What is a Validation Error?
A validation error is an error that occurs when data entered into a system does not meet the specified criteria or rules. These rules are put in place to ensure that the data being processed is accurate, consistent, and meets the requirements of the system.
Validation errors can occur in various contexts, such as:
- Web forms
- Database queries
- API requests
- Data imports
- File uploads
When a validation error occurs, it typically means that the data being submitted is invalid in some way. This could be due to a variety of reasons, such as:
- Required fields being left blank
- Data entered in the wrong format (e.g. text instead of numbers)
- Data exceeding maximum allowed length
- Data not matching a specified pattern (e.g. email address)
- Duplicate data being submitted
Validation errors are an important part of any software system, as they help ensure data integrity and prevent incorrect or malicious data from being processed. However, they can also be a source of frustration for users if not handled properly.
Common Types of Validation Errors
There are many different types of validation errors that can occur, depending on the specific requirements of your system. Here are some of the most common types:
Required Field Validation
One of the most basic types of validation is ensuring that required fields are not left blank. For example, if you have a registration form that requires a user to enter their name and email address, you would want to ensure that both fields are filled out before allowing the form to be submitted.
Here’s an example of what a required field validation error might look like:
Error: Please enter your name.
Data Type Validation
Another common type of validation is ensuring that data is entered in the correct format. For example, if you have a field that expects a number, you would want to ensure that the user enters a valid number and not text.
Here’s an example of what a data type validation error might look like:
Error: Please enter a valid number.
Length Validation
Length validation ensures that data entered does not exceed a specified maximum length. This is often used for fields like usernames, passwords, or descriptions to prevent excessively long input.
Here’s an example of what a length validation error might look like:
Error: Username cannot exceed 20 characters.
Pattern Validation
Pattern validation ensures that data matches a specified pattern or format. This is often used for fields like email addresses, phone numbers, or zip codes.
Here’s an example of what a pattern validation error might look like:
Error: Please enter a valid email address.
Uniqueness Validation
Uniqueness validation ensures that data being submitted is unique and does not already exist in the system. This is often used for fields like usernames or email addresses to prevent duplicate accounts.
Here’s an example of what a uniqueness validation error might look like:
Error: Username already exists. Please choose a different username.
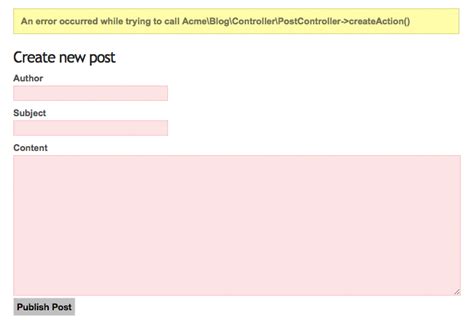
Strategies for Handling Validation Errors
Now that we’ve explored some common types of validation errors, let’s look at some strategies for handling them effectively.
Provide Clear Error Messages
One of the most important things you can do when handling validation errors is to provide clear and descriptive error messages. Your error messages should clearly explain what went wrong and how the user can fix it.
Here are some tips for writing effective error messages:
- Be specific about what went wrong
- Use plain language that is easy to understand
- Provide guidance on how to fix the error
- Highlight the specific field(s) that have errors
- Keep messages concise and to the point
Here’s an example of a clear and descriptive error message:
Error: The email address you entered is not valid. Please enter a valid email address in the format "example@domain.com".
Validate Early and Often
Another important strategy for handling validation errors is to validate data as early and often as possible. This means validating data on both the client side (in the user’s browser) and the server side.
Client-side validation can help catch errors before the form is even submitted, providing a better user experience. Server-side validation is still important, however, as it provides an additional layer of security and ensures that data is valid before being processed.
Here are some examples of when you might want to validate data:
- When a user types into a form field
- When a user submits a form
- When data is received by the server
- Before data is saved to a database
- Before data is used in a calculation or processed in some way
By validating data early and often, you can catch errors as soon as they occur and provide a better overall user experience.
Use Form Validation Libraries
If you’re working with web forms, there are many form validation libraries available that can help simplify the validation process. These libraries often provide pre-built validation rules and can handle displaying error messages and highlighting fields with errors.
Here are some popular form validation libraries:
- jQuery Validation
- Parsley.js
- Validate.js
- Formik (for React)
- Vuelidate (for Vue.js)
Using a form validation library can save you time and effort, as well as provide a more consistent and polished user experience.
Log and Monitor Validation Errors
Another important strategy for handling validation errors is to log and monitor them. This means keeping track of validation errors that occur in your system and analyzing them to identify patterns or common issues.
Here are some things you might want to log:
- Type of validation error
- Field(s) that caused the error
- User input that caused the error
- Time and date of the error
By logging validation errors, you can gain valuable insights into how your system is being used and identify areas for improvement. You can also use this data to prioritize bug fixes and feature enhancements.
Handle Errors Gracefully
Finally, it’s important to handle validation errors gracefully and provide a good user experience even when errors occur. This means displaying error messages in a clear and helpful way, as well as providing options for the user to correct their input.
Here are some tips for handling errors gracefully:
- Display error messages near the relevant fields
- Use color and icons to draw attention to errors
- Provide suggestions or examples of valid input
- Allow users to easily correct their input and resubmit
- Avoid blaming the user or using negative language
By handling errors gracefully, you can minimize user frustration and provide a better overall experience.
