Introduction to DS1307 and Arduino
The DS1307 is a popular real-time clock (RTC) module that provides accurate timekeeping for Arduino projects. It allows your Arduino to keep track of the current time and date, even when the power is disconnected. In this comprehensive article, we will explore how to interface the DS1307 with an Arduino, covering everything from the basics to advanced topics.
What is the DS1307?
The DS1307 is a low-power, serial real-time clock (RTC) module that provides a simple way to add timekeeping functionality to your Arduino projects. It uses the I2C communication protocol to communicate with the Arduino, making it easy to set up and use.
Why Use the DS1307 with Arduino?
There are several reasons why you might want to use the DS1307 with your Arduino projects:
-
Accurate Timekeeping: The DS1307 provides accurate timekeeping, even when the Arduino is powered off or reset. It has a built-in battery backup that maintains the time and date information.
-
Low Power Consumption: The DS1307 is designed for low power consumption, making it suitable for battery-powered projects.
-
Easy Integration: The DS1307 uses the I2C communication protocol, which is widely supported by Arduino libraries, making it easy to integrate into your projects.
DS1307 Features
The DS1307 offers the following features:
- Real-time clock (RTC) counts seconds, minutes, hours, date of the month, month, day of the week, and year with leap-year compensation valid up to 2100
- 56-byte, battery-backed, non-volatile (NV) RAM for data storage
- Two-wire serial interface (I2C)
- Programmable square-wave output signal
- Automatic power-fail detect and switch circuitry
Hardware Requirements
To interface the DS1307 with an Arduino, you will need the following hardware components:
- Arduino board (e.g., Arduino Uno)
- DS1307 RTC module
- Breadboard
- Jumper wires
- 3.3V or 5V power source (depending on your Arduino board)
Wiring the DS1307 to Arduino
Wiring the DS1307 to your Arduino is a straightforward process. Follow these steps to connect the DS1307 to your Arduino:
- Connect the VCC pin of the DS1307 to the 5V pin of the Arduino.
- Connect the GND pin of the DS1307 to the GND pin of the Arduino.
- Connect the SDA pin of the DS1307 to the SDA pin of the Arduino (usually labeled as A4 or SDA).
- Connect the SCL pin of the DS1307 to the SCL pin of the Arduino (usually labeled as A5 or SCL).
Here’s a table summarizing the connections:
DS1307 Pin | Arduino Pin |
---|---|
VCC | 5V |
GND | GND |
SDA | A4 or SDA |
SCL | A5 or SCL |
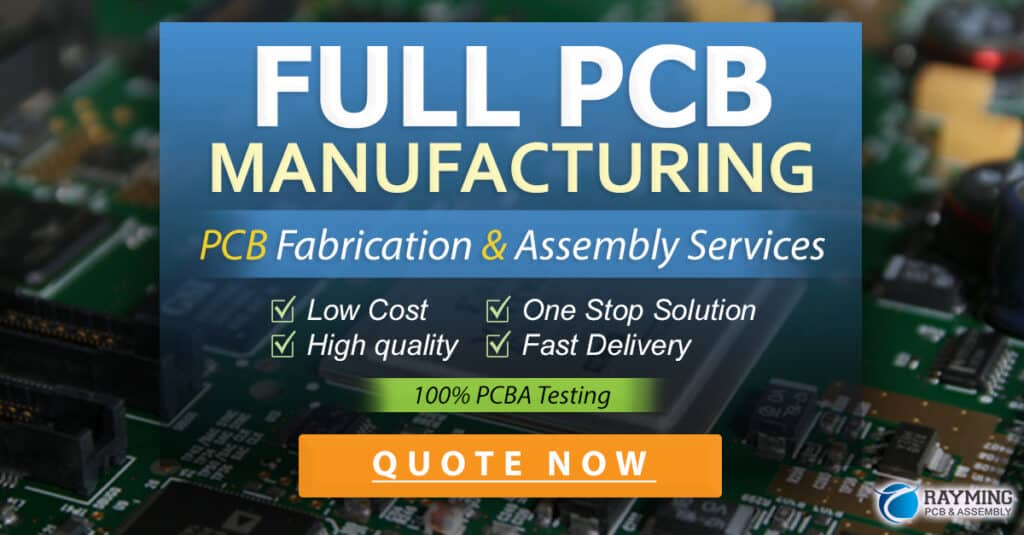
Software Setup
To use the DS1307 with your Arduino, you need to install the necessary libraries and set up the software.
Installing the RTClib Library
The RTClib library simplifies the process of communicating with the DS1307 module. To install the library, follow these steps:
- Open the Arduino IDE.
- Go to Sketch > Include Library > Manage Libraries.
- In the Library Manager, search for “RTClib”.
- Select the “RTClib by Adafruit” library and click on the “Install” button.
Setting the Time on the DS1307
Before using the DS1307 for timekeeping, you need to set the initial time and date. Here’s a simple Arduino sketch that demonstrates how to set the time on the DS1307:
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
// Set the time and date (uncomment the line below and modify as needed)
// rtc.adjust(DateTime(2023, 5, 26, 12, 0, 0));
}
void loop() {
DateTime now = rtc.now();
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(' ');
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.print(now.second(), DEC);
Serial.println();
delay(1000);
}
To set the time, uncomment the line rtc.adjust(DateTime(2023, 5, 26, 12, 0, 0));
and modify the year, month, day, hour, minute, and second parameters according to your desired date and time. Upload the sketch to your Arduino, and the DS1307 will be set with the specified time.
