Introduction to Remote-Control Toys
Remote-controlled toys have been a favorite among children and adults alike for decades. The ability to control a toy from a distance using a wireless remote adds an extra level of excitement and interactivity to playtime. In this article, we will explore the world of remote-control circuits and guide you through the process of building your own DIY toy car with wireless circuits.
What are Remote-Control Toys?
Remote-control toys are toys that can be controlled from a distance using a wireless remote. These toys come in various forms, such as cars, planes, boats, and even robots. The remote communicates with the toy using radio frequency (RF) signals, allowing the user to control the toy’s movements and actions.
History of Remote-Control Toys
The first remote-controlled toy was a boat developed by Nikola Tesla in 1898. Since then, the technology has evolved significantly, with the introduction of transistors and integrated circuits in the 1960s and 1970s, making remote-control toys more affordable and accessible to the general public.
Understanding the Basics of Remote-Control Circuits
Before we dive into building our own remote-control toy car, let’s first understand the basics of remote-control circuits.
Components of a Remote-Control Circuit
A typical remote-control circuit consists of the following components:
- Transmitter: The remote that sends the RF signals to the toy.
- Receiver: The component in the toy that receives the RF signals from the transmitter.
- Actuators: The components in the toy that convert the electrical signals into mechanical actions, such as motors for movement or servos for steering.
- Power Source: Batteries that power the transmitter and the toy.
How Remote-Control Circuits Work
The transmitter sends RF signals containing instructions for the toy’s actions. The receiver in the toy picks up these signals and converts them into electrical signals. These signals are then sent to the actuators, which convert them into mechanical actions, such as moving the toy forward or turning it left or right.
Building a DIY Remote-Control Toy Car
Now that we have a basic understanding of remote-control circuits, let’s build our own DIY toy car with wireless circuits.
Materials Required
To build our remote-control toy car, we will need the following materials:
- Arduino Uno board
- Arduino compatible RF transmitter and receiver modules
- L293D motor driver IC
- Two DC motors
- Battery holder and batteries
- Wheels and chassis for the car
- Jumper wires
- Breadboard
Step-by-Step Instructions
- Assemble the car chassis and attach the wheels and motors.
- Connect the L293D motor driver IC to the Arduino Uno board using jumper wires, following the datasheet for the IC.
- Connect the RF receiver module to the Arduino Uno board, following the module’s documentation.
- Upload the receiver code to the Arduino Uno board.
- Connect the RF transmitter module to another Arduino Uno board, which will act as the remote.
- Upload the transmitter code to the remote Arduino Uno board.
- Power the toy car using the battery holder and batteries.
- Test the remote-control toy car by pressing the buttons on the remote to control the car’s movements.
Circuit Diagram
Component | Pin Connection |
---|---|
L293D Motor Driver IC | EN1 – Arduino pin 6 |
IN1 – Arduino pin 7 | |
IN2 – Arduino pin 8 | |
IN3 – Arduino pin 9 | |
IN4 – Arduino pin 10 | |
EN2 – Arduino pin 11 | |
RF Receiver Module | VCC – Arduino 5V |
GND – Arduino GND | |
DATA – Arduino pin 12 | |
DC Motors | Motor 1 – L293D OUT1 and OUT2 |
Motor 2 – L293D OUT3 and OUT4 |
Code for the Receiver
#include <RH_ASK.h>
#include <SPI.h>
RH_ASK driver(2000, 12, 11, 0); // RF Receiver on pin 12
void setup() {
Serial.begin(9600);
if (!driver.init()) {
Serial.println("RF Receiver init failed");
}
}
void loop() {
uint8_t buf[RH_ASK_MAX_MESSAGE_LEN];
uint8_t buflen = sizeof(buf);
if (driver.recv(buf, &buflen)) {
if (buf[0] == 'F') {
// Move forward
digitalWrite(6, HIGH);
digitalWrite(7, HIGH);
digitalWrite(8, LOW);
digitalWrite(9, HIGH);
digitalWrite(10, LOW);
digitalWrite(11, HIGH);
} else if (buf[0] == 'B') {
// Move backward
digitalWrite(6, HIGH);
digitalWrite(7, LOW);
digitalWrite(8, HIGH);
digitalWrite(9, LOW);
