Table of Contents
- Introduction to DTMF
- MT8870 Features
- MT8870 Pinout
- Decoding DTMF with the MT8870
- Circuit Diagram
- Component List
- Microcontroller Interface
- Applications
- Troubleshooting
- FAQs
- Conclusion
Introduction to DTMF
Dual-tone multi-frequency (DTMF) is a signaling system used in telephone systems to transmit the digits 0-9, the letters A-D, and the symbols # and *. Each digit or symbol is represented by a unique pair of audio frequencies, one from a low group and one from a high group:
1209 Hz | 1336 Hz | 1477 Hz | 1633 Hz | |
---|---|---|---|---|
697 Hz | 1 | 2 | 3 | A |
770 Hz | 4 | 5 | 6 | B |
852 Hz | 7 | 8 | 9 | C |
941 Hz | * | 0 | # | D |
When a button is pressed on a DTMF keypad, the corresponding pair of tones is generated. A DTMF decoder, like the MT8870, can detect these tones and determine which digit or symbol was pressed.
MT8870 Features
The MT8870 is a complete DTMF receiver that integrates both band split filter and decoder functions into a single 18-pin DIP or SOIC package. Its key features include:
- Operation from a single 3.5V to 5.5V supply
- Both 3.58MHz and 4.0MHz crystals supported
- On-chip oscillator and clock generator
- On-chip high and low group bandpass filters
- Adjustable guard time and steering threshold for noise immunity
- Power-down mode for low power consumption
- Inhibit mode for preventing invalid signal output
- Open drain outputs (Q1-Q4) that interface easily with a microcontroller
- StD (delayed steering) output to simplify timing
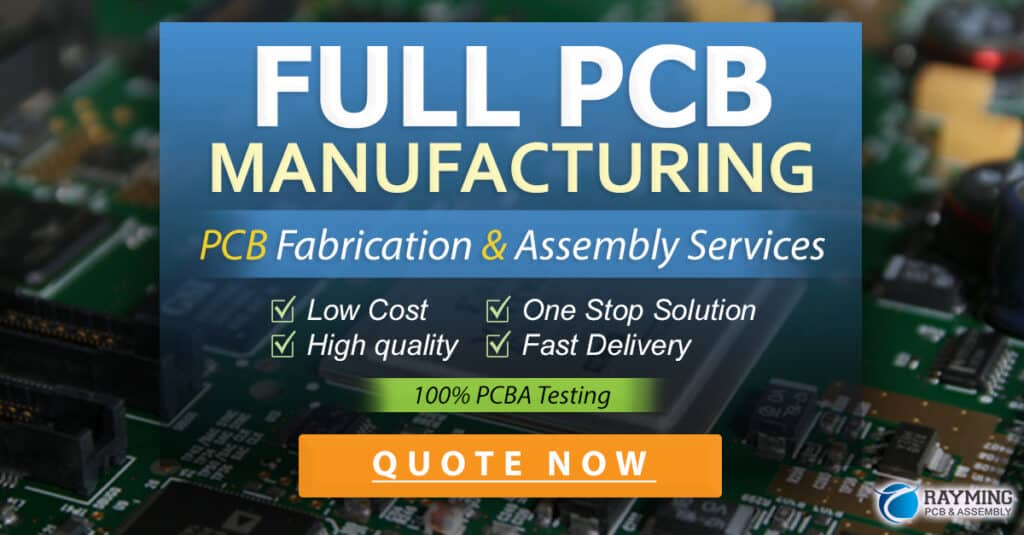
MT8870 Pinout
The MT8870 comes in both 18-pin DIP and SOIC packages. The pinout is as follows:
Pin | Symbol | Function |
---|---|---|
1 | XIN | Clock input (crystal or external) |
2 | XOUT | Clock output (crystal) |
3 | PWDN | Power down (active high) |
4 | GT | Guard time adjust |
5 | In+ | Non-inverting audio input |
6 | In- | Inverting audio input |
7 | GND | Ground |
8 | VREF | Voltage reference output |
9 | ESt | Early steering output |
10 | StD | Delayed steering output |
11 | TOE | Three data bits enable (active high) |
12 | Q4 | Data output D4 |
13 | Q3 | Data output D3 |
14 | Q2 | Data output D2 |
15 | Q1 | Data output D1 |
16 | INH | Inhibit (active high) |
17 | OSC1 | Oscillator input |
18 | VDD | Positive supply (3.5V to 5.5V) |
Decoding DTMF with the MT8870
To decode DTMF signals using the MT8870, you’ll need to build a simple circuit and interface it with a microcontroller. Here’s a step-by-step guide:
Circuit Diagram
MT8870
┌─────────┐
┌────┤1 XIN ├──────┐
│ └─────────┘ │
│ ┌─────────┐ │
└────┤2 XOUT ├──────┘
└─────────┘
┌─────────┐
VDD ───────┤18 VDD ├───────── +5V
└─────────┘
┌─────────┐
Power Down ─────┤3 PWDN ├─────┐
└─────────┘ │
┌─────────┐ │
Guard Time ───────┤4 GT ├─────┘
└─────────┘
