Introduction to the Wio Terminal
The Wio Terminal is a revolutionary all-in-one Arduino compatible development board that combines a powerful microcontroller, a high-resolution LCD screen, wireless connectivity, and a wide range of sensors and interfaces. This compact device is designed to simplify the process of creating interactive projects, making it an ideal choice for makers, hobbyists, and professionals alike.
Key Features of the Wio Terminal
-
Microcontroller: The Wio Terminal is powered by a SAMD51 microcontroller, which is based on the ARM Cortex-M4F core. This 32-bit processor runs at 120MHz and offers 256KB of RAM and 512KB of flash memory, providing ample resources for complex projects.
-
Display: The device features a vibrant 2.4-inch LCD screen with a resolution of 320×240 pixels. The display supports touch input, enabling intuitive user interaction and opening up possibilities for creating graphical user interfaces (GUIs) and interactive applications.
-
Wireless Connectivity: The Wio Terminal comes equipped with both Wi-Fi (802.11 b/g/n) and Bluetooth 5.0 connectivity. This allows the device to communicate with other devices, access the internet, and integrate with IoT platforms, expanding its capabilities beyond standalone applications.
-
Sensors and Interfaces: The Wio Terminal offers a wide array of built-in sensors and interfaces, including:
- 3-axis accelerometer
- 3-axis gyroscope
- Light sensor
- Microphone
- Buzzer
- microSD card slot
- USB Type-C port
- 40-pin Raspberry Pi compatible GPIO header
These features enable developers to create projects that involve motion sensing, audio processing, data logging, and more, without the need for additional modules or components.
- Arduino Compatibility: One of the key advantages of the Wio Terminal is its compatibility with the Arduino ecosystem. The device can be programmed using the Arduino IDE, and it supports a wide range of Arduino libraries and shields. This compatibility allows developers to leverage the vast resources and community support available within the Arduino ecosystem.
Getting Started with the Wio Terminal
Setting Up the Development Environment
To start developing projects with the Wio Terminal, you’ll need to set up the Arduino IDE and install the necessary libraries and board support package. Follow these steps:
-
Download and install the Arduino IDE from the official website: https://www.arduino.cc/en/software
-
Open the Arduino IDE and navigate to File -> Preferences.
-
In the “Additional Boards Manager URLs” field, add the following URL: https://files.seeedstudio.com/arduino/package_seeeduino_boards_index.json
-
Click “OK” to save the preferences.
-
Go to Tools -> Board -> Boards Manager.
-
Search for “Seeed SAMD Boards” and install the package.
-
Once the installation is complete, you can select the Wio Terminal from the list of boards under Tools -> Board.
Programming the Wio Terminal
With the development environment set up, you can start programming the Wio Terminal using the Arduino IDE. Here’s a simple example that demonstrates how to display text on the LCD screen:
#include <TFT_eSPI.h>
TFT_eSPI tft = TFT_eSPI();
void setup() {
tft.begin();
tft.setRotation(3);
tft.fillScreen(TFT_BLACK);
tft.setTextColor(TFT_WHITE);
tft.setTextSize(2);
}
void loop() {
tft.drawString("Hello, Wio Terminal!", 20, 100);
delay(1000);
tft.fillScreen(TFT_BLACK);
delay(1000);
}
In this example, we include the TFT_eSPI
library, which provides functions for controlling the LCD screen. The setup()
function initializes the display, sets the rotation, clears the screen, and configures the text color and size. The loop()
function repeatedly displays the message “Hello, Wio Terminal!” on the screen and clears it after a 1-second delay.
To upload the code to the Wio Terminal, follow these steps:
-
Connect the Wio Terminal to your computer using a USB Type-C cable.
-
In the Arduino IDE, select the appropriate board and port under the Tools menu.
-
Click the “Upload” button to compile the code and upload it to the Wio Terminal.
Once the upload is complete, you should see the message “Hello, Wio Terminal!” displayed on the LCD screen.
Exploring the Wio Terminal’s Capabilities
Utilizing the Built-in Sensors
One of the key advantages of the Wio Terminal is its array of built-in sensors, which allow you to create projects that respond to various environmental factors and user inputs. Let’s explore a few examples:
Accelerometer and Gyroscope
The Wio Terminal’s 3-axis accelerometer and gyroscope enable motion sensing applications, such as tilt-based controls or gesture recognition. Here’s an example that demonstrates how to read the accelerometer data:
#include <Seeed_LSM6DS3.h>
LSM6DS3 imu(I2C_MODE, 0x6A);
void setup() {
Serial.begin(9600);
imu.begin();
}
void loop() {
float x, y, z;
imu.getAcceleration(&x, &y, &z);
Serial.print("Acceleration: ");
Serial.print("X = ");
Serial.print(x);
Serial.print(", Y = ");
Serial.print(y);
Serial.print(", Z = ");
Serial.println(z);
delay(500);
}
In this example, we use the Seeed_LSM6DS3
library to read the accelerometer data. The setup()
function initializes the IMU sensor, and the loop()
function repeatedly reads the acceleration values along the X, Y, and Z axes and prints them to the serial monitor.
