Introduction to the PCB API
The PCB (Printed Circuit Board) API is a powerful tool that enables developers to automate and streamline the process of designing and manufacturing PCBs. With the PCB API, you can programmatically create, modify, and manage PCB designs, as well as integrate PCB design functionality into your own applications.
In this comprehensive guide, we’ll explore the key features and capabilities of the PCB API, providing you with the knowledge and tools to leverage its potential effectively. Whether you’re a seasoned PCB designer or a developer looking to incorporate PCB design into your projects, this article will equip you with the necessary information to get started with the PCB API.
Understanding the PCB Design Process
Before diving into the specifics of the PCB API, let’s briefly overview the PCB design process to understand where the API fits in.
PCB Design Workflow
-
Schematic Design: The first step in PCB design is creating a schematic diagram that represents the electrical connections and components of the circuit.
-
Component Placement: Once the schematic is complete, the components are placed on the PCB layout, considering factors such as signal integrity, thermal management, and manufacturability.
-
Routing: The connections between components are routed using copper traces, following the schematic diagram and adhering to design rules and constraints.
-
Design Rule Check (DRC): A DRC is performed to ensure that the PCB layout complies with the specified design rules, such as minimum trace widths, clearances, and via sizes.
-
Manufacturing Output: Finally, the PCB design is exported in various formats, such as Gerber files, drill files, and bill of materials (BOM), for manufacturing.
The PCB API allows you to automate and control various aspects of this workflow programmatically.
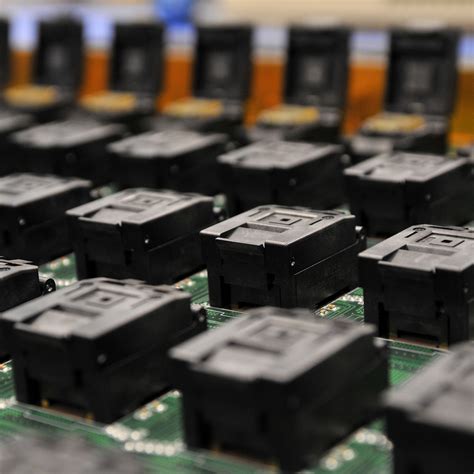
Key Features of the PCB API
Let’s explore some of the key features and capabilities offered by the PCB API.
1. Design Creation and Management
The PCB API provides functions and methods to create and manage PCB designs programmatically. You can create new PCB projects, add schematic sheets, and define the stackup and design rules.
from pcb_api import PCBProject
# Create a new PCB project
project = PCBProject("My PCB Project")
# Add a schematic sheet
schematic = project.add_schematic("Main Schematic")
# Define the stackup
stackup = project.set_stackup(layers=4, thickness=1.6)
# Set design rules
project.set_design_rules(min_trace_width=0.2, min_clearance=0.1)
2. Component Libraries and Management
The PCB API allows you to manage component libraries and create custom components. You can import existing component libraries, search for components, and assign them to your PCB design.
from pcb_api import ComponentLibrary
# Load a component library
library = ComponentLibrary("path/to/library.lib")
# Search for a specific component
component = library.find_component("RES0805")
# Assign the component to the schematic
schematic.add_component(component, "R1")
3. Placement and Routing
With the PCB API, you can automate the placement and routing of components on the PCB layout. You can specify placement constraints, define routing strategies, and optimize the layout for manufacturability.
from pcb_api import PCBLayout
# Create a PCB layout from the schematic
layout = PCBLayout(schematic)
# Place components
layout.place_component("R1", position=(10, 20), rotation=90)
layout.place_component("C1", position=(30, 40))
# Route connections
layout.route_connection("R1", "1", "C1", "2", width=0.2)
layout.route_connection("U1", "VCC", "C2", "1", width=0.3)
4. Design Rule Checking (DRC)
The PCB API provides functions to perform design rule checks on your PCB layout. You can define custom design rules, run DRC checks, and retrieve any violations for further analysis and resolution.
