Introduction to the Mkr1000
The Mkr1000 is a member of the Arduino MKR family, designed specifically for IoT applications. It combines the functionality of the Arduino Zero with the connectivity of the Wi-Fi Shield, all in a small form factor. The board is equipped with a SAMD21 Cortex-M0+ 32-bit ARM MCU, providing plenty of processing power for your projects.
Key Features of the Mkr1000
- Microcontroller: SAMD21 Cortex-M0+ 32-bit ARM MCU
- Operating Voltage: 3.3V
- Digital I/O Pins: 14 (8 provide PWM output)
- Analog Input Pins: 7 (ADC 8/10/12 bit)
- DC Current per I/O Pin: 7 mA
- Flash Memory: 256 KB
- SRAM: 32 KB
- EEPROM: Up to 16 KB
- Clock Speed: 48 MHz
- Wi-Fi: On-board Wi-Fi module (ESP8266)
Understanding the Mkr1000 Pinout
To effectively utilize the Mkr1000 in your IoT projects, it’s essential to understand its pinout. The board features a compact layout with carefully designed pin arrangements for optimal performance and ease of use.
Digital I/O Pins
The Mkr1000 has 14 digital input/output pins, labeled D0 to D13. These pins can be used for various purposes, such as controlling LEDs, reading sensors, or communicating with other devices. Eight of these pins (D3, D4, D5, D6, D8, D9, D10, and D11) also support pulse-width modulation (PWM) output.
Pin | Function | Notes |
---|---|---|
D0 | Digital I/O | Serial RX |
D1 | Digital I/O | Serial TX |
D2 | Digital I/O | |
D3 | Digital I/O, PWM | |
D4 | Digital I/O, PWM | |
D5 | Digital I/O, PWM | |
D6 | Digital I/O, PWM | |
D7 | Digital I/O | |
D8 | Digital I/O, PWM | |
D9 | Digital I/O, PWM | |
D10 | Digital I/O, PWM | SPI SS |
D11 | Digital I/O, PWM | SPI MOSI |
D12 | Digital I/O | SPI MISO |
D13 | Digital I/O | SPI SCK, LED_BUILTIN |
Analog Input Pins
The Mkr1000 features 7 analog input pins, labeled A0 to A6. These pins are used to read analog values from sensors or other devices. The board’s analog-to-digital converter (ADC) supports 8, 10, or 12-bit resolution, allowing for precise measurements.
Pin | Function | Notes |
---|---|---|
A0 | Analog Input | ADC 8/10/12 bit |
A1 | Analog Input | ADC 8/10/12 bit |
A2 | Analog Input | ADC 8/10/12 bit |
A3 | Analog Input | ADC 8/10/12 bit |
A4 | Analog Input | ADC 8/10/12 bit |
A5 | Analog Input | ADC 8/10/12 bit |
A6 | Analog Input | ADC 8/10/12 bit |
Power Pins
The Mkr1000 has several power-related pins that are essential for proper operation:
Pin | Function | Notes |
---|---|---|
VIN | Power Input | 5V external power supply input |
5V | Power Output | Regulated 5V output (from VIN or USB) |
3V3 | Power Output | Regulated 3.3V output |
GND | Ground |
It’s important to note that the Mkr1000 operates at 3.3V, and applying voltages higher than 3.3V to any I/O pin may damage the board.
Connecting Peripherals to the Mkr1000
With its diverse set of I/O pins, the Mkr1000 can interface with a wide range of sensors, actuators, and other peripherals. Here are some common examples:
Connecting LEDs
To connect an LED to the Mkr1000, you’ll need the following components:
– LED
– 220 ohm resistor
– Jumper wires
Follow these steps:
1. Connect the longer leg (anode) of the LED to one end of the resistor.
2. Connect the other end of the resistor to a digital I/O pin (e.g., D3).
3. Connect the shorter leg (cathode) of the LED to the GND pin.
Here’s a simple Arduino sketch to control the LED:
const int ledPin = 3;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
digitalWrite(ledPin, HIGH);
delay(1000);
digitalWrite(ledPin, LOW);
delay(1000);
}
Reading Analog Sensors
The Mkr1000’s analog input pins can be used to read values from various analog sensors, such as temperature sensors or potentiometers. For example, let’s connect a TMP36 temperature sensor:
- Connect the left pin of the TMP36 (facing the flat side) to the 3V3 pin.
- Connect the middle pin to an analog input pin (e.g., A0).
- Connect the right pin to the GND pin.
