What are Data Types in Arduino?
In Arduino programming, data types are used to define the type and size of data that can be stored in a variable. A variable is a container that holds a value, and the data type determines the kind of value it can hold, such as an integer, a floating-point number, a character, or a boolean value.
Choosing the appropriate data type is crucial for efficient memory usage and ensuring that your program works as intended. Arduino offers several built-in data types, each with its own characteristics and limitations.
Integer Data Types
Integer data types are used to store whole numbers, both positive and negative. Arduino provides several integer data types with different ranges and sizes.
int
The int
data type is a signed 16-bit integer, which means it can store values from -32,768 to 32,767. It occupies 2 bytes of memory.
Example:
int myNumber = 42;
unsigned int
The unsigned int
data type is an unsigned 16-bit integer, which means it can store values from 0 to 65,535. It also occupies 2 bytes of memory.
Example:
unsigned int myPositiveNumber = 65000;
long
The long
data type is a signed 32-bit integer, which means it can store values from -2,147,483,648 to 2,147,483,647. It occupies 4 bytes of memory.
Example:
long myLargeNumber = 2000000000;
unsigned long
The unsigned long
data type is an unsigned 32-bit integer, which means it can store values from 0 to 4,294,967,295. It also occupies 4 bytes of memory.
Example:
unsigned long myLargePositiveNumber = 4000000000;
short
The short
data type is a signed 16-bit integer, which means it can store values from -32,768 to 32,767. It occupies 2 bytes of memory, just like the int
data type.
Example:
short mySmallNumber = -10000;
unsigned short
The unsigned short
data type is an unsigned 16-bit integer, which means it can store values from 0 to 65,535. It also occupies 2 bytes of memory.
Example:
unsigned short mySmallPositiveNumber = 60000;
Here’s a table summarizing the integer data types in Arduino:
Data Type | Size (bytes) | Range |
---|---|---|
int | 2 | -32,768 to 32,767 |
unsigned int | 2 | 0 to 65,535 |
long | 4 | -2,147,483,648 to 2,147,483,647 |
unsigned long | 4 | 0 to 4,294,967,295 |
short | 2 | -32,768 to 32,767 |
unsigned short | 2 | 0 to 65,535 |
Floating-Point Data Types
Floating-point data types are used to store decimal numbers. Arduino provides two floating-point data types: float
and double
.
float
The float
data type is a single-precision floating-point number, which means it can store decimal values with a precision of about 7 digits. It occupies 4 bytes of memory.
Example:
float myFloat = 3.14159;
double
The double
data type is a double-precision floating-point number, which means it can store decimal values with a precision of about 15 digits. It occupies 8 bytes of memory.
Example:
double myDouble = 3.141592653589793;
Here’s a table summarizing the floating-point data types in Arduino:
Data Type | Size (bytes) | Precision |
---|---|---|
float | 4 | ~7 digits |
double | 8 | ~15 digits |
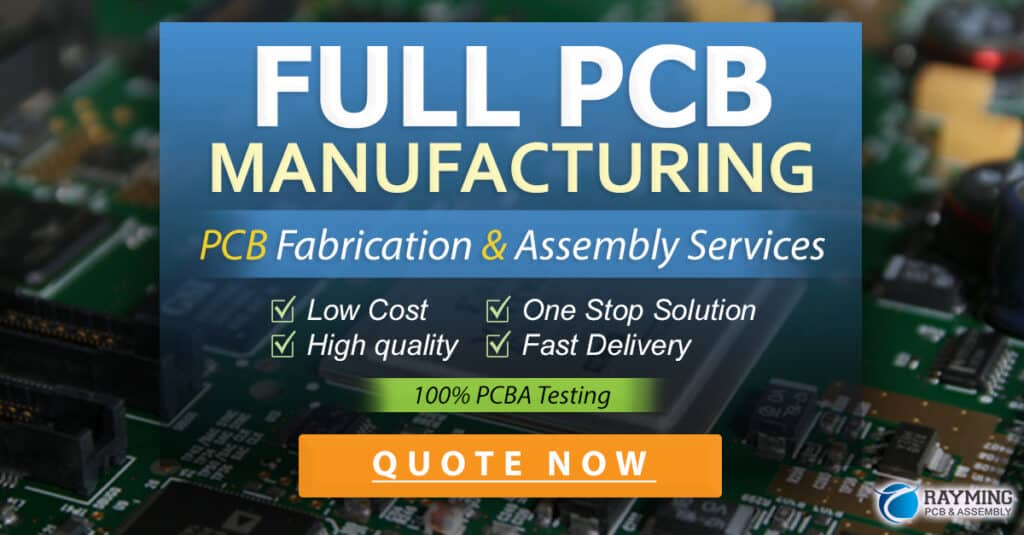
Boolean Data Type
The boolean data type is used to store logical values, which can be either true
or false
. It occupies 1 byte of memory.
Example:
boolean myBoolean = true;
Character Data Type
The character data type is used to store a single character. It is declared using the keyword char
and occupies 1 byte of memory.
Example:
char myChar = 'A';
String Data Type
The string data type is used to store a sequence of characters. It is not a built-in data type in Arduino, but it is provided by the Arduino String library. The String data type can be used to store text and manipulate it easily.
Example:
String myString = "Hello, Arduino!";
Void Data Type
The void data type is used to indicate that a function does not return any value. It is commonly used in function declarations when the function performs an action but does not need to return a value.
Example:
void setup() {
// Setup code here
}
void loop() {
// Loop code here
}
Array Data Type
An array is a collection of elements of the same data type, stored in contiguous memory locations. Arrays in Arduino can be declared using any of the data types mentioned above.
Example:
int myArray[5] = {10, 20, 30, 40, 50};
Choosing the Right Data Type
When choosing a data type for your variables, consider the following factors:
- Range: Ensure that the data type can accommodate the range of values you expect to store.
- Precision: For floating-point numbers, choose between
float
anddouble
based on the required precision. - Memory Usage: Use the smallest data type that can handle your values to optimize memory usage.
- Consistency: Try to use the same data type for related variables to maintain consistency and avoid type conversion issues.
FAQ
Q1: What is the difference between int
and long
data types in Arduino?
A1: The int
data type is a 16-bit signed integer, while long
is a 32-bit signed integer. int
can store values from -32,768 to 32,767, while long
can store values from -2,147,483,648 to 2,147,483,647.
Q2: When should I use the unsigned
versions of integer data types?
A2: Use the unsigned
versions when you are certain that the values stored will always be non-negative. This allows you to extend the positive range of the data type.
Q3: What is the size of the boolean
data type in Arduino?
A3: The boolean
data type occupies 1 byte of memory in Arduino.
Q4: Can I store decimal numbers using the int
data type?
A4: No, the int
data type is used to store whole numbers only. For decimal numbers, use the float
or double
data types.
Q5: What is the purpose of the void
data type in Arduino?
A5: The void
data type is used to indicate that a function does not return any value. It is commonly used in function declarations when the function performs an action but does not need to return a value.
Conclusion
Understanding Arduino Data Types is essential for effective programming and memory management. By choosing the appropriate data type for your variables, you can ensure that your program runs efficiently and accurately. This comprehensive guide has covered the various integer, floating-point, boolean, character, string, void, and array data types available in Arduino.
Remember to consider factors such as range, precision, memory usage, and consistency when selecting data types for your variables. With this knowledge, you’ll be well-equipped to tackle any Arduino project that comes your way.
Happy coding!
No responses yet