Introduction to CANBed
CANBed is a comprehensive Arduino-based development kit designed to simplify the process of working with Controller Area Network (CAN) bus systems. CAN is a robust vehicle bus standard that enables reliable communication between microcontrollers and devices without a host computer. Originally developed for the automotive industry, CAN is now widely used in various applications such as industrial automation, medical equipment, and aerospace systems.
The CANBed kit provides an easy-to-use platform for engineers, hobbyists, and students to learn about CAN communication and develop CAN-based projects. It includes all the necessary hardware components and software libraries to get started with CAN programming on the Arduino platform.
Key Features of CANBed
- Arduino-compatible board with integrated CAN controller (MCP2515) and transceiver (TJA1050)
- Supports CAN 2.0A and CAN 2.0B protocols
- High-speed CAN communication up to 1 Mbps
- Screw terminal connectors for easy wiring
- LED indicators for power, TX, and RX
- Onboard 5V voltage regulator for powering external devices
- Compatible with Arduino IDE and popular CAN libraries (e.g., FlexCAN, mcp_can)
- Comprehensive documentation and example sketches
Getting Started with CANBed
Hardware Setup
To set up the CANBed hardware, follow these steps:
- Connect the CANBed board to your computer using a USB cable. The board will be powered through the USB connection.
- If you want to power external devices, connect a 7-12V DC power supply to the screw terminal labeled “VIN” and “GND”.
- Connect your CAN devices to the screw terminals labeled “CANH” and “CANL”. Ensure that the devices share a common ground with the CANBed board.
Software Setup
- Download and install the Arduino IDE from the official website: https://www.arduino.cc/en/software
- Open the Arduino IDE and navigate to File > Preferences.
- In the “Additional Boards Manager URLs” field, paste the following URL: https://github.com/Seeed-Studio/CANBed/raw/master/package_seeed_index.json
- Click “OK” to close the Preferences window.
- Navigate to Tools > Board > Boards Manager.
- Search for “CANBed” in the search bar and click on the “Install” button next to the CANBed entry.
- Once the installation is complete, select “CANBed CAN-Bus Dev Kit” from the Tools > Board menu.
CAN Communication Basics
Before diving into programming with CANBed, it’s essential to understand the basics of CAN communication.
CAN Bus Topology
A CAN bus system consists of multiple nodes (devices) connected to a shared two-wire bus. The two wires, called CAN High (CANH) and CAN Low (CANL), are used for differential signaling. This differential signaling makes CAN communication highly resistant to electromagnetic interference.
CAN Message Format
CAN messages are broadcast to all nodes on the bus, and each message has a unique identifier (ID) that determines its priority. The message format includes the following fields:
Field | Description |
---|---|
Start of Frame (SOF) | Indicates the beginning of a message |
Identifier (ID) | 11-bit (standard) or 29-bit (extended) unique message ID |
Remote Transmission Request (RTR) | Indicates if the message is a data frame or a remote frame |
Control | Contains the Data Length Code (DLC) and other control bits |
Data | Up to 8 bytes of payload data |
CRC | Cyclic Redundancy Check for error detection |
Acknowledgment (ACK) | Nodes acknowledge the receipt of a valid message |
End of Frame (EOF) | Indicates the end of a message |
CAN Bit Timing
CAN communication relies on precise bit timing to ensure accurate data transmission. The bit timing parameters include:
- Baud rate: The number of bits per second (bps) transmitted on the bus. Common baud rates include 125 kbps, 250 kbps, 500 kbps, and 1 Mbps.
- Propagation segment: The time required for the signal to propagate through the bus.
- Phase segments: Used to compensate for edge phase errors and synchronize nodes.
- Synchronization Jump Width (SJW): The maximum number of time quanta a node can adjust its bit timing during resynchronization.
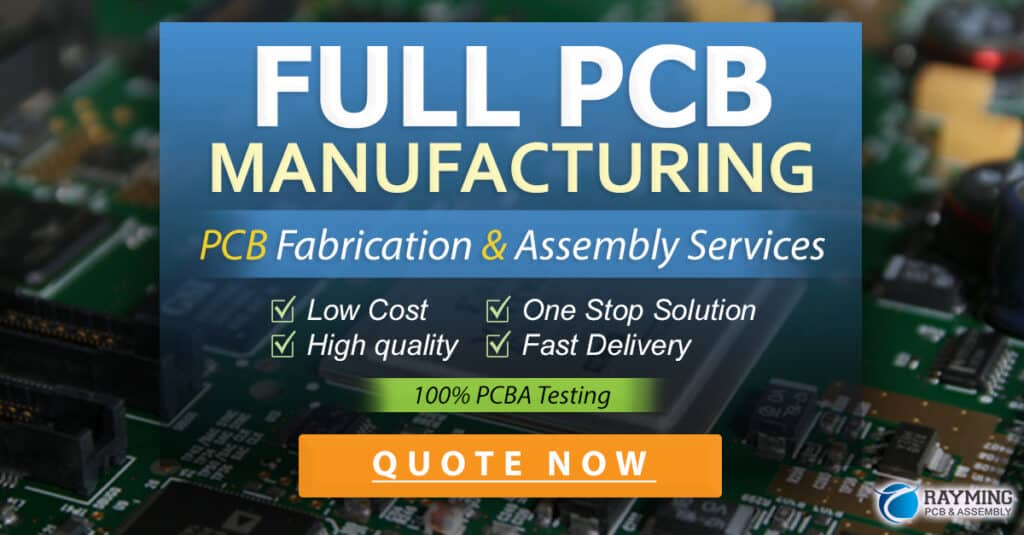
Programming with CANBed
With the hardware and software set up, you can start programming your CANBed board to send and receive CAN messages.
