Key Components of an MCU board
At the heart of any MCU board is the microcontroller chip itself. This is typically an integrated circuit that contains a processor core, memory (RAM and flash), and programmable input/output peripherals. Some common microcontroller families used on MCU boards include:
- AVR (by Microchip)
- PIC (by Microchip)
- STM32 (by STMicroelectronics)
- ESP32 (by Espressif)
In addition to the microcontroller, an MCU board will have several other important components:
Power Supply Circuitry
MCU boards need a stable power supply to operate reliably. They typically have an on-board Voltage Regulator that steps down the input voltage (e.g. 5V from USB) to the operating voltage required by the microcontroller (e.g. 3.3V). There are also filters to smooth out any noise on the power rails.
Oscillator
Microcontrollers require a clock source to synchronize their internal operations. Many MCU boards have a Crystal Oscillator that generates a precise clock signal, usually in the range of 8MHz to 48MHz.
Programming Interface
To load programs onto the microcontroller, MCU boards include a programming interface. The most common is an in-circuit serial programming (ICSP) header that allows an external programmer to communicate with the chip. Some boards have a USB interface that enables direct programming from a PC.
I/O Headers
MCU boards break out the I/O pins of the microcontroller to headers along the edges of the board. This allows easy interfacing with external circuitry using jumper wires or by plugging the board into a solderless breadboard. The pins usually include digital I/O, analog inputs, PWM outputs, and communication interfaces like I2C, SPI, and UART.
Other Features
Depending on the specific board and microcontroller used, MCU boards may have additional features like:
- LEDs for status indication
- Buttons for user input
- USB connector for power and communication
- MicroSD card slot for data logging
- Wireless connectivity via WiFi, Bluetooth, etc.
Here is a table summarizing the common components found on MCU boards:
Component | Function |
---|---|
Microcontroller | The programmable “brain” of the board |
Power Supply | Provides stable voltage to the board |
Oscillator | Clock source for microcontroller |
Programming Interface | Allows loading programs onto chip |
I/O Headers | Breaks out microcontroller pins |
USB Connector | Power and PC communication |
Wireless Module | WiFi, Bluetooth connectivity |
Programming an MCU Board
One of the key functions of an MCU board is that it can be programmed to perform a wide variety of tasks. The microcontroller is like a tiny computer that runs a single program over and over. This program is typically written in C or C++ and compiled into machine code that the microcontroller can execute.
The general steps for programming an MCU board are:
-
Write Code: Use an integrated development environment (IDE) to write and debug your embedded program. Popular choices are Arduino IDE, PlatformIO, and Mbed Studio.
-
Compile and Link: The code is compiled into an object file and linked with the necessary runtime libraries to produce an executable file in the HEX format, ready to be programmed to the microcontroller’s flash memory.
-
Program Chip: The HEX file is loaded onto the microcontroller using a programmer, which communicates with the chip via the board’s programming interface. Many boards have a USB interface that allows programming directly from the IDE.
-
Run and Debug: Once programmed, the microcontroller will run the program continuously. You can use the IDE’s serial monitor to view debug messages sent from the board. Some IDEs also support debugging with breakpoints and variable inspection.
Here’s an example of a simple Arduino program that blinks the on-board LED:
// Pin number for the LED
const int ledPin = 13;
void setup() {
// Configure LED pin as an output
pinMode(ledPin, OUTPUT);
}
void loop() {
digitalWrite(ledPin, HIGH); // Turn LED on
delay(1000); // Wait 1 second
digitalWrite(ledPin, LOW); // Turn LED off
delay(1000); // Wait 1 second
}
This code first configures pin 13 (which is typically connected to an on-board LED) as an output. Then, in an endless loop, it turns the LED on, waits 1 second, turns it off, and waits another second. The result is the LED blinking at a rate of 0.5 Hz.
Interfacing with Sensors and Actuators
Another key role of MCU boards is to interface with external sensors and actuators to allow the microcontroller to interact with the physical world. Sensors are devices that convert physical quantities like temperature, pressure, light, sound, etc. into electrical signals that the microcontroller can read. Actuators do the opposite – they convert electrical signals from the microcontroller into physical action, such as moving a motor, turning on a light, making a sound, etc.
