What is an Arduino Solar Tracker?
An Arduino solar tracker is a device that uses an Arduino microcontroller to control the movement of solar panels, ensuring that they are always oriented towards the sun. By constantly adjusting the panels’ position, the solar tracker maximizes the amount of sunlight captured, resulting in increased energy production.
Key Components of an Arduino Solar Tracker
To build an Arduino solar tracker, you will need the following components:
- Arduino board (e.g., Arduino Uno)
- Light-dependent resistors (LDRs)
- Servo motors
- Solar panels
- Breadboard
- Jumper wires
- Resistors
How Does an Arduino Solar Tracker Work?
The Arduino solar tracker works by using light-dependent resistors (LDRs) to detect the position of the sun. LDRs are sensors that change their resistance based on the amount of light they receive. By strategically placing multiple LDRs around the solar panel, the Arduino can determine the direction of the sun and adjust the panel’s orientation accordingly.
The Role of Servo Motors
Servo motors are responsible for physically moving the solar panels in response to the Arduino’s commands. The Arduino reads the data from the LDRs and sends signals to the servo motors to rotate the panels, ensuring they are always facing the sun at the optimal angle.
Building Your Arduino Solar Tracker
Now that you understand the basic principles behind an Arduino solar tracker, let’s dive into the step-by-step process of building one.
Step 1: Gather the Required Components
Make sure you have all the necessary components listed in the “Key Components” section above. You can purchase these items from various online retailers or local electronics stores.
Step 2: Assemble the Circuit
- Connect the LDRs to the breadboard and wire them to the Arduino’s analog input pins.
- Attach the servo motors to the Arduino’s digital output pins.
- Use jumper wires to make the necessary connections between the components.
Here’s a table summarizing the connections:
Component | Arduino Pin |
---|---|
LDR 1 | A0 |
LDR 2 | A1 |
Servo Motor 1 | D9 |
Servo Motor 2 | D10 |
Step 3: Write the Arduino Code
Now it’s time to write the code that will control your Arduino solar tracker. The code will read the values from the LDRs, determine the position of the sun, and send commands to the servo motors to adjust the solar panel’s orientation.
Here’s a basic example of the Arduino code:
#include <Servo.h>
Servo servoPan;
Servo servoTilt;
int ldrTopLeft = A0;
int ldrTopRight = A1;
int ldrBottomLeft = A2;
int ldrBottomRight = A3;
void setup() {
servoPan.attach(9);
servoTilt.attach(10);
}
void loop() {
int topLeft = analogRead(ldrTopLeft);
int topRight = analogRead(ldrTopRight);
int bottomLeft = analogRead(ldrBottomLeft);
int bottomRight = analogRead(ldrBottomRight);
int averageTop = (topLeft + topRight) / 2;
int averageBottom = (bottomLeft + bottomRight) / 2;
int averageLeft = (topLeft + bottomLeft) / 2;
int averageRight = (topRight + bottomRight) / 2;
if (averageTop < averageBottom) {
servoTilt.write(servoTilt.read() + 1);
} else if (averageTop > averageBottom) {
servoTilt.write(servoTilt.read() - 1);
}
if (averageLeft < averageRight) {
servoPan.write(servoPan.read() + 1);
} else if (averageLeft > averageRight) {
servoPan.write(servoPan.read() - 1);
}
delay(50);
}
Step 4: Upload the Code and Test
- Connect your Arduino board to your computer using a USB cable.
- Open the Arduino IDE and copy the code into a new sketch.
- Verify and upload the code to your Arduino board.
- Power up the Arduino solar tracker and observe its movement as it tracks the sun.