┌──────┐┌─────────┐
Audio─┤ C1 ││5 In+ ├─── Audio Input
└──┬───┘└─────────┘
│
│ ┌─────────┐
└────┤6 In- ├─── GND
└─────────┘
┌─────────┐
──────┤7 GND ├──────── GND
└─────────┘
┌─────────┐
Voltage Ref. ────┤8 VREF ├───────
└─────────┘
┌─────────┐
Early Steer ────┤9 ESt ├───────
└─────────┘
┌─────────┐
Delayed Steer ───┤10 StD ├───── To MCU
└─────────┘
┌─────────┐
──────┤11 TOE ├─────── VDD
└─────────┘
┌─────────┐ ┌─────┐
Q4 ─────────┤12 Q4 ├─────┤ │
└─────────┘ │ │
┌─────────┐ │ To │
Q3 ─────────┤13 Q3 ├─────┤ MCU │
└─────────┘ │ │
┌─────────┐ │ │
Q2 ─────────┤14 Q2 ├─────┤ │
└─────────┘ │ │
┌─────────┐ └─────┘
Q1 ─────────┤15 Q1 ├───────────
└─────────┘
┌─────────┐
Inhibit ───────┤16 INH ├───────── GND
└─────────┘
┌─────────┐
Osc ────────┤17 OSC1 ├──────── 3.58 MHz XTAL
└─────────┘
Component List
- MT8870 DTMF Decoder IC
- 3.58 MHz Crystal
- 100 nF Capacitor (C1)
- 100 kΩ Resistor (Rx)
- Microcontroller (e.g., Arduino, PIC, etc.)
Microcontroller Interface
To read the decoded DTMF data from the MT8870, connect the Q1-Q4 outputs to input pins on your microcontroller. When a valid DTMF tone is detected, the StD output will go high, and the decoded digit will be available on Q1-Q4 in binary format:
DTMF | Q4 | Q3 | Q2 | Q1 |
---|---|---|---|---|
1 | 0 | 0 | 0 | 1 |
2 | 0 | 0 | 1 | 0 |
3 | 0 | 0 | 1 | 1 |
4 | 0 | 1 | 0 | 0 |
5 | 0 | 1 | 0 | 1 |
6 | 0 | 1 | 1 | 0 |
7 | 0 | 1 | 1 | 1 |
8 | 1 | 0 | 0 | 0 |
9 | 1 | 0 | 0 | 1 |
0 | 1 | 0 | 1 | 0 |
* | 1 | 0 | 1 | 1 |
# | 1 | 1 | 0 | 0 |
A | 1 | 1 | 0 | 1 |
B | 1 | 1 | 1 | 0 |
C | 1 | 1 | 1 | 1 |
D | 0 | 0 | 0 | 0 |
Here’s some sample code for reading DTMF data using an Arduino:
#define StD 2
#define Q1 3
#define Q2 4
#define Q3 5
#define Q4 6
void setup() {
pinMode(StD, INPUT);
pinMode(Q1, INPUT);
pinMode(Q2, INPUT);
pinMode(Q3, INPUT);
pinMode(Q4, INPUT);
Serial.begin(9600);
}
void loop() {
if (digitalRead(StD) == HIGH) {
int data = digitalRead(Q1) |
digitalRead(Q2) << 1 |
digitalRead(Q3) << 2 |
digitalRead(Q4) << 3;
Serial.print("Decoded DTMF: ");
switch (data) {
case 0: Serial.println("1"); break;
case 1: Serial.println("2"); break;
case 2: Serial.println("3"); break;
case 3: Serial.println("4"); break;
case 4: Serial.println("5"); break;
case 5: Serial.println("6"); break;
case 6: Serial.println("7"); break;
case 7: Serial.println("8"); break;
case 8: Serial.println("9"); break;
case 9: Serial.println("0"); break;
case 10: Serial.println("*"); break;
case 11: Serial.println("#"); break;
case 12: Serial.println("A"); break;
case 13: Serial.println("B"); break;
case 14: Serial.println("C"); break;
case 15: Serial.println("D"); break;
}
delay(100); // Debounce
}
}
Applications
The MT8870 DTMF decoder has a wide range of applications, including:
- Telephone systems and PBXs
- Interactive voice response (IVR) systems
