Introduction to the DS1307
The DS1307 is an 8-pin RTC IC manufactured by Maxim Integrated (formerly Dallas Semiconductors). It is designed to keep track of time even when the main power supply is interrupted, thanks to its built-in battery backup. The chip maintains seconds, minutes, hours, day, date, month, and year information, with leap-year compensation valid up to 2100.
Key Features of the DS1307
- I2C interface for easy communication with microcontrollers
- Low power consumption
- Battery backup for continuous timekeeping
- 56-byte, battery-backed, non-volatile RAM for data storage
- Programmable square-wave output
- Automatic power-fail detect and switch circuitry
DS1307 Pinout
To effectively use the DS1307 in your projects, it is essential to understand its pinout. The following table provides an overview of the DS1307’s pins and their functions:
Pin Number | Pin Name | Description |
---|---|---|
1 | X1 | 32.768 kHz crystal connection |
2 | X2 | 32.768 kHz crystal connection |
3 | VBAT | Battery input (+3V) |
4 | GND | Ground |
5 | SDA | Serial Data (I2C) |
6 | SCL | Serial Clock (I2C) |
7 | SQW/OUT | Square Wave/Output Driver |
8 | VCC | Power Supply (+5V) |
X1 and X2 (Pins 1 and 2)
The X1 and X2 pins are used to connect a 32.768 kHz crystal to the DS1307. This crystal provides a stable clock source for accurate timekeeping. When selecting a crystal, ensure that it has a low ESR (Equivalent Series Resistance) and a load capacitance of 12.5 pF.
VBAT (Pin 3)
The VBAT pin is used to connect a 3V battery to the DS1307 for backup power. This battery ensures that the RTC continues to keep time even when the main power supply is disconnected. Typically, a coin cell battery (such as a CR2032) is used for this purpose.
GND (Pin 4)
The GND pin is the ground connection for the DS1307. It should be connected to the common ground of your circuit.
SDA and SCL (Pins 5 and 6)
SDA (Serial Data) and SCL (Serial Clock) are the I2C interface pins used for communication between the DS1307 and a microcontroller. The DS1307 operates as a slave device on the I2C bus. These pins require pull-up resistors (typically 4.7kΩ) to VCC.
SQW/OUT (Pin 7)
The SQW/OUT pin can be configured to output a square wave signal with a frequency of 1 Hz, 4 kHz, 8 kHz, or 32 kHz. This feature is useful for generating interrupts or clock signals for other components in your circuit. Alternatively, this pin can be used as a general-purpose output.
VCC (Pin 8)
The VCC pin is the main power supply for the DS1307. It should be connected to a stable +5V source. The DS1307 has a wide operating voltage range of 4.5V to 5.5V.
Interfacing the DS1307 with a Microcontroller
To use the DS1307 in your project, you will need to interface it with a microcontroller using the I2C protocol. Most microcontrollers, such as the Arduino or Raspberry Pi, have built-in I2C peripherals that simplify this process.
I2C Communication
The DS1307 uses I2C communication to exchange data with the microcontroller. The I2C bus consists of two lines: SDA (Serial Data) and SCL (Serial Clock). The microcontroller acts as the master device, initiating and controlling the communication, while the DS1307 acts as the slave device, responding to the master’s requests.
To begin communication, the master sends a START condition followed by the DS1307’s slave address (0x68) and a read/write bit. The DS1307 acknowledges the request, and the master can then read from or write to the RTC’s registers.
Setting and Reading the Time
To set the time on the DS1307, you need to write the appropriate values to the RTC’s registers. The DS1307 has seven time and date registers, each responsible for storing a specific component of the timestamp (seconds, minutes, hours, day, date, month, and year). These registers are located at addresses 0x00 to 0x06.
When writing to the time and date registers, you must use the Binary Coded Decimal (BCD) format. In BCD, each decimal digit is represented by a 4-bit binary value. For example, the decimal value 59 would be written as 0101 1001 in BCD.
To read the current time from the DS1307, you simply need to read the values stored in the time and date registers. The microcontroller can then convert the BCD values back to decimal format for display or further processing.
Using the Non-Volatile RAM
The DS1307 includes 56 bytes of non-volatile RAM (NVRAM) that can be used to store custom data. This memory is battery-backed, ensuring that the data is retained even when the main power supply is disconnected.
To access the NVRAM, you need to write to or read from the memory addresses starting at 0x08. The NVRAM is organized as a linear array of bytes, allowing you to store various types of data, such as calibration values, configuration settings, or user preferences.
