What is Software Serial Arduino?
Software Serial Arduino is a library that allows you to create additional serial ports on your Arduino board using software instead of relying on the hardware serial ports. With SoftwareSerial, you can communicate with multiple devices simultaneously, even if your Arduino board has limited hardware serial ports.
The SoftwareSerial library emulates the functionality of a hardware serial port by using two digital pins of your choice. One pin acts as the transmitter (TX), and the other acts as the receiver (RX). By using software to control these pins, you can send and receive data just like you would with a hardware serial port.
Why Use Software Serial Arduino?
There are several reasons why you might want to use Software Serial Arduino in your projects:
-
Multiple Devices: If you need to communicate with multiple devices simultaneously, such as GPS modules, Bluetooth modules, or sensors, you may run out of hardware serial ports on your Arduino board. SoftwareSerial allows you to create additional serial ports to accommodate all your devices.
-
Pin Flexibility: With SoftwareSerial, you have the flexibility to choose any two digital pins on your Arduino board as the TX and RX pins. This gives you more control over the pin allocation in your project.
-
Baud Rate Flexibility: SoftwareSerial supports a wide range of baud rates, from 300 baud to 115200 baud. This allows you to communicate with devices that require different baud rates.
-
Debugging: SoftwareSerial can be a useful tool for debugging purposes. You can use it to send debug messages or data to your computer or another device without interfering with the hardware serial port.
How to Use Software Serial Arduino
Using Software Serial Arduino is relatively straightforward. Here’s a step-by-step guide to get you started:
- Include the SoftwareSerial Library: In your Arduino sketch, include the SoftwareSerial library at the top of your code:
cpp
#include <SoftwareSerial.h>
- Create a SoftwareSerial Object: Create an instance of the SoftwareSerial class by specifying the RX and TX pins you want to use:
cpp
SoftwareSerial mySerial(2, 3); // RX, TX
In this example, we’re using digital pins 2 and 3 for RX and TX, respectively.
- Initialize the SoftwareSerial Port: In the
setup()
function of your Arduino sketch, initialize the SoftwareSerial port with the desired baud rate:
cpp
void setup() {
mySerial.begin(9600);
}
Replace 9600
with the baud rate required by your connected device.
- Send and Receive Data: You can now use the
mySerial
object to send and receive data. To send data, use theprint()
orprintln()
functions:
cpp
mySerial.print("Hello, world!");
To receive data, use the available()
and read()
functions:
cpp
if (mySerial.available()) {
char data = mySerial.read();
// Process the received data
}
The available()
function checks if there is any data available to read, and the read()
function reads a single byte of data from the SoftwareSerial buffer.
Here’s a complete example that demonstrates sending and receiving data using SoftwareSerial:
#include <SoftwareSerial.h>
SoftwareSerial mySerial(2, 3); // RX, TX
void setup() {
mySerial.begin(9600);
mySerial.println("Hello, world!");
}
void loop() {
if (mySerial.available()) {
char data = mySerial.read();
mySerial.print("Received: ");
mySerial.println(data);
}
}
In this example, the Arduino board sends “Hello, world!” to the connected device and then listens for any incoming data. When data is received, it prints “Received: ” followed by the received character.