- Remote control and monitoring
- Two-factor authentication
- Amateur radio repeater control
- Home automation
- Robotics
By decoding DTMF tones, the MT8870 enables devices to respond to user input from a standard telephone keypad, making it a versatile choice for many projects.
Troubleshooting
If you’re having trouble getting your MT8870 circuit to work, here are a few things to check:
- Verify that your crystal frequency matches the one specified in the datasheet for your MT8870 variant (3.58 MHz or 4.0 MHz).
- Check that your audio input signal is within the recommended range (30 mVrms to 3 Vrms) and has minimal noise.
- Ensure that the power supply voltage is between 3.5V and 5.5V.
- Double-check your connections, especially the Q1-Q4 and StD outputs to your microcontroller.
- If using a breadboard, try cleaning the contacts or moving to a different section of the board.
- Use an oscilloscope or logic analyzer to verify that the StD output is going high when a DTMF tone is present and that the Q1-Q4 outputs are changing accordingly.
FAQs
-
Q: Can the MT8870 decode multiple DTMF tones simultaneously?
A: No, the MT8870 can only decode one DTMF tone at a time. If multiple tones are present, the decoder may produce incorrect results or no output at all. -
Q: What is the maximum input signal level for the MT8870?
A: The maximum input signal level is 3 Vrms. Exceeding this level may cause distortion or damage to the decoder. -
Q: How can I adjust the guard time on the MT8870?
A: The guard time can be adjusted by connecting a resistor (Rx) between the GT pin and ground. The resistor value determines the guard time, with larger values resulting in longer guard times. Consult the datasheet for specific resistor values and corresponding guard times. -
Q: What is the purpose of the TOE pin on the MT8870?
A: The TOE (three data bits enable) pin, when connected to VDD, configures the MT8870 to output only three data bits (Q1-Q3) instead of four. This can be useful in applications where only the digits 0-9 need to be decoded. -
Q: Can I use the MT8870 with a microcontroller that operates at 3.3V?
A: Yes, the MT8870 can operate with a supply voltage as low as 3.5V, making it compatible with 3.3V microcontrollers. However, ensure that the microcontroller’s input pins are 5V tolerant or use appropriate level-shifting circuitry.
Conclusion
The MT8870 is a versatile and easy-to-use DTMF decoder that can add touch-tone input capabilities to a wide range of projects. By understanding its features, pinout, and how to interface it with a microcontroller, you can quickly integrate the MT8870 into your designs. Whether you’re working on a telephone system, remote control, or home automation project, the MT8870 is a reliable choice for decoding DTMF tones.
No responses yet