Introduction to SG 90 Servos and Arduino Servo Control
Servo motors are a type of motor that allows for precise control of angular position, velocity, and acceleration. They are commonly used in robotics, automation, and various other applications requiring accurate positioning or speed control. Among the various types of servo motors available, the SG 90 servo is a popular choice due to its small size, affordability, and ease of use. When combined with an Arduino Microcontroller, controlling SG 90 servos becomes a straightforward task, enabling developers and hobbyists to create impressive projects with minimal effort.
In this article, we will explore the SG 90 servo motor in detail, discussing its specifications, working principle, and how to control it using an Arduino board. We will also provide step-by-step instructions for setting up and programming the servo, along with sample code and practical examples. By the end of this article, you will have a solid understanding of Arduino servo control and be able to incorporate SG 90 servos into your own projects with confidence.
What is an SG 90 Servo?
The SG 90 servo is a mini-sized servo motor that offers precise rotation control within a 180-degree range. It is a popular choice for small-scale robotics projects, such as robotic arms, pan-tilt mechanisms, and remote-controlled vehicles. The servo’s compact size (approximately 22.2 x 11.8 x 31 mm) and lightweight design (around 9 grams) make it suitable for applications where space and weight are critical factors.
SG 90 Servo Specifications
To better understand the capabilities of the SG 90 servo, let’s take a look at its key specifications:
Specification | Value |
---|---|
Operating Voltage | 4.8V – 6V |
Torque | 1.8 kg-cm (4.8V), 2.2 kg-cm (6V) |
Speed | 0.1 sec/60 degrees (4.8V), 0.08 sec/60 degrees (6V) |
Rotation Range | 180 degrees |
Gear Type | Plastic |
Dimensions | 22.2 x 11.8 x 31 mm |
Weight | 9 grams |
As evident from the specifications, the SG 90 servo offers a decent amount of torque for its size, allowing it to handle small to medium-sized loads. The servo’s speed and rotation range make it suitable for applications requiring quick and precise movements within a half-circle range.
How Does an SG 90 Servo Work?
An SG 90 servo consists of several key components that work together to enable precise rotation control:
- DC Motor: The servo contains a small DC motor that generates the necessary torque to rotate the output shaft.
- Gearbox: A set of gears is used to reduce the motor’s speed and increase its torque, allowing for more precise control.
- Potentiometer: A potentiometer is connected to the output shaft, providing feedback on the servo’s current angular position.
- Control Circuit: The servo has an integrated control circuit that receives input signals and drives the DC motor accordingly.
The control circuit of the SG 90 servo expects a specific type of input signal known as Pulse Width Modulation (PWM). PWM signals consist of a series of pulses, where the width of each pulse determines the desired angular position of the servo. Typically, a pulse width of 1000 μs corresponds to a 0-degree position, while a pulse width of 2000 μs corresponds to a 180-degree position. By varying the pulse width between these two extremes, you can achieve any angle within the servo’s rotation range.
Controlling SG 90 Servos with Arduino
An Arduino microcontroller provides an ideal platform for controlling SG 90 servos. With its simple programming language and wide range of libraries, Arduino makes it easy to generate the necessary PWM signals and communicate with the servo. In this section, we will explore how to connect an SG 90 servo to an Arduino board and write code to control its position.
Hardware Setup
To get started, you will need the following components:
- Arduino board (e.g., Arduino Uno)
- SG 90 servo motor
- Jumper wires
- Breadboard (optional)
Follow these steps to connect the SG 90 servo to your Arduino board:
- Connect the servo’s VCC (red wire) to the Arduino’s 5V pin.
- Connect the servo’s GND (brown or black wire) to one of the Arduino’s GND pins.
- Connect the servo’s signal (orange or yellow wire) to one of the Arduino’s PWM pins (e.g., pin 9).
If you’re using a breadboard, you can use jumper wires to create a more organized and stable connection between the servo and the Arduino.
Software Setup
To control the SG 90 servo using Arduino, you will need to use the built-in Servo library. This library provides an easy-to-use interface for generating PWM signals and controlling the servo’s position.
First, make sure you have the Arduino IDE installed on your computer. Open the IDE and create a new sketch. At the top of your sketch, include the Servo library and create a Servo object:
#include <Servo.h>
Servo myServo;
In the setup()
function, attach the servo object to the PWM pin you used in the hardware setup:
void setup() {
myServo.attach(9);
}
Now, you can use the write()
function to set the servo’s position in degrees. For example, to rotate the servo to a 90-degree position, you would use:
myServo.write(90);
Here’s a complete example sketch that demonstrates how to control the SG 90 servo using Arduino:
#include <Servo.h>
Servo myServo;
void setup() {
myServo.attach(9);
}
void loop() {
myServo.write(0); // Rotate to 0 degrees
delay(1000);
myServo.write(90); // Rotate to 90 degrees
delay(1000);
myServo.write(180); // Rotate to 180 degrees
delay(1000);
}
In this example, the servo will rotate to 0 degrees, wait for 1 second, then rotate to 90 degrees, wait for another second, and finally rotate to 180 degrees before starting the sequence again.
