Introduction to DC Motors and Soft Start
DC motors are widely used in various applications, from industrial machinery to consumer electronics. They offer excellent torque, speed control, and efficiency. However, starting a DC motor abruptly can lead to several issues, such as high inrush current, mechanical stress, and reduced motor life. This is where DC Motor Soft Start comes into play.
What is a DC Motor Soft Start?
A DC motor soft start is a technique that gradually increases the voltage or current supplied to the motor during startup. This controlled acceleration reduces the initial inrush current and mechanical stress on the motor and the connected load. Soft starting ensures a smooth and gentle start, prolonging the motor’s life and improving system reliability.
Benefits of Implementing a DC Motor Soft Start
- Reduced inrush current
- Lower mechanical stress on the motor and connected load
- Improved motor life and reliability
- Smooth acceleration and deceleration
- Reduced voltage drop on the power supply
Understanding DC Motor Characteristics
To effectively implement a soft start for a DC motor, it’s essential to understand the motor’s characteristics and behavior during startup.
DC Motor Equivalent Circuit
A DC motor can be represented by an equivalent circuit consisting of an inductance (L), resistance (R), and back electromotive force (back EMF). The following table shows the typical components of a DC motor equivalent circuit:
Component | Symbol | Description |
---|---|---|
Inductance | L | Represents the motor’s windings |
Resistance | R | Represents the resistance of the windings |
Back EMF | Vb | Voltage generated by the motor’s rotation |
DC Motor Startup Behavior
During startup, a DC motor exhibits the following characteristics:
-
High inrush current: When a voltage is applied to a stationary DC motor, it draws a large initial current due to the absence of back EMF. This inrush current can be several times higher than the motor’s rated current.
-
Mechanical stress: The high inrush current generates a strong starting torque, which can cause mechanical stress on the motor and the connected load.
-
Voltage drop: The high inrush current can cause a significant voltage drop on the power supply, potentially affecting other components in the system.
Soft Start Techniques for DC Motors
There are several techniques to implement a soft start for DC motors. Let’s explore some of the most common methods.
1. Voltage Ramping
Voltage ramping is a simple and effective soft start technique. It involves gradually increasing the voltage applied to the motor over a specified time period. This can be achieved using a microcontroller or a dedicated soft start circuit.
Voltage Ramping Implementation
- Select a suitable voltage ramp duration based on the motor’s specifications and the application requirements.
- Use a microcontroller or a soft start circuit to generate a PWM signal with increasing duty cycle.
- Apply the PWM signal to the motor driver or power transistor to control the voltage supplied to the motor.
- Monitor the motor’s speed and current to ensure a smooth and controlled acceleration.
2. Current Limiting
Current limiting is another popular soft start technique that focuses on controlling the inrush current during startup. By limiting the current, you can reduce mechanical stress and protect the motor and power supply.
Current Limiting Implementation
- Choose an appropriate current limit based on the motor’s rated current and the application requirements.
- Implement a current sensing circuit using a shunt resistor or a current sensor.
- Use a microcontroller or a current limiting circuit to monitor and control the current supplied to the motor.
- Gradually increase the current limit over a specified time period to achieve a smooth acceleration.
3. PWM-based Soft Start
PWM (Pulse Width Modulation) is a versatile technique that can be used for DC motor soft start. By gradually increasing the PWM duty cycle, you can control the average voltage supplied to the motor, resulting in a smooth acceleration.
PWM-based Soft Start Implementation
- Select a suitable PWM frequency based on the motor’s characteristics and the application requirements.
- Use a microcontroller or a PWM generator to generate a PWM signal with increasing duty cycle.
- Apply the PWM signal to the motor driver or power transistor to control the voltage supplied to the motor.
- Monitor the motor’s speed and adjust the PWM duty cycle accordingly to achieve the desired acceleration profile.
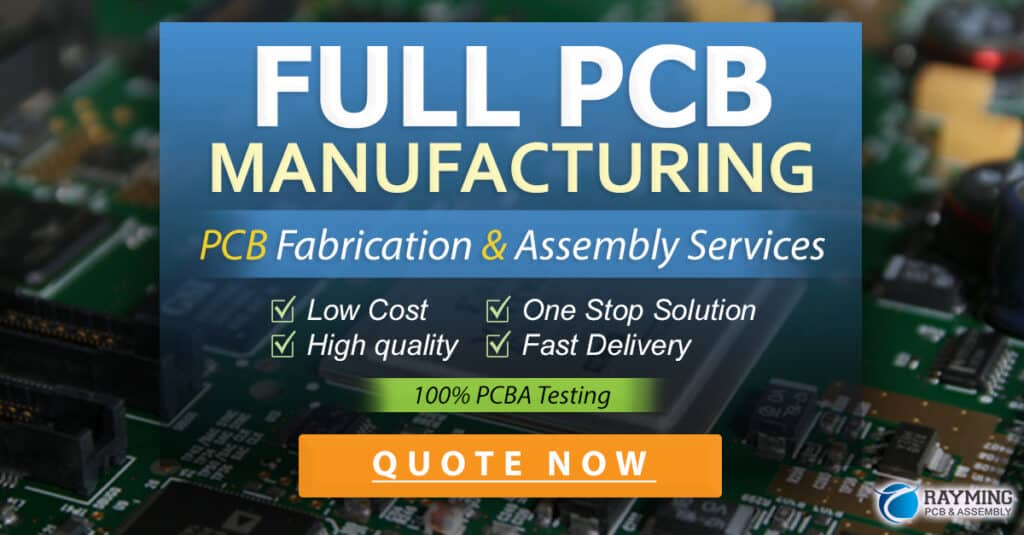
Designing a DC Motor Soft Start Circuit
Now that we’ve explored different soft start techniques, let’s design a simple DC motor soft start circuit using voltage ramping.
