Introduction to Arduino Byte
Arduino is an open-source electronics platform that has revolutionized the world of hobby electronics and prototyping. It provides an easy-to-use hardware and software environment for creating interactive projects. One of the fundamental data types in Arduino programming is the byte type. In this comprehensive guide, we will explore the Arduino byte type in detail, its characteristics, usage, and provide examples to help you understand and utilize it effectively in your Arduino projects.
What is a Byte?
In computer science, a byte is a unit of digital information that consists of 8 bits. It is the smallest addressable unit of memory in most computer systems. A byte can represent a single character, such as a letter, number, or symbol, or it can be used to store a small integer value.
In Arduino, the byte data type is an 8-bit unsigned number, meaning it can hold values ranging from 0 to 255. It is commonly used to store small amounts of data, such as sensor readings, digital pin states, or configuration settings.
Declaring and Initializing Byte Variables
To use a byte variable in your Arduino sketch, you need to declare it first. The syntax for declaring a byte variable is as follows:
byte variableName;
For example, to declare a byte variable named myByte
, you would write:
byte myByte;
You can also initialize a byte variable with a specific value when declaring it. The syntax for initializing a byte variable is:
byte variableName = value;
For instance, to declare and initialize a byte variable named ledPin
with a value of 13, you would write:
byte ledPin = 13;
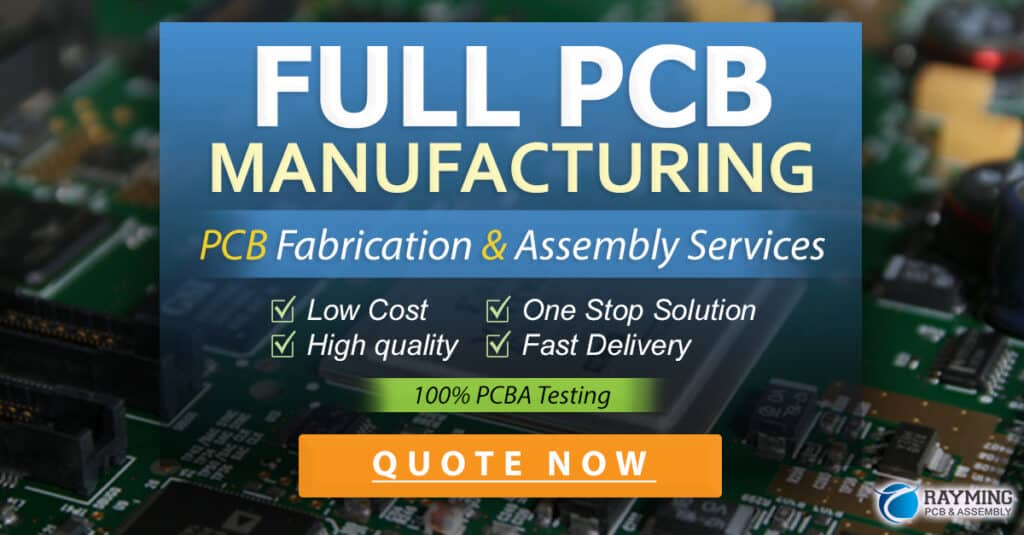
Byte Arithmetic and Operations
Arduino supports various arithmetic and bitwise operations on byte variables. Let’s explore some common operations you can perform with byte variables.
Arithmetic Operations
You can perform basic arithmetic operations on byte variables, such as addition, subtraction, multiplication, and division. Here are some examples:
byte a = 10;
byte b = 5;
byte sum = a + b; // sum = 15
byte difference = a - b; // difference = 5
byte product = a * b; // product = 50
byte quotient = a / b; // quotient = 2
Note that when performing arithmetic operations on byte variables, the result is also a byte. If the result exceeds the range of a byte (0 to 255), it will overflow, and the result will wrap around. For example:
byte a = 250;
byte b = 10;
byte sum = a + b; // sum = 4 (overflow occurred)
Bitwise Operations
Byte variables are often used for bitwise operations, which manipulate individual bits within the byte. Arduino provides several bitwise operators, such as AND (&
), OR (|
), XOR (^
), and NOT (~
). These operators are commonly used for tasks like setting or clearing specific bits, masking, and bit shifting.
Here are some examples of bitwise operations on byte variables:
byte a = 0b10101010; // Binary representation of 170
byte b = 0b11110000; // Binary representation of 240
byte andResult = a & b; // andResult = 0b10100000 (160)
byte orResult = a | b; // orResult = 0b11111010 (250)
byte xorResult = a ^ b; // xorResult = 0b01011010 (90)
byte notResult = ~a; // notResult = 0b01010101 (85)
Bitwise operations are particularly useful when working with registers, flags, or configurations where individual bits have specific meanings.
Using Byte Variables with Arduino Functions
Many Arduino functions and libraries use byte variables as parameters or return types. Let’s explore some common scenarios where byte variables are used in Arduino programming.
Digital I/O
When working with digital input/output pins, byte variables are often used to store pin numbers or states. For example:
byte ledPin = 13;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
digitalWrite(ledPin, HIGH);
delay(1000);
digitalWrite(ledPin, LOW);
delay(1000);
}
In this example, the ledPin
variable is declared as a byte and assigned the value 13, representing the pin number connected to an LED. The pinMode()
function is used to set the pin as an output, and the digitalWrite()
function is used to set the pin state to HIGH or LOW.