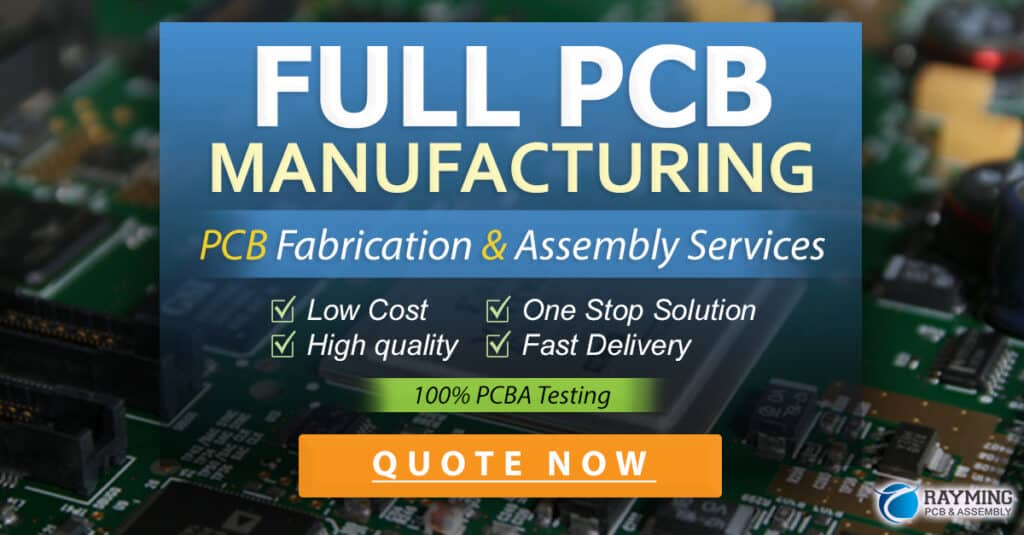
Frequently Asked Questions
What is the difference between client-side and server-side validation?
Client-side validation occurs in the user’s web browser, before the form data is submitted to the server. Server-side validation occurs on the server, after the form data has been submitted. Client-side validation can provide a better user experience, as errors can be caught and displayed immediately. However, server-side validation is still important for security and data integrity.
Can I use both client-side and server-side validation?
Yes, it’s actually recommended to use both client-side and server-side validation for the best results. Client-side validation can catch errors early and provide a better user experience, while server-side validation provides an additional layer of security and ensures that data is valid before being processed.
What should I do if I’m getting a lot of validation errors?
If you’re seeing a high number of validation errors, it may be a sign that there are issues with your form design or validation rules. Some things to consider:
- Are your validation rules too strict or complex?
- Are your form fields clearly labeled and easy to understand?
- Are you providing enough guidance or examples of valid input?
- Are there any technical issues causing validation errors (e.g. browser compatibility, network issues)?
By analyzing the validation errors and making adjustments to your form and validation rules, you can help reduce the number of errors and improve the user experience.
How can I test my validation rules?
Testing your validation rules is an important part of ensuring that they are working correctly and providing a good user experience. Here are some ways to test your validation rules:
- Manually test the form with different inputs and scenarios
- Use automated testing tools to simulate different inputs and check for expected results
- Ask users to test the form and provide feedback
- Monitor validation errors in production and analyze patterns or common issues
By thoroughly testing your validation rules, you can catch issues early and ensure a high-quality user experience.
What are some common validation rule mistakes to avoid?
Here are some common validation rule mistakes to watch out for:
- Overly strict or complex validation rules that are difficult for users to understand or satisfy
- Inconsistent validation rules across different forms or fields
- Lack of clear error messages or guidance on how to fix validation issues
- Failing to validate data on both the client side and server side
- Not logging or monitoring validation errors to identify issues and areas for improvement
By avoiding these common mistakes and following best practices for validation, you can create a more user-friendly and secure system.
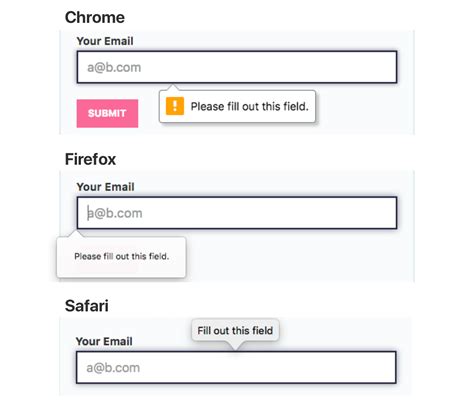
Conclusion
Validation errors are a common issue that developers face when working on projects. By understanding what validation errors are, why they occur, and how to handle them effectively, you can create a better user experience and ensure data integrity in your system.
Some key strategies for handling validation errors include:
- Providing clear and descriptive error messages
- Validating data early and often, on both the client side and server side
- Using form validation libraries to simplify the validation process
- Logging and monitoring validation errors to identify areas for improvement
- Handling errors gracefully and providing a good user experience
By following these strategies and best practices, you can minimize validation errors, create a more user-friendly system, and ultimately deliver a higher-quality product to your users.
I hope this article has provided a helpful overview of validation errors and how to handle them in your projects. Remember, validation is an important part of any software system, and by taking the time to implement it effectively, you can create a better experience for your users and ensure the accuracy and integrity of your data.
No responses yet