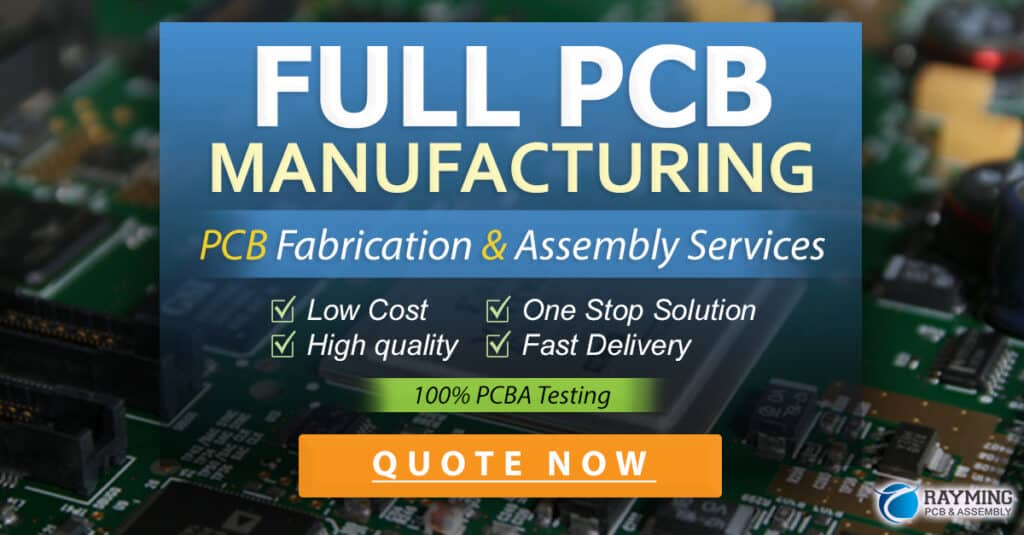
Example Code (Arduino)
Here’s an example of how to interface the DS1307 with an Arduino using the RTClib
library:
#include <Wire.h>
#include <RTClib.h>
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
Wire.begin();
rtc.begin();
// Set the initial time (only needed once)
// rtc.adjust(DateTime(2023, 4, 20, 12, 0, 0));
}
void loop() {
DateTime now = rtc.now();
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(' ');
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.print(now.second(), DEC);
Serial.println();
delay(1000);
}
In this example, we first include the necessary libraries (Wire.h
for I2C communication and RTClib.h
for the DS1307 functionality). We create an instance of the RTC_DS1307
class called rtc
.
In the setup()
function, we initialize the Serial communication, the I2C bus, and the RTC. If the DS1307 is not yet set, you can uncomment the rtc.adjust()
line and set the initial time.
In the loop()
function, we read the current time using rtc.now()
and store it in a DateTime
object called now
. We then print the date and time components to the Serial monitor. The delay(1000)
function introduces a 1-second delay between each reading.
Troubleshooting Common Issues
Crystal Oscillator Not Working
If the DS1307 fails to keep accurate time or the time is not advancing, it may indicate an issue with the crystal oscillator. Ensure that the crystal is properly connected to the X1 and X2 pins and that it meets the required specifications (32.768 kHz, 12.5 pF load capacitance). Also, check for any shorts or loose connections.
Incorrect Time Readings
If the time readings from the DS1307 are incorrect, there could be several reasons:
-
The RTC has not been set initially. Make sure to set the time using the
rtc.adjust()
function. -
The battery backup is weak or disconnected. Check the battery connection and replace the battery if necessary.
-
Interference from other components. Ensure that the DS1307 is not placed near sources of electromagnetic interference, such as motors or power supplies.
I2C Communication Issues
If you encounter problems with I2C communication between the microcontroller and the DS1307, consider the following:
-
Check the wiring connections. Make sure that the SDA and SCL pins are correctly connected and that the pull-up resistors are in place.
-
Verify the I2C address. The default I2C address for the DS1307 is 0x68. If you have changed the address, update your code accordingly.
-
Check for I2C bus conflicts. If other devices are connected to the I2C bus, ensure that they are not causing conflicts or using the same address as the DS1307.
Frequently Asked Questions (FAQ)
- Can I use the DS1307 without a battery backup?
Yes, you can use the DS1307 without a battery backup. However, in this case, the RTC will lose its time setting whenever the main power supply is disconnected. The battery backup ensures continuous timekeeping even during power outages.
- How long does the battery backup last?
The battery backup duration depends on the battery capacity and the DS1307’s power consumption. A typical CR2032 coin cell battery can provide backup power for several years. However, it is recommended to replace the battery periodically to ensure reliable operation.
- Can I use the DS1307 with a 3.3V microcontroller?
Yes, the DS1307 is compatible with 3.3V microcontrollers. However, you need to ensure that the I2C bus is properly level-shifted between the 3.3V and 5V domains. You can use a bi-directional level shifter or a simple voltage divider to achieve this.
- How accurate is the DS1307?
The accuracy of the DS1307 depends on several factors, including the quality of the crystal and the calibration. Typically, the DS1307 has an accuracy of ±2ppm at 25°C, which translates to a drift of about 1 minute per year. However, this can vary depending on temperature and other environmental factors.
- Can I use multiple DS1307 modules on the same I2C bus?
No, you cannot use multiple DS1307 modules on the same I2C bus without modifying their addresses. By default, all DS1307 modules have the same I2C address (0x68). To use multiple modules, you would need to modify the address of each module by connecting the AD0 pin (which is not accessible on the DIP package) to a different logic level.
Conclusion
The DS1307 is a versatile and reliable real-time clock IC that offers an easy-to-use solution for timekeeping in a wide range of applications. By understanding the DS1307 pinout and its features, you can effectively integrate this chip into your projects and ensure accurate timekeeping.
When working with the DS1307, remember to consider factors such as crystal selection, battery backup, and I2C communication. By following best practices and being aware of common issues, you can troubleshoot and resolve problems quickly.
As you explore the capabilities of the DS1307, don’t hesitate to experiment with its features, such as the square wave output and the non-volatile RAM. These additional functions can add value to your projects and open up new possibilities for customization and functionality.
With its low power consumption, wide operating voltage range, and battery backup, the DS1307 is an excellent choice for projects that require reliable and accurate timekeeping. Whether you are building a data logger, a home automation system, or a wearable device, the DS1307 can provide the time and date information you need.
No responses yet