What are Void Pointers?
A void pointer, denoted as void*
, is a special type of pointer that can point to any data type. Unlike regular pointers that are specific to a particular data type (e.g., int*
, char*
), a void pointer can hold the address of any object, regardless of its type.
The key characteristics of void pointers are:
– They can point to any data type
– They don’t have any associated type information
– They cannot be dereferenced directly
– They require explicit casting to access the pointed-to data
Here’s an example of declaring a void pointer:
void* ptr;
In this declaration, ptr
is a void pointer that can hold the address of any object.
Why Use Void Pointers?
Void pointers offer several benefits and use cases in C programming:
-
Generic Programming: Void pointers enable you to write generic functions that can work with different data types. By accepting void pointers as parameters, a function can handle any type of data passed to it.
-
Memory Management: Void pointers are commonly used in memory allocation and deallocation functions, such as
malloc()
andfree()
. These functions return a void pointer to the allocated memory block, allowing flexibility in how the memory is used. -
Opaque Pointers: Void pointers are often used to create opaque pointers, which hide the internal details of a data structure or object. This encapsulation technique allows you to expose only necessary functionality to the user while keeping the implementation details private.
-
Callback Functions: Void pointers are frequently used in callback functions, where a generic pointer is passed as an argument to represent any data that the callback function may need to access.
-
Data Structures: Void pointers can be used to create generic data structures that can hold different types of data. For example, a linked list or a binary tree can use void pointers to store nodes containing various data types.
How to Use Void Pointers
Using void pointers involves three main steps:
- Declaring a Void Pointer: Declare a void pointer using the
void*
syntax.
c
void* ptr;
- Assigning an Address: Assign the address of an object to the void pointer. This can be done using the address-of operator (
&
).
c
int num = 10;
ptr = #
In this example, the address of num
is assigned to the void pointer ptr
.
- Dereferencing with Casting: To access the value pointed to by a void pointer, you need to explicitly cast it to the appropriate data type.
c
int* int_ptr = (int*)ptr;
printf("Value: %d\n", *int_ptr);
Here, ptr
is cast to an int*
pointer, allowing you to dereference it and access the integer value.
It’s important to note that when dereferencing a void pointer, you must cast it to the correct data type. Failing to do so can lead to undefined behavior and potential memory corruption.
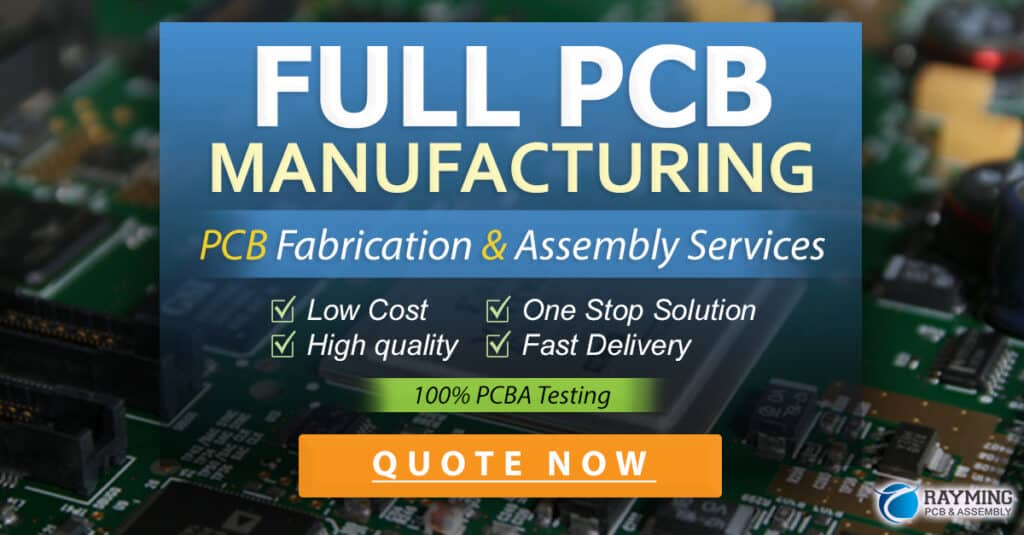
Practical Examples
Let’s explore some practical examples that demonstrate the power and flexibility of void pointers.
Example 1: Generic Swap Function
Void pointers can be used to create generic functions that work with different data types. Here’s an example of a generic swap function that swaps the values of two variables:
void swap(void* a, void* b, size_t size) {
char temp[size];
memcpy(temp, a, size);
memcpy(a, b, size);
memcpy(b, temp, size);
}
In this function:
– a
and b
are void pointers representing the addresses of the variables to be swapped.
– size
is the size of the data type in bytes.
– temp
is a temporary array used for swapping the values.
– memcpy()
is used to copy the values between the variables and the temporary array.
Here’s an example of using the swap()
function:
int x = 10;
int y = 20;
printf("Before swap: x = %d, y = %d\n", x, y);
swap(&x, &y, sizeof(int));
printf("After swap: x = %d, y = %d\n", x, y);
Output:
Before swap: x = 10, y = 20
After swap: x = 20, y = 10
The swap()
function can work with any data type by passing the appropriate size using sizeof()
.
