What are API Interfaces?
An API Interface is a contract between a client and a server that specifies how they should interact with each other. It defines the methods, data formats, and protocols that the client and server use to communicate and exchange data. API Interfaces provide a layer of abstraction that allows developers to create applications that can seamlessly integrate with other systems, without having to understand the underlying implementation details.
There are several types of API Interfaces, including:
- RESTful APIs
- SOAP APIs
- GraphQL APIs
- WebSocket APIs
Each type of API Interface has its own set of characteristics, advantages, and use cases. In this article, we will focus on RESTful APIs, which are the most commonly used type of API Interface in modern web development.
RESTful API Interfaces
RESTful APIs, also known as RESTful Web Services, are based on the Representational State Transfer (REST) architectural style. REST is a set of principles and constraints that define how web-based systems should be designed and implemented. RESTful APIs use HTTP methods (GET, POST, PUT, DELETE) to perform operations on resources, which are identified by URLs.
Key Principles of RESTful API Interfaces
-
Client-Server Architecture: RESTful APIs follow a client-server architecture, where the client and server are separate entities that communicate over a network. The client sends requests to the server, and the server responds with the requested data or performs the requested action.
-
Statelessness: RESTful APIs are stateless, which means that each request from the client to the server must contain all the necessary information to understand and process the request. The server does not retain any client context between requests, making the API more scalable and easier to maintain.
-
Cacheability: RESTful APIs should support caching to improve performance and reduce network traffic. The server can include caching information in the response headers, such as Cache-Control and ETag, to indicate how long the response can be cached by the client or intermediate proxies.
-
Uniform Interface: RESTful APIs provide a uniform interface for interacting with resources. This includes using standard HTTP methods (GET, POST, PUT, DELETE) for CRUD operations, using meaningful URLs to identify resources, and using hypermedia links to enable discoverability and navigation between related resources.
-
Layered System: RESTful APIs can be composed of multiple layers, such as load balancers, caching servers, or security gateways, without affecting the client-server interaction. Each layer has a specific responsibility and can evolve independently, making the system more flexible and maintainable.
Designing RESTful API Interfaces
When designing RESTful API Interfaces, there are several best practices to follow:
-
Use HTTP Methods Appropriately: Use the appropriate HTTP methods for different operations on resources. For example, use GET for retrieving data, POST for creating new resources, PUT for updating existing resources, and DELETE for removing resources.
-
Use Meaningful URLs: Use URLs that clearly identify the resources being accessed or manipulated. For example,
/users
for a collection of users,/users/{id}
for a specific user, and/users/{id}/orders
for the orders of a specific user. -
Use Proper HTTP Status Codes: Use appropriate HTTP status codes in the server responses to indicate the outcome of the request. For example, use 200 OK for successful requests, 201 Created for successfully created resources, 400 Bad Request for invalid requests, and 404 Not Found for non-existent resources.
-
Use Versioning: Include a version number in the API URL or headers to manage the evolution of the API over time. This allows clients to specify the version they are compatible with and enables the server to support multiple versions simultaneously.
-
Use Pagination: For large collections of resources, use pagination to limit the amount of data returned in a single response. Include pagination metadata in the response, such as the total number of resources, the current page, and links to the next and previous pages.
-
Use Error Handling: Provide meaningful error messages and error codes in the server responses when something goes wrong. Include details about the error, such as the error type, message, and any additional information that can help the client understand and resolve the issue.
-
Use Security Measures: Implement appropriate security measures to protect the API and the data it exposes. This includes using HTTPS for encrypted communication, requiring authentication and authorization for accessing protected resources, and validating and sanitizing user input to prevent security vulnerabilities.
Here’s an example of a RESTful API Interface for managing users:
HTTP Method | URL | Description |
---|---|---|
GET | /users |
Retrieve a list of all users |
GET | /users/{id} |
Retrieve a specific user by ID |
POST | /users |
Create a new user |
PUT | /users/{id} |
Update an existing user by ID |
DELETE | /users/{id} |
Delete a user by ID |
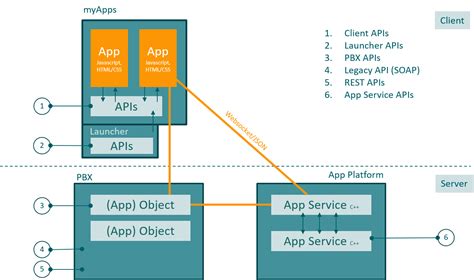
API Documentation
API documentation is essential for developers to understand how to use an API effectively. It provides information about the available endpoints, request and response formats, authentication requirements, and any limitations or constraints.
