Introduction to the TM1637 LED Display Module
The TM1637 is a popular 4-digit 7-segment LED display module commonly used in Arduino, Raspberry Pi, and other microcontroller projects. It provides an easy way to display numbers, characters, and simple animations. In this comprehensive tutorial, we will cover everything you need to know to get started with the TM1637 module, including its features, pinout, wiring, programming, and practical examples.
Key Features of the TM1637
- 4-digit 7-segment LED display
- I2C communication interface
- Adjustable display brightness
- Colon separator between digits
- Low power consumption
- Easy to use with Arduino and other microcontrollers
TM1637 Pinout and Wiring
The TM1637 module has four pins that need to be connected to your microcontroller:
Pin | Description |
---|---|
VCC | Power supply (3.3V to 5V) |
GND | Ground |
DIO | Data input/output |
CLK | Clock signal |
To wire the TM1637 to an Arduino, follow these steps:
- Connect VCC to the 5V pin on the Arduino.
- Connect GND to any GND pin on the Arduino.
- Connect DIO to any digital pin (e.g., pin 2).
- Connect CLK to any digital pin (e.g., pin 3).
Here’s a diagram illustrating the wiring:
TM1637 Arduino
VCC ----> 5V
GND ----> GND
DIO ----> Digital Pin (e.g., 2)
CLK ----> Digital Pin (e.g., 3)
Programming the TM1637 with Arduino
To program the TM1637 module with Arduino, you’ll need to install the TM1637 library. You can do this through the Arduino IDE’s Library Manager or by manually downloading the library from GitHub.
Installing the TM1637 Library
- Open the Arduino IDE.
- Go to Sketch > Include Library > Manage Libraries.
- Search for “TM1637” in the search bar.
- Find the “TM1637” library by Avishay Orpaz and click “Install”.
Once the library is installed, you can include it in your sketches using the following line at the top of your code:
#include <TM1637Display.h>
Basic Usage Example
Here’s a simple example that demonstrates how to display numbers on the TM1637:
#include <TM1637Display.h>
#define CLK 3
#define DIO 2
TM1637Display display(CLK, DIO);
void setup() {
display.setBrightness(0x0f);
}
void loop() {
int value = 1234;
display.showNumberDec(value);
delay(1000);
}
In this example:
1. We define the CLK and DIO pins connected to the TM1637.
2. We create a TM1637Display object with the specified pins.
3. In the setup()
function, we set the display brightness to maximum (0x0f).
4. In the loop()
function, we display the number 1234 using the showNumberDec()
function and delay for 1 second.
Displaying Characters and Animations
The TM1637 library provides functions to display individual segments, characters, and even create simple animations. Here are a few examples:
// Display individual segments
uint8_t segments[] = {0b00111111, 0b00000110, 0b01011011, 0b01001111};
display.setSegments(segments);
// Display a character
display.showNumberDecEx(0, 0b01100011, true);
// Create a simple animation
for (int i = 0; i < 4; i++) {
display.showNumberDecEx(0, 0, (0x80 >> i), true);
delay(250);
}
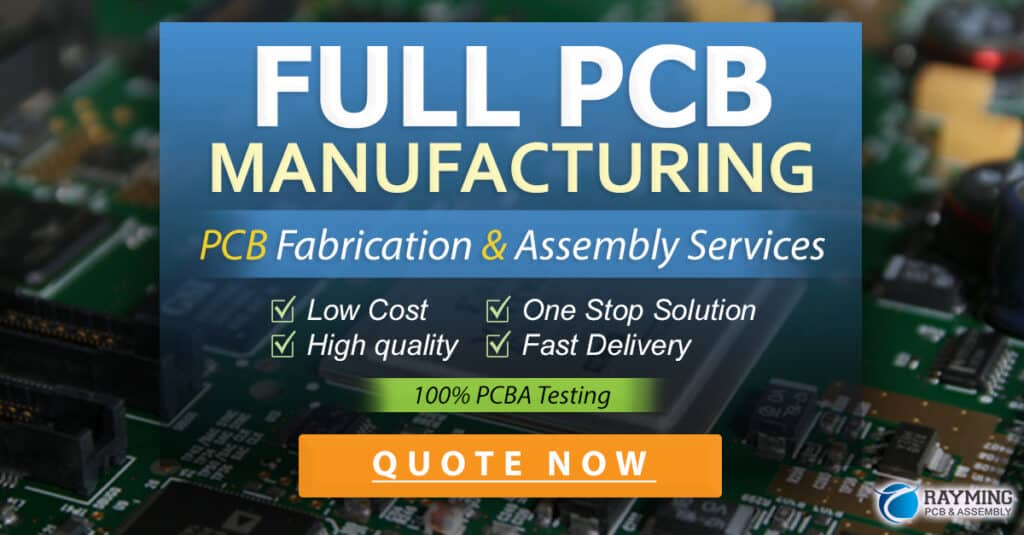
Advanced TM1637 Usage
Adjusting Display Brightness
You can adjust the display brightness using the setBrightness()
function. The brightness level ranges from 0 (lowest) to 7 (highest). For example:
display.setBrightness(3); // Set brightness to level 3
Controlling the Colon Separator
The TM1637 has a colon separator between the second and third digits. You can control its state using the showNumberDecEx()
function’s third argument. For example:
