What is CircuitPython?
CircuitPython is a fork of MicroPython, a Python implementation optimized for microcontrollers. It was created by Adafruit Industries to provide a more accessible and beginner-friendly way to program microcontrollers. CircuitPython is open-source and runs on a variety of microcontroller boards, including many of Adafruit’s own boards.
Advantages of CircuitPython
-
Easy to learn: CircuitPython is based on Python, a widely-used and beginner-friendly programming language. If you’re familiar with Python, you’ll find CircuitPython easy to pick up.
-
No IDE required: CircuitPython doesn’t require an Integrated Development Environment (IDE). You can write your code in any text editor and save it to the microcontroller’s file system.
-
Serial console: CircuitPython provides a built-in serial console, which allows you to interact with your code and debug it easily using a simple serial terminal program.
-
Extensive library support: Adafruit provides a wide range of libraries for CircuitPython, making it easy to interact with various sensors, displays, and other peripherals.
-
Cross-platform: CircuitPython runs on Windows, macOS, and Linux, making it accessible to developers on any platform.
Getting Started with CircuitPython
To start using CircuitPython, you’ll need a compatible microcontroller board and a USB cable to connect it to your computer.
Compatible Boards
Several microcontroller boards support CircuitPython, including:
Board | Microcontroller | Flash Memory |
---|---|---|
Adafruit Circuit Playground Express | ATSAMD21G18 ARM Cortex M0+ | 256KB |
Adafruit Feather M0 Express | ATSAMD21G18 ARM Cortex M0+ | 256KB |
Adafruit Feather M4 Express | ATSAMD51J19 ARM Cortex M4 | 512KB |
Adafruit ItsyBitsy M0 Express | ATSAMD21G18 ARM Cortex M0+ | 256KB |
Adafruit ItsyBitsy M4 Express | ATSAMD51G19A ARM Cortex M4 | 512KB |
Installing CircuitPython
-
Download the latest version of CircuitPython for your board from the CircuitPython downloads page.
-
Connect your board to your computer using a USB cable.
-
Double-click the reset button on your board to enter bootloader mode. The board will appear as a removable drive on your computer.
-
Drag and drop the downloaded CircuitPython UF2 file onto the removable drive. The board will reset and reappear as a new drive named CIRCUITPY.
Creating Your First CircuitPython Program
-
Open your favorite text editor and create a new file named
code.py
in the CIRCUITPY drive. -
Add the following code to your
code.py
file:
import board
import digitalio
import time
led = digitalio.DigitalInOut(board.D13)
led.direction = digitalio.Direction.OUTPUT
while True:
led.value = True
time.sleep(0.5)
led.value = False
time.sleep(0.5)
- Save the file. The board will automatically restart and run your code, blinking the built-in LED.
CircuitPython Libraries
Adafruit provides a wide range of libraries for CircuitPython, which make it easy to interact with various sensors, displays, and other peripherals. Some popular libraries include:
- Adafruit_CircuitPython_BusDevice: Provides helper classes for I2C and SPI communication.
- Adafruit_CircuitPython_NeoPixel: Allows you to control NeoPixel LED strips and matrices.
- Adafruit_CircuitPython_DHT: Enables reading temperature and humidity data from DHT11, DHT22, and AM2302 sensors.
- Adafruit_CircuitPython_Display_Text: Provides classes for displaying text on various displays, such as OLED and LCD screens.
To use a library in your CircuitPython project:
-
Download the library bundle from the CircuitPython libraries page.
-
Extract the downloaded bundle and locate the library you want to use.
-
Copy the library folder to the
lib
directory on your CIRCUITPY drive. -
Import the library in your
code.py
file and start using it in your project.
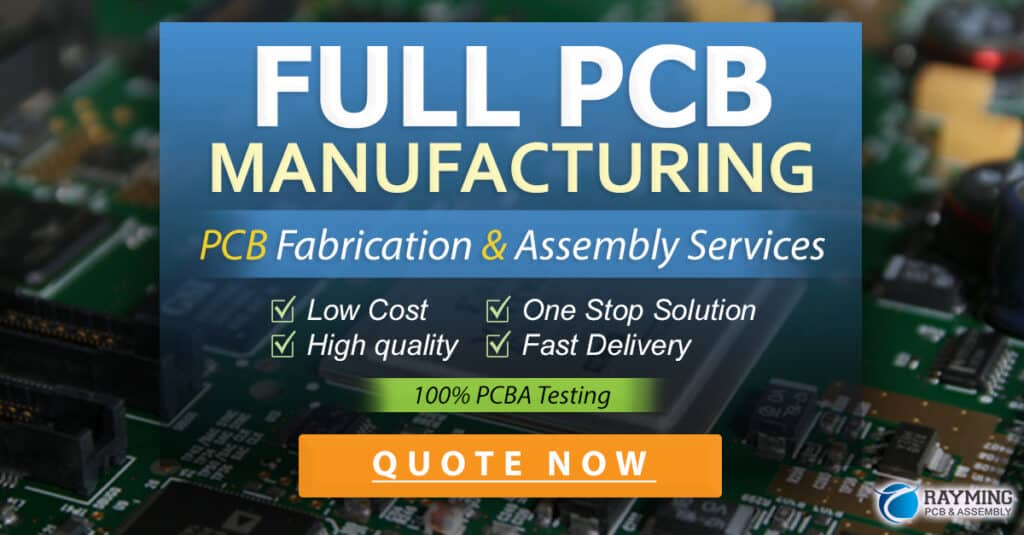
Example Projects
Here are a few example projects to help you get started with CircuitPython:
Project 1: Temperature and Humidity Monitoring
In this project, you’ll use a DHT22 sensor to monitor temperature and humidity and display the readings on an OLED screen.
