What is a Battery Charge Indicator?
A battery charge indicator is a device or system that shows the current charge level of a rechargeable battery. It allows the user to easily check how much charge is remaining in the battery at any given time. Battery Charge Indicators are commonly found in portable electronic devices like smartphones, laptops, power banks, and more.
Battery charge indicators work by measuring the voltage of the battery. As a battery discharges, its voltage decreases in a predictable manner. By comparing the current voltage to the known full and empty voltages, the charge level can be estimated and displayed to the user, usually as a percentage from 0-100%.
There are a few different ways that battery charge can be indicated:
LED Lights
Many devices use a series of LED lights to show battery charge level. Typically, 4 or 5 LEDs are used, with each LED representing about 20-25% of charge. As the battery drains, the LEDs will turn off one by one. When the battery is fully charged, all the LEDs will be illuminated.
LCD Screens
Some more advanced devices feature LCD screens that can display the precise battery percentage remaining. This gives the user more detailed information than just a few LEDs.
Software Indicators
In smart devices like phones and laptops, the battery charge is often displayed via software on the device’s screen. This might be shown as an icon, percentage, or even time estimate until empty or fully charged.
The Benefits of Battery Charge Indicators
Having a battery charge indicator offers several key advantages:
-
Knowing when to recharge – Battery charge indicators let the user know when the battery is running low so they can recharge it before it dies completely. This helps avoid the inconvenience of having a device unexpectedly shut off due to a dead battery.
-
Estimating remaining use time – By seeing the current charge level, users can estimate how much longer they can continue using the device before needing to recharge. This is helpful for planning purposes.
-
Preventing overcharging – Leaving a battery charging for too long can degrade its capacity over time. Charge indicators allow users to see when a battery has reached full charge so they can unplug it.
-
Diagnosing battery health – If a user notices their device’s battery is draining faster than normal or not reaching full charge, it could indicate the battery needs to be replaced. The charge indicator helps identify these issues.
How Battery Charge Indicators Work
To understand how battery charge indicators work, it’s important to first know a bit about the characteristics of rechargeable lithium ion batteries, the most common type used in portable electronics today.
Lithium Ion Battery Characteristics
Lithium ion batteries have a nominal voltage of 3.6V or 3.7V when fully charged. As the battery discharges, this voltage steadily decreases in a linear fashion. Most devices consider a lithium ion battery to be “empty” when its voltage falls to around 3.0V-3.2V, as going lower can damage the battery. The following table shows the approximate charge percentage at different voltage levels:
Battery Voltage | Charge Level |
---|---|
4.20V | 100% |
4.06V | 90% |
3.93V | 80% |
3.82V | 70% |
3.74V | 60% |
3.68V | 50% |
3.61V | 40% |
3.55V | 30% |
3.48V | 20% |
3.37V | 10% |
3.20V | 0% |
Voltage Measurement
To determine the battery’s charge level, the indicator needs to be able to measure its voltage. This is typically done using a simple voltage divider circuit connected to an analog-to-digital converter (ADC).
A voltage divider consists of two resistors in series, with the battery voltage applied across the whole divider, and the ADC measuring the voltage at the point between the two resistors. The values of the resistors set the range and resolution of the measurable voltage.
For example, to measure a ~4V lithium ion battery using a 5V ADC, we could use two resistors of equal value (let’s say 10K ohms each). This would divide the battery voltage in half, converting the 4V max battery voltage to a 2V max ADC input. The ADC would then read this value and software could easily convert it to a battery percentage.
Displaying Charge Level
Once the ADC has measured the battery voltage and converted it to a charge percentage, this information needs to be displayed to the user in a friendly way. As discussed before, this is usually done via a series of LEDs, an LCD screen, or a software battery meter.
For an LED indicator, the software simply needs to determine which LEDs to light up based on the charge percentage. For example:
Charge Level | LEDs Lit |
---|---|
80% – 100% | 4 LEDs |
60% – 79% | 3 LEDs |
40% – 59% | 2 LEDs |
20% – 39% | 1 LED |
0% – 19% | 0 LEDs (flashing) |
An LCD screen or software indicator has even more flexibility in how it displays the information since it’s not limited to a few discrete LEDs. It can display the exact percentage, a battery icon corresponding to the level, or even an estimate of remaining run time.
