Introduction to Raspberry Pi and Microcontrollers
The Raspberry Pi has revolutionized the world of single-board computers since its introduction in 2012. This credit card-sized computer has found its way into countless projects, from home automation systems to retro gaming consoles. However, the Raspberry Pi Foundation has now set its sights on a new market: microcontrollers.
What are Microcontrollers?
Microcontrollers are small, low-cost, and low-power computers that are designed to perform specific tasks. They are widely used in embedded systems, such as appliances, automobiles, and industrial control systems. Unlike the Raspberry Pi, which runs a full operating system and can perform a wide range of tasks, microcontrollers are typically programmed to perform a single function.
The Raspberry Pi Pico
In January 2021, the Raspberry Pi Foundation announced the release of the Raspberry Pi Pico, a microcontroller board based on the RP2040 chip. The RP2040 is a dual-core ARM Cortex-M0+ processor with 264KB of on-chip RAM and support for up to 16MB of off-chip Flash memory.
Specification | Raspberry Pi Pico |
---|---|
Processor | RP2040 (Dual-core Cortex-M0+) |
RAM | 264KB |
Flash Memory | 2MB (supports up to 16MB) |
GPIO Pins | 26 |
ADC Inputs | 3 |
USB | 1x USB 1.1 Host/Device |
Power | 1.8-5.5V |
The Raspberry Pi Pico is designed to be easy to use and accessible to a wide range of users, from beginners to experienced embedded systems developers. It features a USB connector for power and data, as well as 26 GPIO pins for connecting peripherals and sensors.
Programming the Raspberry Pi Pico
MicroPython
One of the key features of the Raspberry Pi Pico is its support for MicroPython, a lightweight implementation of the Python programming language designed for microcontrollers. MicroPython allows users to write high-level code that can be easily uploaded to the Pico via a simple USB connection.
Here’s a simple example of a MicroPython program that blinks an LED connected to GPIO pin 25:
from machine import Pin
from time import sleep
led = Pin(25, Pin.OUT)
while True:
led.on()
sleep(0.5)
led.off()
sleep(0.5)
C/C++
For more advanced users, the Raspberry Pi Pico also supports programming in C/C++ using the Pico SDK. The SDK provides a set of libraries and tools for developing bare-metal applications and interacting with the RP2040’s hardware features.
Here’s the same LED blinking example written in C:
#include "pico/stdlib.h"
int main() {
gpio_init(25);
gpio_set_dir(25, GPIO_OUT);
while (true) {
gpio_put(25, 1);
sleep_ms(500);
gpio_put(25, 0);
sleep_ms(500);
}
}
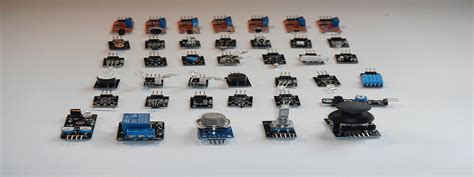
Interfacing with Sensors and Peripherals
One of the key advantages of microcontrollers like the Raspberry Pi Pico is their ability to interface with a wide range of sensors and peripherals. The Pico’s GPIO pins can be used to communicate with devices using protocols like I2C, SPI, and UART.
I2C
I2C (Inter-Integrated Circuit) is a widely used protocol for communicating with sensors and other devices. The Raspberry Pi Pico has two I2C controllers that can be used to communicate with up to 127 devices on a single bus.
Here’s an example of using MicroPython to read data from an MPU6050 accelerometer/gyroscope sensor via I2C:
from machine import Pin, I2C
from mpu6050 import MPU6050
i2c = I2C(0, scl=Pin(1), sda=Pin(0), freq=400000)
mpu = MPU6050(i2c)
while True:
print(mpu.accel.xyz, mpu.gyro.xyz)
SPI
SPI (Serial Peripheral Interface) is another common protocol for communicating with sensors and other devices. The Raspberry Pi Pico has two SPI controllers that can be used to communicate with devices at high speeds.
