What is a Raspberry Pi?
Before we dive into the voice recognition project, let’s take a moment to discuss what a Raspberry Pi is. A Raspberry Pi is a small, low-cost computer that was originally designed for educational purposes, but has since become popular among hobbyists and makers for a wide range of projects. The Raspberry Pi runs on a Linux-based operating system and has a variety of input/output options, including USB ports, HDMI, and GPIO pins for connecting sensors and other components.
Hardware Required for Raspberry Pi Voice Recognition
To create a Raspberry Pi voice recognition system, you’ll need the following hardware components:
Component | Description |
---|---|
Raspberry Pi board | The main computer that will run the voice recognition software. Any model of Raspberry Pi should work, but a Raspberry Pi 3 or 4 is recommended for best performance. |
Microphone | A USB microphone or a microphone connected to the Raspberry Pi’s audio input jack. |
Speakers (optional) | If you want your Raspberry Pi to provide audio output, you’ll need speakers or headphones. |
Power supply | A power supply for your Raspberry Pi, such as a micro USB power adapter. |
microSD card | A microSD card with at least 8GB of storage to hold the operating system and software. |
Software Required for Raspberry Pi Voice Recognition
In addition to the hardware, you’ll also need some software to make your Raspberry Pi voice recognition system work:
Software | Description |
---|---|
Raspberry Pi OS | The official operating system for the Raspberry Pi, which can be downloaded from the Raspberry Pi website. |
Python | The programming language used to write the voice recognition software. Python comes pre-installed on Raspberry Pi OS. |
PyAudio | A Python library for audio input and output. |
SpeechRecognition | A Python library for performing speech recognition with various engines and APIs, including Google Speech Recognition, Sphinx, and Wit.ai. |
PocketSphinx | An open-source speech recognition engine that can be used with the SpeechRecognition library for offline voice recognition. |
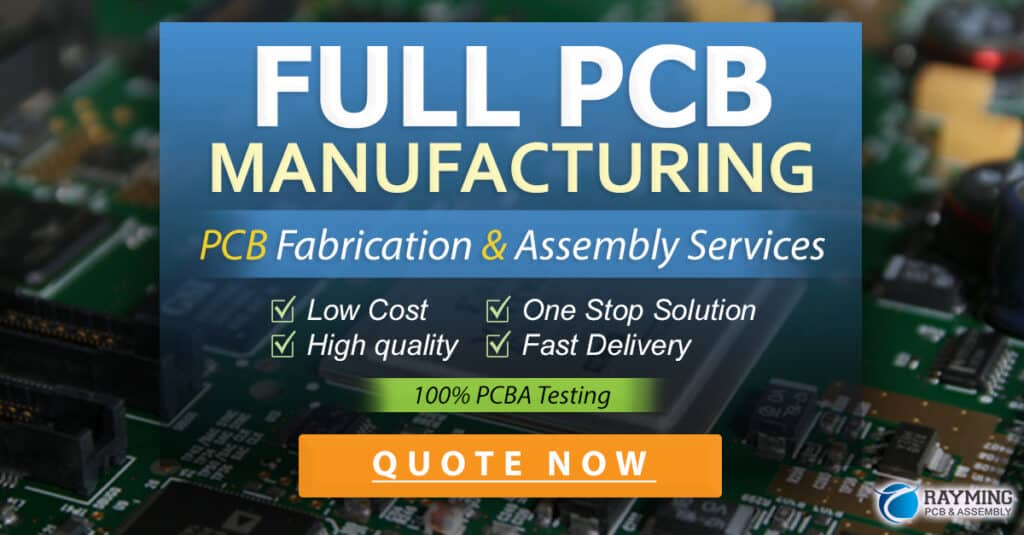
Setting Up Your Raspberry Pi for Voice Recognition
Before you can start using your Raspberry Pi for voice recognition, you’ll need to set it up with the necessary software. Here’s a step-by-step guide:
-
Install Raspberry Pi OS: Download the latest version of Raspberry Pi OS from the official website and install it on your microSD card using the Raspberry Pi Imager tool.
-
Boot up your Raspberry Pi: Insert the microSD card into your Raspberry Pi and connect the power supply to boot it up.