Here’s an Arduino sketch to read the temperature:
const int sensorPin = A0;
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue = analogRead(sensorPin);
float voltage = sensorValue * (3.3 / 1023.0);
float temperature = (voltage - 0.5) * 100;
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println(" °C");
delay(1000);
}
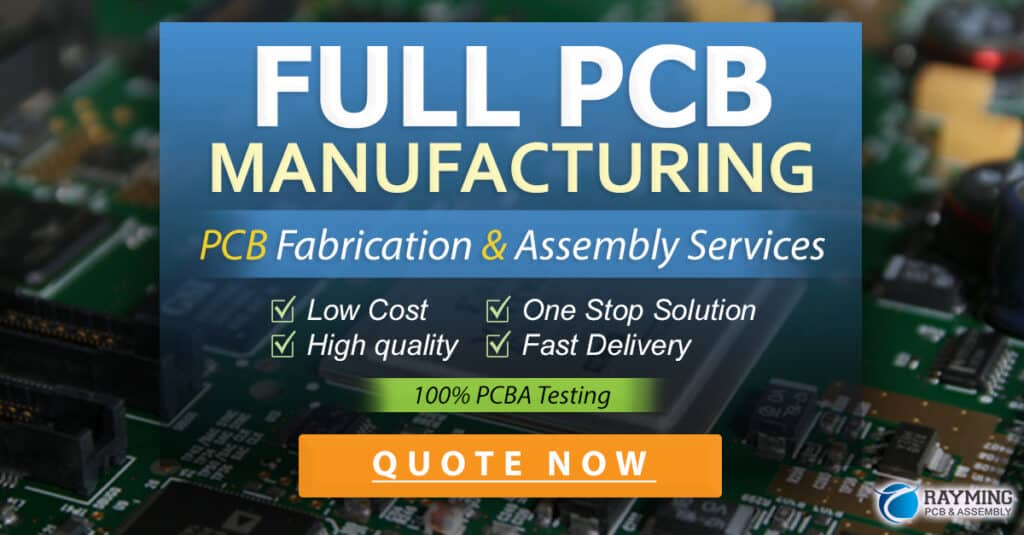
Wi-Fi Connectivity
One of the key features of the Mkr1000 is its built-in Wi-Fi capabilities, making it perfect for IoT projects that require internet connectivity. The on-board ESP8266 Wi-Fi module allows the Mkr1000 to connect to wireless networks and communicate with other devices or cloud services.
Connecting to a Wi-Fi Network
To connect the Mkr1000 to a Wi-Fi network, you’ll need to use the WiFi101 library. Here’s a basic example:
#include <SPI.h>
#include <WiFi101.h>
char ssid[] = "YourNetworkSSID";
char pass[] = "YourNetworkPassword";
void setup() {
Serial.begin(9600);
while (!Serial);
if (WiFi.status() == WL_NO_SHIELD) {
Serial.println("WiFi shield not present");
while (true);
}
Serial.println("Connecting to WiFi...");
while (WiFi.begin(ssid, pass) != WL_CONNECTED) {
delay(1000);
Serial.print(".");
}
Serial.println("Connected to WiFi");
printWiFiStatus();
}
void loop() {
// Your code here
}
void printWiFiStatus() {
Serial.print("SSID: ");
Serial.println(WiFi.SSID());
IPAddress ip = WiFi.localIP();
Serial.print("IP Address: ");
Serial.println(ip);
}
Communicating with Cloud Services
Once connected to a Wi-Fi network, the Mkr1000 can communicate with various cloud services, such as MQTT brokers or REST APIs. Here’s an example using the PubSubClient library to publish messages to an MQTT broker:
#include <SPI.h>
#include <WiFi101.h>
#include <PubSubClient.h>
char ssid[] = "YourNetworkSSID";
char pass[] = "YourNetworkPassword";
char mqttServer[] = "mqtt.example.com";
int mqttPort = 1883;
WiFiClient wifiClient;
PubSubClient mqttClient(wifiClient);
void setup() {
// Connect to Wi-Fi (code omitted for brevity)
mqttClient.setServer(mqttServer, mqttPort);
mqttClient.setCallback(callback);
}
void loop() {
if (!mqttClient.connected()) {
reconnect();
}
mqttClient.loop();
}
void reconnect() {
while (!mqttClient.connected()) {
if (mqttClient.connect("MKR1000Client")) {
Serial.println("Connected to MQTT broker");
mqttClient.subscribe("inTopic");
} else {
Serial.print("Failed, rc=");
Serial.print(mqttClient.state());
Serial.println(" retrying in 5 seconds");
delay(5000);
}
}
}
void callback(char* topic, byte* payload, unsigned int length) {
// Handle incoming MQTT messages
}
void publishMessage(char* topic, char* message) {
mqttClient.publish(topic, message);
}
Frequently Asked Questions
-
What is the operating voltage of the Mkr1000?
The Mkr1000 operates at 3.3V. Applying voltages higher than 3.3V to any I/O pin may damage the board. -
How many analog input pins does the Mkr1000 have?
The Mkr1000 has 7 analog input pins, labeled A0 to A6. -
Can I use the Mkr1000 with 5V sensors or peripherals?
No, the Mkr1000’s I/O pins are not 5V tolerant. If you need to interface with 5V devices, you’ll need to use level shifters or voltage dividers to convert the signals to 3.3V. -
What is the flash memory size of the Mkr1000?
The Mkr1000 has 256 KB of flash memory for storing your Arduino sketches and other data. -
Can I use the Mkr1000 with a LiPo battery?
Yes, the Mkr1000 has a built-in LiPo battery charging circuit. Simply connect a compatible LiPo battery to the JST connector on the board.
Conclusion
The Mkr1000 is a powerful and versatile board that is well-suited for IoT projects. Its compact size, built-in Wi-Fi capabilities, and low power consumption make it an attractive choice for developers looking to create connected devices. By understanding the Mkr1000 pinout and effectively utilizing its features, you can bring your IoT ideas to life.
Remember to always refer to the official Arduino documentation and libraries when working with the Mkr1000 to ensure you’re using the most up-to-date information and best practices. Happy tinkering!
No responses yet