Circuit Components
Component | Description |
---|---|
Microcontroller | Arduino Uno or similar |
MOSFET | IRF540N or similar |
Diode | 1N4007 or similar |
Resistors | 10kΩ, 1kΩ |
Capacitor | 100nF |
Circuit Schematic
+12V
|
|
| |
| | |
| | | |
| | | | |
| | | | | |
| | | | | | |
| | | | | | | |
| | | | | | | | |
| | | | | | | | | |
| | | | | | | | | | |
| | | | | | | | | | |
| | | | | | | | | | |
| | | | | | | | | | |
| | | | | | | | | | |
| | | | | | | | | | |
| | | | | | | | | | |
| | | | | | | | | | |
+---------------------+
| |
| Arduino Uno |
| |
+---------------------+
|
|
|
+------+
| |
| | |
| | | |
| | | | |
| | | | | |
| | | | | | |
|| | | | | ||
|| | | | | ||
| | | | | | | |
| | | | | | | | |
| | | | | | | | | |
| | | | | | | | | | |
| | | | | | | | | | | |
| | | | | | | | | | | | |
| | | | | | | | | | | | | |
| | | | | | | | | | | | | | |
| | | | | | | | | | | | | | | |
| | | | | | | | | | | | | | | |
| | | | | | | | | | | | | | | |
+------------------------------+
| |
| IRF540N |
| |
+------------------------------+
| |
| |
| |
| | | |
| | | | | |
| | | | | | | |
| | | | | | | | | |
| | | | | | | | | | | |
| | | | | | | | | | | | |
+-------------+ | | | | |
| 1N4007 | | | | | |
+-------------+ | | | | |
| | | | |
| | | | |
| | | | |
+-------+
| M |
+-------+
|
|
GND
Code Implementation
const int MOSFET_PIN = 9;
const int RAMP_DURATION = 2000; // Ramp duration in milliseconds
void setup() {
pinMode(MOSFET_PIN, OUTPUT);
}
void loop() {
for (int i = 0; i <= 255; i++) {
analogWrite(MOSFET_PIN, i);
delay(RAMP_DURATION / 255);
}
delay(5000); // Run the motor for 5 seconds
for (int i = 255; i >= 0; i--) {
analogWrite(MOSFET_PIN, i);
delay(RAMP_DURATION / 255);
}
delay(2000); // Pause for 2 seconds before restarting
}
This code gradually increases the PWM duty cycle from 0 to 100% over the specified ramp duration, providing a smooth voltage ramp to the motor. After running the motor for 5 seconds, it gradually decreases the PWM duty cycle to achieve a soft stop. The cycle repeats after a 2-second pause.
Testing and Optimization
After implementing the soft start circuit, it’s crucial to test and optimize its performance. Follow these steps to ensure proper functionality:
- Connect the DC motor to the soft start circuit and power it up.
- Observe the motor’s behavior during startup. It should accelerate smoothly without any sudden jerks or high inrush current.
- Monitor the motor’s speed and current using appropriate measurement tools.
- Adjust the ramp duration and current limit (if applicable) based on the motor’s response and the application requirements.
- Test the soft start circuit under different load conditions to ensure robust performance.
- Optimize the circuit components and code implementation for improved efficiency and reliability.
FAQs
-
Q: Can I use the same soft start circuit for different DC motors?
A: While the general principles remain the same, you may need to adjust the circuit components and parameters based on the specific motor’s ratings and characteristics. Always refer to the motor’s datasheet and application requirements. -
Q: How do I select the appropriate ramp duration for my DC motor?
A: The ramp duration depends on the motor’s size, load, and application requirements. As a general guideline, start with a shorter ramp duration and gradually increase it until you achieve the desired smooth acceleration without compromising the system’s performance. -
Q: Can I use a soft start circuit with a brushless DC motor (BLDC)?
A: Yes, soft start techniques can be applied to BLDC motors as well. However, the implementation may differ due to the specific control requirements of BLDC motors. You may need to use specialized BLDC motor drivers with built-in soft start capabilities or implement the soft start algorithm in the motor controller firmware. -
Q: Are there any off-the-shelf soft start modules available for DC motors?
A: Yes, there are various off-the-shelf soft start modules and motor drivers available that incorporate soft start functionality. These modules simplify the implementation process and provide additional features such as overcurrent protection and speed control. -
Q: Can I implement a soft start for a DC motor using a purely hardware-based solution?
A: Yes, it is possible to design a hardware-based soft start circuit using components like capacitors, resistors, and transistors. However, hardware-based solutions may offer limited flexibility compared to microcontroller-based implementations, which allow for easy adjustments and customization.
Conclusion
Implementing a DC motor soft start is essential for reducing inrush current, mechanical stress, and improving overall system reliability. By understanding the motor’s characteristics and applying techniques like voltage ramping, current limiting, or PWM-based soft start, you can achieve a smooth and controlled acceleration.
When designing a soft start circuit, consider factors such as the motor’s ratings, application requirements, and available components. Test and optimize the circuit to ensure optimal performance under various load conditions.
By following the guidelines and examples provided in this article, you can create your own DC motor soft start solution and enjoy the benefits of improved motor life and system efficiency.
No responses yet