What is a GPS Module?
A GPS (Global Positioning System) module is an electronic device that receives signals from GPS satellites and calculates its location on Earth. It provides latitude, longitude, and sometimes altitude coordinates that pinpoint the module’s precise position. GPS modules are used in a wide variety of applications such as navigation, tracking, mapping, surveying, and timekeeping.
How GPS Works
GPS is based on a constellation of 24+ satellites orbiting the Earth at an altitude of approximately 12,500 miles. These satellites are positioned so that a GPS receiver on the ground can always receive signals from at least four of them at any given time and location on the planet.
Each GPS satellite continuously transmits a radio signal containing its current time and position. A GPS module receives these signals and measures the time it took for them to arrive. By knowing the speed of the radio waves (the speed of light) and the transmit time encoded in the signals, the module can calculate its distance from each satellite. With distance measurements from at least four satellites, the module can then use trilateration to determine its precise location on Earth.
Here is a simplified overview of how GPS positioning works:
- GPS satellites transmit signals with timing and orbital data
- GPS module receives signals from 4+ satellites
- Module measures arrival times to calculate distances
- Trilateration is used to pinpoint location from distances
- Location is output as latitude, longitude, altitude coordinates
GPS Module Interfaces
GPS modules typically provide a serial interface to communicate location data to a host system, such as a microcontroller or computer. The most common interfaces used by GPS modules are:
Interface | Description |
---|---|
UART | Universal Asynchronous Receiver/Transmitter serial interface |
I2C | Inter-Integrated Circuit synchronous serial interface |
SPI | Serial Peripheral Interface synchronous serial interface |
The de facto standard protocol used over these serial interfaces is NMEA 0183. This is a text-based protocol that outputs lines of data called sentences. Each sentence starts with a dollar sign and a two letter talker ID, followed by a three letter sentence ID that indicates the type of data, and ends with an asterisk and checksum. The data fields in between are comma delimited.
Here is an example of a GGA (Global Positioning System Fix Data) sentence from a GPS module:
$GPGGA,123519,4807.038,N,01131.000,E,1,08,0.9,545.4,M,46.9,M,,*47
The fields in this sentence contain the following data:
Field | Example | Description |
---|---|---|
Message ID | $GPGGA | GGA protocol header |
UTC Time | 123519 | Fix taken at 12:35:19 UTC |
Latitude | 4807.038,N | Latitude 48 deg 07.038′ N |
Longitude | 01131.000,E | Longitude 11 deg 31.000′ E |
Fix Quality | 1 | Data is from a GPS fix |
Number of Satellites | 08 | 8 satellites being tracked |
HDOP | 0.9 | Horizontal dilution of position |
MSL Altitude | 545.4,M | 545.4 meters above mean sea level |
Geoid Separation | 46.9,M | 46.9 meters geoid separation |
Checksum | *47 | Checksum data |
A GPS module will output a stream of NMEA sentences at a periodic interval, usually every second. The host system parses these sentences to extract the relevant location information.
In addition to NMEA output, some GPS modules offer more concise binary protocols for location data. These may be proprietary or based on an industry standard like SiRF One Socket Protocol (OSP). Binary protocols are more efficient than NMEA but less interoperable between different GPS module brands.