Here’s an example of using C to read data from an SPI-based ADC (Analog-to-Digital Converter):
#include "pico/stdlib.h"
#include "hardware/spi.h"
#define SPI_PORT spi0
#define SPI_MISO 4
#define SPI_CS 5
#define SPI_SCK 6
#define SPI_MOSI 7
uint16_t read_adc() {
uint8_t buf[2];
gpio_put(SPI_CS, 0);
spi_read_blocking(SPI_PORT, 0, buf, 2);
gpio_put(SPI_CS, 1);
return (buf[0] << 8) | buf[1];
}
int main() {
spi_init(SPI_PORT, 1000000);
gpio_set_function(SPI_MISO, GPIO_FUNC_SPI);
gpio_set_function(SPI_SCK, GPIO_FUNC_SPI);
gpio_set_function(SPI_MOSI, GPIO_FUNC_SPI);
gpio_init(SPI_CS);
gpio_set_dir(SPI_CS, GPIO_OUT);
gpio_put(SPI_CS, 1);
while (true) {
uint16_t value = read_adc();
// Do something with the value
}
}
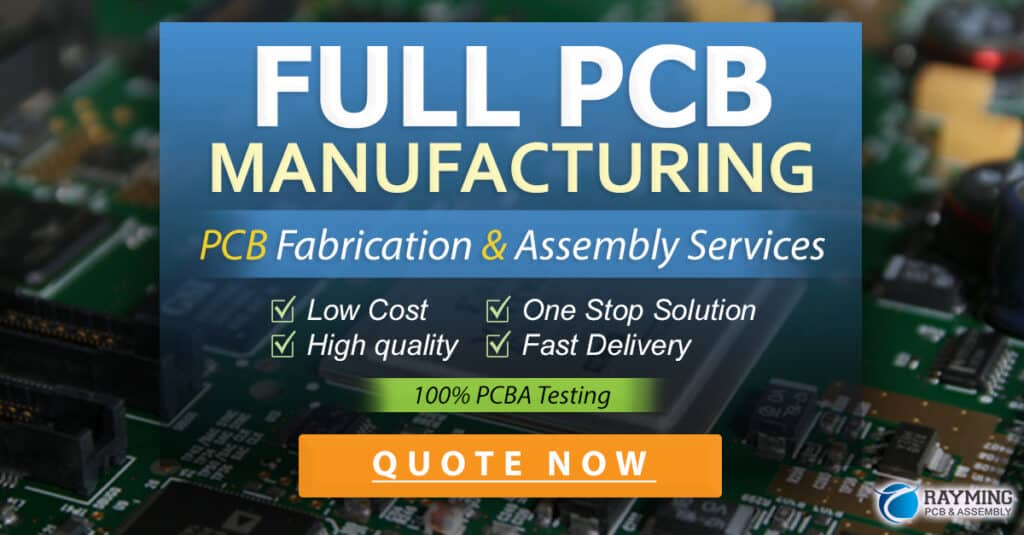
Example Projects
Temperature and Humidity Monitor
One simple project that demonstrates the capabilities of the Raspberry Pi Pico is a temperature and humidity monitor. This project uses a DHT22 sensor to measure the ambient temperature and humidity, and displays the readings on an OLED display.
Components:
– Raspberry Pi Pico
– DHT22 sensor
– 128×64 OLED display (I2C)
– Breadboard and jumper wires
Here’s the MicroPython code for this project:
from machine import Pin, I2C
from dht import DHT22
from ssd1306 import SSD1306_I2C
dht_pin = Pin(15, Pin.IN, Pin.PULL_UP)
dht_sensor = DHT22(dht_pin)
i2c = I2C(0, scl=Pin(1), sda=Pin(0), freq=400000)
oled = SSD1306_I2C(128, 64, i2c)
while True:
dht_sensor.measure()
temp = dht_sensor.temperature()
hum = dht_sensor.humidity()
oled.fill(0)
oled.text("Temperature: {:.1f}C".format(temp), 0, 0)
oled.text("Humidity: {:.1f}%".format(hum), 0, 20)
oled.show()
sleep(2)
Servo Motor Control
Another example project is controlling a servo motor using the Raspberry Pi Pico. Servo motors are commonly used in robotics and automation projects to provide precise angular control.
