What is a DIY Timer?
A DIY timer is a device that can be programmed to control the duration of a specific process or task. It consists of a few essential components, including a microcontroller, a display, and input buttons. The microcontroller is the brain of the timer, processing the user’s input and controlling the output. The display shows the countdown or elapsed time, while the input buttons allow the user to set the desired time and start/stop the timer.
Components of a DIY Timer
To build a DIY timer, you will need the following components:
Component | Description |
---|---|
Microcontroller | The brain of the timer, such as an Arduino board |
Display | LCD or LED display to show the time |
Input Buttons | Buttons to set the time and start/stop the timer |
Buzzer (optional) | To alert the user when the set time has elapsed |
Relay (optional) | To control external devices or circuits |
Microcontroller
The microcontroller is the central processing unit of the DIY timer. It is responsible for executing the programmed instructions, processing user input, and controlling the output. Arduino boards, such as the Arduino Uno or Nano, are popular choices for DIY projects due to their ease of use and wide compatibility with various sensors and modules.
Display
The display is an essential component of the DIY timer, as it provides visual feedback to the user. LCD (Liquid Crystal Display) and LED (Light Emitting Diode) displays are commonly used in DIY projects. LCD displays offer the advantage of displaying text and numbers, while LED displays are simpler and more energy-efficient.
Input Buttons
Input buttons allow the user to interact with the DIY timer. They are used to set the desired time, start and stop the timer, and reset it when necessary. Tactile buttons or membrane switches are often used in DIY projects due to their low cost and ease of integration.
Buzzer (optional)
A buzzer is an optional component that can be added to the DIY timer to alert the user when the set time has elapsed. Piezo buzzers are commonly used in DIY projects because they are small, low-cost, and easy to integrate with microcontrollers.
Relay (optional)
A relay is an electrically operated switch that can be used to control external devices or circuits. By incorporating a relay into the DIY timer, you can automate the switching of lights, motors, or other devices based on the timer’s output.
How a DIY Timer Works
The working principle of a DIY timer is relatively simple. The user sets the desired time using the input buttons, and the microcontroller stores this value. When the user starts the timer, the microcontroller begins counting down from the set time. The display continuously updates to show the remaining time. When the count reaches zero, the microcontroller can trigger an output, such as activating a buzzer or switching a relay.
Here’s a basic flowchart illustrating the working of a DIY timer:
graph TD
A[Start] --> B[Set Time]
B --> C[Start Timer]
C --> D[Update Display]
D --> E{Time = 0?}
E -->|No| D
E -->|Yes| F[Trigger Output]
F --> G[Stop]
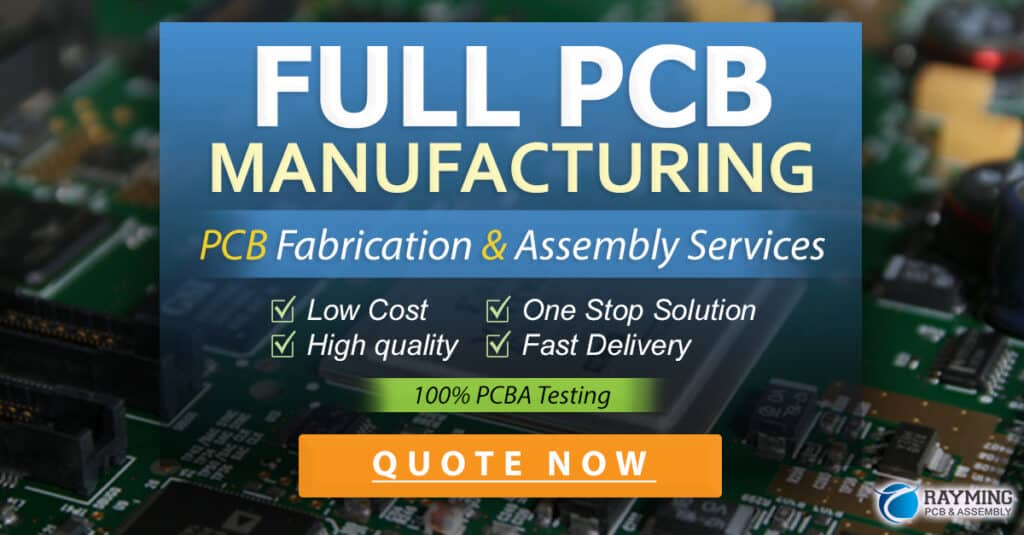
Programming a DIY Timer
To program a DIY timer, you need to use a microcontroller development environment, such as the Arduino IDE (Integrated Development Environment). The Arduino IDE allows you to write, compile, and upload code to the microcontroller board.