Good API documentation should include:
-
Overview: A high-level description of the API, its purpose, and the services it provides.
-
Authentication: Instructions on how to authenticate and obtain access tokens to use the API.
-
Endpoints: A list of all available endpoints, including their URLs, HTTP methods, request parameters, and response formats.
-
Request and Response Examples: Sample requests and responses for each endpoint, illustrating how to use the API and what to expect in return.
-
Error Handling: Information about the possible error codes and their meanings, along with examples of error responses.
-
Versioning: Details about the API versioning scheme and how to specify the desired version in requests.
-
SDKs and Libraries: Links to any official SDKs or libraries that simplify the integration of the API into different programming languages or frameworks.
API documentation can be provided in various formats, such as OpenAPI (formerly Swagger) specifications, API blueprints, or custom documentation websites. It’s important to keep the documentation up to date as the API evolves over time.
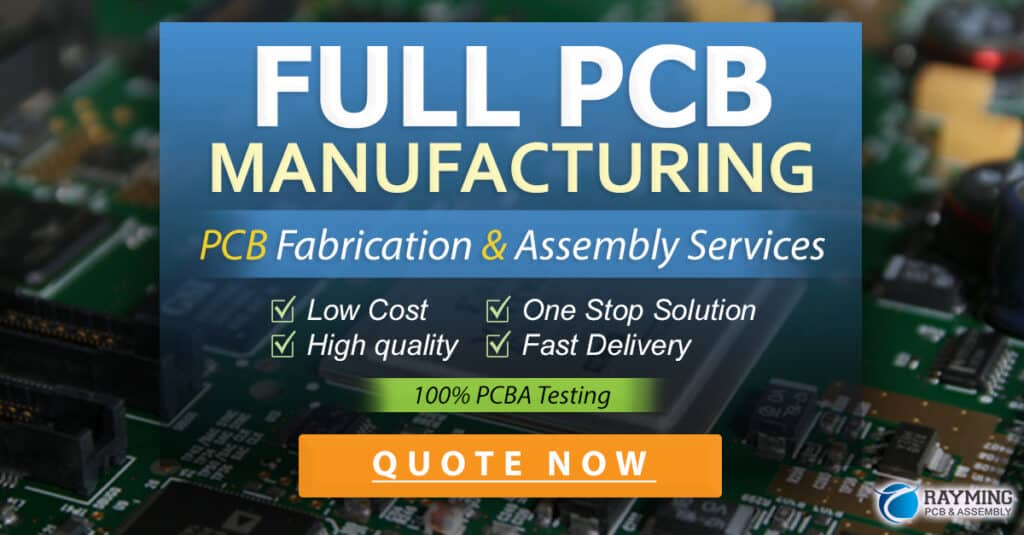
API Testing
API testing is crucial to ensure the reliability, functionality, and performance of an API. It involves validating the API endpoints, request and response formats, error handling, and edge cases.
There are different types of API tests, including:
-
Unit Testing: Testing individual API components or functions in isolation to verify their correctness.
-
Integration Testing: Testing the integration between different API components or with external systems to ensure they work together as expected.
-
Functional Testing: Testing the API endpoints with various input parameters and scenarios to validate the expected behavior and response formats.
-
Performance Testing: Measuring the API’s performance under different load conditions to identify any bottlenecks or scalability issues.
-
Security Testing: Assessing the API’s security measures, such as authentication, authorization, and input validation, to identify and mitigate any vulnerabilities.
API testing can be performed manually using tools like Postman or curl, or automated using frameworks like JUnit, pytest, or Postman’s automated testing features. It’s important to have a comprehensive test suite that covers all the essential scenarios and edge cases to ensure the API’s reliability and maintainability.