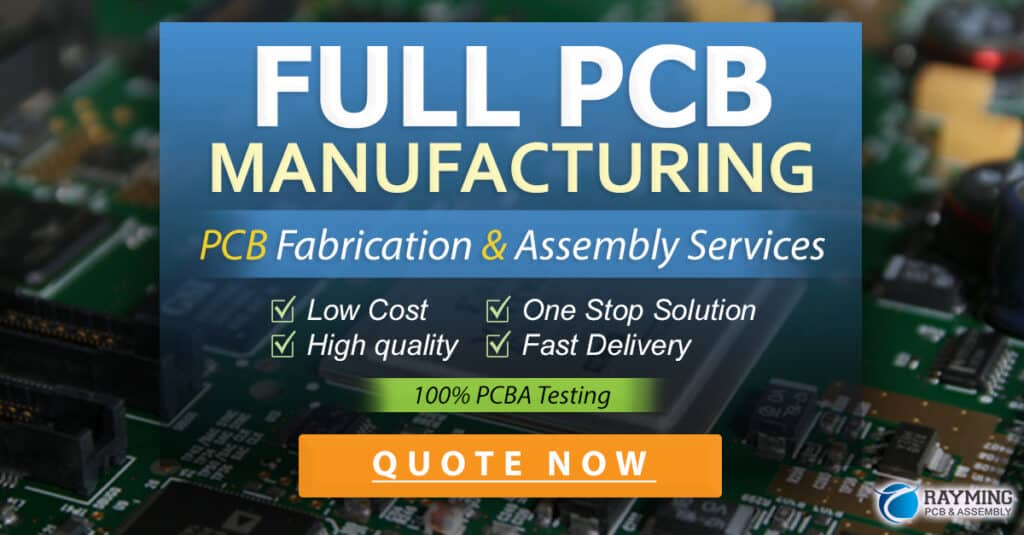
Enhancing Your Arduino Solar Tracker
Once you have a basic Arduino solar tracker up and running, you can consider adding additional features and enhancements to improve its performance and functionality.
Adding a Real-Time Clock (RTC)
By incorporating a real-time clock module, you can program your solar tracker to adjust its position based on the time of day. This can be particularly useful for optimizing energy production during peak sunlight hours.
Implementing a Dual-Axis Tracking System
While the basic Arduino solar tracker described in this article uses a single-axis tracking system (moving the panels horizontally), you can upgrade to a dual-axis system that also adjusts the tilt angle of the panels. This allows for even more precise sun tracking and increased energy efficiency.
Integrating Weather Sensors
Adding weather sensors, such as temperature and humidity sensors, can help you monitor the environmental conditions affecting your solar panels. This data can be used to optimize the performance of your solar tracker and protect your equipment from extreme weather conditions.
Frequently Asked Questions (FAQ)
-
Q: Can I use any type of solar panel with an Arduino solar tracker?
A: Yes, you can use any type of solar panel with an Arduino solar tracker, as long as it is compatible with the servo motors and can be securely mounted to the tracking mechanism. -
Q: How much energy can an Arduino solar tracker save compared to fixed solar panels?
A: The energy savings from using an Arduino solar tracker can vary depending on factors such as location, weather conditions, and the efficiency of the solar panels. However, studies have shown that solar tracking systems can increase energy production by 20-30% compared to fixed panels. -
Q: Can I power the Arduino solar tracker using the solar panels themselves?
A: Yes, it is possible to power the Arduino solar tracker using the energy generated by the solar panels. However, you will need to include additional components, such as a battery and charge controller, to ensure a stable power supply. -
Q: How often should I adjust the code for my Arduino solar tracker?
A: Once you have uploaded the code to your Arduino board, it should continue to function without needing frequent adjustments. However, you may need to update the code if you add new features or components to your solar tracker. -
Q: Can I scale up the Arduino solar tracker for larger solar panel installations?
A: While the basic Arduino solar tracker described in this article is suitable for small-scale projects, scaling up to larger installations may require more powerful motors and a more robust tracking mechanism. However, the fundamental principles of using an Arduino and LDRs for sun tracking remain the same.
Conclusion
Creating an Arduino solar tracker is an excellent way to maximize the efficiency of your solar panels and harness the full potential of solar energy. By following the steps outlined in this article, you can build your own solar tracking system and enjoy the benefits of increased energy production.
Remember to start with a basic design and gradually add enhancements as you become more comfortable with the technology. With a little patience and experimentation, you can create a highly effective Arduino solar tracker that will serve you well for years to come.
No responses yet