Reading Time and Date from the DS1307
Once the DS1307 is set with the correct time and date, you can easily read the current time and date in your Arduino sketches. Here’s an example sketch that demonstrates how to read the time and date from the DS1307:
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
}
void loop() {
DateTime now = rtc.now();
Serial.print("Date: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.println(now.day(), DEC);
Serial.print("Time: ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.println(now.second(), DEC);
delay(1000);
}
This sketch retrieves the current date and time from the DS1307 using the rtc.now()
function and prints the values to the serial monitor. The date is displayed in the format “YYYY/MM/DD,” and the time is displayed in the format “HH:MM:SS.”
Advanced Topics
Using the DS1307 with Other Libraries
While the RTClib library is a popular choice for interfacing the DS1307 with Arduino, there are other libraries available that provide additional functionality or compatibility with different platforms. Some notable libraries include:
- DS1307RTC: This library is part of the Arduino Time library and provides a simple interface for working with the DS1307.
- Rtc_Pcf8563: This library is designed for the PCF8563 RTC module but also supports the DS1307.
- uRTCLib: This library is a lightweight alternative to the RTClib library and supports various RTC modules, including the DS1307.
When choosing a library, consider factors such as ease of use, compatibility with your specific Arduino board, and the features you require for your project.
Battery Backup and Power Considerations
The DS1307 module includes a built-in battery backup feature that allows it to maintain accurate timekeeping even when the main power supply is disconnected. To take advantage of this feature, you need to connect a 3V coin cell battery (such as CR2032) to the designated battery pins on the module.
When the main power supply is available, the DS1307 automatically switches to it and charges the backup battery. If the main power is disconnected, the module seamlessly transitions to battery power to keep the time and date information intact.
It’s important to note that the battery life depends on various factors, such as the ambient temperature and the frequency of power outages. In general, a high-quality coin cell battery can provide backup power for several years.
Handling Daylight Saving Time (DST)
Daylight Saving Time (DST) is a practice of advancing clocks during summer months to make better use of daylight hours. If your project needs to handle DST, you’ll need to implement additional logic in your Arduino sketch.
The DS1307 itself does not have built-in support for DST, so you’ll need to manually adjust the time when DST begins and ends. You can use conditional statements in your sketch to check for specific dates and times when DST changes occur and make the necessary adjustments to the RTC.
Here’s a simplified example of how you can handle DST in your Arduino sketch:
void loop() {
DateTime now = rtc.now();
// Check if it's the start of DST (e.g., second Sunday in March at 2:00 AM)
if (now.month() == 3 && now.day() >= 8 && now.day() <= 14 && now.dayOfTheWeek() == 0 && now.hour() == 2) {
// Adjust the time forward by one hour
rtc.adjust(DateTime(now.year(), now.month(), now.day(), now.hour() + 1, now.minute(), now.second()));
}
// Check if it's the end of DST (e.g., first Sunday in November at 2:00 AM)
if (now.month() == 11 && now.day() >= 1 && now.day() <= 7 && now.dayOfTheWeek() == 0 && now.hour() == 2) {
// Adjust the time backward by one hour
rtc.adjust(DateTime(now.year(), now.month(), now.day(), now.hour() - 1, now.minute(), now.second()));
}
// Rest of the code...
}
In this example, the sketch checks for the specific conditions that determine the start and end of DST (assuming the United States DST rules). When the conditions are met, the time is adjusted accordingly using the rtc.adjust()
function.
Keep in mind that DST rules vary by country and region, so you may need to modify the conditions based on your specific location and the applicable DST rules.
FAQ
-
Q: Can I use the DS1307 with a 3.3V Arduino board?
A: Yes, the DS1307 is compatible with both 3.3V and 5V Arduino boards. However, make sure to connect the VCC pin of the DS1307 to the appropriate power supply (3.3V or 5V) depending on your Arduino board. -
Q: How accurate is the DS1307 RTC module?
A: The DS1307 has a typical accuracy of ±2 ppm (parts per million) at room temperature, which translates to an error of about 1 minute per year. However, the actual accuracy may vary depending on factors such as temperature and aging of the crystal oscillator. -
Q: Can I use multiple DS1307 modules with a single Arduino board?
A: Yes, you can connect multiple DS1307 modules to a single Arduino board using the I2C bus. Each module should have a unique I2C address, which is typically configurable using solder jumpers or by modifying the module’s firmware. -
Q: How long does the backup battery last in the DS1307?
A: The backup battery life depends on factors such as the battery type, ambient temperature, and the frequency of power outages. A high-quality CR2032 coin cell battery can typically provide backup power for several years. -
Q: Can I use the DS1307 for timing-critical applications?
A: While the DS1307 provides accurate timekeeping, it may not be suitable for timing-critical applications that require high precision or real-time performance. For such applications, you may need to consider more advanced timing solutions or dedicated real-time operating systems (RTOS).
Conclusion
In this article, we explored how to interface the DS1307 real-time clock module with an Arduino. We covered the basics of wiring, software setup, setting the time, and reading the current time and date. We also discussed advanced topics such as using alternative libraries, handling battery backup, and dealing with Daylight Saving Time.
The DS1307 is a reliable and easy-to-use RTC module that adds timekeeping functionality to your Arduino projects. By following the steps outlined in this article, you can easily integrate the DS1307 into your own projects and leverage its features to keep track of time accurately.
Remember to consider factors such as power requirements, backup battery life, and the specific needs of your application when using the DS1307. With the knowledge gained from this article, you’ll be well-equipped to incorporate the DS1307 into your Arduino projects and build innovative timekeeping solutions.
No responses yet