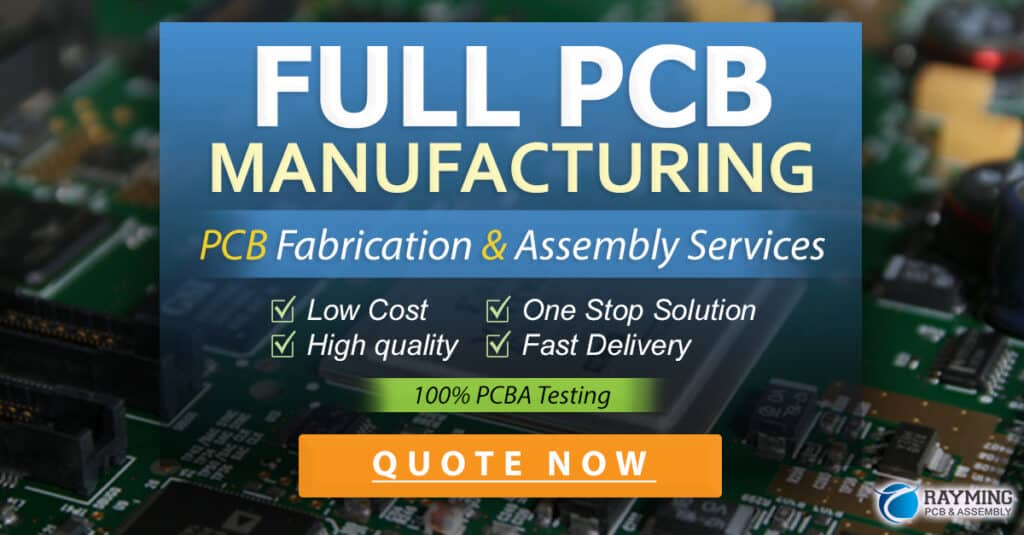
Advanced Servo Control Techniques
Now that you have a basic understanding of how to control an SG 90 servo using Arduino, let’s explore some advanced techniques that can help you create more sophisticated servo-based projects.
Smooth Servo Movement
Instead of abruptly moving the servo from one position to another, you can achieve smoother and more natural-looking motion by gradually changing the servo’s position over time. To do this, you can use a for loop to increment or decrement the servo’s position in small steps, with a short delay between each step.
Here’s an example sketch that demonstrates smooth servo movement:
#include <Servo.h>
Servo myServo;
void setup() {
myServo.attach(9);
}
void loop() {
for (int pos = 0; pos <= 180; pos += 1) {
myServo.write(pos);
delay(15);
}
for (int pos = 180; pos >= 0; pos -= 1) {
myServo.write(pos);
delay(15);
}
}
In this example, the servo will smoothly rotate from 0 to 180 degrees and then back to 0 degrees, with a small delay of 15 milliseconds between each step.
Controlling Multiple Servos
You can easily control multiple SG 90 servos using Arduino by creating additional Servo objects and attaching them to different PWM pins. This allows you to create more complex projects, such as robotic arms or multi-joint mechanisms.
Here’s an example sketch that demonstrates how to control two SG 90 servos simultaneously:
#include <Servo.h>
Servo myServo1;
Servo myServo2;
void setup() {
myServo1.attach(9);
myServo2.attach(10);
}
void loop() {
myServo1.write(0);
myServo2.write(180);
delay(1000);
myServo1.write(90);
myServo2.write(90);
delay(1000);
myServo1.write(180);
myServo2.write(0);
delay(1000);
}
In this example, the two servos will move in opposite directions, creating a mirrored motion effect.
Servo Calibration
Due to manufacturing variations, the actual range of motion for an SG 90 servo may not exactly match the specified 180-degree range. To ensure accurate positioning, you can calibrate your servo by determining its actual minimum and maximum pulse widths.
To calibrate your servo, follow these steps:
- Upload a sketch that slowly sweeps the servo from 0 to 180 degrees, similar to the smooth servo movement example above.
- Observe the servo’s movement and note the positions where it starts and stops rotating.
- Modify the minimum and maximum pulse widths in your sketch to match the observed values.
For example, if you find that your servo starts rotating at a pulse width of 500 μs and stops at 2500 μs, you can update your sketch to use these values:
myServo.writeMicroseconds(500); // Minimum pulse width
myServo.writeMicroseconds(2500); // Maximum pulse width
By calibrating your servo, you can ensure that your projects will have accurate and consistent servo positioning.
Practical Examples and Projects
Now that you have a solid understanding of how to control SG 90 servos using Arduino, let’s explore some practical examples and projects that showcase the capabilities of these mini-sized motors.
Pan-Tilt Mechanism
A pan-tilt mechanism is a common application for SG 90 servos, allowing you to control the horizontal (pan) and vertical (tilt) rotation of a camera, sensor, or other device.
To create a pan-tilt mechanism, you will need two SG 90 servos, a pan-tilt bracket, and your Arduino board. Follow these steps:
- Assemble the pan-tilt bracket according to its instructions, attaching one servo for the pan motion and another for the tilt motion.