Here’s a simple example code for a DIY timer using an Arduino board, an LCD display, and input buttons:
#include <LiquidCrystal.h>
const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
const int startButton = 8;
const int resetButton = 9;
int timeLeft = 0;
bool timerRunning = false;
void setup() {
lcd.begin(16, 2);
pinMode(startButton, INPUT_PULLUP);
pinMode(resetButton, INPUT_PULLUP);
}
void loop() {
if (digitalRead(startButton) == LOW) {
timerRunning = !timerRunning;
delay(200);
}
if (digitalRead(resetButton) == LOW) {
timeLeft = 0;
timerRunning = false;
delay(200);
}
if (timerRunning && timeLeft > 0) {
delay(1000);
timeLeft--;
}
lcd.setCursor(0, 0);
lcd.print("Time Left: ");
lcd.print(timeLeft);
lcd.print("s ");
}
This code sets up an LCD display and two input buttons, one for starting/stopping the timer and another for resetting it. The loop()
function continuously checks the state of the buttons and updates the timer accordingly. The remaining time is displayed on the LCD.
Applications of DIY Timers
DIY timers have a wide range of applications, from simple household projects to complex industrial processes. Some common applications include:
1. Kitchen Timers
A DIY kitchen timer can be used to keep track of cooking or baking times. It can be programmed to alert the user when the set time has elapsed, ensuring that food is cooked to perfection.
2. Workout Timers
DIY timers can be used to create personalized workout routines. Users can set the duration of each exercise and rest periods, and the timer will alert them when it’s time to switch between activities.
3. Pomodoro Technique Timers
The Pomodoro Technique is a time management method that involves working in 25-minute intervals, followed by short breaks. A DIY timer can be programmed to follow this technique, helping users stay focused and productive.
4. Irrigation Systems
DIY timers can be used to automate irrigation systems in gardens or agricultural settings. By connecting the timer to a relay and solenoid valves, users can set specific watering schedules for their plants.
5. Lighting Control
DIY timers can be used to control lighting in homes, offices, or public spaces. By connecting the timer to a relay and light fixtures, users can set specific times for lights to turn on and off, saving energy and adding convenience.
Advantages of DIY Timers
-
Customization: DIY timers can be tailored to specific needs and requirements, allowing users to create personalized timing solutions.
-
Cost-effective: Building a DIY timer is often more affordable than purchasing a commercial timer with similar features.
-
Educational: Creating a DIY timer is an excellent way to learn about electronics, programming, and problem-solving.
-
Flexibility: DIY timers can be easily modified or expanded to include additional features or functionalities.
Challenges and Considerations
-
Accuracy: The accuracy of a DIY timer depends on the quality of the components used and the stability of the power supply. Using high-quality components and a stable power source can help ensure accurate timing.
-
Safety: When working with electronics, it’s essential to follow proper safety guidelines, especially when dealing with mains-powered devices. Always ensure that the components and wiring are properly insulated and secured.
-
Compatibility: When selecting components for a DIY timer, ensure that they are compatible with each other and with the microcontroller being used. Refer to the component datasheets and specifications for guidance.
-
Debugging: As with any DIY project, debugging is an essential skill. When encountering issues, use serial communication to print debug messages, and carefully review the code and wiring for potential errors.
FAQ
1. What is the difference between a DIY timer and a commercial timer?
A DIY timer is a custom-built device created by an individual, while a commercial timer is a pre-manufactured product available for purchase. DIY timers offer more flexibility and customization options, while commercial timers are often more polished and may have additional features.
2. Can a DIY timer be used for high-precision timing applications?
While DIY timers can be quite accurate, they may not be suitable for applications requiring extremely high precision, such as scientific experiments or industrial processes. For such applications, it’s best to use specialized, high-precision timing equipment.
3. How long does it take to build a DIY timer?
The time required to build a DIY timer varies depending on the complexity of the design and the builder’s experience level. A simple timer can be built in a few hours, while more complex projects may take several days or weeks.
4. What safety precautions should I take when building a DIY timer?
Always follow proper safety guidelines when working with electronics. Use insulated tools, wear protective gear, and ensure that components and wiring are properly secured. When dealing with mains-powered devices, consult with a qualified electrician or engineer.
5. Where can I find more resources on building DIY timers?
There are numerous online resources available for building DIY timers, including tutorials, forums, and project repositories. Websites like Instructables, Hackaday, and Arduino Project Hub are excellent starting points for finding inspiration and guidance.
Conclusion
DIY timers are versatile devices that can be used in a wide range of applications, from simple household projects to complex industrial processes. By understanding the components, working principles, and programming concepts behind DIY timers, users can create personalized timing solutions that meet their specific needs.
Building a DIY timer is an excellent way to learn about electronics, programming, and problem-solving. It offers the opportunity to customize and expand the device’s capabilities, while also being a cost-effective alternative to commercial timers.
When embarking on a DIY timer project, it’s essential to prioritize safety, select compatible components, and be prepared to debug and troubleshoot issues that may arise. With patience, persistence, and a willingness to learn, anyone can successfully build a DIY timer and unlock its many applications.
No responses yet