-
Connect to Wi-Fi: If your Raspberry Pi has built-in Wi-Fi, you can connect to your network using the desktop GUI or by editing the
wpa_supplicant.conf
file. If you’re using an older model without Wi-Fi, you’ll need to connect an Ethernet cable. -
Update the system: Open a terminal window and run the following commands to update your Raspberry Pi’s software:
sudo apt update
sudo apt upgrade
- Install PyAudio: To install PyAudio, run the following command in the terminal:
sudo apt-get install python3-pyaudio
- Install SpeechRecognition: To install the SpeechRecognition library, run the following command:
pip3 install SpeechRecognition
- Install PocketSphinx (optional): If you want to use the PocketSphinx engine for offline voice recognition, you’ll need to install it with the following commands:
sudo apt-get install libpulse-dev
pip3 install pocketsphinx
Writing the Voice Recognition Code
Now that your Raspberry Pi is set up with the necessary software, it’s time to write the code for your voice recognition system. Here’s a basic Python script that uses the SpeechRecognition library to listen for speech and print out the recognized text:
import speech_recognition as sr
# Create a recognizer object
r = sr.Recognizer()
# Use the default microphone as the audio source
with sr.Microphone() as source:
print("Speak now!")
# Adjust for ambient noise
r.adjust_for_ambient_noise(source)
# Listen for speech
audio = r.listen(source)
# Recognize speech using Google Speech Recognition
try:
text = r.recognize_google(audio)
print(f"You said: {text}")
except sr.UnknownValueError:
print("Google Speech Recognition could not understand audio")
except sr.RequestError as e:
print(f"Could not request results from Google Speech Recognition service; {e}")
This script does the following:
- Imports the SpeechRecognition library.
- Creates a recognizer object.
- Uses the default microphone as the audio source.
- Adjusts for ambient noise.
- Listens for speech and stores the audio data in the
audio
variable. - Attempts to recognize the speech using Google Speech Recognition and prints out the recognized text.
- Handles any errors that may occur during the recognition process.
You can save this script to a file with a .py
extension (e.g. voice_recognition.py
) and run it from the terminal with the command python3 voice_recognition.py
.
Expanding on the Basic Project
Once you have the basic voice recognition system up and running, there are many ways you can expand on the project to make it more useful or interesting. Here are a few ideas:
Add voice commands
Instead of just recognizing and printing out the speech, you can add voice commands that trigger specific actions. For example, you could create a voice-controlled home automation system that turns lights on and off or adjusts the thermostat based on voice commands.
Integrate with other APIs
The SpeechRecognition library supports several different speech recognition engines and APIs, including Google Speech Recognition, Sphinx, and Wit.ai. You can experiment with different engines to see which one works best for your needs, or even combine multiple engines for improved accuracy.
Build a voice-controlled robot
If you have a robotics kit or other hardware that can be controlled by a Raspberry Pi, you can use voice commands to control the robot’s movements or actions. For example, you could build a voice-controlled toy car or a robotic arm that responds to spoken instructions.
Create a voice-activated virtual assistant
With some additional programming, you can turn your Raspberry Pi voice recognition system into a virtual assistant that can answer questions, set reminders, play music, and more. You can use APIs like Google Calendar, Wikipedia, and Spotify to give your assistant a wide range of capabilities.
Frequently Asked Questions (FAQ)
1. Can I use a different microphone or audio input device?
Yes, you can use any microphone or audio input device that is compatible with your Raspberry Pi. Just make sure to select the correct device in your Python code.
2. Can I use offline voice recognition instead of Google Speech Recognition?
Yes, you can use the PocketSphinx engine for offline voice recognition. However, keep in mind that offline recognition may not be as accurate as cloud-based services like Google Speech Recognition.
3. How can I improve the accuracy of the voice recognition?
There are several things you can do to improve the accuracy of your voice recognition system:
- Use a high-quality microphone and ensure it is positioned close to the speaker.
- Adjust for ambient noise using the
adjust_for_ambient_noise()
function. - Speak clearly and at a consistent volume.
- Train the recognizer with your own voice using the
recognizer_instance.recognize_sphinx()
function. - Use multiple recognition engines and combine the results.
4. Can I use this project for commercial purposes?
The SpeechRecognition library and most of the other software used in this project are open-source and free to use for any purpose. However, if you plan to use a cloud-based recognition service like Google Speech Recognition, you may need to pay for API access depending on your usage volume.
5. What other programming languages can I use for voice recognition on the Raspberry Pi?
While Python is a popular choice for Raspberry Pi projects due to its ease of use and wide range of libraries, you can use other programming languages like C++, Java, or Node.js for voice recognition on the Raspberry Pi. There are libraries and APIs available for these languages that provide similar functionality to the SpeechRecognition library in Python.
Conclusion
Voice recognition technology is a fascinating and rapidly evolving field, and the Raspberry Pi provides an accessible and affordable platform for experimenting with this technology. By following the steps outlined in this article, you can create your own voice recognition system using a Raspberry Pi, a microphone, and some open-source software. Whether you’re a hobbyist, a student, or a professional developer, there are endless possibilities for what you can create with Raspberry Pi voice recognition. So grab your Raspberry Pi and start exploring the world of voice-controlled computing today!
No responses yet