What is a Remote Control Tester?
A remote control tester is a device that helps you diagnose problems with your remote control. It can detect issues such as weak batteries, faulty buttons, or malfunctioning infrared (IR) sensors. By using a remote control tester, you can quickly identify the problem and take appropriate action to fix it.
Why Should You Use a Remote Control Tester?
There are several reasons why you should use a remote control tester:
-
Save Money: Instead of buying a new remote control every time your old one malfunctions, you can use a remote control tester to diagnose and fix the problem. This can save you a lot of money in the long run.
-
Convenience: With a remote control tester, you can quickly diagnose the problem and take appropriate action. This can save you time and effort, especially if you have multiple remote controls in your home.
-
Learn New Skills: Building a remote control tester is a great way to learn new skills such as soldering, programming, and electronics. These skills can be useful in other DIY Projects as well.
DIY Project 1: Building a Simple Remote Control Tester
In this project, we will build a simple remote control tester using an Arduino board and an IR receiver. This tester will allow you to detect the IR signals from your remote control and display them on a serial monitor.
Materials Required
Component | Quantity |
---|---|
Arduino Uno Board | 1 |
IR Receiver | 1 |
Jumper Wires | 3 |
Breadboard | 1 |
Step-by-Step Instructions
-
Connect the IR receiver to the Arduino board using jumper wires. Connect the VCC pin of the IR receiver to the 5V pin of the Arduino, the GND pin to the GND pin of the Arduino, and the OUT pin to digital pin 11 of the Arduino.
-
Open the Arduino IDE and create a new sketch.
-
Copy and paste the following code into the sketch:
#include <IRremote.h>
int RECV_PIN = 11;
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup() {
Serial.begin(9600);
irrecv.enableIRIn();
}
void loop() {
if (irrecv.decode(&results)) {
Serial.println(results.value, HEX);
irrecv.resume();
}
}
-
Upload the sketch to the Arduino board.
-
Open the serial monitor and press a button on your remote control. You should see the IR code of the button displayed on the serial monitor.
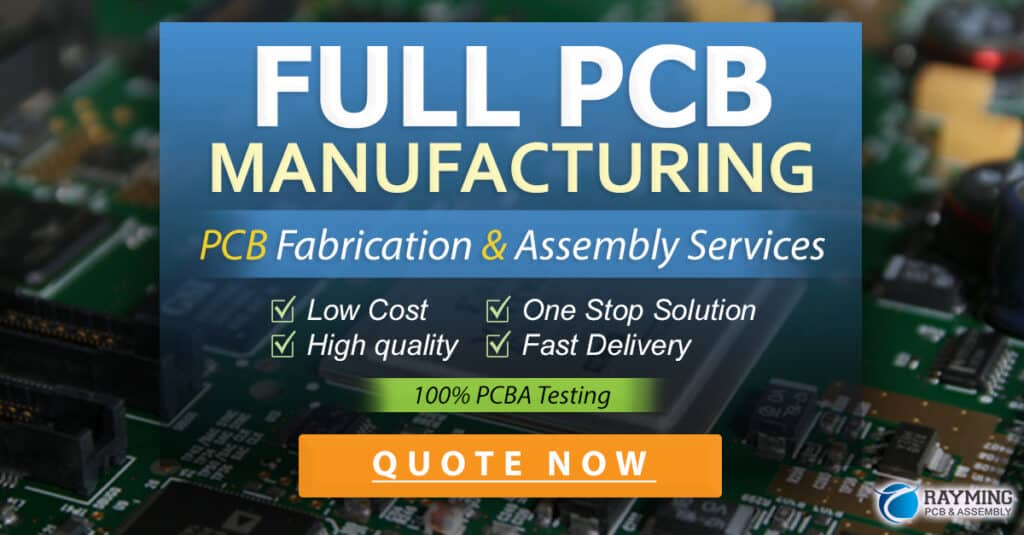
DIY Project 2: Building an Advanced Remote Control Tester
In this project, we will build an advanced remote control tester that can not only detect IR signals but also transmit them. This tester will allow you to test the functionality of your remote control buttons and even clone your remote control.
Materials Required
Component | Quantity |
---|---|
Arduino Uno Board | 1 |
IR Receiver | 1 |
IR Transmitter | 1 |
Jumper Wires | 5 |
Breadboard | 1 |
Pushbutton | 1 |
10K Ohm Resistor | 1 |
Step-by-Step Instructions
-
Connect the IR receiver and transmitter to the Arduino board using jumper wires. Connect the VCC pin of the IR receiver and transmitter to the 5V pin of the Arduino, the GND pin to the GND pin of the Arduino, and the OUT pin of the receiver to digital pin 11 of the Arduino. Connect the IN pin of the transmitter to digital pin 3 of the Arduino.
-
Connect the pushbutton to the Arduino board. Connect one leg of the pushbutton to digital pin 2 of the Arduino and the other leg to the GND pin of the Arduino. Connect the 10K ohm resistor between digital pin 2 and the 5V pin of the Arduino.
-
Open the Arduino IDE and create a new sketch.
-
Copy and paste the following code into the sketch:
#include <IRremote.h>
int RECV_PIN = 11;
int SEND_PIN = 3;
int BUTTON_PIN = 2;
IRrecv irrecv(RECV_PIN);
IRsend irsend;
decode_results results;
void setup() {
Serial.begin(9600);
irrecv.enableIRIn();
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
if (irrecv.decode(&results)) {
Serial.println(results.value, HEX);
if (digitalRead(BUTTON_PIN) == LOW) {
irsend.sendNEC(results.value, 32);
Serial.println("IR code sent!");
}
irrecv.resume();
}
}
-
Upload the sketch to the Arduino board.
