Table of Contents
- Introduction to Line Follower Robots
- Components of a Line Follower Robot
- Building a Line Follower Robot with Arduino
- Creating a Line Follower Robot using Raspberry Pi
- Implementing Line Following with Microcontrollers
- Advanced Techniques and Enhancements
- Troubleshooting Common Issues
- Applications and Future Scope
- Frequently Asked Questions (FAQ)
- Conclusion
Introduction to Line Follower Robots
A line follower robot is an autonomous vehicle that follows a predefined path, typically a black line on a white background or a white line on a black background. These robots rely on sensors to detect the contrast between the line and the surrounding surface, enabling them to navigate along the path.
The basic principle behind line following is the use of reflectance sensors, which measure the amount of light reflected from the surface. When the sensor is positioned over a dark line, it receives less reflected light compared to when it is over a lighter surface. By strategically placing multiple sensors and processing their readings, the robot can determine its position relative to the line and make appropriate adjustments to its movement.
Line follower robots have found numerous applications in various domains. They are commonly used in industrial settings for material handling, product transportation, and automated guided vehicles (AGVs). In warehouses, line follower robots can efficiently navigate through aisles and retrieve items. Additionally, line following is often used as an introductory project in robotics education to teach basic concepts of sensor integration, motor control, and programming.
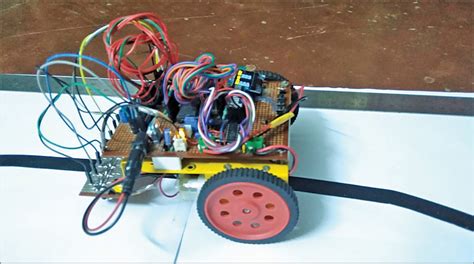
Components of a Line Follower Robot
To build a line follower robot, several essential components are required. Let’s discuss each component in detail:
1. Microcontroller or Single-Board Computer
The heart of a line follower robot is a microcontroller or a single-board computer that acts as the brain of the system. It processes the sensor data, makes decisions based on the programmed algorithm, and controls the robot’s movement. Popular choices for this component include:
- Arduino: Arduino is an open-source microcontroller platform that is widely used in robotics projects. It offers a simple programming environment and a wide range of compatible sensors and actuators.
- Raspberry Pi: Raspberry Pi is a single-board computer that runs a full-fledged operating system, making it suitable for more complex projects. It provides higher processing power and connectivity options compared to Arduino.
- Other Microcontrollers: Various other microcontrollers, such as PIC, AVR, or STM32, can also be used depending on the specific requirements and preferences of the project.
2. Reflectance Sensors
Reflectance sensors, also known as line sensors or IR sensors, are the eyes of a line follower robot. These sensors consist of an infrared (IR) emitter and an IR receiver. The emitter sends out infrared light, and the receiver measures the amount of light reflected back from the surface.
Commonly used reflectance sensors for line following include:
– QTR-8A: This sensor module consists of eight IR reflectance sensors arranged in a linear array. It provides analog output values corresponding to the reflected light intensity.
– TCRT5000: TCRT5000 is a single reflectance sensor that can be used in pairs or arrays to detect the line. It offers adjustable sensitivity and is commonly used in DIY projects.
– IR Proximity Sensors: Some projects utilize IR proximity sensors, such as the Sharp GP2Y0A21YK, which provide distance measurements based on the reflected IR light.
The choice of reflectance sensors depends on factors such as the desired resolution, sensor placement, and the specific line following algorithm being implemented.
3. Motors and Motor Driver
To move the line follower robot, motors are used in conjunction with a motor driver. The most common motor types used in line follower robots are DC motors and servo motors.
- DC Motors: DC motors are simple and easy to control. They require a motor driver, such as the L298N or L293D, to regulate the voltage and current supplied to the motors based on the microcontroller’s commands.
- Servo Motors: Servo motors are precise and offer accurate position control. They have built-in circuitry that allows them to be directly controlled by the microcontroller using pulse-width modulation (PWM) signals.
The choice between DC motors and servo motors depends on the desired speed, torque, and precision of the line follower robot.
4. Chassis and Wheels
The chassis provides the structural framework for the line follower robot, while the wheels enable its movement. The chassis can be made from various materials, such as acrylic, wood, or 3D-printed plastic. It should be sturdy enough to hold all the components securely.