Example 2: Opaque Pointer
Void pointers can be used to create opaque pointers that hide the internal details of a data structure. Here’s an example of an opaque pointer implementation for a simple stack:
typedef struct {
void* data;
int size;
int top;
} Stack;
Stack* create_stack(int size) {
Stack* stack = (Stack*)malloc(sizeof(Stack));
stack->data = malloc(size * sizeof(int));
stack->size = size;
stack->top = -1;
return stack;
}
void push(Stack* stack, int value) {
if (stack->top < stack->size - 1) {
int* data = (int*)stack->data;
data[++stack->top] = value;
}
}
int pop(Stack* stack) {
if (stack->top >= 0) {
int* data = (int*)stack->data;
return data[stack->top--];
}
return -1;
}
void destroy_stack(Stack* stack) {
free(stack->data);
free(stack);
}
In this example:
– Stack
is a structure representing a stack, with a void pointer data
to hold the stack elements.
– create_stack()
creates a new stack with a specified size and returns a pointer to it.
– push()
adds an element to the top of the stack.
– pop()
removes and returns the top element from the stack.
– destroy_stack()
frees the memory allocated for the stack.
The void pointer data
in the Stack
structure allows the stack to store elements of any data type. The actual data type is determined by the user when using the stack.
Here’s an example of using the opaque stack:
Stack* stack = create_stack(5);
push(stack, 10);
push(stack, 20);
push(stack, 30);
printf("Popped element: %d\n", pop(stack));
printf("Popped element: %d\n", pop(stack));
destroy_stack(stack);
Output:
Popped element: 30
Popped element: 20
The opaque pointer implementation hides the internal details of the stack and provides a clean interface for the user to interact with.
Example 3: Callback Function
Void pointers are commonly used in callback functions to allow generic arguments. Here’s an example of a callback function that compares two integers:
int compare_ints(const void* a, const void* b) {
const int* x = (const int*)a;
const int* y = (const int*)b;
return (*x - *y);
}
In this callback function:
– a
and b
are void pointers representing the addresses of the integers to be compared.
– The void pointers are cast to const int*
to access the integer values.
– The function returns the difference between the two integers.
Here’s an example of using the compare_ints()
callback function with the qsort()
standard library function to sort an array of integers:
int arr[] = {5, 2, 9, 1, 7};
int size = sizeof(arr) / sizeof(arr[0]);
qsort(arr, size, sizeof(int), compare_ints);
printf("Sorted array: ");
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
Output:
Sorted array: 1 2 5 7 9
The qsort()
function uses the compare_ints()
callback to compare the elements of the array during the sorting process. The void pointers allow the callback function to work with any data type.
Frequently Asked Questions (FAQ)
- What is the difference between a void pointer and a regular pointer?
- A void pointer can hold the address of any data type, while a regular pointer is specific to a particular data type.
- Void pointers don’t have any associated type information, whereas regular pointers know the type of data they point to.
-
Void pointers cannot be dereferenced directly and require explicit casting, while regular pointers can be dereferenced directly.
-
Can a void pointer be dereferenced directly?
-
No, a void pointer cannot be dereferenced directly. You must explicitly cast it to the appropriate data type before dereferencing.
-
How do you cast a void pointer to a specific data type?
- To cast a void pointer to a specific data type, use the casting operator
()
followed by the desired data type and an asterisk*
. -
For example, to cast a void pointer
ptr
to an integer pointer, use(int*)ptr
. -
Can you perform arithmetic operations on void pointers?
- No, arithmetic operations cannot be directly performed on void pointers because they lack type information.
-
To perform arithmetic on a void pointer, you need to first cast it to a specific data type and then perform the desired operation.
-
Are there any limitations or potential issues with using void pointers?
- Void pointers can lead to code that is harder to read and maintain if not used carefully.
- Improper casting of void pointers can result in undefined behavior and memory corruption.
- Void pointers do not provide type safety, so it’s the programmer’s responsibility to ensure the correct usage and casting.
Conclusion
Void pointers are a powerful feature in C that offer flexibility and versatility in programming. They allow you to write generic code, create opaque pointers, handle memory allocation, and work with callback functions effectively.
By understanding how to declare, assign, and dereference void pointers, you can leverage their capabilities to write more reusable and adaptable code. However, it’s crucial to use void pointers responsibly and ensure proper casting to avoid potential issues.
Incorporating void pointers into your C programming toolkit will expand your problem-solving abilities and enable you to tackle a wider range of programming challenges. Embrace the power of void pointers and unlock new possibilities in your coding experience!
Summary Table
Concept | Description |
---|---|
Void Pointer | A special type of pointer that can point to any data type |
Declaring a Void Pointer | Use the void* syntax to declare a void pointer |
Assigning an Address | Assign the address of an object to the void pointer using the address-of operator & |
Dereferencing with Casting | Explicitly cast the void pointer to the appropriate data type before dereferencing |
Generic Programming | Use void pointers to write generic functions that can work with different data types |
Opaque Pointers | Create opaque pointers with void pointers to hide the internal details of a data structure |
Callback Functions | Pass void pointers as arguments to callback functions for generic data access |
Remember, void pointers are a powerful tool, but they should be used judiciously and with proper care to ensure code correctness and maintainability.
Happy coding with void pointers!
No responses yet