Light Sensor
The Wio Terminal’s built-in light sensor can be used to create projects that respond to ambient light levels, such as automatic screen brightness adjustment or light-triggered events. Here’s an example that demonstrates how to read the light sensor data:
void setup() {
Serial.begin(9600);
}
void loop() {
int lightLevel = analogRead(WIO_LIGHT);
Serial.print("Light level: ");
Serial.println(lightLevel);
delay(500);
}
In this example, we use the analogRead()
function to read the light sensor value from the WIO_LIGHT
pin. The loop()
function repeatedly reads the light level and prints it to the serial monitor.
Creating Graphical User Interfaces (GUIs)
The Wio Terminal’s LCD screen and touch input capabilities make it an ideal platform for creating graphical user interfaces (GUIs). The TFT_eSPI
library provides a wide range of functions for drawing shapes, displaying text, and handling touch events. Let’s explore an example that demonstrates how to create a simple GUI:
#include <TFT_eSPI.h>
TFT_eSPI tft = TFT_eSPI();
void setup() {
tft.begin();
tft.setRotation(3);
tft.fillScreen(TFT_BLACK);
tft.setTextColor(TFT_WHITE);
tft.setTextSize(2);
// Draw a button
tft.fillRect(100, 100, 120, 40, TFT_BLUE);
tft.drawString("Button", 110, 110);
}
void loop() {
if (tft.getTouch(&x, &y)) {
if (x >= 100 && x <= 220 && y >= 100 && y <= 140) {
tft.fillScreen(TFT_BLACK);
tft.drawString("Button Pressed!", 50, 100);
delay(1000);
setup();
}
}
}
In this example, we create a simple GUI with a button. The setup()
function initializes the display, clears the screen, and draws a blue rectangle with the text “Button” inside it. The loop()
function continuously checks for touch events using the getTouch()
function. If a touch is detected within the button area, the screen is cleared, and the message “Button Pressed!” is displayed for 1 second before redrawing the button.
This is just a basic example, but the possibilities for creating GUIs on the Wio Terminal are virtually endless. You can create more complex layouts, add multiple buttons, display images, and implement various types of user interactions.
Wireless Connectivity and IoT Integration
The Wio Terminal’s built-in Wi-Fi and Bluetooth connectivity open up a world of possibilities for creating connected projects and integrating with IoT platforms. Let’s explore a few examples:
Connecting to Wi-Fi Networks
To connect the Wio Terminal to a Wi-Fi network, you can use the rpcWiFi
library. Here’s an example that demonstrates how to connect to a Wi-Fi network and print the device’s IP address:
#include <rpcWiFi.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
void setup() {
Serial.begin(9600);
while (!Serial);
WiFi.begin(ssid, password);
Serial.println("Connecting to WiFi...");
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting...");
}
Serial.println("Connected to WiFi!");
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
}
void loop() {
// Your code here
}
In this example, we include the rpcWiFi
library and define the Wi-Fi network’s SSID and password. The setup()
function initializes the serial communication and attempts to connect to the Wi-Fi network. Once connected, it prints the device’s IP address to the serial monitor.
MQTT Communication
MQTT (Message Queuing Telemetry Transport) is a lightweight messaging protocol commonly used in IoT applications. The Wio Terminal can easily integrate with MQTT brokers to send and receive data. Here’s an example that demonstrates how to publish and subscribe to MQTT topics:
#include <rpcWiFi.h>
#include <PubSubClient.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const char* mqttServer = "your_MQTT_broker_IP";
WiFiClient wioClient;
PubSubClient client(wioClient);
void callback(char* topic, byte* payload, unsigned int length) {
Serial.print("Message received [");
Serial.print(topic);
Serial.print("] ");
for (int i = 0; i < length; i++) {
Serial.print((char)payload[i]);
}
Serial.println();
}
void reconnect() {
while (!client.connected()) {
Serial.print("Attempting MQTT connection...");
if (client.connect("WioTerminal")) {
Serial.println("connected");
client.subscribe("wio/input");
} else {
Serial.print("failed, rc=");
Serial.print(client.state());
Serial.println(" try again in 5 seconds");
delay(5000);
}
}
}
void setup() {
Serial.begin(9600);
while (!Serial);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
client.setServer(mqttServer, 1883);
client.setCallback(callback);
}
void loop() {
if (!client.connected()) {
reconnect();
}
client.loop();
client.publish("wio/output", "Hello from Wio Terminal!");
delay(5000);
}
In this example, we include the rpcWiFi
and PubSubClient
libraries. The setup()
function connects to the Wi-Fi network and sets up the MQTT client. The callback()
function is called when a message is received on the subscribed topic. The reconnect()
function ensures that the MQTT connection is maintained. The loop()
function publishes a message to the “wio/output” topic every 5 seconds and handles incoming messages on the “wio/input” topic.
This is just a basic example of MQTT communication, but you can expand on this to create more complex IoT applications, such as sensor data logging, remote control, or integration with cloud platforms like AWS IoT or Google Cloud IoT.
