What is the Arduino Serial Monitor?
The Arduino Serial Monitor is a built-in feature of the Arduino IDE (Integrated Development Environment) that allows you to send and receive data between your Arduino board and a computer. It provides a simple way to debug your code, monitor sensor readings, and interact with your Arduino projects in real-time.
How does the Serial Monitor work?
The Serial Monitor establishes a serial communication link between your Arduino board and your computer using the USB cable. It uses the Universal Asynchronous Receiver/Transmitter (UART) protocol to send and receive data. When you open the Serial Monitor in the Arduino IDE, it listens for any incoming data from the Arduino board and displays it on the screen. You can also send data from the computer to the Arduino board using the input field at the bottom of the Serial Monitor window.
Setting up the Serial Monitor
To use the Serial Monitor, you first need to set up serial communication in your Arduino sketch. Here’s how you can do it:
- Open the Arduino IDE and create a new sketch or open an existing one.
- In the
setup()
function, initialize serial communication using theSerial.begin()
function. Specify the baud rate (data transfer speed) as a parameter. For example:
cpp
void setup() {
Serial.begin(9600);
}
- In the
loop()
function, you can send data to the Serial Monitor using theSerial.print()
orSerial.println()
functions. For example:
cpp
void loop() {
Serial.println("Hello, Serial Monitor!");
delay(1000);
}
- Upload the sketch to your Arduino board.
- Open the Serial Monitor by clicking on the magnifying glass icon in the top-right corner of the Arduino IDE or by selecting “Tools” > “Serial Monitor” from the menu.
Using the Serial Monitor
Now that you have set up the Serial Monitor, let’s explore how you can use it effectively in your Arduino projects.
Sending data from Arduino to the Serial Monitor
To send data from your Arduino board to the Serial Monitor, you can use the Serial.print()
or Serial.println()
functions. The difference between the two is that Serial.println()
automatically appends a newline character at the end of the data, moving the cursor to the next line.
Here’s an example that sends sensor readings to the Serial Monitor:
int sensorPin = A0;
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue = analogRead(sensorPin);
Serial.print("Sensor value: ");
Serial.println(sensorValue);
delay(1000);
}
In this example, the Arduino board reads the value from an analog sensor connected to pin A0 and sends it to the Serial Monitor every second. The output will look something like this:
Sensor value: 512
Sensor value: 520
Sensor value: 508
Receiving data from the Serial Monitor
You can also send data from the Serial Monitor to your Arduino board. This is useful for controlling your Arduino project or sending configuration parameters. To receive data in your Arduino sketch, you can use the Serial.available()
and Serial.read()
functions.
Here’s an example that receives data from the Serial Monitor and controls an LED:
int ledPin = 13;
void setup() {
Serial.begin(9600);
pinMode(ledPin, OUTPUT);
}
void loop() {
if (Serial.available() > 0) {
char incomingData = Serial.read();
if (incomingData == '1') {
digitalWrite(ledPin, HIGH);
Serial.println("LED turned ON");
} else if (incomingData == '0') {
digitalWrite(ledPin, LOW);
Serial.println("LED turned OFF");
}
}
}
In this example, the Arduino board listens for incoming data from the Serial Monitor. When a character is received, it checks if it is ‘1’ or ‘0’. If it is ‘1’, the LED connected to pin 13 is turned on, and a message is sent back to the Serial Monitor. If it is ‘0’, the LED is turned off, and a corresponding message is sent.
To send data from the Serial Monitor, simply type the character in the input field at the bottom of the Serial Monitor window and press Enter or click the “Send” button.
Formatting data in the Serial Monitor
When sending data to the Serial Monitor, you can format it to make it more readable. Here are a few formatting techniques:
- Use the
Serial.print()
function to send data without a newline character. This allows you to send multiple values on the same line. - Use the
Serial.println()
function to send data with a newline character, moving the cursor to the next line. - Use the
Serial.print()
function with a format specifier to send formatted data. For example:
cpp
float temperature = 25.5;
Serial.print("Temperature: ");
Serial.print(temperature, 2); // Print with 2 decimal places
Serial.println(" °C");
Output:
Temperature: 25.50 °C
- Use the
Serial.write()
function to send binary data or characters.
Advanced Serial Monitor Techniques
Plotting data in the Serial Plotter
The Arduino IDE also includes a Serial Plotter tool that allows you to visualize data sent from your Arduino board as a graph. To use the Serial Plotter, your sketch should send data in a specific format: each data point should be a numeric value, and multiple data points should be separated by a comma or a space.
Here’s an example that sends data suitable for plotting:
int sensorPin = A0;
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue = analogRead(sensorPin);
Serial.println(sensorValue);
delay(100);
}
To open the Serial Plotter, select “Tools” > “Serial Plotter” from the Arduino IDE menu. You will see a real-time graph of the sensor values.
Using multiple Serial ports
Some Arduino boards, such as the Arduino Mega, have multiple hardware Serial ports (e.g., Serial1, Serial2, etc.). You can use these additional Serial ports to communicate with other devices or modules while still using the main Serial port for communication with the computer.