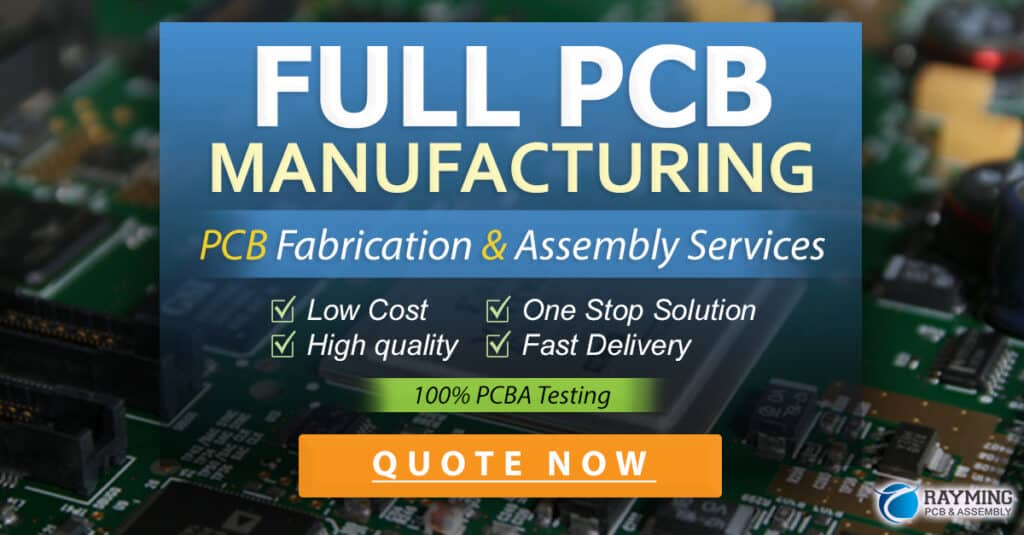
Limitations of Software Serial Arduino
While SoftwareSerial is a powerful tool, it does have some limitations compared to hardware serial communication:
-
Speed: SoftwareSerial is slower than hardware serial communication. It relies on software timing, which can be affected by other processes running on the Arduino board. As a result, the maximum reliable baud rate for SoftwareSerial is typically lower than that of hardware serial.
-
Interrupt Handling: SoftwareSerial relies on interrupts to handle the timing of the serial communication. If your Arduino sketch performs tasks that disable interrupts for a significant amount of time, it can interfere with the SoftwareSerial communication and cause data loss or corruption.
-
Pin Usage: Using SoftwareSerial occupies two digital pins on your Arduino board. These pins cannot be used for other purposes while SoftwareSerial is active.
Despite these limitations, SoftwareSerial remains a valuable tool for many Arduino projects that require multiple serial communication channels.
Advanced Topics
Multiple SoftwareSerial Instances
You can create multiple instances of the SoftwareSerial class to communicate with multiple devices simultaneously. Each instance requires its own set of RX and TX pins. Here’s an example:
#include <SoftwareSerial.h>
SoftwareSerial gpsSerial(2, 3); // RX, TX for GPS module
SoftwareSerial btSerial(4, 5); // RX, TX for Bluetooth module
void setup() {
gpsSerial.begin(9600);
btSerial.begin(38400);
}
void loop() {
// Communicate with GPS module
if (gpsSerial.available()) {
// Process GPS data
}
// Communicate with Bluetooth module
if (btSerial.available()) {
// Process Bluetooth data
}
}
In this example, we create two instances of SoftwareSerial: gpsSerial
for communicating with a GPS module and btSerial
for communicating with a Bluetooth module. Each instance uses its own set of RX and TX pins and can operate at different baud rates.
Combining SoftwareSerial with Hardware Serial
You can use SoftwareSerial in combination with the hardware serial port of your Arduino board. This allows you to communicate with multiple devices while still using the hardware serial port for other purposes, such as debugging or communication with the computer. Here’s an example:
#include <SoftwareSerial.h>
SoftwareSerial mySerial(2, 3); // RX, TX
void setup() {
Serial.begin(9600); // Hardware serial for debugging
mySerial.begin(9600); // SoftwareSerial for communication with a device
}
void loop() {
// Communicate with the device using SoftwareSerial
if (mySerial.available()) {
char data = mySerial.read();
// Process the received data
}
// Debug messages using hardware serial
Serial.println("Debug message");
}
In this example, we use the hardware serial port (Serial
) for sending debug messages to the computer, while using SoftwareSerial (mySerial
) for communication with a connected device.
SoftwareSerial and Interrupts
As mentioned earlier, SoftwareSerial relies on interrupts for accurate timing. If your Arduino sketch performs tasks that disable interrupts for a long period, it can interfere with the SoftwareSerial communication. To mitigate this issue, you can use the SoftwareSerial::listen()
function to explicitly tell SoftwareSerial to start listening for incoming data. Here’s an example:
#include <SoftwareSerial.h>
SoftwareSerial mySerial(2, 3); // RX, TX
void setup() {
mySerial.begin(9600);
}
void loop() {
// Perform tasks that may disable interrupts
// ...