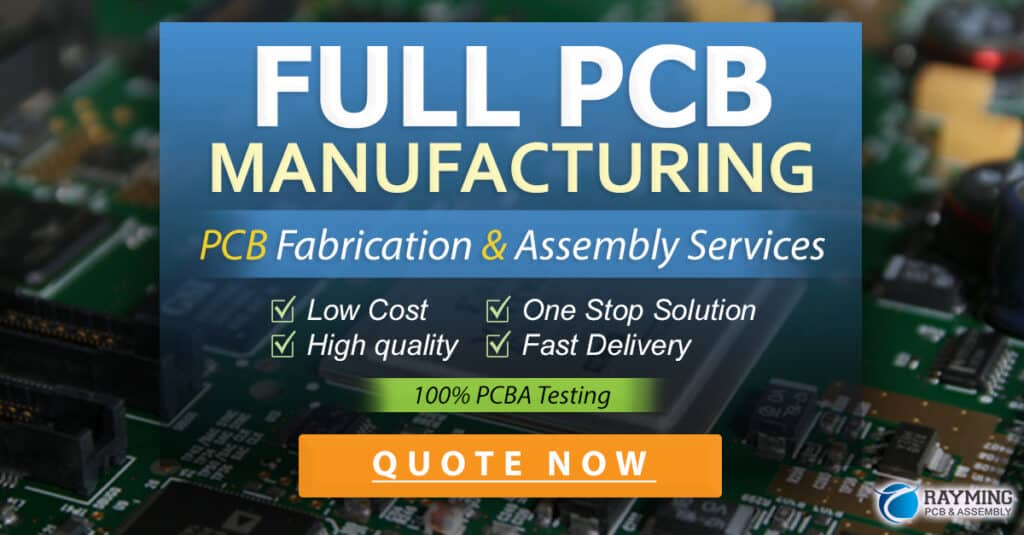
FAQ
-
What programming languages can be used with the Wio Terminal?
The Wio Terminal is primarily programmed using the Arduino framework, which is based on C++. However, it also supports other programming languages, such as MicroPython, through the use of compatible libraries and toolchains. -
Can I use Arduino libraries with the Wio Terminal?
Yes, the Wio Terminal is compatible with a wide range of Arduino libraries. You can use existing libraries or create your own to extend the functionality of the device. However, keep in mind that some libraries may require modifications to work with the Wio Terminal’s specific hardware configuration. -
How do I update the firmware on the Wio Terminal?
To update the firmware on the Wio Terminal, you can use the Arduino IDE. First, ensure that you have the latest version of the Seeed SAMD Boards package installed. Then, connect the Wio Terminal to your computer, select the appropriate board and port in the Arduino IDE, and upload the new firmware using the “Upload” button. -
Can I connect external sensors and actuators to the Wio Terminal?
Yes, the Wio Terminal features a 40-pin Raspberry Pi compatible GPIO header, which allows you to connect various external sensors, actuators, and modules. You can use the built-in interfaces, such as I2C, SPI, and UART, to communicate with these devices. Additionally, the Wio Terminal’s 5V and 3.3V power pins can be used to power external components. -
Is the Wio Terminal suitable for battery-powered projects?
Yes, the Wio Terminal can be powered using a lithium-ion battery (3.7V) connected to the device’s JST battery connector. The onboard battery management system ensures safe charging and discharging of the battery. When running on battery power, you can optimize your code to minimize power consumption by utilizing sleep modes and reducing the use of power-hungry features like the LCD screen and wireless connectivity when not needed.
Conclusion
The Wio Terminal is a versatile and powerful all-in-one Arduino compatible development board that offers a wide range of features and capabilities. Its combination of a high-performance microcontroller, integrated sensors, wireless connectivity, and a user-friendly display makes it an ideal platform for creating interactive projects and IoT applications.
Throughout this article, we explored various aspects of the Wio Terminal, including its hardware specifications, setting up the development environment, programming examples, and utilizing its built-in features like sensors, GUI creation, and wireless connectivity. We also discussed how the Wio Terminal can be integrated with IoT platforms and protocols, such as MQTT, to create connected applications.
The Wio Terminal’s compatibility with the Arduino ecosystem and its extensive library support make it accessible to both beginners and experienced developers. Its compact form factor and comprehensive feature set enable rapid prototyping and development of a wide range of projects, from simple sensor-based applications to complex IoT solutions.
As the IoT landscape continues to evolve, the Wio Terminal is well-positioned to meet the growing demand for flexible, high-performance, and user-friendly development platforms. Its combination of hardware features, software compatibility, and ease of use make it a compelling choice for makers, hobbyists, and professionals alike.
In conclusion, the Wio Terminal is a powerful and versatile all-in-one Arduino compatible device that offers endless possibilities for creating innovative projects and IoT applications. With its rich feature set, extensive library support, and user-friendly design, the Wio Terminal is an excellent choice for anyone looking to explore the world of IoT and interactive project development.
No responses yet