == HIGH && distance < 100) {
digitalWrite(led, HIGH);
delay(5000); // Keep the LED on for 5 seconds
} else {
digitalWrite(led, LOW);
}
}
Project 5: Wireless Motion Detector
This project uses a PIR sensor and an ESP8266 Wi-Fi module to create a wireless motion detector that sends notifications to a smartphone.
Components Required
- PIR sensor (HC-SR501)
- ESP8266 Wi-Fi module (NodeMCU)
- Breadboard
- Jumper wires
Circuit Diagram
PIR Sensor NodeMCU
VCC --------- 3.3V
GND --------- GND
OUT --------- Digital Pin D1
Code
#include <ESP8266WiFi.h>
#include <ESP8266HTTPClient.h>
const char* ssid = "your_wifi_ssid";
const char* password = "your_wifi_password";
const char* serverName = "http://your_server_address/motion_detected";
int pirSensor = D1;
void setup() {
pinMode(pirSensor, INPUT);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
}
}
void loop() {
int sensorValue = digitalRead(pirSensor);
if (sensorValue == HIGH) {
if (WiFi.status() == WL_CONNECTED) {
HTTPClient http;
http.begin(serverName);
int httpResponseCode = http.GET();
if (httpResponseCode > 0) {
// Motion detection notification sent successfully
}
http.end();
}
}
delay(1000);
}
Frequently Asked Questions (FAQ)
- What is the difference between PIR, microwave, and ultrasonic sensors?
-
PIR Sensors detect changes in infrared radiation, microwave sensors emit and detect changes in microwave radiation, and ultrasonic sensors emit and detect changes in high-frequency sound waves.
-
Can I use a different microcontroller instead of Arduino?
-
Yes, you can use any compatible microcontroller, such as Raspberry Pi or ESP32, with appropriate modifications to the code and circuit connections.
-
How can I adjust the sensitivity of the motion sensors?
-
Most motion sensors have built-in potentiometers or sensitivity adjustment pins that allow you to fine-tune the sensitivity according to your requirements.
-
What is the maximum range of these motion sensors?
-
The detection range varies depending on the sensor type. PIR and ultrasonic sensors typically have a range of 5-10 meters, while microwave sensors can detect motion up to 10-30 meters.
-
Can I power the motion detector circuits using batteries?
- Yes, you can power the circuits using batteries. However, ensure that the battery voltage is compatible with the components used in the circuit and consider the power consumption of the sensors and microcontroller.
By following these DIY projects, you can create your own motion sensor circuits and customize them to suit your specific needs. Remember to always handle electronic components with care and follow proper safety precautions while working on these projects.
No responses yet