Understanding RFID Technology
Before diving into the DIY project, it’s essential to have a basic understanding of RFID technology. RFID systems consist of two main components: a reader and a tag. The reader emits radio waves that power and communicate with the tag, which contains a unique identifier. When the tag is within range of the reader, it sends its identifier back to the reader, allowing the system to identify and track the tagged object.
Types of RFID Systems
There are three main types of RFID systems, each with its own characteristics and applications:
-
Low-Frequency (LF) RFID: Operating at 125-134 kHz, LF RFID has a short read range (up to 10 cm) and is commonly used for animal tracking and access control.
-
High-Frequency (HF) RFID: Operating at 13.56 MHz, HF RFID has a medium read range (up to 1 m) and is often used for smart cards, library books, and contactless payments.
-
Ultra-High-Frequency (UHF) RFID: Operating at 860-960 MHz, UHF RFID has a long read range (up to 12 m) and is used for inventory management, asset tracking, and supply chain optimization.
For this DIY project, we’ll focus on building an HF RFID reader, as it offers a good balance between read range and compatibility with widely available tags.
Gathering the Components
To build your DIY RFID Reader, you’ll need the following components:
- Arduino Uno or compatible microcontroller board
- MFRC522 RFID reader module
- Breadboard
- Jumper wires
- USB cable
- Computer with Arduino IDE installed
MFRC522 RFID Reader Module
The MFRC522 is a popular HF RFID reader module that is compatible with the Arduino platform. It supports ISO/IEC 14443A/MIFARE protocols and can read and write to various types of RFID tags. The module communicates with the Arduino using the SPI (Serial Peripheral Interface) protocol.
Pin | Function |
---|---|
VCC | Power supply (3.3V) |
RST | Reset pin |
GND | Ground |
MISO | Master In Slave Out (SPI) |
MOSI | Master Out Slave In (SPI) |
SCK | Serial Clock (SPI) |
SDA | Slave Select (SPI) |
Wiring the Components
Now that you have all the necessary components, it’s time to wire them together. Follow these steps:
- Connect the VCC pin of the MFRC522 module to the 3.3V pin on the Arduino.
- Connect the RST pin of the MFRC522 module to digital pin 9 on the Arduino.
- Connect the GND pin of the MFRC522 module to a GND pin on the Arduino.
- Connect the MISO pin of the MFRC522 module to digital pin 12 on the Arduino.
- Connect the MOSI pin of the MFRC522 module to digital pin 11 on the Arduino.
- Connect the SCK pin of the MFRC522 module to digital pin 13 on the Arduino.
- Connect the SDA pin of the MFRC522 module to digital pin 10 on the Arduino.
Here’s a table summarizing the connections:
MFRC522 Pin | Arduino Pin |
---|---|
VCC | 3.3V |
RST | 9 |
GND | GND |
MISO | 12 |
MOSI | 11 |
SCK | 13 |
SDA | 10 |
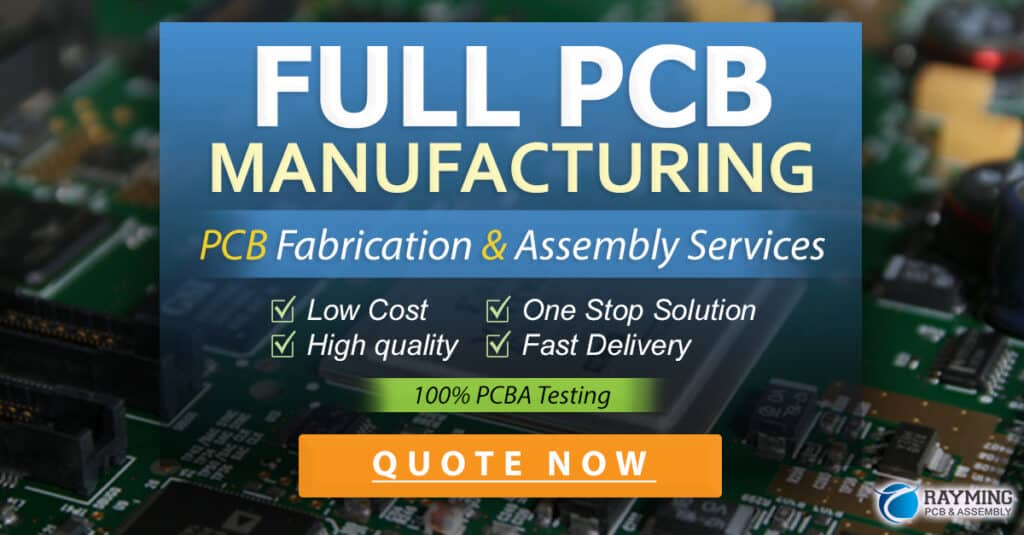
Setting Up the Arduino IDE
To program your Arduino and communicate with the MFRC522 module, you’ll need to set up the Arduino IDE on your computer. Follow these steps:
- Download and install the Arduino IDE from the official website: https://www.arduino.cc/en/software
- Open the Arduino IDE and navigate to Sketch > Include Library > Manage Libraries.