// Start listening for incoming data
mySerial.listen();
// Communicate with the device using SoftwareSerial
if (mySerial.available()) {
char data = mySerial.read();
// Process the received data
}
}
In this example, we explicitly call mySerial.listen()
before checking for available data. This ensures that SoftwareSerial starts listening for incoming data even if interrupts were disabled earlier.
Troubleshooting
If you encounter issues while using SoftwareSerial, here are a few troubleshooting tips:
-
Check Wiring: Ensure that the RX and TX pins of your Arduino board are correctly connected to the TX and RX pins of the device you’re communicating with, respectively. Make sure the ground (GND) pins of both devices are connected.
-
Verify Baud Rate: Double-check that the baud rate specified in your Arduino sketch matches the baud rate of the connected device. Mismatched baud rates can result in garbled or missing data.
-
Reduce Baud Rate: If you’re experiencing data loss or corruption, try reducing the baud rate. SoftwareSerial may have limitations at higher baud rates, especially if your Arduino board is running at a lower clock speed.
-
Minimize Interrupt Blocking: If you have code that disables interrupts for an extended period, it can interfere with SoftwareSerial communication. Try to minimize the duration of interrupt blocking or use the
SoftwareSerial::listen()
function to explicitly start listening for incoming data. -
Check Power Supply: Ensure that your Arduino board and the connected devices have a stable and sufficient power supply. Inconsistent or inadequate power can cause communication issues.
Frequently Asked Questions (FAQ)
-
Can I use SoftwareSerial with any Arduino board?
Yes, SoftwareSerial is compatible with most Arduino boards, including Arduino Uno, Arduino Mega, Arduino Nano, and more. -
How many SoftwareSerial instances can I create?
The number of SoftwareSerial instances you can create depends on the available memory and digital pins on your Arduino board. Typically, you can create multiple instances as long as you have enough free digital pins and sufficient memory. -
Can I use SoftwareSerial for high-speed communication?
SoftwareSerial is limited in terms of speed compared to hardware serial communication. The maximum reliable baud rate for SoftwareSerial is typically lower than that of hardware serial. For high-speed communication, it’s recommended to use hardware serial if available. -
What should I do if I’m experiencing data loss or corruption with SoftwareSerial?
If you’re experiencing data loss or corruption, try reducing the baud rate, minimizing interrupt blocking, and ensuring a stable power supply. You can also use theSoftwareSerial::listen()
function to explicitly start listening for incoming data. -
Can I use SoftwareSerial and hardware serial simultaneously?
Yes, you can use SoftwareSerial in combination with the hardware serial port of your Arduino board. This allows you to communicate with multiple devices while still using the hardware serial port for other purposes, such as debugging or communication with the computer.
Conclusion
SoftwareSerial is a powerful library that extends the serial communication capabilities of Arduino boards. It allows you to create additional serial ports using software, enabling communication with multiple devices simultaneously. By understanding how to use SoftwareSerial effectively, you can expand the possibilities of your Arduino projects and create more complex and interactive systems.
In this article, we covered the fundamentals of Software Serial Arduino, including what it is, why you might want to use it, and how to implement it in your Arduino sketches. We also explored advanced topics such as using multiple SoftwareSerial instances, combining SoftwareSerial with hardware serial, and handling interrupts.
Remember to consider the limitations of SoftwareSerial, such as slower speed compared to hardware serial and the potential for interference from interrupt-blocking code. By keeping these limitations in mind and following best practices, you can successfully integrate SoftwareSerial into your Arduino projects.
As you continue your Arduino journey, don’t hesitate to experiment with SoftwareSerial and explore its possibilities. With creativity and persistence, you can create remarkable projects that push the boundaries of what’s possible with Arduino.
Happy coding and happy tinkering with Software Serial Arduino!
No responses yet