Introduction to API Design
API design is a crucial aspect of software development that focuses on creating effective and efficient interfaces for communication between different software components. A well-designed API enables developers to build scalable, maintainable, and user-friendly applications. In this article, we will explore the concepts of schematic API design, objects, and interfaces, and how they contribute to the overall success of an API.
What is an API?
An API, or Application Programming Interface, is a set of rules, protocols, and tools that define how software components should interact with each other. It acts as a bridge between different systems, allowing them to communicate and exchange data seamlessly. APIs can be used to integrate different applications, services, or platforms, enabling developers to create complex and powerful software solutions.
Why is API Design Important?
API design is essential because it directly impacts the usability, performance, and maintainability of an application. A poorly designed API can lead to confusion, errors, and inefficiencies, while a well-designed API can streamline development processes, reduce complexity, and enhance the overall user experience. Some key benefits of good API design include:
-
Ease of Use: A well-designed API is intuitive and easy to understand, making it simple for developers to integrate and use in their applications.
-
Consistency: Consistent API design ensures that developers can easily navigate and work with the API, reducing the learning curve and increasing productivity.
-
Scalability: A properly designed API can handle growth and changes in the application, allowing for easy expansion and modification as needed.
-
Maintainability: By following best practices and design principles, an API becomes more maintainable, reducing the effort required for updates, bug fixes, and feature enhancements.
Schematic API Design
Schematic API design is an approach that focuses on creating a clear and structured representation of an API’s components and their relationships. It involves defining the data models, endpoints, request/response formats, and authentication mechanisms in a systematic and organized manner.
Objects in API Design
In the context of API design, objects refer to the data entities or resources that an API exposes. These objects represent the core concepts and entities within the application domain. For example, in an e-commerce API, objects might include products, orders, customers, and payments.
When designing objects for an API, consider the following best practices:
-
Naming Conventions: Use clear, descriptive, and consistent names for objects and their properties. Follow established naming conventions, such as camelCase or snake_case, to ensure readability and maintainability.
-
Granularity: Define objects at the right level of granularity. Avoid exposing too much detail or creating overly complex objects. Instead, focus on providing the essential information needed for the specific use case.
-
Relationships: Clearly define the relationships between objects, such as one-to-one, one-to-many, or many-to-many. Use appropriate APIs like hypermedia links or foreign keys to represent these relationships.
-
Validation: Implement proper validation rules for object properties to ensure data integrity and consistency. Specify required fields, data types, and constraints to prevent invalid or inconsistent data from being stored or processed.
Interfaces in API Design
Interfaces in API design define the contract between the API and its consumers. They specify the operations, parameters, and expected responses for interacting with the API. Interfaces act as a boundary that encapsulates the internal implementation details and provides a stable and consistent way for clients to interact with the API.
When designing interfaces for an API, consider the following guidelines:
-
RESTful Principles: Follow RESTful principles when designing interfaces. Use HTTP methods (GET, POST, PUT, DELETE) to represent operations on resources, and leverage HTTP status codes to indicate the outcome of API requests.
-
Versioning: Implement versioning for your API Interfaces to manage changes and ensure backward compatibility. Use version numbers in the API URL or headers to allow clients to specify the desired version.
-
Request/Response Formats: Choose appropriate request and response formats, such as JSON or XML, based on the needs of your API consumers. Ensure that the formats are well-defined, consistent, and easy to parse.
-
Error Handling: Define clear and informative error responses for various scenarios, such as invalid requests, authentication failures, or server errors. Include meaningful error codes and messages to help clients understand and handle errors effectively.
-
Documentation: Provide comprehensive and up-to-date documentation for your API interfaces. Include details on endpoints, request/response formats, authentication requirements, and example code snippets to facilitate integration and usage.
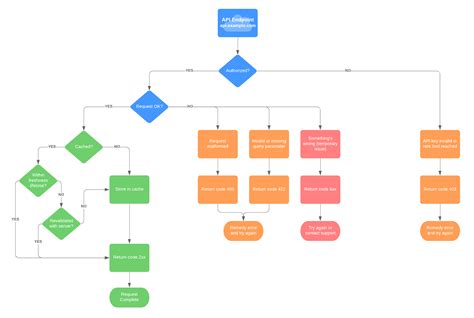
API Design Patterns
API design patterns are proven solutions to common design problems encountered when building APIs. These patterns provide guidelines and best practices to ensure consistency, scalability, and maintainability. Some popular API design patterns include:
Resource-Oriented Architecture (ROA)
ROA is a design pattern that focuses on representing API resources as addressable entities. Each resource is identified by a unique URL, and clients interact with these resources using standard HTTP methods. ROA promotes a clear separation of concerns and enables clients to perform CRUD (Create, Read, Update, Delete) operations on resources.
