Introduction to LoPy
LoPy is a powerful IoT development platform that combines the ease of use of Python programming with the versatility and efficiency of the ESP32 microcontroller. Developed by PyCom, LoPy is designed to simplify the process of creating IoT applications by providing a comprehensive set of tools and features that enable developers to quickly prototype and deploy their projects.
Key Features of LoPy
Feature | Description |
---|---|
Micropython | LoPy supports Micropython, a lightweight and efficient implementation of Python 3 optimized for microcontrollers. |
ESP32 Microcontroller | LoPy is powered by the ESP32 microcontroller, which offers dual-core processing, Wi-Fi, Bluetooth, and various peripherals. |
Multiple Network Protocols | LoPy supports a wide range of network protocols, including Wi-Fi, Bluetooth, LoRa, and Sigfox. |
Low Power Consumption | LoPy is designed for low power consumption, making it suitable for battery-powered applications. |
Expandable with Shields | LoPy can be expanded with various shields, such as the PyTrack, PySense, and PyScan, to add additional functionality. |
Hardware Specifications
ESP32 Microcontroller
At the heart of LoPy is the ESP32 microcontroller, which provides the following features:
- Dual-core Xtensa LX6 microprocessor, running at 240 MHz
- 520 KB SRAM
- 4 MB flash memory
- Wi-Fi (802.11 b/g/n)
- Bluetooth Classic and Bluetooth Low Energy (BLE)
- Multiple peripherals (I2C, SPI, UART, ADC, DAC, PWM, etc.)
LoPy Pin Layout
LoPy has a compact form factor with a pin layout that includes:
- 24 GPIO pins
- 2 UART interfaces
- 2 SPI interfaces
- 2 I2C interfaces
- 3 ADC channels
- 2 DAC channels
- 8 PWM channels
Power Management
LoPy has a built-in power management system that enables low power consumption and long battery life. It supports the following power modes:
Power Mode | Description |
---|---|
Active Mode | The microcontroller is fully operational, and all peripherals are available. |
Sleep Mode | The microcontroller enters a low-power state, while retaining RAM contents and allowing the RTC and certain peripherals to operate. |
Deep Sleep Mode | The microcontroller enters an ultra-low-power state, where RAM contents are lost, and only the RTC and specific GPIO pins can wake the device. |
Software Development with LoPy
Micropython
LoPy supports Micropython, a lean and efficient implementation of Python 3 designed for microcontrollers. Micropython provides a familiar Python syntax and a subset of the Python standard library, making it easy for developers with Python experience to get started with LoPy.
Some key features of Micropython on LoPy include:
- Support for Python 3.4 syntax
- Access to LoPy-specific libraries for networking, sensors, and other peripherals
- Interactive REPL (Read-Eval-Print Loop) for quick testing and debugging
- Ability to run scripts from the built-in flash memory or an external SD card
Pymakr IDE
To simplify the development process, PyCom provides the Pymakr IDE, a free and cross-platform integrated development environment for LoPy and other PyCom devices. Pymakr offers the following features:
- Syntax highlighting and auto-completion for Micropython
- Built-in terminal for REPL access and debugging
- Support for uploading and running scripts on LoPy
- Integration with version control systems like Git
- Available as a standalone application or a plugin for Visual Studio Code and Atom
LoPy Libraries
LoPy comes with a set of built-in libraries that provide access to various features and peripherals of the device. Some of the key libraries include:
Library | Description |
---|---|
machine |
Provides access to the hardware peripherals, such as GPIO, I2C, SPI, and UART. |
network |
Enables the use of Wi-Fi, Bluetooth, and other network protocols. |
pycom |
Offers LoPy-specific functions and constants. |
utime |
Provides time-related functions, such as delays and timers. |
uos |
Allows access to the file system and basic OS functions. |
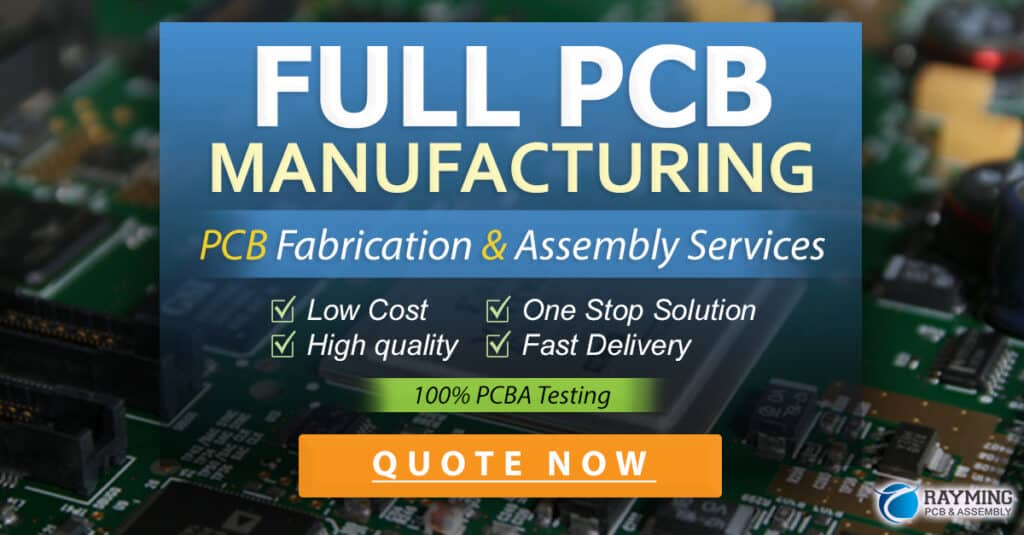
Networking with LoPy
One of the key strengths of LoPy is its support for multiple network protocols, which enables developers to create IoT applications that can communicate with a wide range of devices and services.
Wi-Fi
LoPy supports 802.11 b/g/n Wi-Fi, allowing it to connect to or create Wi-Fi networks. The network
library provides classes and methods for configuring and managing Wi-Fi connections, such as:
- Scanning for available networks
- Connecting to a Wi-Fi network
- Creating a Wi-Fi access point
- Retrieving network information (IP address, MAC address, etc.)
Example code for connecting to a Wi-Fi network:
from network import WLAN
wlan = WLAN(mode=WLAN.STA)
wlan.connect('your_ssid', auth=(WLAN.WPA2, 'your_password'))
while not wlan.isconnected():
pass
print('Connected to Wi-Fi:', wlan.ifconfig())
Bluetooth
LoPy supports both Bluetooth Classic and Bluetooth Low Energy (BLE). The bluetooth
library allows developers to use LoPy as a Bluetooth peripheral or central device, enabling communication with other Bluetooth devices, such as smartphones, tablets, or sensors.
Example code for creating a Bluetooth Low Energy peripheral:
from network import Bluetooth
bluetooth = Bluetooth()
bluetooth.set_advertisement(name='My LoPy', service_uuid=b'1234567890123456')
def conn_cb (bt_o):
events = bt_o.events()
if events & Bluetooth.CLIENT_CONNECTED:
print("Client connected")
elif events & Bluetooth.CLIENT_DISCONNECTED:
print("Client disconnected")
bluetooth.callback(trigger=Bluetooth.CLIENT_CONNECTED | Bluetooth.CLIENT_DISCONNECTED, handler=conn_cb)
bluetooth.advertise(True)
LoRa
LoRa is a long-range, low-power wireless communication protocol designed for IoT applications. LoPy supports LoRa through the LoRa
class in the network
library, enabling developers to create LoRa networks or connect to existing ones, such as The Things Network (TTN).
Example code for sending data via LoRa:
from network import LoRa
import socket
lora = LoRa(mode=LoRa.LORA, frequency=868000000)
s = socket.socket(socket.AF_LORA, socket.SOCK_RAW)
s.setblocking(True)
s.send('Hello, LoRa!')
s.close()
Sigfox
Sigfox is another low-power, long-range network protocol supported by LoPy. The Sigfox
class in the network
library allows developers to send and receive messages using the Sigfox network.