display.showNumberDecEx(1234, 0, true); // Display 12:34
display.showNumberDecEx(1234, 0, false); // Display 1234
Creating Custom Characters
You can create custom characters by defining the segment patterns using a byte value. Each bit in the byte represents a segment of the 7-Segment Display. Here’s an example:
// Create a custom character
uint8_t smile[] = {
SEG_A | SEG_B | SEG_C | SEG_D | SEG_E | SEG_F, // Smiley face
SEG_A | SEG_B | SEG_C | SEG_D | SEG_E | SEG_F, // Smiley face
SEG_A | SEG_B | SEG_C | SEG_D | SEG_E | SEG_F, // Smiley face
SEG_A | SEG_B | SEG_C | SEG_D | SEG_E | SEG_F // Smiley face
};
display.setSegments(smile);
Practical TM1637 Project Ideas
Now that you know how to use the TM1637 module, here are some practical project ideas to inspire you:
- Digital Clock: Use the TM1637 to display the current time, controlled by a real-time clock (RTC) module.
- Temperature Display: Connect a temperature sensor (e.g., DS18B20) to your microcontroller and display the temperature on the TM1637.
- Countdown Timer: Create a countdown timer that displays the remaining time on the TM1637.
- Score Board: Use the TM1637 to display scores for games or sports.
- Morse Code Translator: Display Morse code messages on the TM1637 based on user input.
Frequently Asked Questions (FAQ)
1. Can I connect multiple TM1637 modules to the same microcontroller?
Yes, you can connect multiple TM1637 modules to the same microcontroller by using different CLK and DIO pins for each module. Make sure to create separate TM1637Display objects for each module in your code.
2. How can I display floating-point numbers on the TM1637?
The TM1637 library does not have built-in support for displaying floating-point numbers. However, you can convert the floating-point number to a string and then display it using the showString()
function.
3. Can I control the TM1637 module with a Raspberry Pi?
Yes, you can control the TM1637 module with a Raspberry Pi using the Raspberry Pi’s GPIO pins. You’ll need to install the appropriate libraries (e.g., WiringPi) and adapt the code examples to work with the Raspberry Pi’s programming language (e.g., Python).
4. What should I do if the TM1637 module is not displaying anything?
If the TM1637 module is not displaying anything, check the following:
– Make sure the module is properly wired to your microcontroller.
– Verify that the correct pins are defined in your code.
– Ensure that the TM1637 library is properly installed and included in your sketch.
– Double-check the power supply and ground connections.
5. Can I change the I2C address of the TM1637 module?
No, the TM1637 module does not have a configurable I2C address. The communication is done through a custom protocol using the DIO and CLK pins, not the standard I2C protocol with addressable devices.
Conclusion
The TM1637 LED display module is a versatile and easy-to-use component for adding visual output to your Arduino, Raspberry Pi, and other microcontroller projects. With its 4-digit 7-segment display, adjustable brightness, and colon separator, you can create a wide range of applications, from simple number displays to more complex animations and custom characters.
By following this comprehensive tutorial, you should now have a solid understanding of how to wire, program, and use the TM1637 module in your projects. Don’t hesitate to experiment and explore the various functions and capabilities of the TM1637 library to create unique and exciting projects.
Happy coding and displaying with the TM1637!
No responses yet