Introduction to Atmega2560
The Atmega2560 is an 8-bit microcontroller based on the AVR enhanced RISC architecture. It offers a wide range of features and capabilities, making it suitable for a variety of applications, from simple hobby projects to complex industrial systems.
Key Features
- High-performance, low-power AVR® 8-bit microcontroller
- Advanced RISC architecture
- 135 powerful instructions
- 32 x 8 general purpose working registers
- Fully static operation
- Non-volatile program and data memories
- 256K bytes of in-system self-programmable Flash
- 4K bytes EEPROM
- 8K bytes internal SRAM
- Peripheral features
- Two 8-bit timer/counters with separate prescaler and compare mode
- Four 16-bit timer/counters with separate prescaler, compare mode, and capture mode
- Real-time counter with separate oscillator
- Six PWM channels
- 16-channel, 10-bit ADC
- Programmable serial USART
- Master/slave SPI serial interface
- Byte-oriented 2-wire serial interface (Philips I2C compatible)
- Programmable watchdog timer with separate on-chip oscillator
- On-chip analog comparator
- Special microcontroller features
- Power-on reset and programmable brown-out detection
- Internal calibrated oscillator
- External and internal interrupt sources
- Six sleep modes
- I/O and packages
- 86 programmable I/O lines
- 100-pin TQFP package
- Operating voltage
- 4.5V to 5.5V (Atmega2560V)
- 2.7V to 5.5V (Atmega2560)
- Temperature range
- -40°C to 85°C (industrial)
Atmega2560 Pinout and Configuration
Understanding the pinout and configuration of the Atmega2560 is crucial for properly connecting peripherals and designing circuits. The following table provides an overview of the Atmega2560’s pinout:
Pin Range | Description |
---|---|
VCC | Supply voltage |
GND | Ground |
Port A (PA0-PA7) | 8-bit bi-directional I/O port with internal pull-up resistors |
Port B (PB0-PB7) | 8-bit bi-directional I/O port with internal pull-up resistors |
Port C (PC0-PC7) | 8-bit bi-directional I/O port with internal pull-up resistors |
Port D (PD0-PD7) | 8-bit bi-directional I/O port with internal pull-up resistors |
Port E (PE0-PE7) | 8-bit bi-directional I/O port with internal pull-up resistors |
Port F (PF0-PF7) | 8-bit bi-directional I/O port with internal pull-up resistors |
Port G (PG0-PG5) | 6-bit bi-directional I/O port with internal pull-up resistors |
Port H (PH0-PH7) | 8-bit bi-directional I/O port with internal pull-up resistors |
Port J (PJ0-PJ7) | 8-bit bi-directional I/O port with internal pull-up resistors |
Port K (PK0-PK7) | 8-bit bi-directional I/O port with internal pull-up resistors |
Port L (PL0-PL7) | 8-bit bi-directional I/O port with internal pull-up resistors |
RESET | Reset input |
XTAL1 | External oscillator input |
XTAL2 | External oscillator output |
For a more detailed pinout diagram, please refer to the Atmega2560 datasheet.
Power Supply and Clock Configuration
The Atmega2560 can operate on a wide range of power supply voltages and clock frequencies. The following table summarizes the power supply and clock configuration options:
Parameter | Atmega2560 | Atmega2560V |
---|---|---|
Operating voltage | 2.7V to 5.5V | 4.5V to 5.5V |
Operating frequency | Up to 16 MHz | Up to 16 MHz |
Power consumption @ 1 MHz, 3V, 25°C | Active: 1.1 mA, Power-down: 0.1 μA | Active: 1.1 mA, Power-down: 0.1 μA |
The Atmega2560 supports various clock sources, including:
- External crystal/ceramic resonator
- External low-frequency crystal
- Internal calibrated RC oscillator
- External clock input
To configure the clock source and frequency, use the appropriate fuse settings as described in the datasheet.
Programming the Atmega2560
Programming the Atmega2560 involves writing code in C or Assembly language, compiling it, and uploading the generated binary to the microcontroller’s flash memory. There are several ways to program the Atmega2560, including:
Using the Arduino IDE
- Install the Arduino IDE from the official website (https://www.arduino.cc/en/software).