- Connect the servos to your Arduino board as described in the hardware setup section above.
- Create a sketch that allows you to control the pan and tilt servos independently, using either predetermined positions or input from sensors or other sources.
Here’s an example sketch for a basic pan-tilt mechanism:
#include <Servo.h>
Servo panServo;
Servo tiltServo;
void setup() {
panServo.attach(9);
tiltServo.attach(10);
}
void loop() {
// Pan left to right
for (int pos = 0; pos <= 180; pos += 1) {
panServo.write(pos);
delay(15);
}
for (int pos = 180; pos >= 0; pos -= 1) {
panServo.write(pos);
delay(15);
}
// Tilt up and down
for (int pos = 0; pos <= 90; pos += 1) {
tiltServo.write(pos);
delay(15);
}
for (int pos = 90; pos >= 0; pos -= 1) {
tiltServo.write(pos);
delay(15);
}
}
In this example, the pan servo will rotate from left to right and back, while the tilt servo will tilt up and down, creating a basic scanning motion.
Robotic Arm
SG 90 servos can also be used to create small robotic arms capable of picking up and manipulating lightweight objects. A simple robotic arm can be constructed using three or more SG 90 servos, a set of linkages, and a gripper or end effector.
To build a robotic arm, follow these steps:
- Design and construct the linkages and gripper for your robotic arm, ensuring that the SG 90 servos can provide sufficient torque to move the arm and manipulate objects.
- Connect the servos to your Arduino board as described in the hardware setup section above.
- Create a sketch that allows you to control the individual servos, either through predetermined positions or by using input from sensors, joysticks, or other control mechanisms.
Here’s an example sketch for a basic three-servo robotic arm:
#include <Servo.h>
Servo baseServo;
Servo shoulderServo;
Servo elbowServo;
void setup() {
baseServo.attach(9);
shoulderServo.attach(10);
elbowServo.attach(11);
}
void loop() {
// Move the base servo
baseServo.write(0);
delay(1000);
baseServo.write(90);
delay(1000);
baseServo.write(180);
delay(1000);
// Move the shoulder servo
shoulderServo.write(0);
delay(1000);
shoulderServo.write(90);
delay(1000);
// Move the elbow servo
elbowServo.write(0);
delay(1000);
elbowServo.write(90);
delay(1000);
}
In this example, the base servo will rotate to three different positions, while the shoulder and elbow servos will move between two positions each, demonstrating the basic motion of a robotic arm.
Troubleshooting and FAQs
Why isn’t my SG 90 servo responding to Arduino commands?
If your SG 90 servo isn’t responding to Arduino commands, there could be several reasons:
- Incorrect wiring: Double-check your connections to ensure that the servo is properly connected to the Arduino board, with the signal wire connected to a PWM pin and the power and ground wires connected to the appropriate pins.
- Insufficient power supply: Make sure your Arduino board can provide enough power to the servo. If the servo is drawing too much current, it may cause the Arduino to reset or behave erratically.
- Incorrect library or sketch: Verify that you have included the Servo library in your sketch and that you are using the correct functions to control the servo, such as
attach()
andwrite()
.
Can I control an SG 90 servo without using the Arduino Servo library?
Yes, it is possible to control an SG 90 servo without using the Arduino Servo library. You can generate the necessary PWM signals using the digitalWrite()
and delayMicroseconds()
functions. However, using the Servo library is generally recommended, as it simplifies the process and handles the timing and pulse width calculations for you.
How much torque can an SG 90 servo provide?
The SG 90 servo can provide a torque of 1.8 kg-cm at 4.8V and 2.2 kg-cm at 6V. This means that the servo can apply a force of 1.8 kg or 2.2 kg, respectively, at a distance of 1 cm from the shaft. Keep in mind that the actual torque may vary slightly due to manufacturing variations and the specific load conditions.
Can I use an external power source for my SG 90 servo?
Yes, you can use an external power source for your SG 90 servo if the Arduino board cannot provide sufficient power. To do this, connect the servo’s VCC wire to the positive terminal of your external power source (4.8V to 6V) and the GND wire to the negative terminal. Make sure to connect the external power source’s ground to the Arduino’s ground pin to ensure a common ground reference.
How can I control the speed of my SG 90 servo?
To control the speed of your SG 90 servo, you can adjust the delay between each step when moving the servo between positions. A shorter delay will result in faster servo movement, while a longer delay will slow down the servo. You can also use the writeMicroseconds()
function to directly control the pulse width, which in turn affects the servo’s speed. However, keep in mind that the SG 90 servo has physical limitations on its maximum speed, and attempting to move it faster than its specifications may result in poor performance or damage to the servo.
Conclusion
In this article, we have explored the SG 90 servo motor and how to control it using an Arduino microcontroller. We discussed the servo’s specifications, working principle, and the steps required to set up and program the servo using Arduino. We also covered advanced servo control techniques, such as smooth servo movement and controlling multiple servos simultaneously.
Additionally, we provided practical examples and projects, including a
No responses yet