from pcb_api import DesignRuleCheck
# Define design rules
drc_rules = {
"min_trace_width": 0.2,
"min_clearance": 0.1,
"min_via_size": 0.4
}
# Create a DRC object
drc = DesignRuleCheck(layout, drc_rules)
# Run the DRC check
violations = drc.run()
# Analyze violations
for violation in violations:
print(violation)
5. Manufacturing Output Generation
Once your PCB design is complete and has passed the DRC checks, you can use the PCB API to generate manufacturing output files. This includes Gerber files, drill files, and bill of materials (BOM).
from pcb_api import ManufacturingOutput
# Create a manufacturing output object
output = ManufacturingOutput(layout)
# Generate Gerber files
output.generate_gerber_files("path/to/output/gerber")
# Generate drill files
output.generate_drill_files("path/to/output/drill")
# Generate BOM
bom = output.generate_bom()
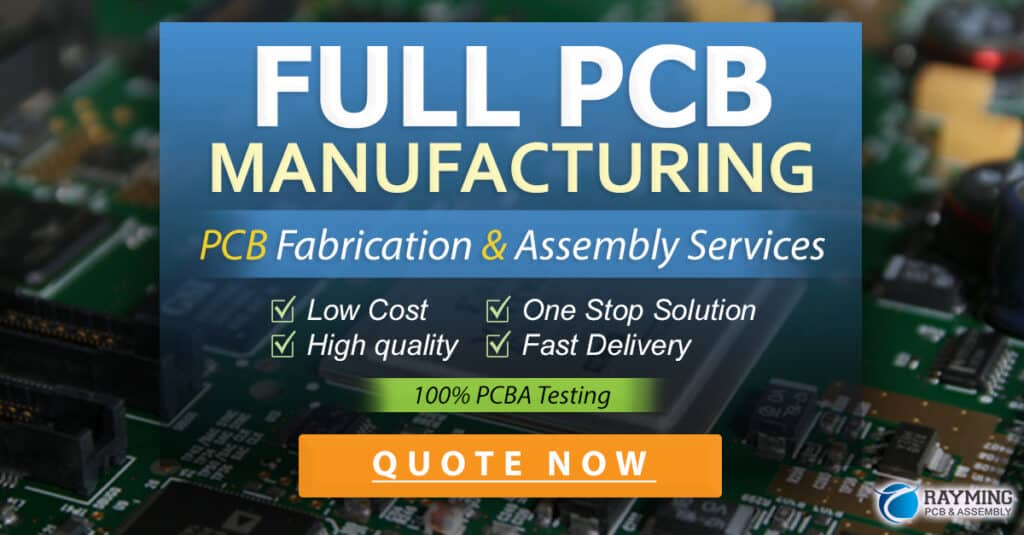
Integrating the PCB API into Your Workflow
Now that we’ve explored the key features of the PCB API, let’s discuss how you can integrate it into your existing workflow or build new applications around it.
Integration with Existing PCB Design Tools
Many popular PCB design tools, such as Altium Designer, KiCad, and Eagle, provide API support or scripting capabilities. You can leverage the PCB API to automate tasks, extend functionality, or create custom plugins for these tools.
For example, you can use the PCB API to:
– Import and export designs between different PCB design tools
– Automate repetitive tasks, such as component placement or routing
– Implement custom design rule checks or optimization algorithms
– Generate customized manufacturing output files
Building Custom PCB Design Applications
The PCB API also enables you to build custom PCB design applications from scratch. Whether you want to create a web-based PCB design tool, a mobile app for PCB layout, or a specialized tool for a specific industry, the PCB API provides the foundation for building powerful and flexible PCB design software.