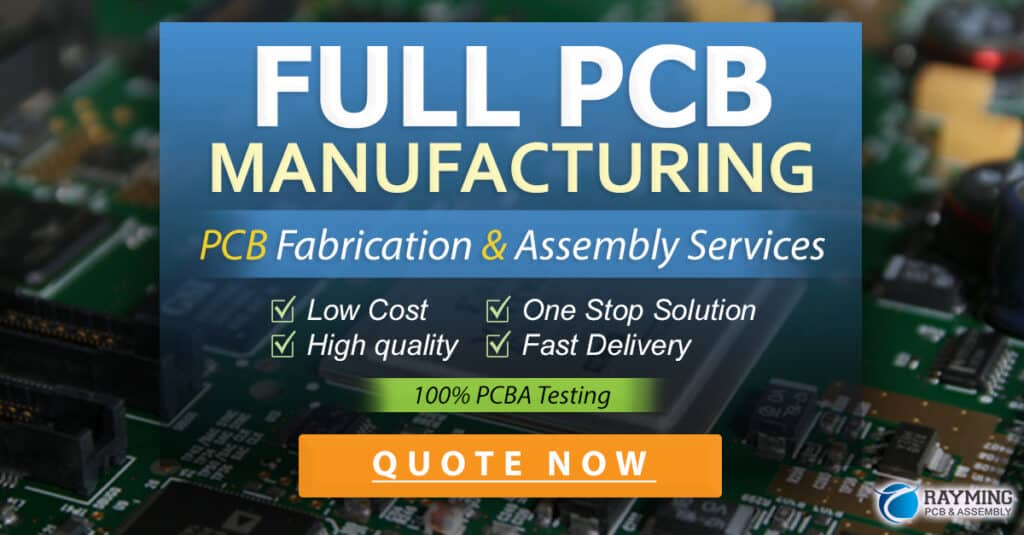
How to Make a DIY Battery Charge Indicator
Building your own battery charge indicator is a great way to learn about the fundamentals of ADCs, programming, and circuit design. Here’s a simple project that shows the core concepts of a battery charge indicator. We’ll make one using an Arduino microcontroller, a few LEDs and resistors, and a lithium ion battery.
Materials Needed
- Arduino Uno
- Breadboard
- Jumper wires
- 2x 10K ohm resistors
- 4x LEDs (any color)
- 4x 220 ohm resistors
- 3.7V lithium ion battery
- Battery holder with on/off switch
The Circuit
- Place the Arduino on the breadboard.
- Connect the 5V pin of the Arduino to the breadboard’s power rail.
- Connect the GND pin of the Arduino to the breadboard’s ground rail.
- Connect one end of a 10K resistor to the Arduino’s 5V pin, and the other end to an empty row on the breadboard.
- Connect a jumper wire from the A0 pin on the Arduino to the same row as the 10K resistor.
- Connect another 10K resistor from the breadboard row connected to the A0 pin to the Arduino’s GND pin.
- Connect the positive terminal of the lithium battery to the breadboard row with the first 10K resistor.
- Connect the negative terminal of the battery to the Arduino’s GND pin.
- Place the 4 LEDs on the breadboard. For each LED:
- Connect the anode (longer leg) to a digital I/O pin on the Arduino (let’s use pins 2-5).
- Connect a 220 ohm resistor between the cathode (shorter leg) and the ground rail.
When finished, your circuit should look something like this:
5V ---|10K|---+--- A0
|
|
|
+---|10K|--- GND
|
|
BAT
|
|
GND
Digital Pins:
2 ---|LED|--+
|
|220
GND
3 ---|LED|--+
|
|220
GND
4 ---|LED|--+
|
|220
GND
5 ---|LED|--+
|
|220
GND
The Code
Here’s the Arduino code to read the battery voltage and light up the LEDs based on the charge level:
// Define analog input
#define BATTERY_PIN A0
// Define LED outputs
#define LED_1 2
#define LED_2 3
#define LED_3 4
#define LED_4 5
// Define battery levels in volts
#define BATTERY_MAX 4.2
#define BATTERY_MIN 3.2
void setup() {
// Set LED pins as outputs
pinMode(LED_1, OUTPUT);
pinMode(LED_2, OUTPUT);
pinMode(LED_3, OUTPUT);
pinMode(LED_4, OUTPUT);
}
void loop() {
// Read battery voltage
float voltage = analogRead(BATTERY_PIN) * (5.0 / 1023.0) * 2.0;
// Convert voltage to percentage
int percentage = 100 * ((voltage - BATTERY_MIN) / (BATTERY_MAX - BATTERY_MIN));
percentage = min(100, max(0, percentage)); // Clamp to 0-100%
// Light LEDs based on percentage
digitalWrite(LED_1, percentage >= 25);
digitalWrite(LED_2, percentage >= 50);
digitalWrite(LED_3, percentage >= 75);
digitalWrite(LED_4, percentage >= 95);
delay(500); // Wait half a second before next reading
}
Let’s break this down section by section:
-
First, we define some constants for the analog input pin connected to the voltage divider (A0) and the digital output pins connected to the LEDs (2-5). We also define the max and min voltages of our lithium battery.
