Introduction to the L293D Motor Driver
The L293D is a popular motor driver IC that allows you to easily control the speed and direction of two DC motors simultaneously using an Arduino or other microcontroller. It acts as an interface between the low current control signals from the Arduino and the high current load of the motors.
Some key features of the L293D include:
– Dual H-bridge motor driver configuration
– Wide supply voltage range: 4.5V to 36V
– Output current capability of 600mA per channel
– Separate input logic supply
– Internal ESD protection
– Thermal shutdown capability
The L293D is a great choice for controlling small to medium sized DC motors in robotic projects, RC vehicles, and other applications requiring bidirectional motor control.
Understanding the L293D Pinout
Before connecting the L293D to your Arduino and DC motors, it’s important to understand the pin configuration and functions:
Pin | Function |
---|---|
1 (Enable 1,2) | Enables motor channel 1. Must be high for motors to run. PWM capable. |
2 (Input 1) | Control input for motor channel 1. Configures direction. |
3 (Output 1) | Output for motor channel 1. Connects to one motor terminal. |
4 (Ground) | Ground (0V) |
5 (Ground) | Ground (0V) |
6 (Output 2) | Output for motor channel 1. Connects to the other motor terminal. |
7 (Input 2) | Control input for motor channel 1. Configures direction. |
8 (Vcc2) | Power supply for motors, typically 6-12V. |
9 (Enable 3,4) | Enables motor channel 2. Must be high for motors to run. PWM capable. |
10 (Input 3) | Control input for motor channel 2. Configures direction. |
11 (Output 3) | Output for motor channel 2. Connects to one motor terminal. |
12 (Ground) | Ground (0V) |
13 (Ground) | Ground (0V) |
14 (Output 4) | Output for motor channel 2. Connects to the other motor terminal. |
15 (Input 4) | Control input for motor channel 2. Configures direction. |
16 (Vss) | Logic power supply, typically 5V from Arduino. |
Circuit Diagram for Connecting L293D to DC Motors and Arduino
Here is a circuit diagram illustrating how to wire up the L293D to two DC motors and an Arduino Uno:
[Insert circuit diagram image]
The key connections are:
– L293D Vcc2 (pin 8) to external 9V battery positive terminal
– L293D Vss and Enable pins (1,9,16) to Arduino 5V pin
– L293D Input pins (2,7,10,15) to Arduino digital output pins
– L293D motor output pins (3,6,11,14) to DC motor terminals
– L293D ground pins (4,5,12,13) and Arduino GND to common ground (battery negative)
– 100uF capacitor connected between Vcc2 and GND to suppress noise
Make sure to double check all your connections before powering up the circuit to avoid damage to the components.
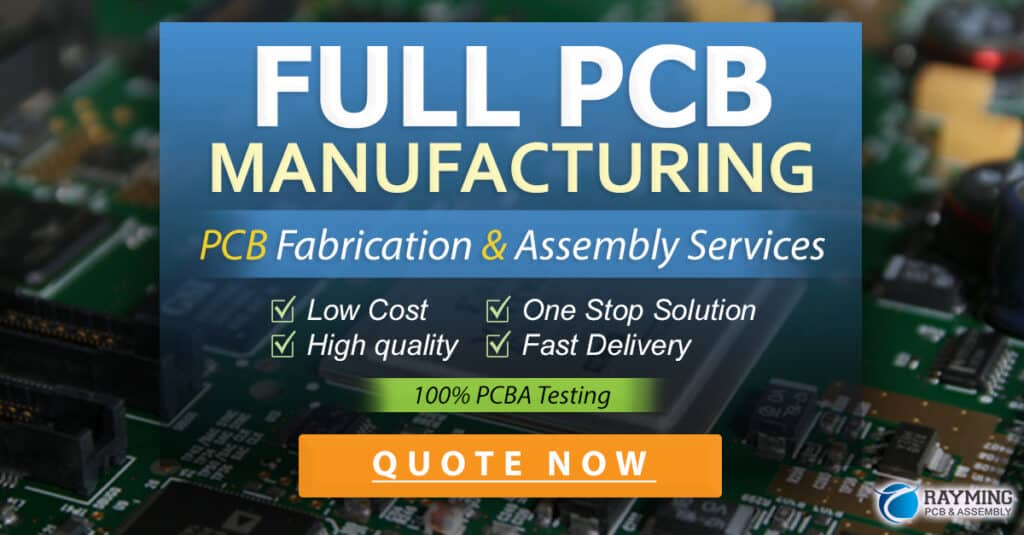
Arduino Code for Controlling DC Motors with L293D
Once you have the L293D wired up to your Arduino and DC motors, you can use the following Arduino code to control the speed and direction of the motors:
// Define motor control pins
#define ENA 5 // PWM control for motor A
#define IN1 6 // Direction control for motor A
#define IN2 7 // Direction control for motor A
#define ENB 10 // PWM control for motor B
#define IN3 8 // Direction control for motor B
#define IN4 9 // Direction control for motor B
void setup() {
// Set motor control pins as outputs
pinMode(ENA, OUTPUT);
pinMode(ENB, OUTPUT);
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
}
void loop() {
// Run both motors forward at 50% speed for 2 seconds
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
analogWrite(ENA, 127);
analogWrite(ENB, 127);
delay(2000);
// Stop motors for 1 second
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
delay(1000);
// Run motor A backward and motor B forward at 100% speed for 2 seconds
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
analogWrite(ENA, 255);
analogWrite(ENB, 255);
delay(2000);
// Stop motors for 1 second
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
delay(1000);
}
This code defines the motor control pin mappings and then demonstrates basic motor control within the loop() function. It runs both motors forward at 50% speed, stops them, runs motor A backward and motor B forward at full speed, then stops again, repeating indefinitely.