The wheels are typically made of rubber or plastic and are attached to the motors. The number of wheels and their arrangement can vary based on the design of the robot. Common configurations include two-wheel drive with a caster wheel for balance, or four-wheel drive for enhanced stability.
5. Power Source
A reliable power source is essential to keep the line follower robot running. The most common power sources used are batteries, such as rechargeable lithium-ion batteries or disposable alkaline batteries. The choice of battery depends on the voltage and current requirements of the motors and other components.
It’s important to include a voltage regulator or a dedicated battery management system to ensure stable and safe power delivery to the components.
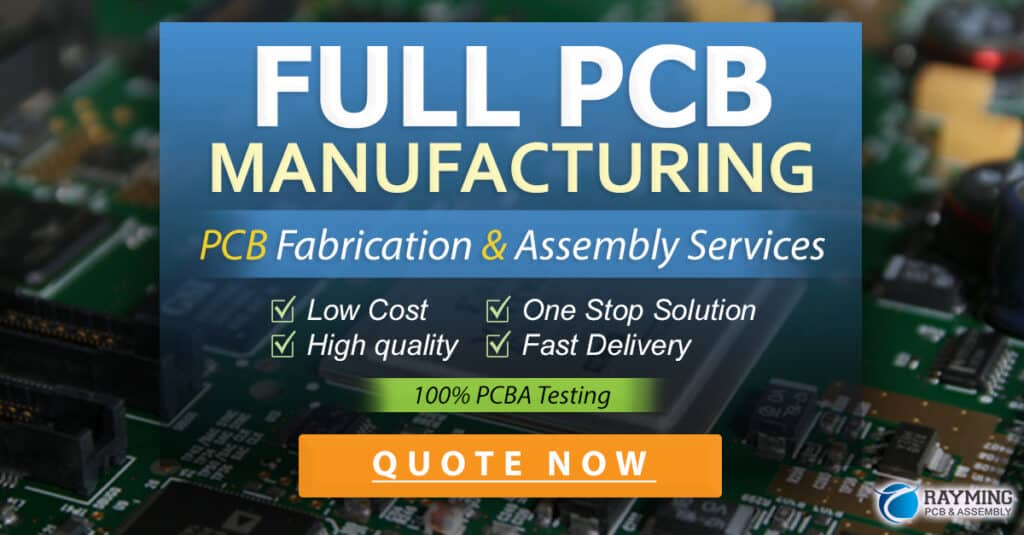
Building a Line Follower Robot with Arduino
Arduino is a popular choice for building line follower robots due to its simplicity and extensive community support. Let’s walk through the steps to create a basic line follower robot using Arduino.
Step 1: Gather the Components
To build an Arduino-based line follower robot, you will need the following components:
– Arduino board (e.g., Arduino Uno)
– Motor driver (e.g., L298N)
– Two DC motors
– Two wheels
– Caster wheel
– Reflectance sensors (e.g., QTR-8A)
– Battery pack
– Jumper wires
– Breadboard (optional)
Step 2: Connect the Components
- Connect the motor driver to the Arduino board. The motor driver’s input pins should be connected to the Arduino’s digital pins, while the output pins should be connected to the DC motors.
- Connect the reflectance sensors to the Arduino’s analog pins. If using the QTR-8A sensor, you can connect the sensor’s pins to consecutive analog pins on the Arduino.
- Connect the battery pack to the motor driver’s power input. Make sure to follow the voltage and current requirements of the motor driver and motors.
Step 3: Upload the Code
- Open the Arduino IDE and create a new sketch.
- Include the necessary libraries for the reflectance sensors and motor control.
- Initialize the pins for the sensors and motors.
- Write the code to read the sensor values and control the motors based on the line following algorithm. A simple algorithm could be:
- If the left sensor detects the line, turn left.
- If the right sensor detects the line, turn right.
- If both sensors detect the line, move forward.
- If no sensors detect the line, stop.
- Upload the code to the Arduino board.
Step 4: Test and Calibrate
- Place the line follower robot on a surface with a contrasting line.
- Power on the robot and observe its behavior.
- If necessary, adjust the sensor thresholds or motor speeds to achieve optimal line following performance.