- In the Library Manager, search for “MFRC522” and install the “MFRC522 by GithubCommunity” library.
Programming the RFID Reader
With the hardware setup complete and the Arduino IDE ready, it’s time to write the code for your DIY RFID reader. Here’s a sample sketch that demonstrates how to read RFID tags and display their unique identifiers:
#include <SPI.h>
#include <MFRC522.h>
#define RST_PIN 9
#define SS_PIN 10
MFRC522 mfrc522(SS_PIN, RST_PIN);
void setup() {
Serial.begin(9600);
SPI.begin();
mfrc522.PCD_Init();
Serial.println("RFID reader is ready");
}
void loop() {
if (mfrc522.PICC_IsNewCardPresent() && mfrc522.PICC_ReadCardSerial()) {
Serial.print("Card UID: ");
for (byte i = 0; i < mfrc522.uid.size; i++) {
Serial.print(mfrc522.uid.uidByte[i] < 0x10 ? "0" : "");
Serial.print(mfrc522.uid.uidByte[i], HEX);
}
Serial.println();
mfrc522.PICC_HaltA();
}
}
This sketch does the following:
- Includes the necessary libraries (SPI and MFRC522).
- Defines the reset and slave select pins for the MFRC522 module.
- Initializes the MFRC522 object with the defined pins.
- Sets up the serial communication and initializes the SPI and MFRC522 module in the
setup()
function. - Continuously checks for the presence of a new RFID tag in the
loop()
function. - If a new tag is detected, reads its unique identifier (UID) and prints it to the serial monitor.
Upload this sketch to your Arduino and open the serial monitor. When you bring an RFID tag close to the MFRC522 module, you should see its UID printed in the serial monitor.
Testing and Troubleshooting
After uploading the sketch, it’s time to test your DIY RFID reader. Here are some tips for testing and troubleshooting:
- Ensure that the wiring is correct and secure.
- Verify that the MFRC522 module is properly powered (the LED should be on).
- Make sure that the RFID tag you’re using is compatible with the MFRC522 module (ISO/IEC 14443A/MIFARE).
- Check the serial monitor for any error messages or unexpected output.
- If the reader is not detecting tags, try adjusting the position or orientation of the tag relative to the reader.
Common Issues and Solutions
Issue | Solution |
---|---|
MFRC522 module not detected | Check the wiring and ensure that the slave select pin is correctly defined in the sketch. |
RFID tag not detected | Verify that the tag is compatible with the MFRC522 module and within the read range. |
Inconsistent readings | Ensure that there are no interfering objects or electromagnetic sources nearby. |
Arduino not recognized by computer | Make sure that the USB cable is securely connected and the correct board and port are selected in the Arduino IDE. |
Expanding Your DIY RFID Reader
Now that you have a basic DIY RFID reader up and running, you can explore various ways to expand its functionality and integrate it into your projects. Here are a few ideas:
- Add an LCD or OLED display to show the UID of the detected tags.
- Connect the RFID reader to a Wi-Fi module (e.g., ESP8266) to send tag data to a web server or database.
- Integrate the RFID reader with an access control system, such as an electric door lock or turnstile.
- Use the RFID reader to track inventory or assets by associating each tag with a specific item in a database.
- Combine the RFID reader with other sensors or actuators to create interactive projects or installations.
Frequently Asked Questions (FAQ)
-
Can I use a different Arduino board for this project?
Yes, you can use any Arduino board that is compatible with the MFRC522 library and has sufficient digital pins for the required connections. -
What is the maximum read range of the MFRC522 module?
The MFRC522 module has a typical read range of up to 5 cm, depending on the size and type of the RFID tag being used. -
Can I use this DIY RFID reader to read NFC tags?
The MFRC522 module is compatible with some NFC tags that use the ISO/IEC 14443A protocol, such as the Mifare Ultralight. However, it may not be compatible with all NFC tags or devices. -
How can I power my DIY RFID reader independently of a computer?
You can power your RFID reader using a battery pack or an external power supply that provides 5V. Make sure to connect the power supply to the VIN and GND pins on the Arduino board. -
Can I use multiple MFRC522 modules with a single Arduino?
Yes, you can connect multiple MFRC522 modules to a single Arduino by assigning each module a unique slave select (SS) pin and modifying the sketch accordingly.
Conclusion
Building your own DIY RFID reader is a rewarding and educational experience that can open up a world of possibilities for your projects. By following this guide and experimenting with different configurations and applications, you can create a versatile and effective RFID reader that suits your needs. Remember to always test and troubleshoot your setup and consider the security and privacy implications of using RFID technology in your projects.
No responses yet