Example code for sending data via Sigfox:
from network import Sigfox
import socket
sigfox = Sigfox(mode=Sigfox.SIGFOX, rcz=Sigfox.RCZ1)
s = socket.socket(socket.AF_SIGFOX, socket.SOCK_RAW)
s.setblocking(True)
s.send('Hello, Sigfox!')
s.close()
Shields and Expansions
LoPy can be extended with various shields and expansions that add additional functionality to the device. Some popular shields include:
PyTrack
PyTrack is a shield that adds GPS and accelerometer capabilities to LoPy. It features a GPS receiver and a 3-axis accelerometer, enabling developers to create location-aware and motion-sensing applications.
Feature | Description |
---|---|
GPS | u-blox MAX-M8Q GPS receiver with integrated antenna |
Accelerometer | ST LIS2HH12 3-axis accelerometer |
Backup Battery | CR1220 coin cell battery for RTC backup and GPS RAM retention |
PySense
PySense is a shield that provides a range of Environmental Sensors, including temperature, humidity, pressure, light, and acceleration. It is designed for applications that require monitoring of ambient conditions, such as weather stations or smart home devices.
Feature | Description |
---|---|
Temperature and Humidity | SI7006-A20 sensor |
Pressure | MPL3115A2 sensor |
Light | LTR-329ALS-01 sensor |
Acceleration | ST LIS2HH12 3-axis accelerometer |
PyScan
PyScan is a shield that adds RFID and NFC capabilities to LoPy. It features an MFRC630 RFID/NFC reader, allowing developers to create applications that can read and write to RFID tags and NFC devices.
Feature | Description |
---|---|
RFID/NFC | NXP MFRC630 reader |
Frequency | 13.56 MHz |
Standards | ISO/IEC 14443 A/B, ISO/IEC 15693, ISO/IEC 18000-3, NFC Forum Type 1-5 |
Real-World Applications
LoPy’s versatility and support for multiple network protocols make it suitable for a wide range of IoT applications. Some examples include:
Smart Agriculture
- Monitoring soil moisture, temperature, and humidity
- Controlling irrigation systems based on sensor data
- Tracking livestock with GPS and LoRa
Industrial Automation
- Monitoring machine performance and energy consumption
- Predictive maintenance based on sensor data
- Remote control of industrial equipment
Smart Cities
- Environmental monitoring (air quality, noise levels, etc.)
- Smart parking systems with RFID/NFC
- Waste management with fill-level sensors and LoRa
Home Automation
- Smart thermostats and HVAC control
- Lighting control based on ambient light levels
- Security systems with motion detection and Bluetooth connectivity
Getting Started with LoPy
To start developing with LoPy, follow these steps:
- Purchase a LoPy device and any necessary shields or expansions.
- Install the Pymakr IDE on your computer.
- Connect your LoPy to your computer via USB.
- Open Pymakr and create a new project.
- Write your Micropython code using the LoPy libraries and features.
- Upload and run your code on the LoPy device.
For more detailed tutorials and examples, refer to the official PyCom documentation and community resources.
FAQ
What is the difference between LoPy and other PyCom devices?
LoPy is one of several devices offered by PyCom, each with different features and capabilities. Other devices include WiPy (focused on Wi-Fi), SiPy (focused on Sigfox), and GPy (focused on cellular connectivity). LoPy stands out for its support of multiple network protocols, including Wi-Fi, Bluetooth, LoRa, and Sigfox.
Can I use LoPy without any shields or expansions?
Yes, LoPy can be used as a standalone device without any additional shields or expansions. However, shields like PyTrack, PySense, and PyScan can greatly extend the capabilities of LoPy and make it easier to develop certain types of applications.
Is LoPy suitable for beginners?
While some prior experience with Python and electronics can be helpful, LoPy is designed to be beginner-friendly. The use of Micropython and the Pymakr IDE makes it easier for developers to get started with LoPy compared to other microcontroller platforms that require more low-level programming.
What is the maximum range of LoRa and Sigfox on LoPy?
The maximum range of LoRa and Sigfox on LoPy depends on various factors, such as the environment, antenna design, and transmission power. In ideal conditions, LoRa can achieve a range of up to 10 km, while Sigfox can reach up to 50 km. However, in urban areas or environments with obstacles, the range may be significantly lower.
Can I use LoPy for commercial projects?
Yes, LoPy can be used for both personal and commercial projects. PyCom offers a range of services and support options for commercial customers, including custom firmware development, hardware design, and certification assistance.
No responses yet