Introduction to the DHT11 Sensor
The DHT11 is a low-cost digital temperature and humidity sensor that provides reliable readings for various applications. This sensor consists of a capacitive humidity sensing element and a thermistor for temperature measurement. It offers excellent long-term stability and high reliability, making it a popular choice for environmental monitoring, HVAC systems, and IoT projects.
Key Features of the DHT11 Sensor
- Relative Humidity Range: 20-90% RH
- Temperature Range: 0-50°C
- Humidity Accuracy: ±5% RH
- Temperature Accuracy: ±2°C
- Operating Voltage: 3.3-5.5V DC
- Low Power Consumption: 2.5mA max current use during conversion
- Small Size: 15.5mm x 12mm x 5.5mm
- 4-pin Single Row Pin Package
DHT11 Pinout and Connections
The DHT11 sensor has a 4-pin single row pin package. The pinout is as follows:
Pin | Function |
---|---|
1 | VCC (Power Supply: 3.3-5.5V DC) |
2 | Data Output (Digital Signal) |
3 | Not Connected |
4 | Ground |
To connect the DHT11 sensor to a microcontroller, follow these steps:
- Connect VCC to the power supply (3.3-5.5V DC)
- Connect the Data Output pin to a digital input pin on the microcontroller
- Connect the Ground pin to the common ground of the system
- Use a 4.7kΩ-10kΩ pull-up resistor between the Data Output pin and VCC
DHT11 Communication Protocol
The DHT11 uses a proprietary 1-wire communication protocol to transmit data. The communication process involves the following steps:
- The microcontroller sends a start signal by pulling the data line low for at least 18ms.
- The microcontroller pulls the data line high and waits for 20-40μs for the DHT11 to respond.
- The DHT11 responds by pulling the data line low for 80μs, then high for 80μs.
- The DHT11 sends 40 bits of data, consisting of 16 bits for relative humidity, 16 bits for temperature, and an 8-bit checksum.
- Each bit transmission begins with a 50μs low signal, followed by a high signal. The duration of the high signal determines the bit value: 26-28μs for a “0” or 70μs for a “1”.
Data Format
The 40 bits of data transmitted by the DHT11 are organized as follows:
Data | Bits | Description |
---|---|---|
Humidity Integer | 8 | Integer part of relative humidity |
Humidity Decimal | 8 | Decimal part of relative humidity (always 0 for DHT11) |
Temperature Integer | 8 | Integer part of temperature |
Temperature Decimal | 8 | Decimal part of temperature (always 0 for DHT11) |
Checksum | 8 | Sum of the first 32 bits |
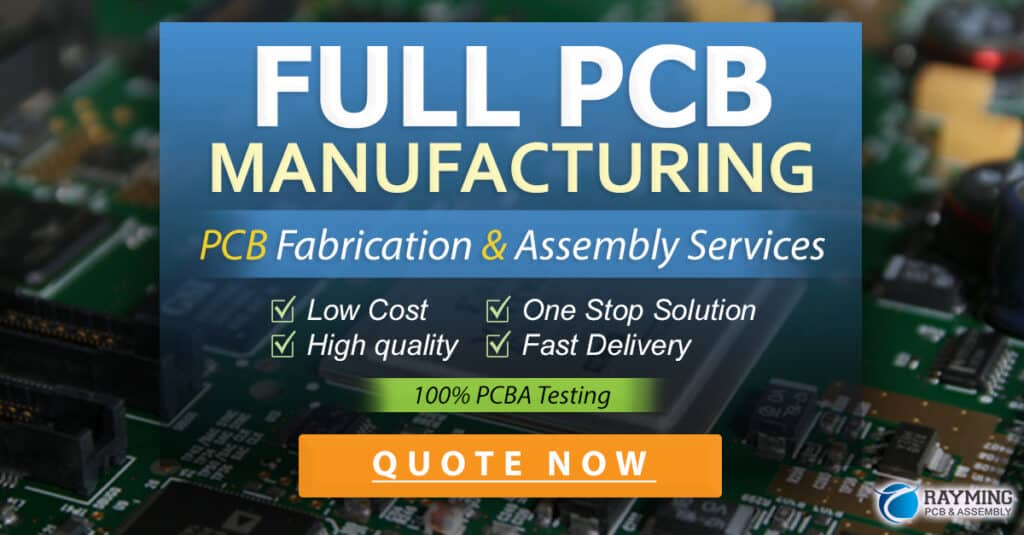
Measuring Relative Humidity and Temperature
To measure relative humidity and temperature using the DHT11 sensor, follow these steps:
- Send a start signal to the DHT11 by pulling the data line low for at least 18ms.