- Fine-tune the code and hardware until the robot follows the line accurately and smoothly.
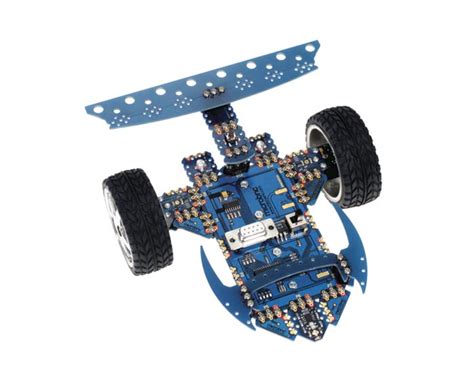
Creating a Line Follower Robot using Raspberry Pi
Raspberry Pi offers more advanced capabilities compared to Arduino, making it suitable for complex line following projects. Here’s a high-level overview of building a line follower robot with Raspberry Pi:
Step 1: Gather the Components
- Raspberry Pi board (e.g., Raspberry Pi 4)
- Motor driver (e.g., L298N)
- Two DC motors
- Two wheels
- Caster wheel
- Reflectance sensors (e.g., TCRT5000)
- Battery pack
- Jumper wires
- Breadboard (optional)
Step 2: Connect the Components
- Connect the motor driver to the Raspberry Pi’s GPIO pins. Use PWM-capable pins for motor speed control.
- Connect the reflectance sensors to the Raspberry Pi’s GPIO pins. You can use digital pins for simple binary detection or analog pins for more precise readings.
- Connect the battery pack to the motor driver’s power input, ensuring proper voltage and current supply.
Step 3: Set up the Raspberry Pi
- Install the necessary operating system on the Raspberry Pi (e.g., Raspbian).
- Configure the Raspberry Pi for remote access (e.g., SSH or VNC) if desired.
- Install the required libraries and dependencies for GPIO control and sensor interfacing (e.g., RPi.GPIO, wiringpi).
Step 4: Write the Code
- Choose a programming language supported by Raspberry Pi (e.g., Python, C++).
- Initialize the GPIO pins for the sensors and motors.
- Implement the line following algorithm, which may involve more advanced techniques such as PID control or machine learning.
- Test and refine the code until the robot exhibits accurate and stable line following behavior.
Implementing Line Following with Microcontrollers
Microcontrollers, such as PIC or AVR, can also be used to build line follower robots. The process is similar to using Arduino, but with some differences in programming and pin configurations. Here’s a brief overview:
- Select a suitable microcontroller (e.g., PIC16F877A, ATmega328P).
- Connect the motors, motor driver, and reflectance sensors to the microcontroller’s pins.
- Set up the development environment for the chosen microcontroller (e.g., MPLAB X IDE for PIC, Atmel Studio for AVR).
- Write the code in the microcontroller’s native language (e.g., C) or use a high-level language with a compatible compiler.
- Implement the line following algorithm, considering the specific features and limitations of the microcontroller.
- Program the microcontroller and test the line follower robot.
Advanced Techniques and Enhancements
Once you have a basic line follower robot up and running, there are several advanced techniques and enhancements you can explore to improve its performance and functionality. Some ideas include:
- PID Control: Implement a PID (Proportional-Integral-Derivative) controller to achieve smoother and more precise line following. PID control helps minimize deviations from the line and provides better stability.
- Adaptive Thresholding: Develop an adaptive thresholding algorithm that dynamically adjusts the sensor thresholds based on the lighting conditions and surface variations. This enhances the robot’s ability to follow lines in different environments.
- Intersection Detection: Add logic to detect and handle intersections or junctions in the line. This allows the robot to make decisions and navigate through more complex paths.
- Wireless Control: Integrate wireless communication modules (e.g., Bluetooth, Wi-Fi) to control the robot remotely or send commands for different behaviors.
- Obstacle Avoidance: Incorporate additional sensors (e.g., ultrasonic sensors) to detect obstacles and implement obstacle avoidance algorithms. This enables the robot to navigate around obstacles while following the line.
- Machine Learning: Apply machine learning techniques, such as reinforcement learning or neural networks, to improve the robot’s decision-making and adaptability. This can help the robot learn from its experiences and optimize its line following performance over time.
