What is the ATTINY45 Microcontroller?
The ATTINY45 is an 8-bit AVR microcontroller that comes in a small 8-pin package. It features 4 KB of flash memory, 256 bytes of EEPROM, and 256 bytes of SRAM. The microcontroller operates at a frequency of up to 20 MHz and supports a wide range of peripherals, including:
- 8-bit Timer/Counter with Prescaler
- 16-bit Timer/Counter with Prescaler
- USI (Universal Serial Interface)
- 10-bit ADC (Analog-to-Digital Converter)
- Analog Comparator
- Watchdog Timer
- Power-on Reset and Programmable Brown-out Detection
- Internal Calibrated Oscillator
ATTINY45 Pinout
To effectively use the ATTINY45 microcontroller, it’s essential to understand its pinout. The following table and image provide a detailed overview of the ATTINY45 pinout:
Pin Number | Pin Name | Description |
---|---|---|
1 | PB5 | GPIO, ADC0, PCINT5, RESET |
2 | PB3 | GPIO, ADC3, PCINT3, XTAL1, CLKI |
3 | PB4 | GPIO, ADC2, PCINT4, XTAL2, CLKO |
4 | GND | Ground |
5 | PB0 | GPIO, AIN0, OC0A, MOSI, PCINT0, SDA |
6 | PB1 | GPIO, AIN1, OC0B, MISO, PCINT1, DO |
7 | PB2 | GPIO, ADC1, SCK, PCINT2, SCL, USCK |
8 | VCC | Supply Voltage |
GPIO Pins
The ATTINY45 has 6 GPIO (General Purpose Input/Output) pins: PB0 to PB5. These pins can be configured as either inputs or outputs and are used for digital communication with external devices, such as sensors, LEDs, and switches.
ADC Pins
The microcontroller features a 10-bit ADC with 4 channels: ADC0 to ADC3. These pins can be used to measure analog voltages and convert them to digital values for processing. The ADC has a resolution of 1024 steps and can measure voltages between 0V and VCC.
PCINT Pins
All 6 GPIO pins can also function as PCINT (Pin Change Interrupt) pins. These pins can trigger interrupts when a change in the pin state is detected, allowing for efficient event-driven programming.
USI Pins
The ATTINY45 has a USI (Universal Serial Interface) that can be configured to support various serial communication protocols, such as SPI and I2C. The USI uses the following pins:
- PB0 (MOSI, SDA)
- PB1 (MISO, DO)
- PB2 (SCK, USCK, SCL)
XTAL Pins
PB3 and PB4 can be used to connect an external crystal oscillator for more precise timing. If an external oscillator is not used, these pins can function as regular GPIO pins.
Reset Pin
PB5 serves as the reset pin for the microcontroller. When this pin is pulled low, the microcontroller restarts its execution from the beginning of the program.
Programming the ATTINY45
To program the ATTINY45, you’ll need a programmer, such as the AVR ISP (In-System Programmer) or the USBASP. The microcontroller can be programmed using the Arduino IDE or Atmel Studio.
Using the Arduino IDE
- Install the Arduino IDE on your computer.
- Add support for the ATTINY45 by installing the “ATTinyCore” package in the Arduino IDE’s Board Manager.
- Select “ATtiny25/45/85” from the “Tools > Board” menu and choose the appropriate clock speed and programmer.
- Write your code in the Arduino IDE and click the “Upload” button to program the microcontroller.
Using Atmel Studio
- Install Atmel Studio on your computer.
- Create a new project and select the ATTINY45 as the target device.
- Write your code in Atmel Studio using C or Assembly language.
- Connect your programmer to the ATTINY45 and click the “Debug” button to program the microcontroller.