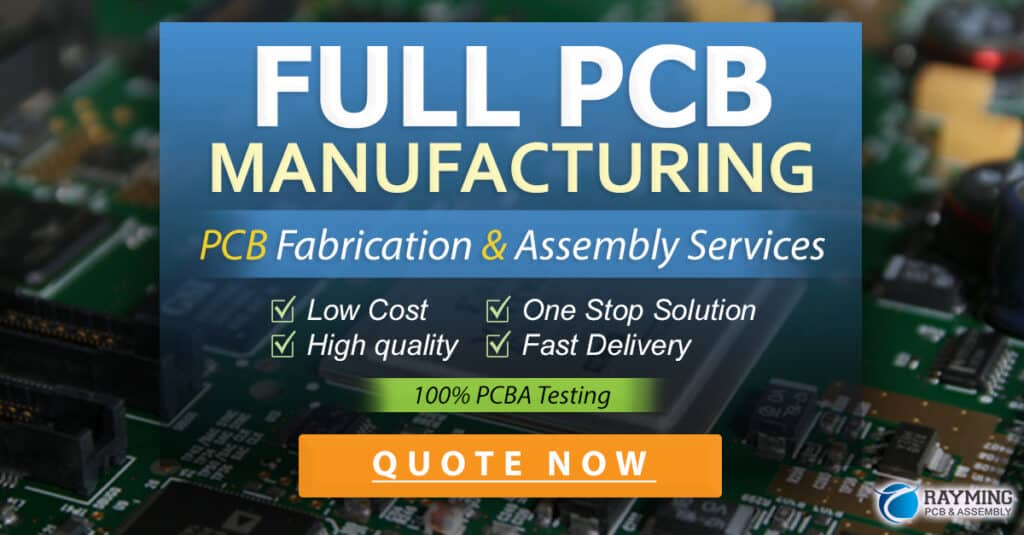
Connecting a GPS Module
The steps required to connect a GPS module to a host system will vary depending on the module and interfaces used. But in general, you will need to:
-
Determine the module’s power requirements and connect to an appropriate supply. Most operate at 3.3V or 5V.
-
Connect the module’s serial transmit pin (TX) to a receive pin (RX) on the host, and the module’s receive pin (RX) if needed to a transmit pin (TX) on the host. Use level shifters if the module and host are at different voltage levels.
-
For modules with I2C or SPI interfaces, connect the clock and data lines to the appropriate pins on the host. Pullup resistors may be needed on the data lines.
-
Connect an active GPS antenna to the module’s antenna input if it doesn’t have a built-in antenna. The antenna should be positioned with a clear view of the sky.
-
Configure the host’s serial port to match the GPS module’s settings, which are typically:
-
9600 baud
- 8 data bits
- No parity
-
1 stop bit
-
Write code on the host to parse the incoming NMEA sentences or binary messages and extract the desired location data.
Here is an example connection between a 5V GPS module with UART interface and a 3.3V microcontroller:
Note the use of resistor dividers to convert the 5V TX output from the GPS module to a safe 3.3V level for the microcontroller. The microcontroller’s 3.3V TX output can generally be connected directly to the GPS module’s RX input without level shifting.
Parsing NMEA Data
To make use of the location data from a GPS module, the host system needs to parse the NMEA sentences output by the module. The key steps in parsing NMEA data are:
-
Read in characters from the serial port until a complete NMEA sentence is received. NMEA sentences end with a newline character.
-
Check the checksum of the sentence to verify its integrity. The checksum is calculated as the XOR of all characters between the $ and * delimiters. It should match the two hex digits after the * in the sentence.
-
Split the sentence into its comma-delimited fields.
-
Check the talker ID and sentence ID to determine what kind of data the sentence contains. The most commonly used sentences for location data are:
Sentence ID | Description |
---|---|
GGA | Global Positioning System Fix Data |
RMC | Recommended Minimum Navigation Information |
GSA | GPS DOP and active satellites |
GLL | Geographic Position – Latitude/Longitude |
VTG | Course Over Ground and Ground Speed |
-
Extract the relevant data fields from the sentence based on their position. Convert fields to the appropriate data types as needed (e.g. latitude/longitude from DMM to DD format).
-
Use the extracted data as needed in the host application, e.g. to display the current position on a map, log a track, calculate navigation routes, etc.