-
Press a button on your remote control. The IR code of the button will be displayed on the serial monitor.
-
Press the pushbutton on the breadboard. The IR code displayed on the serial monitor will be transmitted by the IR transmitter.
DIY Project 3: Building a Universal Remote Control Tester
In this project, we will build a universal remote control tester that can learn and transmit IR codes from any remote control. This tester will allow you to control multiple devices with a single remote control.
Materials Required
Component | Quantity |
---|---|
Arduino Uno Board | 1 |
IR Receiver | 1 |
IR Transmitter | 1 |
Jumper Wires | 5 |
Breadboard | 1 |
Pushbutton | 2 |
10K Ohm Resistor | 2 |
OLED Display | 1 |
Step-by-Step Instructions
-
Connect the IR receiver and transmitter to the Arduino board using jumper wires. Connect the VCC pin of the IR receiver and transmitter to the 5V pin of the Arduino, the GND pin to the GND pin of the Arduino, and the OUT pin of the receiver to digital pin 11 of the Arduino. Connect the IN pin of the transmitter to digital pin 3 of the Arduino.
-
Connect the pushbuttons to the Arduino board. Connect one leg of each pushbutton to digital pins 2 and 4 of the Arduino and the other leg to the GND pin of the Arduino. Connect a 10K ohm resistor between each pushbutton and the 5V pin of the Arduino.
-
Connect the OLED display to the Arduino board using jumper wires. Connect the VCC pin of the display to the 5V pin of the Arduino, the GND pin to the GND pin of the Arduino, the SCL pin to analog pin 5 of the Arduino, and the SDA pin to analog pin 4 of the Arduino.
-
Open the Arduino IDE and create a new sketch.
-
Copy and paste the following code into the sketch:
#include <IRremote.h>
#include <SPI.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
#define OLED_RESET -1
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
int RECV_PIN = 11;
int SEND_PIN = 3;
int LEARN_BUTTON_PIN = 2;
int SEND_BUTTON_PIN = 4;
IRrecv irrecv(RECV_PIN);
IRsend irsend;
decode_results results;
unsigned long irCode = 0;
void setup() {
Serial.begin(9600);
irrecv.enableIRIn();
pinMode(LEARN_BUTTON_PIN, INPUT_PULLUP);
pinMode(SEND_BUTTON_PIN, INPUT_PULLUP);
if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;);
}
display.clearDisplay();
display.setTextSize(1);
display.setTextColor(WHITE);
display.setCursor(0,0);
display.println("Universal Remote");
display.println("Control Tester");
display.display();
delay(2000);
}
void loop() {
if (digitalRead(LEARN_BUTTON_PIN) == LOW) {
display.clearDisplay();
display.setCursor(0,0);
display.println("Learning IR Code...");
display.display();
irrecv.resume();
while (!irrecv.decode(&results)) {}
irCode = results.value;
display.clearDisplay();
display.setCursor(0,0);
display.println("IR Code Learned:");
display.println(irCode, HEX);
display.display();
delay(2000);
}
if (digitalRead(SEND_BUTTON_PIN) == LOW) {
display.clearDisplay();
display.setCursor(0,0);
display.println("Sending IR Code...");
display.display();
irsend.sendNEC(irCode, 32);
display.clearDisplay();
display.setCursor(0,0);
display.println("IR Code Sent:");
display.println(irCode, HEX);
display.display();
delay(2000);
}
}
-
Upload the sketch to the Arduino board.
-
Press the “learn” pushbutton on the breadboard and aim a remote control at the IR receiver. The IR code of the button pressed will be displayed on the OLED display.
-
Press the “send” pushbutton on the breadboard. The IR code displayed on the OLED display will be transmitted by the IR transmitter.
Frequently Asked Questions (FAQ)
-
Can I use any Arduino board for these projects?
Yes, you can use any Arduino board that has enough digital and analog pins for the components used in each project. However, the code provided in this article is written for the Arduino Uno board. -
What is the range of the IR receiver and transmitter?
The range of the IR receiver and transmitter depends on several factors such as the quality of the components, the voltage supplied, and the environmental conditions. Generally, the range is around 10-20 feet. -
Can I use these testers with any remote control?
Yes, these testers can be used with any remote control that uses IR technology. However, some remote controls may use different IR protocols or frequencies that may not be compatible with the code provided in this article. -
What if the IR code is not displayed on the serial monitor or OLED display?
There could be several reasons for this such as a faulty IR receiver, incorrect wiring, or incompatible IR protocol. Double-check the wiring and make sure the IR receiver is working properly. You may also need to modify the code to support different IR protocols. -
Can I use these testers to control other devices besides remote controls?
Yes, you can use these testers to control any device that can be controlled by an IR remote control such as TVs, air conditioners, and sound systems. However, you will need to know the IR codes for the specific device you want to control.
Conclusion
In this article, we explored three key DIY projects that you can build to test and repair your remote controls. These projects range from a simple IR receiver to a universal remote control tester that can learn and transmit IR codes from any remote control. By building these projects, you can not only save money and time but also learn valuable skills in electronics and programming. So, grab your tools and start building your own remote control tester today!
No responses yet