-
In the
setup()
function, we set the LED pins as outputs. -
In the
loop()
function, we read the analog voltage from the divider circuit. TheanalogRead()
function returns a value from 0-1023 corresponding to a voltage from 0-5V. We convert this to the actual voltage by multiplying by5.0 / 1023.0
. -
However, remember that our voltage divider halves the battery voltage, so we multiply by 2 to get the real battery voltage.
-
Next, we convert the voltage to a percentage using the formula:
percentage = 100 * ((voltage - BATTERY_MIN) / (BATTERY_MAX - BATTERY_MIN))
. This maps the battery voltage to a percentage from 0-100%. We usemin()
andmax()
to ensure the percentage stays within 0-100% even if the voltage goes outside the expected range. -
Finally, we use the percentage to determine which LEDs to light up. Each LED corresponds to a quartile of the percentage range, so LED_1 lights up for 25% and above, LED_2 for 50% and above, etc.
-
We call
delay(500)
to pause for half a second before repeating the loop.
Upload this code to your Arduino and you should see the LEDs light up according to the battery level! Try disconnecting the battery and replacing it with a variable power supply if you have one – you can then adjust the voltage and see how the LEDs respond.
Frequently Asked Questions
1. How accurate are battery charge indicators?
Battery charge indicators are generally fairly accurate, especially those that use voltage-based sensing like the one in our project. However, there are some factors that can affect accuracy:
-
Battery age and health: As batteries age, their capacity decreases and voltage behavior changes slightly, so an indicator calibrated for a new battery may become less accurate over time.
-
Temperature: Battery voltage varies with temperature. Most indicators are calibrated for room temperature, so extreme hot or cold environments can reduce accuracy.
-
Load: A battery’s voltage will dip temporarily under heavy load, which could cause the charge indicator to read lower than the actual average charge level.
-
Indicator quality: Cheaper or poorly calibrated indicators may have lower accuracy.
In general, you can expect around ±5-10% accuracy from a voltage-based battery charge indicator, which is sufficient for most practical purposes.
2. Can I use this Arduino project with a different kind of battery?
Yes, the concept can be adapted to other battery chemistries. The main things you’ll need to change are:
-
The
BATTERY_MAX
andBATTERY_MIN
constants should be set to the full and empty voltages for your specific battery chemistry. -
The voltage divider resistor values may need to be adjusted to ensure the battery voltage gets mapped to the 0-5V range of the Arduino’s ADC.
3. Can I make a more precise indicator with a numerical display?
Absolutely! Instead of using LEDs, you can connect an LCD screen or even a small OLED display to show the exact percentage or battery voltage. You’ll need to modify the code to output to the screen instead of the LEDs.
For a simple character LCD, you can use the LiquidCrystal
library to print the percentage:
#include <LiquidCrystal.h>
LiquidCrystal lcd(7, 8, 9, 10, 11, 12);
void setup() {
lcd.begin(16, 2);
}
void loop() {
// ... Battery reading code ...
lcd.clear();
lcd.print("Battery: ");
lcd.print(percentage);
lcd.print("%");
delay(500);
}
4. What about a low battery warning?
It’s easy to add a low battery warning to the project. One way would be to flash an LED or print a message to the LCD when the percentage drops below a certain threshold, say 20%.
if (percentage <= 20) {
digitalWrite(LOW_BATTERY_LED, millis() % 200 < 100); // Flash at 2.5Hz
}
Alternatively, you could sound an audible warning by connecting a small speaker or piezo buzzer.
5. Can I use this indicator for rechargeable AA or AAA batteries?
The principles are the same, but you would need to make a few changes:
- Use a battery holder that fits your chosen battery size.
- Adjust the voltage divider for the lower voltage of the batteries (1.5V max for alkaline, 1.2V for NiMH).
- Change the
BATTERY_MAX
andBATTERY_MIN
constants. For NiMH, a typical full voltage is about 1.4V and empty is around 1.0V.
Keep in mind that the discharge curve of NiMH batteries is less linear than lithium ion, so the percentage calculation may be a bit less accurate, especially in the middle of the range. But it can still give a useful indication of remaining charge.
Conclusion
Battery charge indicators are a small but essential feature in portable electronics. They help us manage our device usage and avoid unexpected power loss. By understanding the basics of how they work – measuring battery voltage and mapping it to a percentage – you can not only use battery-powered devices more effectively, but even build your own indicator for any project.
With a simple microcontroller like Arduino and a few extra components, it’s easy to add an informative battery meter to your own battery-powered projects. And once you understand the core concept, the possibilities are endless – from simple LED “fuel gauges” to sophisticated numerical displays, low battery alarms, and more.
So next time you glance at your phone’s battery icon, spare a thought for the clever electronics working behind the scenes to keep you informed. And if you’re inspired to try your hand at a DIY battery monitor, go for it! It’s a fun and rewarding project that will give you a deeper appreciation for this ubiquitous but often overlooked technology.
No responses yet