- Open the Arduino IDE and select “Tools” > “Board” > “Arduino Mega or Mega 2560”.
- Write your code in the Arduino IDE using the Arduino programming language (based on C/C++).
- Connect your Atmega2560 board to your computer using a USB cable.
- Select the appropriate serial port under “Tools” > “Port”.
- Click the “Upload” button to compile and upload your code to the Atmega2560.
Using Atmel Studio
- Download and install Atmel Studio from the Microchip website (https://www.microchip.com/mplab/avr-support/atmel-studio-7).
- Create a new project in Atmel Studio and select the Atmega2560 as your target device.
- Write your code in C or Assembly language.
- Configure your programmer (e.g., JTAG, ISP) in the project settings.
- Build your project and upload the generated binary to the Atmega2560 using your programmer.
Using avr-gcc and avrdude
- Install avr-gcc (AVR GNU Compiler Collection) and avrdude (AVR Downloader/UploaDEr) on your system.
- Write your code in C or Assembly language.
- Use avr-gcc to compile your code and generate a binary file.
- Use avrdude to upload the binary file to the Atmega2560 using a programmer (e.g., JTAG, ISP).
Example command for compiling with avr-gcc:
avr-gcc -mmcu=atmega2560 -O2 -o output.elf input.c
Example command for uploading with avrdude:
avrdude -c stk500v2 -p m2560 -P /dev/ttyUSB0 -U flash:w:output.hex
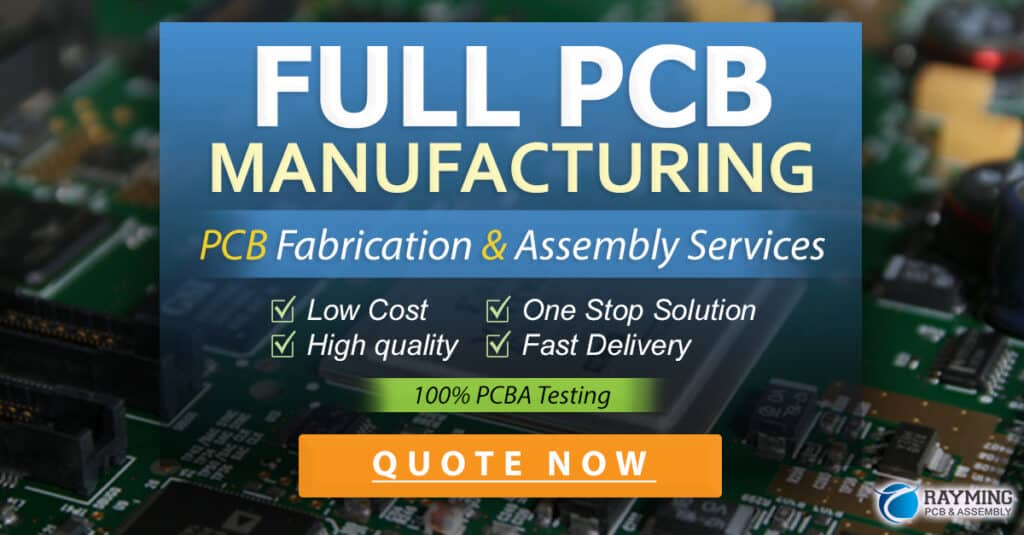
Atmega2560 Peripherals and Libraries
The Atmega2560 offers a wide range of built-in peripherals, which can be easily controlled using pre-existing libraries or by directly accessing the corresponding registers.