Hypermedia as the Engine of Application State (HATEOAS)
HATEOAS is a design pattern that emphasizes the use of hypermedia links within API responses. These links provide navigational information and allow clients to discover and navigate the API’s resources dynamically. HATEOAS enables loose coupling between the client and server, as the server can guide the client through the available actions and state transitions.
Versioning Strategies
Versioning is crucial for managing the evolution of an API over time. There are different versioning strategies to consider, such as:
- URL Versioning: Including the version number in the API URL, e.g.,
/api/v1/resources
. - Header Versioning: Specifying the version in the request headers, e.g.,
API-Version: 1.0
. - Parameter Versioning: Including the version as a query parameter, e.g.,
/api/resources?version=1.0
.
Choose a versioning strategy that aligns with your API’s requirements and development lifecycle.
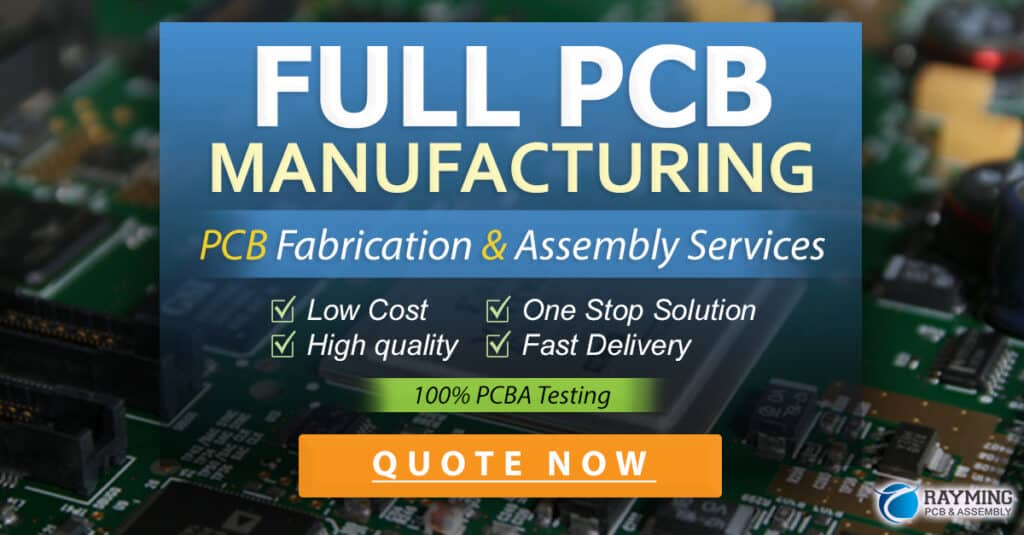
API Security
Security is a critical aspect of API design, as APIs often expose sensitive data and functionality. When designing an API, consider the following security measures:
Authentication and Authorization
Implement robust authentication mechanisms to verify the identity of API clients. Common authentication methods include API keys, OAuth tokens, or JSON Web Tokens (JWT). Use authorization controls to ensure that clients have the necessary permissions to access specific resources or perform certain actions.
Input Validation and Sanitization
Validate and sanitize all input data received from API clients to prevent security vulnerabilities such as SQL injection or cross-site scripting (XSS) attacks. Implement strict input validation rules and use secure input handling techniques to mitigate potential risks.
Secure Communication
Ensure that API communication is encrypted using secure protocols like HTTPS (HTTP over SSL/TLS). This protects sensitive data from eavesdropping and tampering during transmission. Implement proper certificate management and keep your SSL/TLS certificates up to date.
Rate Limiting and Throttling
Implement rate limiting and throttling mechanisms to prevent abuse and protect your API from excessive or malicious requests. Set appropriate limits on the number of requests allowed per client or IP address within a specific timeframe. Use techniques like request queuing or back-off algorithms to handle bursts of requests gracefully.
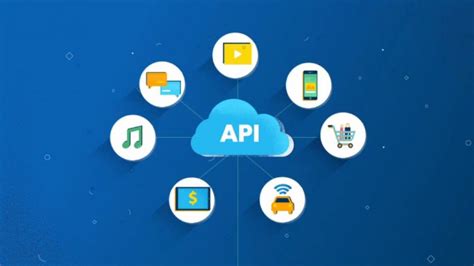
API Documentation
Comprehensive and well-structured documentation is essential for the success of an API. It helps developers understand how to use the API effectively and facilitates integration and adoption. When creating API documentation, consider the following:
Overview and Getting Started
Provide a high-level overview of your API, including its purpose, key features, and target audience. Include a getting started guide that walks developers through the process of setting up their development environment, authentication, and making their first API requests.
Endpoint Documentation
Document each endpoint of your API in detail, including the URL, HTTP method, request parameters, request body (if applicable), response format, and possible error codes. Provide clear examples of request and response payloads to illustrate usage.
Code Samples and SDKs
Include code samples in popular programming languages to demonstrate how to interact with your API. Consider providing software development kits (SDKs) or client libraries that simplify integration and reduce the effort required by developers to consume your API.