- Wait for the DHT11 to respond with an 80μs low signal followed by an 80μs high signal.
- Read the 40 bits of data transmitted by the DHT11, following the timing diagram for each bit.
- Verify the checksum by adding the first 32 bits and comparing the result with the received checksum.
- Extract the humidity and temperature values from the received data.
Example code for reading DHT11 data using Arduino:
#include <DHT.h>
#define DHTPIN 2
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
delay(2000);
float humidity = dht.readHumidity();
float temperature = dht.readTemperature();
if (isnan(humidity) || isnan(temperature)) {
Serial.println("Failed to read from DHT sensor!");
return;
}
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.print("% Temperature: ");
Serial.print(temperature);
Serial.println("°C");
}
DHT11 Timing Diagram
The following timing diagram illustrates the communication protocol between the microcontroller and the DHT11 sensor:
+--------------+ +------------------+
| | | |
| Start | Data | Idle |
| Signal | (40 bits) | |
| | | |
+-------+ | +-------+ | | |
| | | | | | | |
| | | | | | | |
| | | | | | | |
| | | | | | | |
| +-----+ +----+ +--+--------------------------------+ +-->
| | | | | |
| LOW | LOW | HIGH| HIGH | HIGH |
| | | | | |
| | | +--------------------------------+ |
| | | | | |
| | | | BIT "0" | BIT "1" |
| | | | | |
+--------------+ +-----+ +------------------+
18ms min 80μs 50μs 26-28μs 70μs
DHT11 Sensor Characteristics
Relative Humidity
Parameter | Condition | Min | Typical | Max | Unit |
---|---|---|---|---|---|
Resolution | 1 | % | |||
Range | 20 | 90 | % | ||
Accuracy | 25°C | ±5 | % | ||
Repeatability | 25°C | ±1 | % | ||
Hysteresis | ±1 | % | |||
Long-term Stability | ±1 | %/year | |||
Response Time | 1/e (63%) | 6 | 10 | 15 | s |
Temperature
Parameter | Condition | Min | Typical | Max | Unit |
---|---|---|---|---|---|
Resolution | 1 | °C | |||
Range | 0 | 50 | °C | ||
Accuracy | 25°C | ±2 | °C | ||
Repeatability | ±1 | °C | |||
Long-term Stability | ±1 | °C/year | |||
Response Time | 1/e (63%) | 6 | 10 | 30 | s |
FAQ
-
Q: What is the power supply voltage for the DHT11 sensor?
A: The DHT11 sensor requires a power supply voltage between 3.3V and 5.5V DC. -
Q: How often can I read data from the DHT11 sensor?
A: It is recommended to read data from the DHT11 sensor every 1-2 seconds to ensure accurate measurements and avoid self-heating of the sensor. -
Q: What is the maximum distance between the DHT11 sensor and the microcontroller?
A: The maximum distance depends on factors such as the cable quality and environmental noise. Generally, a cable length of up to 20 meters is acceptable, but it is recommended to keep the distance as short as possible for better signal integrity. -
Q: Can I use multiple DHT11 sensors with a single microcontroller?
A: Yes, you can connect multiple DHT11 sensors to a single microcontroller by using different digital input pins for each sensor’s data line. Ensure that each sensor has its own pull-up resistor. -
Q: How do I calibrate the DHT11 sensor?
A: The DHT11 sensor is factory calibrated and does not require user calibration. However, if you notice significant deviations in the readings, you can compare the sensor’s measurements with a reference device and apply an offset in your code to compensate for any discrepancies.
Conclusion
The DHT11 digital temperature and humidity sensor is a cost-effective solution for measuring environmental conditions in various applications. By understanding the sensor’s pinout, communication protocol, and characteristics, you can easily integrate it into your projects and obtain reliable relative humidity and temperature readings. Always ensure proper connections, timings, and data validation for optimal performance.
No responses yet