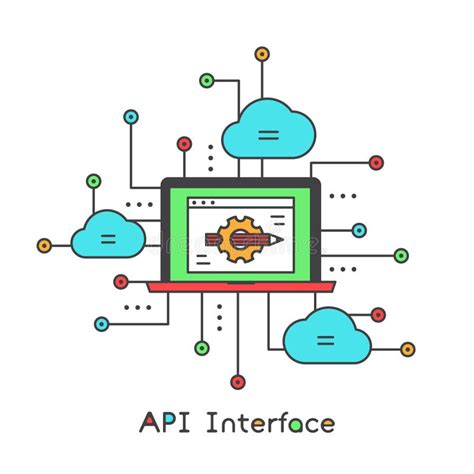
API Monitoring
API monitoring is the process of continuously observing and tracking the performance, availability, and usage of an API in production environments. It helps identify and resolve issues quickly, optimize performance, and ensure a smooth user experience.
API monitoring typically involves:
-
Logging: Capturing and storing API requests and responses, along with relevant metadata, for troubleshooting and analysis.
-
Metrics: Collecting and aggregating metrics such as response times, error rates, and throughput to monitor the API’s performance and health.
-
Alerts: Setting up alerts and notifications based on predefined thresholds or anomalies to proactively detect and respond to issues.
-
Dashboards: Providing real-time visualizations and reports of the API’s performance, usage, and trends for monitoring and decision-making.
API monitoring tools like Prometheus, Grafana, ELK stack (Elasticsearch, Logstash, Kibana), or cloud-based services like Amazon CloudWatch or Google Cloud Monitoring can help streamline the monitoring process and provide valuable insights into the API’s behavior.
FAQ
- What is the difference between an API and an API Interface?
-
An API (Application Programming Interface) is a set of rules, protocols, and tools that define how software components should interact with each other. An API Interface is the specific contract or agreement between a client and a server that specifies how they should communicate and exchange data using the API.
-
What are the advantages of using RESTful API Interfaces?
-
RESTful API Interfaces offer several advantages, including:
- Simplicity and ease of use, as they leverage standard HTTP methods and protocols.
- Scalability and performance, as they are stateless and cacheable.
- Flexibility and interoperability, as they provide a uniform interface for accessing resources.
- Wide support and tooling, as REST is a widely adopted architectural style.
-
How do I secure my API Interfaces?
-
To secure your API Interfaces, you can:
- Use HTTPS for encrypted communication to protect data in transit.
- Implement authentication mechanisms, such as API keys, OAuth, or JWT tokens, to verify the identity of clients.
- Enforce authorization rules to control access to specific resources based on user roles or permissions.
- Validate and sanitize user input to prevent common security vulnerabilities like SQL injection or cross-site scripting (XSS).
- Limit the rate of API requests to prevent abuse or denial-of-service attacks.
-
What tools can I use for API testing and monitoring?
- For API testing, you can use tools like:
- Postman: A popular GUI tool for manually testing APIs and creating automated test suites.
- JUnit, pytest, or other testing frameworks for writing automated API tests in various programming languages.
- Swagger or OpenAPI tools for generating API documentation and client SDKs.
-
For API monitoring, you can use tools like:
- Prometheus and Grafana: Open-source tools for collecting metrics, visualizing data, and setting up alerts.
- ELK stack (Elasticsearch, Logstash, Kibana): A suite of tools for logging, analyzing, and visualizing API data.
- Cloud-based monitoring services like Amazon CloudWatch or Google Cloud Monitoring.
-
How do I version my API Interfaces?
- API versioning is important to manage the evolution of your API over time. You can version your API Interfaces by:
- Including a version number in the API URL, such as
/v1/users
or/v2/products
. - Using version-specific headers, such as
API-Version: 1.0
orX-API-Version: 2.0
. - Providing different endpoints or subdomains for different versions, such as
https://api.example.com/v1
andhttps://api.example.com/v2
.
- Including a version number in the API URL, such as
- It’s crucial to communicate version changes to API clients, provide backward compatibility when possible, and deprecate older versions gracefully with sufficient notice.
System API Client Server Interfaces are essential for building modern, interconnected software systems. By following best practices for designing, documenting, testing, and monitoring API Interfaces, developers can create robust, scalable, and maintainable APIs that enable seamless integration and communication between different applications and services.
No responses yet