GPIO (General Purpose Input/Output)
- Configure and control individual I/O pins
- Use libraries like
avr/io.h
for register access
Example code for toggling an LED connected to PB7:
#include <avr/io.h>
#include <util/delay.h>
int main() {
DDRB |= (1 << PB7); // Set PB7 as output
while (1) {
PORTB ^= (1 << PB7); // Toggle PB7
_delay_ms(500); // Delay for 500ms
}
return 0;
}
ADC (Analog-to-Digital Converter)
- 16-channel, 10-bit ADC
- Use libraries like
avr/io.h
andavr/interrupt.h
for configuration and interrupt handling
Example code for reading an analog value from ADC0:
#include <avr/io.h>
int main() {
ADMUX = (1 << REFS0); // Set ADC reference to AVCC
ADCSRA = (1 << ADEN) | (1 << ADPS2) | (1 << ADPS1) | (1 << ADPS0); // Enable ADC, set prescaler to 128
while (1) {
ADCSRA |= (1 << ADSC); // Start conversion
while (ADCSRA & (1 << ADSC)); // Wait for conversion to complete
uint16_t adc_value = ADC; // Read ADC value
// Do something with adc_value
}
return 0;
}
Timer/Counter
- Two 8-bit timers and four 16-bit timers
- Use libraries like
avr/io.h
andavr/interrupt.h
for configuration and interrupt handling
Example code for generating a PWM signal on OC1A (PB5):
#include <avr/io.h>
int main() {
DDRB |= (1 << PB5); // Set PB5 as output
ICR1 = 19999; // Set ICR1 for 50 Hz PWM at 16 MHz
OCR1A = 1999; // Set OCR1A for 10% duty cycle
TCCR1A = (1 << COM1A1) | (1 << WGM11); // Set non-inverting mode and 10-bit phase correct PWM
TCCR1B = (1 << WGM13) | (1 << WGM12) | (1 << CS10); // Set prescaler to 1 and start timer
while (1) {
// Main loop
}
return 0;
}
USART (Universal Synchronous/Asynchronous Receiver/Transmitter)
- Programmable serial communication interface
- Use libraries like
avr/io.h
andavr/interrupt.h
for configuration and interrupt handling
Example code for transmitting data over USART0:
#include <avr/io.h>
#include <util/delay.h>
void USART_transmit(uint8_t data) {
while (!(UCSR0A & (1 << UDRE0))); // Wait for empty transmit buffer
UDR0 = data; // Put data into buffer, sends the data
}
int main() {
UBRR0H = (103 >> 8); // Set baud rate to 9600 at 16 MHz
UBRR0L = 103;
UCSR0B = (1 << TXEN0); // Enable transmitter
UCSR0C = (1 << UCSZ01) | (1 << UCSZ00); // Set 8-bit data format
while (1) {
USART_transmit('H');
USART_transmit('e');
USART_transmit('l');
USART_transmit('l');
USART_transmit('o');
USART_transmit('\n');
_delay_ms(1000);
}
return 0;
}
Frequently Asked Questions (FAQ)
-
Q: What is the difference between Atmega2560 and Atmega2560V?
A: The main difference is the operating voltage range. Atmega2560 operates from 2.7V to 5.5V, while Atmega2560V operates from 4.5V to 5.5V. -
Q: Can I use the Arduino IDE to program the Atmega2560?
A: Yes, the Arduino IDE fully supports the Atmega2560. Simply select “Arduino Mega or Mega 2560” from the “Tools” > “Board” menu. -
Q: What is the maximum clock frequency of the Atmega2560?
A: The Atmega2560 can operate at a maximum clock frequency of 16 MHz. -
Q: How much flash memory does the Atmega2560 have?
A: The Atmega2560 has 256 KB of flash memory for storing the program code. -
Q: Can I use the Atmega2560 for battery-powered applications?
A: Yes, the Atmega2560 has various sleep modes and low-power features that make it suitable for battery-powered applications. However, for ultra-low-power applications, you may consider using microcontrollers specifically designed for that purpose, such as the Atmel ATtiny series.
Conclusion
The Atmega2560 is a versatile and powerful microcontroller that offers a wide range of features and capabilities. With its extensive I/O ports, various peripherals, and ample memory, the Atmega2560 is well-suited for a wide range of applications, from simple hobby projects to complex embedded systems.
By understanding the pinout, configuration, programming methods, and available libraries, you can effectively utilize the Atmega2560 to create innovative and efficient solutions. Whether you are using the Arduino IDE, Atmel Studio, or avr-gcc with avrdude, the Atmega2560 provides a solid foundation for your embedded projects.
For more detailed information, please refer to the official Atmega2560 datasheet and the Atmel AVR user guides.
No responses yet