Troubleshooting Common Issues
When building and testing a line follower robot, you may encounter various issues. Here are some common problems and troubleshooting tips:
- Robot not following the line:
- Check the sensor connections and ensure they are properly wired.
- Verify that the sensors are positioned correctly and at the appropriate height from the surface.
- Adjust the sensor thresholds in the code to match the lighting conditions and line contrast.
-
Ensure that the motors are functioning properly and respond to the control signals.
-
Robot wobbling or veering off the line:
- Check the wheel alignment and ensure they are properly attached to the motors.
- Balance the weight distribution of the components on the chassis.
- Tune the motor speeds and adjust the turning angles in the code.
-
Implement PID control or other stabilization techniques.
-
Inconsistent sensor readings:
- Ensure that the sensors are clean and free from dust or debris.
- Verify that the sensors are not being interfered with by external light sources.
- Use shielded cables or filter capacitors to reduce electrical noise.
-
Implement software filtering techniques, such as averaging or median filtering, to smooth out the sensor readings.
-
Battery issues:
- Make sure the batteries are fully charged and provide the required voltage and current.
- Use a voltage regulator or a dedicated battery management system to ensure stable power supply.
- Monitor the battery level and implement low-battery warnings or automatic shutdown mechanisms.
Remember to approach troubleshooting systematically, isolating each component and testing them individually to identify the root cause of the issue.
Applications and Future Scope
Line follower robots have a wide range of applications and offer exciting possibilities for future development. Some notable applications include:
- Industrial Automation:
- Material handling and transportation in factories and warehouses.
- Automated guided vehicles (AGVs) for efficient logistics and inventory management.
-
Quality control and inspection processes.
-
Agriculture:
- Autonomous navigation in agricultural fields for planting, harvesting, and monitoring crops.
-
Precision agriculture techniques using line following robots for targeted fertilization and pest control.
-
Healthcare:
- Delivery of medical supplies and equipment in hospitals and clinics.
-
Autonomous navigation in assisted living facilities for monitoring and assisting elderly or disabled individuals.
-
Education and Research:
- Teaching robotics concepts and programming skills to students.
- Conducting research in areas such as robot navigation, sensor fusion, and machine learning.
As technology advances, the future scope of line follower robots is promising. Some potential future developments include:
- Integration with AI and computer vision for more intelligent navigation and decision-making.
- Collaboration with other robots or systems for complex tasks and swarm robotics applications.
- Miniaturization and optimization for deployment in space exploration missions or medical procedures.
- Adaptation to different environments and surfaces, such as uneven terrain or underwater.
Frequently Asked Questions (FAQ)
-
What is the difference between a line follower robot and a maze-solving robot?
A line follower robot is designed to follow a predefined path, typically a contrasting line on a surface. It uses sensors to detect the line and navigate along it. On the other hand, a maze-solving robot is capable of navigating through a maze by exploring different paths, making decisions at intersections, and finding the optimal route to reach the goal. -
Can a line follower robot be used on any surface?
Line follower robots work best on surfaces with a clear contrast between the line and the background. The surface should be relatively flat and non-reflective to ensure accurate sensor readings. Commonly used surfaces include white paper with black lines or black surfaces with white lines. Adjustments may be needed for different surface colors or textures. -
How accurate are line follower robots?
The accuracy of a line follower robot depends on several factors, including the quality of the sensors, the robustness of the line following algorithm, and the calibration of the robot. With proper design and tuning, line follower robots can achieve high accuracy, staying within a few millimeters of the line. However, external factors such as lighting conditions and surface irregularities can impact the accuracy. -
What is the maximum speed a line follower robot can achieve?
The maximum speed of a line follower robot depends on the specific design, motors, and control algorithms used. Factors such as the weight of the robot, the type of motors, and the processing speed of the microcontroller or single-board computer also play a role. Typical line follower robots can achieve speeds ranging from a few centimeters per second to over a meter per second, depending on the application requirements. -
Can line follower robots be used for outdoor applications?
Line follower robots are primarily designed for indoor use on controlled surfaces. Outdoor environments present challenges such as uneven terrain, varying lighting conditions, and weather factors that can affect the performance of the sensors and the robot’s stability. While it is possible to adapt line follower robots for outdoor use
No responses yet