The analogWrite()
function is used to send PWM signals to the Enable pins to control the speed. You can pass it values between 0 (fully off) and 255 (fully on).
The direction is controlled by the states of the Input pins for each motor:
IN1 | IN2 | Result |
---|---|---|
LOW | LOW | Motor stop |
HIGH | LOW | Motor forward |
LOW | HIGH | Motor backward |
HIGH | HIGH | Motor stop |
Feel free to experiment with different speed and direction combinations to suit your specific application!
Tips for Powering Motors with L293D
Driving motors requires a decent amount of current, typically much more than the Arduino can supply. That’s why the L293D has a separate power input (Vcc2) for the motors. Some tips for powering your motors adequately:
- Use an external battery pack or power supply rated for at least the voltage and current your specific motors require under load. A common choice is a 9V battery.
- Place a large capacitor (e.g. 100uF) between Vcc2 and GND, physically close to the L293D. This helps suppress voltage spikes.
- Keep motor wires as short as possible to minimize losses and EMI.
- If the motors draw too much current, the L293D may overheat and go into thermal protection mode, shutting down. Consider adding a heatsink if needed.
- PWM control is great for varying speed, but at very low duty cycles, the motors may not have enough torque to start turning. Overcome this with gearing or by increasing the minimum duty cycle.
Setting Up a Simple Robotics Project with L293D and DC Motors
Now that you know how to control DC motors with the L293D and Arduino, let’s create a simple autonomous robot! Here’s what you’ll need:
- Arduino Uno and breadboard
- L293D motor driver IC
- 2 DC motors with wheels
- Robot chassis or platform
- Ultrasonic distance sensor (HC-SR04)
- Jumper wires
- 9V battery pack
Step 1: Assemble the robot chassis
Attach the DC motors and wheels to the chassis, making sure they’re mounted securely and aligned properly.
Step 2: Wire up the circuit
Connect the L293D to the Arduino, motors, and battery pack as shown in the previous circuit diagram. Add the ultrasonic sensor, with its Trigger and Echo pins connected to Arduino digital pins 2 and 3 respectively.