Here are a few examples of custom PCB design applications you can build using the PCB API:
– A web-based PCB design tool that allows users to create and share PCB designs collaboratively
– A mobile app for on-the-go PCB layout and component placement
– An automated PCB design system that optimizes layouts based on specific criteria, such as signal integrity or thermal management
– A specialized PCB design tool for a specific industry, such as automotive or aerospace, with industry-specific design rules and requirements
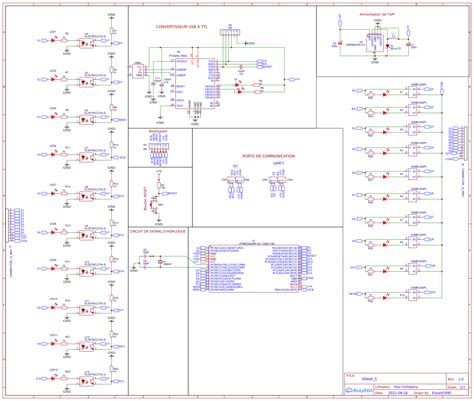
Best Practices and Tips
When working with the PCB API, consider the following best practices and tips to ensure a smooth and efficient workflow:
-
Familiarize yourself with the API documentation: Take the time to read and understand the API documentation thoroughly. It will provide valuable information on available functions, parameters, and usage examples.
-
Start with simple designs: If you’re new to the PCB API, start with simple PCB designs to familiarize yourself with the workflow and API functions. Gradually increase the complexity as you become more comfortable.
-
Use version control: Implement version control for your PCB designs and API scripts. This allows you to track changes, collaborate with others, and revert to previous versions if needed.
-
Modularize your code: Break down your PCB API scripts into modular and reusable functions. This promotes code readability, maintainability, and reusability.
-
Validate and test your designs: Always run design rule checks and perform thorough testing on your PCB designs before proceeding to manufacturing. The PCB API provides functions for DRC, but it’s important to validate the results and address any violations.
-
Collaborate and seek community support: Engage with the PCB design community, participate in forums, and seek guidance from experienced designers and developers. The PCB design community is a valuable resource for knowledge sharing, troubleshooting, and learning best practices.
Frequently Asked Questions (FAQ)
-
Q: What programming languages can I use with the PCB API?
A: The PCB API is typically accessible through programming languages such as Python, C++, and JavaScript. However, the specific language support may vary depending on the PCB design tool or API provider. -
Q: Can I use the PCB API with my existing PCB design tool?
A: Many popular PCB design tools provide API support or scripting capabilities. Check the documentation or contact the vendor of your specific PCB design tool to determine compatibility with the PCB API. -
Q: Is the PCB API suitable for beginners?
A: While the PCB API offers powerful capabilities, it does require some programming knowledge and familiarity with PCB design concepts. If you’re new to PCB design or programming, it’s recommended to start with simpler tools and gradually work your way up to using the PCB API. -
Q: Can I use the PCB API for commercial projects?
A: Yes, the PCB API can be used for both personal and commercial projects. However, make sure to review the licensing terms and conditions of the specific API provider to ensure compliance with their usage policies. -
Q: How can I get support or troubleshoot issues with the PCB API?
A: Most PCB API providers offer documentation, forums, and support channels to assist users. Consult the documentation or reach out to the API provider’s support team for assistance with specific issues or questions.
Conclusion
The PCB API is a powerful tool that empowers developers and designers to automate and streamline the PCB design process. By leveraging the capabilities of the PCB API, you can create, modify, and manage PCB designs programmatically, as well as integrate PCB design functionality into your own applications.
Throughout this article, we explored the key features and capabilities of the PCB API, including design creation and management, component libraries, placement and routing, design rule checking, and manufacturing output generation. We also discussed how you can integrate the PCB API into your existing workflow or build custom PCB design applications.
By following best practices, starting with simple designs, and seeking community support, you can effectively harness the power of the PCB API to enhance your PCB design process and create innovative solutions.
Remember, the PCB API is a versatile tool that opens up a wide range of possibilities in the world of PCB design. Whether you’re automating repetitive tasks, building custom applications, or pushing the boundaries of PCB design, the PCB API provides the foundation for turning your ideas into reality.
So go ahead, explore the PCB API, experiment with its capabilities, and unlock the full potential of programmatic PCB design. Happy designing!
No responses yet