Here is an example of parsing code in Python for a GGA sentence:
def parse_gga(sentence):
fields = sentence.split(',')
if len(fields) < 14:
return None
# Extract data fields
utc_time = fields[1]
latitude_dmm = fields[2]
latitude_dir = fields[3]
longitude_dmm = fields[4]
longitude_dir = fields[5]
fix_quality = int(fields[6])
num_satellites = int(fields[7])
hdop = float(fields[8])
altitude_msl = float(fields[9])
# Convert latitude and longitude to decimal degrees
latitude_dd = dmm_to_dd(latitude_dmm, latitude_dir)
longitude_dd = dmm_to_dd(longitude_dmm, longitude_dir)
return {
'utc_time': utc_time,
'latitude': latitude_dd,
'longitude': longitude_dd,
'fix_quality': fix_quality,
'num_satellites': num_satellites,
'hdop': hdop,
'altitude': altitude_msl
}
Many programming languages have existing libraries that handle the low-level details of reading and parsing NMEA data from a GPS module. Using one of these libraries is recommended over writing your own NMEA parser from scratch, unless you have specific needs that the library doesn’t meet.
GPS Accuracy and Errors
The accuracy of a GPS position fix depends on several factors, including:
- Number of satellites visible
- Geometric arrangement of satellites in the sky
- Signal obstructions and multipath interference
- Ionospheric and tropospheric delays
- Receiver clock errors
Under ideal conditions with a clear view of the sky, a modern GPS module can achieve a horizontal position accuracy of 2-3 meters. Vertical accuracy is usually worse, around 5 meters. However, accuracy can degrade significantly in urban canyons, under dense foliage, or indoors where satellite signals are blocked or reflected.
The geometric arrangement of satellites is measured by the Dilution of Precision (DOP). A lower DOP value indicates a better arrangement and higher potential accuracy. DOP is reported in the GSA sentence in the following components:
DOP | Description |
---|---|
GDOP | Geometric DOP |
PDOP | Position (3D) DOP |
HDOP | Horizontal DOP |
VDOP | Vertical DOP |
TDOP | Time DOP |
To improve the accuracy and reliability of GPS, various augmentation systems have been developed. These include:
- Wide Area Augmentation System (WAAS) – Covers North America
- European Geostationary Navigation Overlay Service (EGNOS) – Covers Europe and Africa
- Multi-functional Satellite Augmentation System (MSAS) – Covers Japan
- GPS-Aided GEO Augmented Navigation (GAGAN) – Covers India
These satellite-based augmentation systems (SBAS) provide correction data that GPS receivers can use to refine their position calculations. With SBAS, accuracy can be improved to less than 1 meter in open sky conditions. Many mid-range and high-end GPS modules have built-in SBAS support.
Tips for Using GPS Modules
Here are some general tips to get the best performance out of GPS modules:
-
Mount the GPS antenna where it will have a clear view of the sky. Avoid placing it near obstructions like buildings, trees, or in vehicles with metallized window tint.
-
Allow the GPS module to run for a few minutes when first powered on to download a fresh set of ephemeris data from the satellites. This will speed up the time to first fix.
-
Consider using a real-time clock and backup battery with the GPS module to preserve time and almanac data between power cycles. This allows for faster start-up times.
-
Monitor the GPS fix quality and number of satellites in use. Don’t rely on position data until a 3D fix is achieved.
-
Take into account the GPS receiver’s update rate and latency when using it for navigation or tracking. Some low-cost modules only provide updates once per second.
-
Use a Kalman filter or similar algorithm to smooth out noisy GPS data and combine it with data from other sensors like accelerometers or vehicle odometry.
-
Be aware of the limitations of GPS in indoor, underground, or urban canyon environments. Consider using other location technologies like Wi-Fi, Bluetooth beacons, or dead reckoning to supplement GPS in these situations.
Choosing a GPS Module
There are many GPS modules on the market from various manufacturers, with a range of features and capabilities. When selecting a GPS module for your application, consider the following factors:
-
Receiver type – Older modules may only support GPS, while newer ones may be multi-GNSS receivers that can also use GLONASS, Galileo, and Beidou satellite systems for improved accuracy and coverage.
-
Channels – More receiver channels allows for tracking more satellites simultaneously. 24-66 channels is typical for modern receivers.
-
Sensitivity – Higher sensitivity allows for better performance in weak signal environments. Sensitivity is measured in dBm, with -165 dBm being a typical value for modern receivers.
-
Update rate – Faster update rates (e.g. 10 Hz) are useful for high dynamic applications like drones. 1 Hz is sufficient for most navigation uses.