To use multiple Serial ports, you need to initialize them in the setup()
function and use the corresponding Serial object for communication. For example:
void setup() {
Serial.begin(9600); // Initialize main Serial port
Serial1.begin(9600); // Initialize Serial1 port
}
void loop() {
if (Serial1.available()) {
char data = Serial1.read();
Serial.print("Received from Serial1: ");
Serial.println(data);
}
}
In this example, the Arduino board listens for data on the Serial1 port and sends it to the main Serial port for display in the Serial Monitor.
Debugging with the Serial Monitor
The Serial Monitor is a valuable tool for debugging your Arduino sketches. You can use it to print debug messages, variable values, and other relevant information to help identify and fix issues in your code.
Here are a few debugging techniques using the Serial Monitor:
-
Print debug messages: Use
Serial.print()
orSerial.println()
to print messages at critical points in your code to track the flow of execution and identify where issues may occur. -
Print variable values: Print the values of variables at different stages of your program to ensure they are being assigned and modified correctly.
-
Use conditional debugging: Use conditional statements (e.g.,
if
statements) to print debug information only when certain conditions are met, such as when an error occurs or a specific value is encountered. -
Print millis(): Use the
millis()
function to print timestamps along with your debug messages. This can help you determine the timing and duration of specific events in your program.
Remember to remove or comment out the debug statements once you have finished debugging your code to avoid unnecessary serial communication overhead.
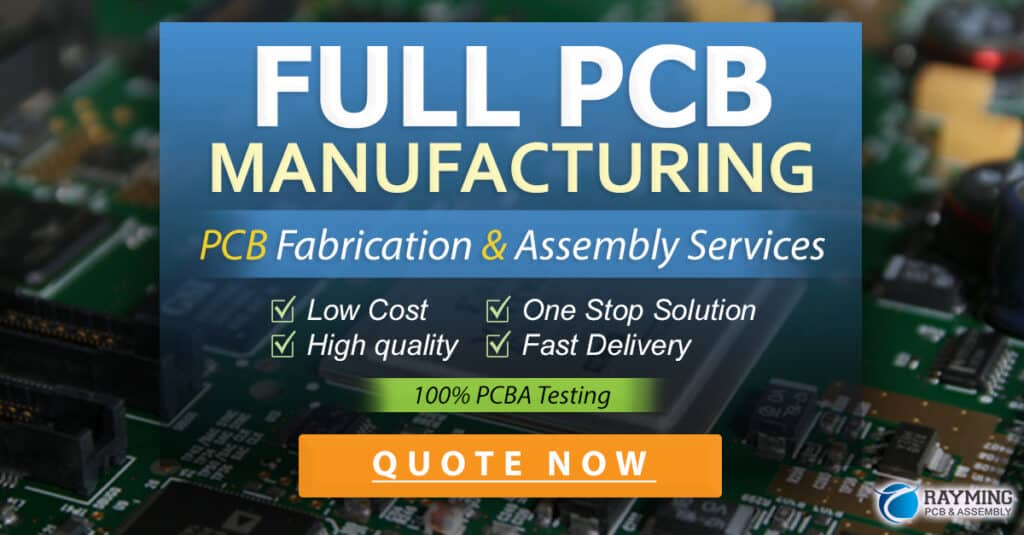
FAQs
-
What is the default baud rate for the Arduino Serial Monitor?
The default baud rate for the Arduino Serial Monitor is 9600 bps (bits per second). However, you can change the baud rate in your Arduino sketch using theSerial.begin()
function and selecting the corresponding baud rate in the Serial Monitor dropdown menu. -
Can I use the Serial Monitor with a USB-to-TTL serial adapter?
Yes, you can use the Serial Monitor with a USB-to-TTL serial adapter. Connect the adapter to your computer and select the appropriate port in the Arduino IDE. Make sure the baud rate in your sketch matches the baud rate of the serial adapter. -
How do I clear the Serial Monitor output?
To clear the Serial Monitor output, click on the “Clear output” button in the top-right corner of the Serial Monitor window. Alternatively, you can close and reopen the Serial Monitor. -
What happens if I send data to the Arduino board faster than it can process?
If you send data to the Arduino board faster than it can process, some data may be lost. The Arduino board has a limited serial buffer size, and if the buffer fills up before the data is processed, the oldest data will be discarded. To avoid this, ensure that your Arduino sketch can handle the incoming data rate and implement appropriate flow control mechanisms if necessary. -
Can I use the Serial Monitor with other programming languages?
Yes, you can use the Serial Monitor with other programming languages that support serial communication. Many programming languages, such as Python, Java, and C#, have libraries or modules that allow you to establish serial communication with Arduino boards. You can use these libraries to send and receive data between your computer and the Arduino board, similar to how the Arduino Serial Monitor works.
Conclusion
The Arduino Serial Monitor is a powerful tool that enables seamless communication between your Arduino board and a computer. It provides a simple and effective way to debug your code, monitor sensor readings, and interact with your Arduino projects in real-time.
In this comprehensive guide, we covered the fundamentals of the Arduino Serial Monitor, including setting it up, sending and receiving data, formatting data, using the Serial Plotter, working with multiple Serial ports, and debugging techniques. By mastering these concepts, you can unlock the full potential of your Arduino projects and create more interactive and dynamic applications.
Remember to experiment, explore, and have fun with the Arduino Serial Monitor. It is an essential tool in your Arduino toolkit that will help you bring your ideas to life and take your projects to the next level.
Happy coding and happy tinkering with Arduino and the Serial Monitor!
No responses yet