Introduction to Arduino Essentials
Arduino is an open-source electronics platform that has revolutionized the way people learn about and create electronic projects. At the heart of the Arduino ecosystem is the Arduino circuit board, a microcontroller-based board that allows users to easily program and control various electronic components. In this article, we will explore the essential things you need to know about Arduino circuit boards to get started with your own projects.
What is an Arduino Circuit Board?
An Arduino circuit board is a printed circuit board (PCB) that contains a microcontroller, along with other electronic components that enable it to function as a programmable device. The microcontroller is the brain of the board, and it can be programmed using the Arduino Integrated Development Environment (IDE) to control various inputs and outputs, such as sensors, motors, and lights.
Component | Description |
---|---|
Microcontroller | The brain of the Arduino board, programmable using the Arduino IDE |
Power Supply | Provides power to the board, typically via USB or external power source |
Input/Output Pins | Used to connect various electronic components to the board |
USB Interface | Allows the board to be connected to a computer for programming and power |
Reset Button | Resets the microcontroller to its default state |
Types of Arduino Boards
There are several different types of Arduino boards available, each with its own unique features and capabilities. Some of the most popular Arduino boards include:
Arduino Uno
The Arduino Uno is the most popular and widely used Arduino board. It is based on the ATmega328P microcontroller and has 14 digital input/output pins, 6 analog inputs, and a USB interface for programming and power.
Arduino Nano
The Arduino Nano is a compact version of the Arduino Uno, with a smaller form factor and fewer input/output pins. It is ideal for projects where space is limited.
Arduino Mega
The Arduino Mega is a larger and more powerful version of the Arduino Uno, with 54 digital input/output pins, 16 analog inputs, and 4 hardware serial ports. It is ideal for more complex projects that require more processing power and input/output capabilities.
Getting Started with Arduino
To get started with Arduino, you will need the following:
- An Arduino board
- A computer with the Arduino IDE installed
- A USB cable to connect the board to the computer
- Basic electronic components, such as LEDs, resistors, and sensors
Installing the Arduino IDE
The Arduino IDE is a free, open-source software that allows you to write, compile, and upload code to your Arduino board. To install the Arduino IDE, follow these steps:
- Go to the Arduino website (https://www.arduino.cc/en/software) and download the latest version of the Arduino IDE for your operating system.
- Run the installer and follow the prompts to complete the installation process.
- Launch the Arduino IDE and select your Arduino board from the “Tools” menu.
Writing and Uploading Code
Once you have the Arduino IDE installed and your board selected, you can begin writing and uploading code to your board. The Arduino IDE uses a simplified version of the C++ programming language, with built-in functions and libraries that make it easy to control various electronic components.
To upload code to your Arduino board, follow these steps:
- Connect your Arduino board to your computer using a USB cable.
- Open the Arduino IDE and create a new sketch (file > new).
- Write your code in the sketch window.
- Select your Arduino board and serial port from the “Tools” menu.
- Click the “Upload” button to compile and upload your code to the board.
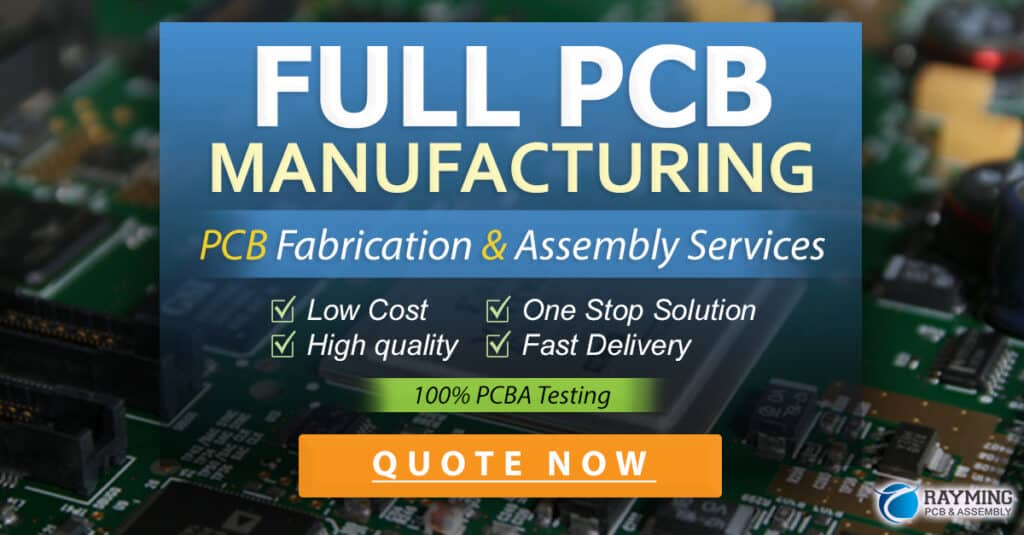
Examples of Arduino Projects
Arduino can be used to create a wide variety of electronic projects, from simple blinking LED circuits to complex robotics and automation systems. Here are a few examples of popular Arduino projects:
Blinking LED
A blinking LED is a simple project that demonstrates the basic functionality of the Arduino board. To create a blinking LED circuit, you will need:
- An Arduino board
- An LED
- A 220-ohm resistor
- Jumper wires
Connect the LED and resistor to the Arduino board as shown in the diagram below:
220Ω
Arduino -----/\/\/\------->|---------- GND
Pin 13
Then, upload the following code to the board:
void setup() {
pinMode(13, OUTPUT);
}
void loop() {
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(13, LOW);
delay(1000);
}
This code will cause the LED to blink on and off every second.