Serial Communication
When sending or receiving data over serial communication, byte variables are commonly used. For example:
void setup() {
Serial.begin(9600);
}
void loop() {
if (Serial.available() > 0) {
byte data = Serial.read();
// Process the received data
Serial.write(data);
}
}
In this example, the Serial.read()
function reads a single byte of data from the serial buffer and assigns it to the data
variable. The received data can then be processed or sent back over serial using the Serial.write()
function.
I2C and SPI Communication
When communicating with external devices using protocols like I2C or SPI, byte variables are often used to store device addresses, register addresses, or data values. For example, when using the Wire library for I2C communication:
#include <Wire.h>
byte deviceAddress = 0x68; // I2C device address
void setup() {
Wire.begin();
}
void loop() {
Wire.beginTransmission(deviceAddress);
Wire.write(0x00); // Register address
Wire.write(0x42); // Data to write
Wire.endTransmission();
}
In this example, the deviceAddress
variable is declared as a byte and assigned the I2C address of the external device. The Wire.write()
function is used to send bytes of data to the device, such as register addresses or data values.
Byte Arrays
In addition to single byte variables, Arduino also supports byte arrays, which are collections of byte elements. Byte arrays are useful for storing and manipulating larger amounts of data, such as buffers, sensor readings, or configuration settings.
To declare a byte array, you specify the array name followed by the number of elements in square brackets. For example:
byte myArray[5];
This declares a byte array named myArray
with a size of 5 elements. You can access individual elements of the array using the array index, starting from 0. For example:
myArray[0] = 10;
myArray[1] = 20;
byte value = myArray[2];
You can also initialize a byte array with specific values when declaring it:
byte myArray[] = {10, 20, 30, 40, 50};
This creates a byte array named myArray
and initializes it with the values 10, 20, 30, 40, and 50.
Byte arrays are commonly used in Arduino programming for tasks such as storing sensor readings, buffering data, or holding configuration settings.
Byte Type Conversion
In certain situations, you may need to convert between different data types in Arduino. Arduino provides built-in functions and operators for type conversion.
To convert a value to a byte, you can use the byte()
function. For example:
int myInt = 257;
byte myByte = byte(myInt); // myByte = 1 (truncated to fit in a byte)
In this example, the byte()
function is used to convert the integer value 257 to a byte. Since a byte can only hold values from 0 to 255, the result is truncated to 1.
You can also use the assignment operator to implicitly convert between compatible types. For example:
int myInt = 100;
byte myByte = myInt; // Implicit conversion from int to byte
In this case, the integer value 100 is implicitly converted to a byte and assigned to myByte
.
It’s important to be aware of the range limitations when converting between types to avoid unexpected behavior or loss of data.
Best Practices and Tips
Here are some best practices and tips to keep in mind when working with byte variables in Arduino:
- Use descriptive names for your byte variables to enhance code readability and maintainability.
- Be mindful of the range limitations of the byte type (0 to 255) to avoid overflow or unexpected behavior.
- Use appropriate data types based on the range and nature of the data you are working with.
- When performing bitwise operations, use parentheses to ensure the desired order of operations.
- Use constants or enums to represent specific byte values or bit patterns, making your code more readable and maintainable.
- When working with byte arrays, ensure that you stay within the bounds of the array to avoid accessing invalid memory locations.
- Consider using the
PROGMEM
keyword when storing large amounts of constant data in byte arrays to optimize memory usage.
Frequently Asked Questions (FAQ)
-
What is the range of values that a byte variable can hold in Arduino?
A byte variable in Arduino can hold unsigned integer values ranging from 0 to 255. -
Can I use negative numbers with byte variables in Arduino?
No, byte variables in Arduino are unsigned, meaning they can only hold non-negative values. If you need to work with negative numbers, you should use a signed data type such asint
orfloat
. -
What happens if I assign a value outside the range of a byte variable?
If you assign a value outside the range of a byte variable (0 to 255), the value will be truncated to fit within the range. For example, if you assign 260 to a byte variable, it will be truncated to 4 (260 – 256). -
Can I perform arithmetic operations on byte variables?
Yes, you can perform arithmetic operations on byte variables, such as addition, subtraction, multiplication, and division. However, keep in mind that the result of the operation will also be a byte, and any overflow will result in wrapping around. -
How do I convert a byte variable to another data type in Arduino?
Arduino provides built-in functions for type conversion. To convert a byte variable to another data type, you can use the corresponding type casting function. For example, to convert a byte to an integer, you can use theint()
function:int myInt = int(myByte);
.
Conclusion
In this comprehensive guide, we explored the Arduino byte type in detail. We covered the basics of bytes, declaring and initializing byte variables, performing arithmetic and bitwise operations, using byte variables with Arduino functions, working with byte arrays, and type conversion. We also discussed best practices and provided answers to frequently asked questions.
Understanding and utilizing the byte type effectively is crucial in Arduino programming, especially when dealing with low-level operations, sensor data, or communication protocols. By following the concepts and examples presented in this guide, you can confidently work with byte variables in your Arduino projects and create efficient and reliable code.
Remember to keep the range limitations and best practices in mind when using byte variables, and don’t hesitate to refer back to this guide as a resource whenever needed. Happy coding with Arduino and byte variables!
No responses yet