MCU boards make it easy to connect sensors and actuators thanks to their I/O headers which provide access to the microcontroller’s peripheral interfaces. Here are some common interfaces and their uses:
Digital I/O
Digital I/O pins can read or write binary states – either 0 (LOW) or 1 (HIGH). They are commonly used for:
- Reading switch states (e.g. buttons, limit switches)
- Turning on/off LEDs, transistors, relays
- Generating pulses for Stepper Motors, servo motors
Analog Input
Analog input pins have an analog-to-digital converter (ADC) that reads voltage levels and converts them to a digital value. They are used for reading analog sensors like:
- Potentiometers for position, angle sensing
- LDRs, phototransistors for light sensing
- Thermistors for temperature measurement
- Microphones for sound detection
Pulse-Width Modulation (PWM)
PWM is a technique for generating analog-like signals using digital means. PWM pins output a high-frequency square wave where the width of the pulses can be varied to change the average voltage. PWM is used to:
- Control LED brightness
- Regulate motor speed, servo position
- Generate audio tones with a buzzer
Communication Interfaces
MCU boards often have dedicated hardware for serial Communication Protocols like:
- UART/USART for asynchronous serial (e.g. RS-232)
- I2C for connecting multiple devices on a bus
- SPI for high-speed synchronous serial
- 1-Wire for accessing small devices like EEPROMs
Using these, you can interface the MCU with all kinds of external peripherals like GPS modules, graphical LCDs, SD cards, and wireless transceivers.
Here’s a table of some popular sensors and their interfaces:
Sensor | Interface | Usage |
---|---|---|
DHT11 | 1-Wire | Temperature and humidity |
MPU6050 | I2C | Accelerometer, gyroscope |
ADXL345 | SPI | 3-axis accelerometer |
DS18B20 | 1-Wire | Waterproof temperature probe |
HC-SR501 | Digital I/O | PIR motion sensor |
ADMP401 | Analog Input | MEMS microphone |
GP2Y0A21 | Analog Input | IR distance sensor |
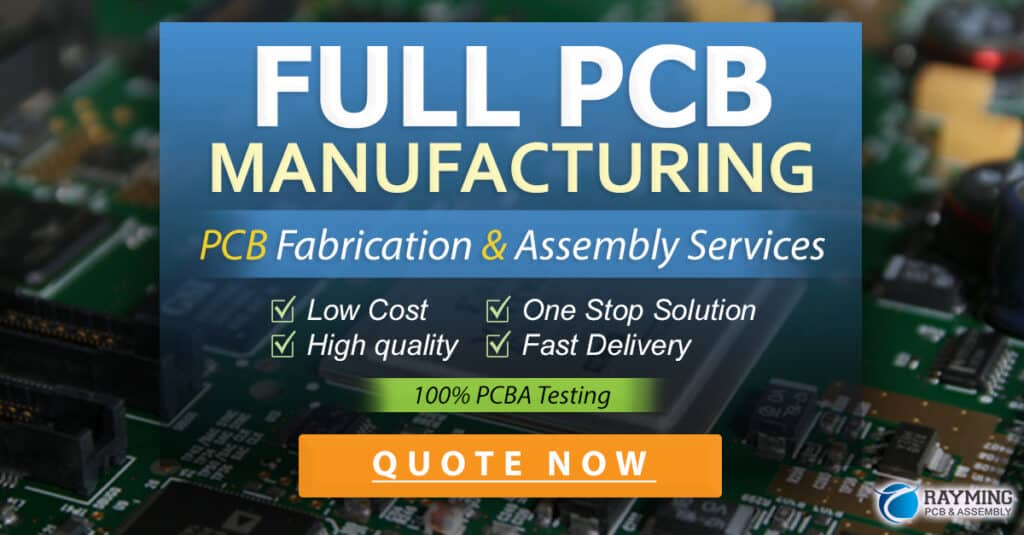
Example MCU Board Projects
To give you an idea of what’s possible with MCU boards, here are a few example projects:
Temperature Logger
A temperature logger uses a DS18B20 waterproof sensor to measure temperature at regular intervals, then saves the data along with a timestamp to a microSD card. The logged data can later be imported into Excel for analysis and graphing. Here’s a block diagram:
graph LR
A[DS18B20] --1-Wire--> B[MCU Board]
B --SPI--> C[MicroSD Card]
Wireless Weather Station
This project combines a BME280 environmental sensor (temperature, humidity, pressure) with an ESP8266 WiFi MCU board to create a wireless weather station. The MCU reads the sensor data and serves up a web page showing the current readings, which can be viewed from any device on the local network.