Changelog and Versioning
Maintain a changelog that documents changes made to your API over time, including new features, deprecations, and breaking changes. Clearly communicate the versioning scheme used and provide guidelines on how to handle version upgrades or backward compatibility.
API Monitoring and Analytics
Monitoring and analytics are crucial for understanding the performance, usage, and health of your API. Implement monitoring and analytics tools to gain insights into API metrics and behavior. Some key aspects to monitor include:
- Response Time: Track the response time of API requests to identify performance bottlenecks and optimize API performance.
- Error Rates: Monitor the number and frequency of API errors to proactively identify and resolve issues.
- Request Volume: Measure the number of API requests over time to understand usage patterns and plan for scalability.
- Latency: Monitor the latency between the client and server to ensure acceptable response times and identify network issues.
- Usage Metrics: Track usage metrics such as the number of API calls per client, most frequently accessed endpoints, or geographical distribution of requests.
Use the collected data to make data-driven decisions, optimize API performance, and improve the overall developer experience.
Best Practices and Tips
Here are some additional best practices and tips to consider when designing APIs:
-
Use Meaningful HTTP Status Codes: Leverage appropriate HTTP status codes to convey the outcome of API requests, such as 200 for success, 400 for client errors, or 500 for server errors. Use specific status codes to provide more granular information about the response.
-
Pagination and Filtering: Implement pagination and filtering mechanisms to handle large datasets efficiently. Allow clients to specify the page size, page number, and filter criteria to retrieve subsets of data.
-
Caching: Utilize caching mechanisms to improve API performance and reduce the load on the server. Implement client-side caching using HTTP caching headers or server-side caching using techniques like Redis or Memcached.
-
Idempotency: Design API endpoints to be idempotent, meaning that multiple identical requests should have the same effect as a single request. This helps prevent unintended side effects and ensures data consistency.
-
Error Handling and Logging: Implement robust error handling and logging mechanisms to capture and diagnose issues effectively. Provide meaningful error messages and include relevant details in error responses to assist with troubleshooting.
-
API Webhooks: Consider implementing webhooks to enable real-time notifications and event-driven communication between systems. Webhooks allow clients to subscribe to specific events and receive notifications when those events occur.
-
API Versioning and Deprecation: Plan for API versioning and deprecation from the beginning. Establish a clear versioning strategy and communicate deprecation policies to clients. Provide sufficient notice and support during the transition period.
FAQ
-
What is the difference between an API and a web service?
An API is a broader term that encompasses various types of interfaces for communication between software components. A web service is a specific type of API that uses web technologies (such as HTTP and XML/JSON) to enable communication over a network. -
How do I choose the right authentication mechanism for my API?
The choice of authentication mechanism depends on the security requirements and the nature of your API. For public APIs, API keys or OAuth tokens are commonly used. For internal or sensitive APIs, more robust mechanisms like JWT or two-factor authentication may be appropriate. Consider factors like ease of use, scalability, and the level of security needed. -
What is the purpose of API versioning?
API versioning allows you to manage the evolution of your API over time. It enables you to introduce changes, additions, or deprecations to the API without breaking existing integrations. Versioning helps maintain backward compatibility and allows clients to migrate to newer versions at their own pace. -
How can I ensure the scalability of my API?
To ensure the scalability of your API, consider factors such as performance optimization, load balancing, caching, and horizontal scaling. Use techniques like pagination and filtering to handle large datasets efficiently. Monitor API performance and resource utilization to identify bottlenecks and optimize accordingly. -
What should I include in API documentation?
API documentation should provide a comprehensive overview of your API, including endpoints, request/response formats, authentication mechanisms, and error handling. Include code samples, example requests and responses, and clear explanations of each endpoint’s functionality. Provide guidelines on getting started, versioning, and any specific usage instructions.
Conclusion
Designing a schematic API involves careful consideration of objects, interfaces, security, documentation, and best practices. By following the guidelines and patterns discussed in this article, you can create APIs that are scalable, maintainable, and developer-friendly.
Remember to focus on clarity, consistency, and usability when designing your API. Provide comprehensive documentation, implement robust security measures, and leverage monitoring and analytics to gain insights into API performance and usage.
Continuously iterate and improve your API based on feedback from developers and evolving business requirements. Stay updated with the latest industry trends and best practices to ensure your API remains competitive and aligned with modern development practices.
By investing time and effort into schematic API design, you can build APIs that empower developers, streamline integration, and drive the success of your software applications.
Feature | Description |
---|---|
Objects | Represent core entities and resources in the API |
Interfaces | Define the contract for interacting with the API |
Versioning | Manage the evolution of the API over time |
Authentication | Verify the identity of API clients |
Documentation | Provide comprehensive guidelines for using the API |
Monitoring and Analytics | Track API performance, usage, and health |
By incorporating these key features and following best practices, you can create robust, user-friendly, and maintainable APIs that drive the success of your software applications.
No responses yet