Understanding Text Truncation and Its Impact on Copying Multiline Text
Text truncation is a common issue that occurs when attempting to copy and paste multiline text into a string variable. When this happens, only the first line of the text gets pasted, while the rest of the lines are truncated or cut off. This can be a frustrating problem for developers and users alike, as it limits the ability to work with and manipulate multiline text effectively.
What Causes Text Truncation?
Text truncation can occur due to various reasons, such as:
- String variable limitations
- Incorrect handling of newline characters
- Insufficient buffer size
- Improper text parsing techniques
Understanding the root cause of text truncation is crucial in finding an appropriate solution to ensure that multiline text can be copied and pasted in its entirety.
Techniques to Prevent Text Truncation and Copy Multiline Text Successfully
1. Using a Text Area or Multiline Text Input
One of the simplest and most effective ways to handle multiline text is by using a text area or multiline text input field. These input elements are specifically designed to accept and display multiple lines of text, making them ideal for scenarios where preserving line breaks and formatting is important.
Here’s an example of how to create a text area in HTML:
<textarea rows="5" cols="50">
This is a multiline text area.
It allows you to enter and display multiple lines of text.
</textarea>
By using a text area, users can easily copy and paste multiline text without any truncation issues.
2. Handling Newline Characters Properly
When working with multiline text programmatically, it’s essential to handle newline characters correctly. Different operating systems and environments may use different newline conventions, such as \n
(Unix), \r\n
(Windows), or \r
(Mac).
To ensure compatibility and avoid truncation, you can normalize the newline characters in your code. Here’s an example in Python:
text = "Line 1\nLine 2\r\nLine 3\rLine 4"
normalized_text = text.replace("\r\n", "\n").replace("\r", "\n")
print(normalized_text)
Output:
Line 1
Line 2
Line 3
Line 4
By normalizing the newline characters, you can consistently handle multiline text across different platforms.
3. Increasing Buffer Size
In some cases, text truncation may occur due to insufficient buffer size when reading or copying text. If you’re working with large amounts of multiline text, ensure that your buffer size is adequately set to accommodate the entire content.
Here’s an example in C:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFFER_SIZE 1024
int main() {
char* buffer = (char*)malloc(BUFFER_SIZE * sizeof(char));
if (buffer == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
FILE* file = fopen("example.txt", "r");
if (file == NULL) {
printf("Failed to open the file.\n");
free(buffer);
return 1;
}
fread(buffer, sizeof(char), BUFFER_SIZE, file);
printf("Content:\n%s\n", buffer);
fclose(file);
free(buffer);
return 0;
}
In this example, the buffer size is set to BUFFER_SIZE
(1024 bytes) to ensure that it can hold a substantial amount of text. Adjust the buffer size according to your specific requirements to prevent truncation.
4. Using Appropriate Text Parsing Techniques
When working with multiline text, it’s crucial to use appropriate text parsing techniques to extract and manipulate the desired information accurately. This includes handling line breaks, whitespace, and other formatting characters correctly.
Consider the following example in Python:
text = """
Name: John Doe
Age: 25
City: New York
"""
lines = text.strip().split("\n")
for line in lines:
key, value = line.split(":")
print(f"{key.strip()}: {value.strip()}")
Output:
Name: John Doe
Age: 25
City: New York
By splitting the multiline text into individual lines and then parsing each line using the appropriate delimiter (in this case, the colon character), you can extract and process the desired information without any truncation issues.
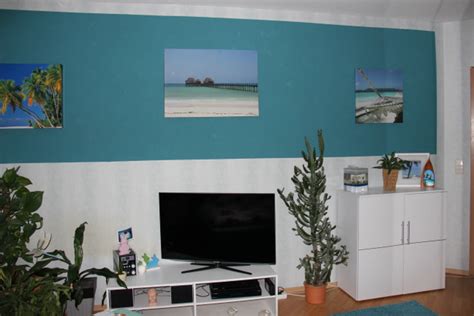
Common Scenarios and Solutions for Copying Multiline Text
Scenario 1: Copying Multiline Text from a Web Page
When copying multiline text from a web page, you may encounter truncation issues if the text is not properly formatted or if the copying mechanism is limited. To overcome this, you can:
- Use the browser’s built-in “Inspect Element” or “Developer Tools” to locate the specific element containing the multiline text.
- Copy the text directly from the HTML source code, ensuring that all line breaks and formatting are preserved.
- If the text is dynamically generated or loaded through JavaScript, you may need to use browser automation tools like Selenium or Puppeteer to programmatically extract the complete multiline text.
Scenario 2: Copying Multiline Text from a File
When copying multiline text from a file, ensure that you use the appropriate file reading techniques to handle line breaks correctly. Here’s an example in Python:
with open("example.txt", "r") as file:
content = file.read()
print(content)
By using the read()
method, you can read the entire contents of the file, including multiline text, without any truncation.
Scenario 3: Copying Multiline Text from a Console or Terminal
When copying multiline text from a console or terminal, be aware of the limitations and formatting conventions of the specific environment. Some consoles may have line wrapping or truncation behavior that can affect the copied text.
To mitigate this, you can:
- Use a terminal emulator that supports proper multiline text handling and copying.
- Redirect the console output to a file and then copy the contents of the file.
- Use command-line tools like
cat
orless
to display the multiline text and then copy it from the terminal.
Here’s an example of redirecting console output to a file in Bash:
command > output.txt
The output of the command will be saved to the output.txt
file, which you can then open and copy the multiline text from.