graph TD
A[BME280] --I2C--> B[ESP8266 MCU]
B --WiFi--> C[Web Browser]
Bluetooth Robot
A Bluetooth-controlled robot uses an HC-05 Bluetooth module to receive commands from a smartphone app. The MCU board reads these commands and controls motor drivers to move the robot accordingly. It also reads data from an ultrasonic distance sensor to avoid obstacles.
graph LR
A[Smartphone] --Bluetooth--> B[HC-05]
B --UART--> C[MCU Board]
C --PWM--> D[Motor Driver]
C --Trigger/Echo--> E[Ultrasonic Sensor]
As you can see, the combination of an MCU board’s processing power and diverse I/O capabilities allows it to be used in all kinds of applications from data logging to robotics to IoT and much more.
Frequently Asked Questions
What’s the difference between an MCU board and a single-board computer?
An MCU board is designed around a microcontroller chip, which typically has lower processing power but more flexible I/O compared to the microprocessors used in single-board computers (SBCs) like the Raspberry Pi. MCU boards are ideal for real-time control and interfacing with external electronics. SBCs run full operating systems like Linux and are more suited for high-level tasks like video processing, machine learning, etc.
Can I use an MCU board to run Linux?
Typically, no. Microcontrollers do not have the memory capacity or processing power needed to run a full Linux OS. There are some high-end microcontrollers like the STM32H7 that can run a very minimal Linux, but these are much more expensive and complex to work with compared to typical MCU boards. If you need to run Linux for your application, it’s better to choose a single-board computer instead.
How do I choose the right MCU board for my project?
Here are some factors to consider:
- I/O requirements: Make sure the board has the necessary peripherals (PWM, analog inputs, UARTs, etc.) to interface with your sensors and actuators.
- Processing power: Consider the complexity of your program and algorithms. For simple control tasks, a basic 8-bit AVR is sufficient, but for math-heavy tasks like digital signal processing, you may need a higher performance 32-bit ARM Cortex-M MCU.
- Memory: Your program needs to fit in the available flash memory, and you need sufficient RAM for any buffers and dynamic data.
- Power consumption: If your project is battery-powered, look for boards with lower-power MCUs and power-saving features.
- Development tools: Choose a board with good documentation, libraries, and an active community so you can get support and find example code.
Can I use an MCU board for machine learning?
Machine learning, especially deep learning, is very computationally intensive and requires a lot of memory. Most microcontrollers are not powerful enough to run complex machine learning models. However, there is a growing trend of “TinyML” – running optimized ML models on resource-constrained devices. Some higher-end MCU boards like the OpenMV H7 and Sony SPRESENSE have special accelerators for neural networks. But in general, if you’re doing heavy ML, it’s better to use a more powerful single-board computer or a dedicated ML module like the Intel Neural Compute Stick.
How can I learn more about programming MCU boards?
Here are some great resources to get started with MCU programming:
-
Arduino Tutorials: Arduino has a huge collection of beginner-friendly tutorials covering everything from blinking an LED to reading sensors and controlling motors. Even if you’re not using an official Arduino board, the concepts are applicable to other MCU boards.
-
MCU Manufacturer Sites: Microcontroller manufacturers like Microchip, STMicroelectronics, and NXP have extensive documentation, application notes, and example code for their chips. These go into more depth than the Arduino tutorials and cover advanced topics like low-power modes, bootloaders, and using the chip peripherals directly.
-
Online Courses: There are many online courses teaching embedded systems and microcontroller programming. Some popular ones are:
- Microcontrollers and the C Programming Language on Udemy
- Making Embedded Systems on Coursera
-
Books: Some recommended books for learning MCU programming are:
- “Making Embedded Systems” by Elicia White
- “Practical Electronics for Inventors” by Paul Scherz and Simon Monk
- “The Art of Electronics” by Paul Horowitz and Winfield Hill
Happy learning!
No responses yet