Components:
– Raspberry Pi Pico
– Servo motor
– Breadboard and jumper wires
Here’s the C code for this project:
#include "pico/stdlib.h"
#include "hardware/pwm.h"
#define SERVO_PIN 15
void servo_write(uint8_t angle) {
uint16_t pulse_width = 500 + (angle * 2000) / 180;
pwm_set_gpio_level(SERVO_PIN, pulse_width);
}
int main() {
gpio_set_function(SERVO_PIN, GPIO_FUNC_PWM);
uint slice_num = pwm_gpio_to_slice_num(SERVO_PIN);
pwm_set_wrap(slice_num, 20000);
pwm_set_clkdiv(slice_num, 39.0f); // 50Hz
pwm_set_enabled(slice_num, true);
while (true) {
for (uint8_t angle = 0; angle <= 180; angle++) {
servo_write(angle);
sleep_ms(10);
}
for (uint8_t angle = 180; angle > 0; angle--) {
servo_write(angle);
sleep_ms(10);
}
}
}
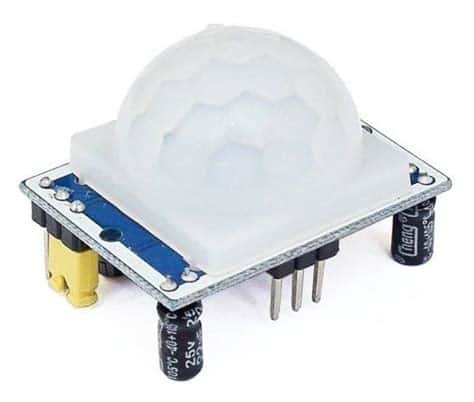
Conclusion
The Raspberry Pi Pico is a powerful and versatile microcontroller that opens up new possibilities for embedded systems projects. With its support for MicroPython and C/C++ programming, as well as its extensive GPIO capabilities, the Pico is well-suited for a wide range of applications.
Whether you’re a beginner looking to get started with microcontrollers or an experienced developer seeking a low-cost platform for your next project, the Raspberry Pi Pico is definitely worth considering.
FAQ
What is the difference between the Raspberry Pi Pico and other Raspberry Pi models?
The Raspberry Pi Pico is a microcontroller board, while other Raspberry Pi models (such as the Raspberry Pi 4) are single-board computers. Microcontrollers are designed for low-power, real-time applications, while single-board computers run full operating systems and are capable of more complex tasks.
Can I use the Raspberry Pi Pico with Arduino libraries?
While the Raspberry Pi Pico is not directly compatible with Arduino libraries, many Arduino libraries have been ported to work with the Pico using the Arduino-Pico framework. This allows users to leverage the extensive Arduino ecosystem while taking advantage of the Pico’s unique features.
What programming languages can I use with the Raspberry Pi Pico?
The Raspberry Pi Pico supports programming in MicroPython and C/C++. MicroPython is a beginner-friendly language that allows for rapid prototyping, while C/C++ offers more advanced control over the hardware for experienced developers.
How do I connect sensors and peripherals to the Raspberry Pi Pico?
The Raspberry Pi Pico has 26 GPIO pins that can be used to connect sensors and peripherals using protocols like I2C, SPI, and UART. The specific connections will depend on the device being used, but the Pico’s documentation provides detailed information on how to interface with common sensors and peripherals.
Where can I find more resources and examples for the Raspberry Pi Pico?
The Raspberry Pi Foundation provides extensive documentation and resources for the Pico on their website, including datasheets, schematics, and example code. Additionally, there is a growing community of Pico users who share their projects and experiences on platforms like GitHub and the Raspberry Pi forums.
No responses yet