-
Time to first fix – How quickly the receiver can obtain a position fix after startup. Aided start modes like AGPS can reduce TTFF.
-
Accuracy – Consider both the horizontal and vertical accuracy specifications, with and without SBAS. Sub-meter accuracy is possible with high-end modules.
-
Output protocols – Check that the module supports the desired output protocol (e.g. NMEA, OSP) and interface (e.g. UART, I2C, SPI).
-
Power consumption – GPS receivers can draw significant power, especially when actively tracking. Look for low-power modes if that’s a concern.
-
Antenna type – Patch antennas are lower profile, while helical antennas provide better reception. Active antennas with LNAs are used for long cable runs.
-
Cost – Receiver modules range from under $10 for basic units to over $100 for high-performance ones.
Some popular GPS module chipsets and receivers include:
Manufacturer | Model | Receiver Chipset |
---|---|---|
Ublox | NEO-6M | Ublox 6 |
Ublox | NEO-M8N | Ublox M8030 |
Ublox | SAM-M8Q | Ublox M8030 |
Adafruit | Ultimate GPS | MTK3339 |
SparkFun | ZOE-M8Q | Ublox M8030 |
SparkFun | Qwiic Titan X1 | Titan X1 |
DFRobot | GPS NEO-6M | Ublox 6 |
DFRobot | GPS NEO-M8N | Ublox M8030 |
GPS Module Projects and Applications
Here are a few example projects and applications that make use of GPS modules:
-
Vehicle tracking – Use a GPS module with a cellular modem to track the location and status of fleet vehicles in real-time.
-
Asset tracking – Attach GPS trackers to high-value assets like equipment or shipping containers to monitor their location and movement.
-
Weather balloon tracking – Use GPS to track the position of high altitude weather balloons and recover the payloads.
-
Drone navigation – Integrate GPS into a drone’s autopilot system for waypoint navigation and automatic return-to-home.
-
Geocaching – Build a handheld GPS device for playing outdoor treasure hunting games like geocaching or orienteering.
-
GPS clock – Use the precise time signals from GPS satellites to build a reference clock or synchronize networks.
-
Satellite ground station tracking – Incorporate GPS into antenna tracking systems for satellite ground stations.
-
Hiker/runner tracker – Build a GPS tracking device for hikers or runners to record their routes and stats.
-
Pet tracking – Put a GPS collar on pets to track their location if they wander off.
-
Bike computer – Build a GPS-enabled bike computer to display location, speed, distance, and route information to the rider.
The possibilities for GPS-based projects and products are nearly endless. As GPS modules continue to get smaller, cheaper, and more capable, they’re becoming a common component in all sorts of consumer and industrial devices.
FAQ about GPS Modules
-
What’s the difference between GPS, GLONASS, Galileo, and Beidou?
These are all Global Navigation Satellite Systems (GNSS) that work similarly to GPS but are operated by different countries or regions. GLONASS is Russia’s system, Galileo is European, and Beidou is Chinese. Using a receiver that supports multiple GNSS can improve accuracy and reliability. -
Can a GPS receiver work indoors?
GPS relies on line-of-sight signals from satellites, so it generally doesn’t work well indoors where the signals are blocked by buildings. Assisted GPS (AGPS) can help improve indoor location performance by using cell network data to estimate position. But for reliable indoor positioning, other technologies like Wi-Fi, Bluetooth, or UWB are often used instead or in conjunction with GPS. -
How much power does a GPS module use?
Power consumption varies between modules but is typically in the range of 30-50 mA while actively tracking and higher during signal acquisition. Most modules have low-power standby modes that use <1 mA. The power requirements are important to consider for battery-operated applications. -
How often does a GPS module send location updates?
The update rate depends on the specific module and how it’s configured. Most modules support update rates of 1 Hz (once per second) by default, which is sufficient for many applications. Higher update rates of 5-10 Hz are possible with some modules but require more processing power and bandwidth. -
Do I need an external antenna with a GPS module?
Many GPS modules
No responses yet