Step 3: Upload the code
Use the following Arduino sketch to make the robot autonomously drive around while avoiding obstacles:
#include <Servo.h>
// Define motor control pins
#define ENA 5
#define IN1 6
#define IN2 7
#define ENB 10
#define IN3 8
#define IN4 9
// Define ultrasonic sensor pins
#define TRIG_PIN 2
#define ECHO_PIN 3
Servo myservo; // create servo object to control a servo
void setup() {
// Set motor control pins as outputs
pinMode(ENA, OUTPUT);
pinMode(ENB, OUTPUT);
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
// Set ultrasonic sensor pins
pinMode(TRIG_PIN, OUTPUT);
pinMode(ECHO_PIN, INPUT);
myservo.attach(11); // attaches the servo on pin 11 to the servo object
}
void loop() {
int distance = measureDistance();
if (distance > 20) { // If distance > 20cm
// Move forward
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
analogWrite(ENA, 200);
analogWrite(ENB, 200);
} else { // If distance <= 20cm
// Stop
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
// Look left
myservo.write(180);
delay(500);
int leftDistance = measureDistance();
// Look right
myservo.write(0);
delay(1000);
int rightDistance = measureDistance();
// Decide which direction to turn
if (leftDistance > rightDistance) {
// Turn left
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
analogWrite(ENA, 200);
analogWrite(ENB, 200);
} else {
// Turn right
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
analogWrite(ENA, 200);
analogWrite(ENB, 200);
}
delay(500);
}
}
int measureDistance() {
// Send a 10us pulse to the TRIG pin
digitalWrite(TRIG_PIN, LOW);
delayMicroseconds(2);
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
// Measure the duration of the pulse on the ECHO pin
int duration = pulseIn(ECHO_PIN, HIGH);
// Calculate the distance in cm
int distance = duration * 0.034 / 2;
return distance;
}
This code uses the ultrasonic sensor to continuously measure the distance in front of the robot. If the distance is greater than 20cm, it moves forward. If the distance is less than or equal to 20cm, it stops, looks left and right to measure distances, then turns in the direction with more clearance. The measureDistance()
function sends a pulse to the ultrasonic sensor and calculates the distance based on the pulse duration.
Step 4: Power up and let it roam!
Turn on the battery pack and watch your robot navigate around autonomously, avoiding obstacles in its path. You can experiment with different distance thresholds, motor speeds, and turning angles to customize its behavior.
Troubleshooting L293D Motor Driver Issues
If you’re having trouble getting your motors to run properly with the L293D, here are some things to check:
- Make sure you have adequate power supplied to the Vcc2 pin, and that your Arduino is powered separately from the USB or an external power supply. Insufficient voltage or current can cause the motors to stall or behave erratically.
- Double-check your wiring against the circuit diagram, making sure all connections are secure and correct. A loose or misplaced wire can prevent the motors from running.
- Verify that your code is correct, with the proper pin mappings and control logic. Upload a simple test sketch to isolate any hardware issues.
- Check the datasheet for your specific DC motors and make sure they’re compatible with the L293D in terms of voltage and current requirements. Overpowering or underpowering the motors can damage them or the driver IC.
- If the L293D seems to be overheating, make sure you’re not exceeding its maximum current rating of 600mA per channel. Add a heatsink if necessary, or use a higher-powered motor driver like the L298N.
- If the motors are running but seem weak or inconsistent, make sure your power supply can deliver enough current under load. Consider using a higher voltage battery pack or a DC power supply with sufficient amperage.
- Noise from the motors can sometimes interfere with the Arduino’s operation. Make sure your Vcc2 supply is clean and filtered, with a large capacitor to suppress voltage spikes. You can also try adding a separate logic supply for the L293D Vss pin.
By carefully analyzing your circuit and code, you should be able to diagnose and fix most common issues with the L293D motor driver. Don’t be afraid to experiment and seek help from the Arduino community if you get stuck!
FAQ
Can I use the L293D to control Stepper Motors?
No, the L293D is designed specifically for DC motors. For stepper motors, you’ll need a dedicated stepper motor driver like the A4988 or DRV8825.
How much current can the L293D supply to the motors?
The L293D has a maximum continuous current rating of 600mA per channel, with peak current up to 1.2A. However, it’s recommended to stay well below these limits to avoid overheating and damage to the IC.
Can I use the L293D with 3.3V logic?
Yes, the L293D has separate logic and motor voltage supplies. As long as your logic supply (Vss) is within the acceptable range of 4.5V to 7V, you can use 3.3V logic from a microcontroller to control the inputs. Just make sure the motor supply (Vcc2) is at the appropriate voltage for your motors.
Why are my motors running backwards?
This is probably due to the motor polarity being reversed. To fix it, simply swap the two wires going to the motor’s terminals. Alternatively, you can swap the Input pin states in your code to change the direction.
Can I control more than 2 motors with one L293D?
No, each L293D has 2 motor channels, so you’d need multiple L293D ICs to control more than 2 motors. You can also look into other motor driver options like the L298N (2 motors) or a TB6612FNG (4 motors).
Conclusion
In this article, we’ve covered everything you need to know to get started with the L293D motor driver and DC motors using Arduino. We explained the pinout and features of the L293D, provided a complete circuit diagram and code examples, and walked through a simple robot project. With this knowledge, you’re ready to tackle all kinds of motor control applications – the possibilities are endless! Just remember to always double-check your connections, provide adequate power, and have fun experimenting. Happy motoring!
No responses yet