Temperature and Humidity Sensor
A temperature and humidity sensor is a more advanced project that demonstrates how to use sensors with the Arduino board. To create a temperature and humidity sensor, you will need:
- An Arduino board
- A DHT11 or DHT22 temperature and humidity sensor
- Jumper wires
Connect the sensor to the Arduino board as shown in the diagram below:
DHT11/DHT22
___
| |
| |
|___|
| |
| |
| | Arduino
S | | + - -----
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | | |
| | | | | |GND|
Then, upload the following code to the board:
#include <DHT.h>
#define DHTPIN 2
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
delay(2000);
float h = dht.readHumidity();
float t = dht.readTemperature();
if (isnan(h) || isnan(t)) {
Serial.println("Failed to read from DHT sensor!");
return;
}
Serial.print("Humidity: ");
Serial.print(h);
Serial.print(" %\t");
Serial.print("Temperature: ");
Serial.print(t);
Serial.println(" *C");
}
This code will read the temperature and humidity from the sensor every 2 seconds and print the values to the serial monitor.
Common Issues and Troubleshooting
While working with Arduino boards, you may encounter various issues and errors. Here are some common issues and their solutions:
Issue | Solution |
---|---|
Board not detected by computer | Make sure the board is properly connected to the computer via USB and that the correct board and port are selected in the Arduino IDE. |
Code not uploading to board | Check that the correct board and port are selected in the Arduino IDE, and that the code compiles without errors. |
Components not working as expected | Double-check the wiring and connections, and ensure that the correct pins are being used in the code. |
If you encounter an issue that you cannot resolve on your own, there are many online resources and communities available to help, such as the official Arduino forums and Stack Overflow.
Frequently Asked Questions (FAQ)
-
What is the difference between Arduino and Raspberry Pi?
Arduino is a microcontroller-based platform designed for simple electronics projects, while Raspberry Pi is a single-board computer that runs a full operating system and is more suited for complex projects involving multimedia and networking. -
Can I use Arduino for commercial projects?
Yes, Arduino is an open-source platform, which means that you can use it for both personal and commercial projects without any licensing fees or restrictions. -
What programming languages can be used with Arduino?
Arduino uses a simplified version of the C++ programming language, but there are also libraries and tools available that allow you to use other languages, such as Python and MATLAB, with Arduino boards. -
How do I choose the right Arduino board for my project?
The choice of Arduino board depends on the specific requirements of your project, such as the number of input/output pins needed, the processing power required, and the form factor of the board. Consider factors such as cost, availability, and community support when making your decision. -
Can I power an Arduino board using batteries?
Yes, Arduino boards can be powered using batteries, either through the built-in power jack or by connecting the batteries directly to the VIN and GND pins. However, be sure to use batteries with the appropriate voltage and current ratings for your specific board and project.
Conclusion
Arduino circuit boards are a versatile and powerful tool for learning about and creating electronic projects. By understanding the basics of Arduino boards, programming, and troubleshooting, you can unlock a world of possibilities for your own projects. Whether you are a beginner or an experienced maker, Arduino offers a platform for creativity, innovation, and learning. So grab a board, start experimenting, and see what you can create!
No responses yet