Components needed:
– Adafruit Feather M0 Express
– DHT22 sensor
– 128×32 OLED display
– Breadboard and jumper wires
Code:
import time
import board
import adafruit_dht
import displayio
import terminalio
from adafruit_display_text import label
# Initialize the DHT22 sensor
dht = adafruit_dht.DHT22(board.D6)
# Initialize the OLED display
display = board.DISPLAY
font = terminalio.FONT
while True:
try:
# Read temperature and humidity from the DHT22 sensor
temperature = dht.temperature
humidity = dht.humidity
# Create the display text
text = f"Temp: {temperature:.1f}C\nHumidity: {humidity:.1f}%"
text_area = label.Label(font, text=text, color=0xFFFFFF, x=0, y=0)
display.show(text_area)
except RuntimeError as e:
# Handle sensor reading errors
print(f"Reading from DHT failed: {e.args}")
time.sleep(2) # Wait for 2 seconds before taking the next reading
Project 2: NeoPixel Mood Light
In this project, you’ll create a mood light using a NeoPixel LED strip and a potentiometer to control the color.
Components needed:
– Adafruit Feather M0 Express
– NeoPixel LED strip
– 10K potentiometer
– Breadboard and jumper wires
Code:
import time
import board
import analogio
import neopixel
# Initialize the NeoPixel strip
pixels = neopixel.NeoPixel(board.D6, 10, brightness=0.2)
# Initialize the potentiometer
potentiometer = analogio.AnalogIn(board.A0)
def get_color(value):
# Map potentiometer value to RGB color
if value < 16000:
return (value // 256, 0, 0) # Red to Yellow
elif value < 32000:
return (64, (value - 16000) // 256, 0) # Yellow to Green
elif value < 48000:
return (0, 64, (value - 32000) // 256) # Green to Blue
else:
return (0, 0, 64) # Blue
while True:
# Read the potentiometer value
value = potentiometer.value
# Set the NeoPixel color based on the potentiometer value
color = get_color(value)
pixels.fill(color)
time.sleep(0.1) # Add a short delay to avoid flickering
Advanced Topics
As you become more comfortable with CircuitPython, you may want to explore some advanced topics:
-
Interfacing with I2C and SPI devices: CircuitPython makes it easy to communicate with I2C and SPI devices using the
busio
module and device-specific libraries. -
Writing custom libraries: If you can’t find a library for your specific needs, you can write your own CircuitPython library to encapsulate the functionality you require.
-
Bluetooth Low Energy (BLE): Some CircuitPython-compatible boards, like the Adafruit Feather nRF52840 Express, support BLE communication, allowing you to create wireless projects.
-
CircuitPython and IoT: You can use CircuitPython to create Internet of Things (IoT) projects by connecting your microcontroller to the internet using Wi-Fi or Ethernet modules.
Frequently Asked Questions (FAQ)
-
Can I use CircuitPython with non-Adafruit boards?
While CircuitPython was created by Adafruit and is primarily supported on Adafruit boards, it can be used with other compatible microcontroller boards. However, support and documentation for non-Adafruit boards may be limited. -
Is CircuitPython compatible with all Python libraries?
CircuitPython is a subset of Python optimized for microcontrollers, so not all Python libraries will work with CircuitPython. However, Adafruit provides a wide range of CircuitPython-specific libraries that cover most common use cases. -
Can I use CircuitPython for commercial projects?
Yes, you can use CircuitPython for commercial projects. CircuitPython is released under the MIT license, which allows for commercial use, modification, and distribution. -
How do I troubleshoot CircuitPython issues?
If you encounter issues with your CircuitPython project, you can: - Check the serial console for error messages.
- Ensure you’re using the latest version of CircuitPython and libraries.
- Consult the CircuitPython documentation and forums for help.
-
Use the
print()
function to output debug information in your code. -
Can I contribute to the development of CircuitPython?
Yes, CircuitPython is an open-source project, and contributions from the community are welcome. You can contribute by submitting bug reports, feature requests, or pull requests on the CircuitPython GitHub repository.
Conclusion
CircuitPython is a powerful and user-friendly tool for programming microcontrollers, making it easier for beginners and experienced developers to create projects quickly. With its growing community, extensive library support, and compatibility with a wide range of boards, CircuitPython is an excellent choice for anyone looking to get started with microcontroller programming or develop more advanced projects.
No responses yet