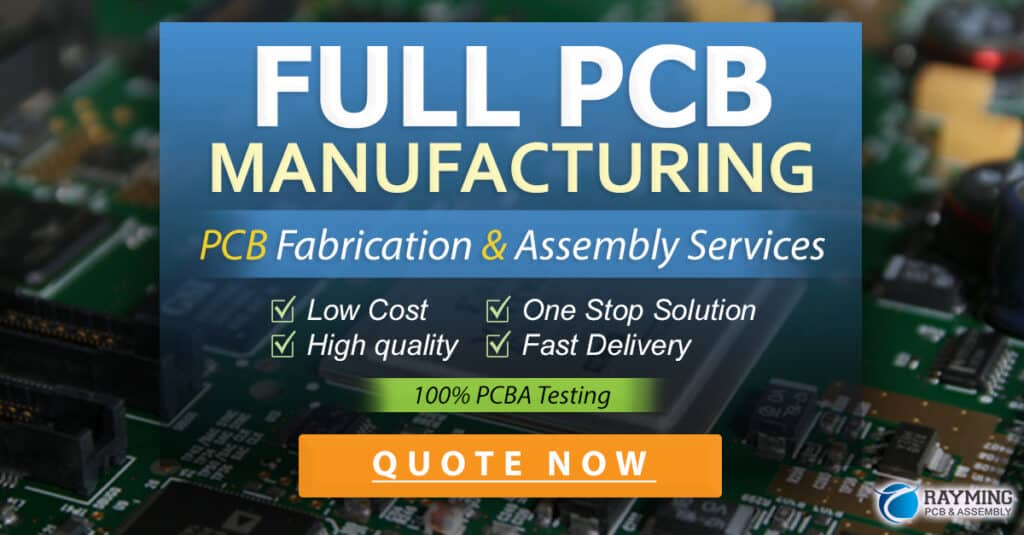
Best Practices for Working with Multiline Text
To ensure smooth handling of multiline text and avoid truncation issues, follow these best practices:
-
Use appropriate input fields: When designing user interfaces, use text areas or multiline text input fields for accepting and displaying multiline text.
-
Handle newline characters consistently: Normalize newline characters in your code to ensure compatibility across different platforms and environments.
-
Allocate sufficient buffer size: When working with large amounts of multiline text, ensure that your buffer size is adequate to hold the entire content without truncation.
-
Use proper text parsing techniques: Employ appropriate text parsing techniques, such as splitting lines and handling delimiters, to extract and manipulate multiline text accurately.
-
Test with diverse input: Thoroughly test your code with various multiline text inputs, including edge cases and different line break conventions, to ensure robustness.
-
Document limitations and requirements: Clearly document any limitations or specific requirements related to multiline text handling in your codebase or user documentation.
By following these best practices, you can minimize the occurrence of text truncation issues and ensure that multiline text is handled correctly in your applications.
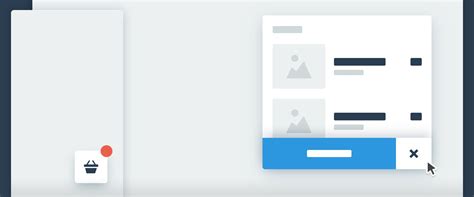
Frequently Asked Questions (FAQ)
-
Q: Why does only the first line get pasted when I copy multiline text to a string variable?
A: This issue occurs due to text truncation, which can be caused by limitations in string variables, incorrect handling of newline characters, insufficient buffer size, or improper text parsing techniques. -
Q: How can I prevent text truncation when copying multiline text?
A: To prevent text truncation, you can use text areas or multiline text input fields, handle newline characters properly, increase buffer size if necessary, and use appropriate text parsing techniques. -
Q: What should I do if I encounter truncation issues when copying multiline text from a web page?
A: When copying multiline text from a web page, you can use the browser’s developer tools to locate the specific element containing the text and copy it directly from the HTML source code. If the text is dynamically generated, you may need to use browser automation tools. -
Q: How can I ensure that multiline text is copied correctly from a file?
A: To copy multiline text from a file correctly, use appropriate file reading techniques that handle line breaks properly, such as theread()
method in Python. -
Q: Are there any best practices to follow when working with multiline text?
A: Yes, some best practices include using appropriate input fields, handling newline characters consistently, allocating sufficient buffer size, using proper text parsing techniques, testing with diverse input, and documenting any limitations or requirements related to multiline text handling.
Conclusion
Copying multiline text to a string variable and encountering truncation issues can be a frustrating experience. By understanding the causes of text truncation and employing the techniques and best practices discussed in this article, you can effectively handle multiline text and ensure that it is copied and pasted in its entirety.
Remember to use appropriate input fields, handle newline characters consistently, allocate sufficient buffer size, and employ proper text parsing techniques to minimize truncation issues. Additionally, thorough testing and clear documentation can go a long way in ensuring smooth multiline text handling in your applications.
By addressing text truncation and implementing robust solutions, you can enhance the user experience and streamline the process of working with multiline text in various scenarios.
Technique | Description |
---|---|
Using a Text Area | Utilize text areas or multiline text input fields |
Handling Newline Characters | Normalize newline characters for cross-platform compatibility |
Increasing Buffer Size | Allocate sufficient buffer size to accommodate multiline text |
Appropriate Text Parsing Techniques | Use proper techniques to extract and manipulate multiline text |
By applying these techniques and following best practices, you can overcome the challenges associated with copying multiline text to string variables and ensure reliable handling of multiline content in your applications.
No responses yet