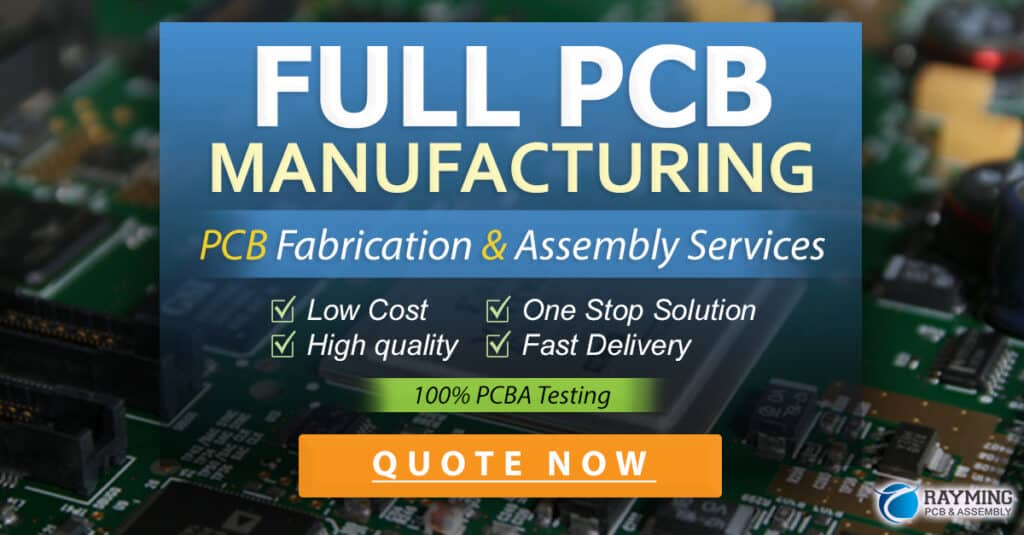
Example Projects
Here are a few example projects that demonstrate the capabilities of the ATTINY45:
LED Blink
This simple project blinks an LED connected to one of the GPIO pins.
#include <avr/io.h>
#include <util/delay.h>
#define LED_PIN PB0
int main() {
DDRB |= (1 << LED_PIN); // Set LED_PIN as output
while (1) {
PORTB ^= (1 << LED_PIN); // Toggle LED_PIN
_delay_ms(500); // Delay for 500ms
}
return 0;
}
Analog Temperature Sensor
This project demonstrates how to use the ADC to read an analog temperature sensor (e.g., LM35) and display the temperature on a serial monitor.
#include <avr/io.h>
#include <util/delay.h>
#include <stdio.h>
#define ADC_PIN PB2
void uart_init() {
UBRRL = 103; // Set baud rate to 9600 at 20MHz
UCSRB = (1 << TXEN); // Enable UART transmitter
}
void uart_putchar(char c) {
while (!(UCSRA & (1 << UDRE))); // Wait until UART data register is empty
UDR = c; // Send character
}
int main() {
uart_init();
ADMUX = (1 << REFS0) | (ADC_PIN & 0x0F); // Set ADC reference to VCC and select ADC pin
ADCSRA = (1 << ADEN) | (1 << ADPS2) | (1 << ADPS1); // Enable ADC and set prescaler to 64
char temperature[10];
while (1) {
ADCSRA |= (1 << ADSC); // Start ADC conversion
while (ADCSRA & (1 << ADSC)); // Wait for conversion to complete
uint16_t adc_value = ADC; // Read ADC value
float temperature_c = (adc_value * 5.0 / 1024.0) * 100.0; // Convert ADC value to temperature in Celsius
sprintf(temperature, "%.2f\n", temperature_c); // Format temperature as string
for (uint8_t i = 0; temperature[i] != '\0'; i++) {
uart_putchar(temperature[i]); // Send temperature string over UART
}
_delay_ms(1000); // Delay for 1 second
}
return 0;
}
FAQs
-
Q: What is the difference between the ATTINY45 and ATTINY85?
A: The main difference between the ATTINY45 and ATTINY85 is the amount of memory. The ATTINY45 has 4 KB of flash memory, while the ATTINY85 has 8 KB. Both microcontrollers share the same pinout and peripheral features. -
Q: Can I use the ATTINY45 with the Arduino IDE?
A: Yes, you can use the ATTINY45 with the Arduino IDE by installing the “ATTinyCore” package in the Board Manager. This package adds support for various ATTINY microcontrollers, including the ATTINY45. -
Q: What is the maximum operating frequency of the ATTINY45?
A: The ATTINY45 can operate at a maximum frequency of 20 MHz when powered with 4.5V to 5.5V. At lower voltages, the maximum operating frequency decreases. -
Q: How many PWM channels does the ATTINY45 have?
A: The ATTINY45 has two PWM channels: OC0A (PB0) and OC0B (PB1). These channels are associated with the 8-bit Timer/Counter0. -
Q: Can I use the ATTINY45 in low-power applications?
A: Yes, the ATTINY45 is well-suited for low-power applications. It features various sleep modes and power-reduction techniques that can significantly reduce power consumption when the microcontroller is not actively performing tasks.
Conclusion
The ATTINY45 is a versatile and compact microcontroller that offers a wide range of features for embedded systems, robotics, and IoT projects. By understanding the ATTINY45 pinout and its capabilities, you can create efficient and innovative projects with ease. Whether you’re a beginner or an experienced developer, the ATTINY45 is an excellent choice for your next microcontroller-based project.
No responses yet