Sending CAN Messages
To send a CAN message using CANBed and the mcp_can library, follow these steps:
- Include the required libraries:
#include <SPI.h>
#include <mcp_can.h>
- Define the CS pin and create an MCP_CAN object:
const int SPI_CS_PIN = 10;
MCP_CAN CAN(SPI_CS_PIN);
- Initialize the CAN bus in the setup() function:
void setup() {
Serial.begin(115200);
while(!Serial);
if (CAN.begin(MCP_ANY, CAN_500KBPS, MCP_16MHZ) == CAN_OK) {
Serial.println("CAN initialized successfully.");
} else {
Serial.println("CAN initialization failed.");
while(1);
}
}
- Create a CAN message and send it in the loop() function:
void loop() {
unsigned char message[8] = {0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08};
unsigned long id = 0x100;
if (CAN.sendMsgBuf(id, 0, 8, message) == CAN_OK) {
Serial.println("Message sent successfully.");
} else {
Serial.println("Error sending message.");
}
delay(1000);
}
Receiving CAN Messages
To receive CAN messages with CANBed and the mcp_can library, use the following code:
-
Include the required libraries and set up the MCP_CAN object as shown in the previous section.
-
In the loop() function, check for incoming messages and process them:
void loop() {
unsigned char len = 0;
unsigned char buf[8];
if (CAN_MSGAVAIL == CAN.checkReceive()) {
CAN.readMsgBuf(&len, buf);
unsigned long id = CAN.getCanId();
Serial.print("Received message with ID: 0x");
Serial.print(id, HEX);
Serial.print(", Data: ");
for (int i = 0; i < len; i++) {
Serial.print(buf[i], HEX);
Serial.print(" ");
}
Serial.println();
}
}
Advanced CANBed Applications
CANBed can be used for a wide range of applications, from simple data logging to complex control systems. Here are a few advanced applications you can explore:
CAN-Based Data Logging
You can use CANBed to log data from multiple CAN devices and store it on an SD card or send it to a computer for analysis. To do this, you’ll need to:
- Connect an SD card module to the CANBed board using the SPI interface.
- Modify the code to receive CAN messages and write the data to the SD card.
- Implement a method to retrieve the logged data from the SD card for analysis.
Multi-Node CAN Network
CANBed can be used to create a multi-node CAN network, where multiple CANBed boards communicate with each other. To set up a multi-node network:
- Assign unique IDs to each CANBed board.
- Connect the CANH and CANL pins of all boards together.
- Modify the code on each board to send and receive messages with the appropriate IDs.
- Implement a higher-level protocol to manage communication between nodes.
CAN-Based Control Systems
CANBed can be used to control various actuators and sensors in a distributed control system. For example, you can:
- Connect CANBed to motor controllers, encoders, and limit switches to create a CAN-based motion control system.
- Implement PID control algorithms on the CANBed board to control the motion of the actuators based on feedback from the sensors.
- Use CANBed to monitor and control industrial processes by interfacing with sensors and actuators using CAN communication.
FAQs
1. What is the maximum baud rate supported by CANBed?
CANBed supports baud rates up to 1 Mbps, which is the maximum specified in the CAN 2.0 standard. However, the actual maximum baud rate may be limited by factors such as bus length, number of nodes, and signal quality.
2. Can I use CANBed with other Arduino libraries?
Yes, CANBed is compatible with other Arduino libraries. You can use it in combination with libraries for SD card modules, displays, sensors, and more. Just make sure to manage the pin assignments and avoid conflicts.
3. How many devices can be connected to a single CANBed board?
The number of devices that can be connected to a single CANBed board depends on the CAN bus’s physical characteristics, such as bus length and termination. In general, CAN supports up to 30 nodes per segment, but this number can be increased using repeaters or bridges.
4. Is CANBed suitable for automotive applications?
Yes, CANBed can be used for automotive applications. However, you should ensure that your application meets the relevant automotive standards (e.g., ISO 11898) and that the CANBed board is properly protected against the harsh automotive environment (e.g., using conformal coating).
5. Can I use CANBed with non-Arduino microcontrollers?
While CANBed is designed for use with Arduino boards, you can use it with other microcontrollers that support CAN communication. However, you’ll need to adapt the software and wiring to match your specific microcontroller and development environment.
Conclusion
CANBed is a versatile and user-friendly development kit that simplifies the process of working with CAN bus systems. With its Arduino-compatible design and comprehensive documentation, CANBed is an excellent choice for engineers, hobbyists, and students looking to learn about CAN communication and develop CAN-based projects.
By mastering CANBed, you’ll gain valuable skills in embedded systems, communication protocols, and control systems. These skills are highly sought-after in industries such as automotive, aerospace, and industrial automation.
So, whether you’re a beginner or an experienced developer, give CANBed a try and unlock the potential of CAN communication in your projects!
No responses yet