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
} else if (buf[0] == 'L') {
// Turn left
digitalWrite(6, HIGH);
digitalWrite(7, HIGH);
digitalWrite(8, LOW);
digitalWrite(9, LOW);
digitalWrite(10, LOW);
digitalWrite(11, HIGH);
} else if (buf[0] == 'R') {
// Turn right
digitalWrite(6, HIGH);
digitalWrite(7, LOW);
digitalWrite(8, LOW);
digitalWrite(9, HIGH);
digitalWrite(10, LOW);
digitalWrite(11, HIGH);
} else if (buf[0] == 'S') {
// Stop
digitalWrite(6, LOW);
digitalWrite(7, LOW);
digitalWrite(8, LOW);
digitalWrite(9, LOW);
digitalWrite(10, LOW);
digitalWrite(11, LOW);
}
}
}
Code for the Transmitter
#include <RH_ASK.h>
#include <SPI.h>
RH_ASK driver(2000, 11, 12, 0); // RF Transmitter on pin 11
void setup() {
if (!driver.init()) {
Serial.println("RF Transmitter init failed");
}
}
void loop() {
if (digitalRead(2) == HIGH) {
// Move forward
const char *msg = "F";
driver.send((uint8_t *)msg, strlen(msg));
driver.waitPacketSent();
} else if (digitalRead(3) == HIGH) {
// Move backward
const char *msg = "B";
driver.send((uint8_t *)msg, strlen(msg));
driver.waitPacketSent();
} else if (digitalRead(4) == HIGH) {
// Turn left
const char *msg = "L";
driver.send((uint8_t *)msg, strlen(msg));
driver.waitPacketSent();
} else if (digitalRead(5) == HIGH) {
// Turn right
const char *msg = "R";
driver.send((uint8_t *)msg, strlen(msg));
driver.waitPacketSent();
} else {
// Stop
const char *msg = "S";
driver.send((uint8_t *)msg, strlen(msg));
driver.waitPacketSent();
}
}
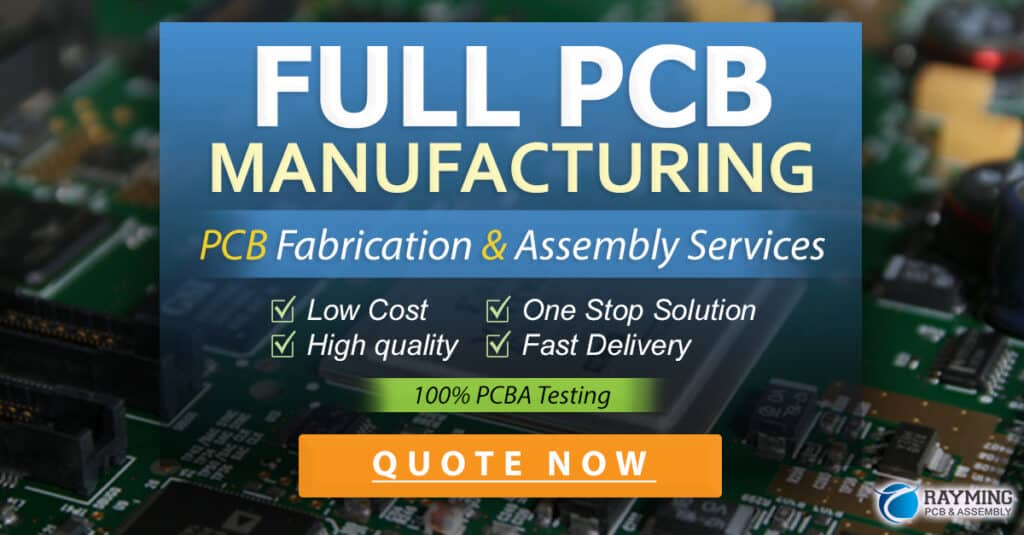
Customizing Your Remote-Control Toy Car
Now that you have built your basic remote-control toy car, you can customize it to your liking.
Adding Lights
You can add LED lights to your toy car to make it more visually appealing and to provide illumination in low-light conditions. To do this, simply connect the LEDs to the Arduino Uno board and control them using the appropriate code.
Adding Sound Effects
You can also add sound effects to your toy car to make it more engaging. This can be done using a piezo buzzer connected to the Arduino Uno board and controlled using the appropriate code.
Modifying the Chassis
You can modify the chassis of your toy car to improve its performance or to change its appearance. For example, you can add suspension to improve its handling on rough terrain or change the shape of the chassis to make it more aerodynamic.
Troubleshooting
If you encounter any issues while building or operating your remote-control toy car, here are some troubleshooting tips:
- Check all the connections to ensure they are secure and correct.
- Ensure that the batteries are fully charged and properly connected.
- Double-check the code to ensure it is correct and uploaded properly to the Arduino Uno boards.
- If the car is not responding to the remote, ensure that the RF transmitter and receiver modules are properly connected and configured.
Conclusion
Building your own remote-control toy car with wireless circuits is a fun and educational project that can be enjoyed by people of all ages. By following the step-by-step instructions and customizing your car to your liking, you can create a unique and engaging toy that will provide hours of entertainment.
Frequently Asked Questions (FAQ)
-
Can I use a different type of motor for my toy car?
Yes, you can use any type of DC motor that is compatible with the L293D motor driver IC. -
Can I use a different type of wireless communication module?
Yes, you can use any type of wireless communication module that is compatible with the Arduino Uno board, such as Bluetooth or Wi-Fi. -
Can I control multiple toy cars with one remote?
Yes, you can control multiple toy cars with one remote by assigning each car a unique identifier and modifying the code accordingly. -
How long will the batteries last?
The battery life will depend on the type and capacity of the batteries used, as well as the usage of the toy car. On average, a set of fully charged batteries should last for several hours of continuous use. -
Can I make my toy car faster?
Yes, you can make your toy car faster by using higher-speed motors or by modifying the gear ratio of the car’s drivetrain. However, be careful not to exceed the maximum speed rating of the motors or the capabilities of the Arduino Uno board.
No responses yet