What is the ADS1115?
The ADS1115 is a delta-sigma ADC developed by Texas Instruments. It offers 16-bit resolution, allowing for precise measurements of analog signals. The device operates on a wide supply voltage range of 2.0V to 5.5V, making it compatible with different power sources, including the Arduino’s 5V and 3.3V pins.
Key Features of the ADS1115
- High Resolution: The ADS1115 provides 16-bit resolution, enabling the measurement of small signal changes with high accuracy.
- Programmable Gain Amplifier (PGA): The built-in PGA allows for input signal amplification up to 16x, enhancing the dynamic range and signal-to-noise ratio.
- Multiple Input Channels: The ADS1115 features four single-ended or two differential input channels, allowing for simultaneous measurement of multiple signals.
- I2C Interface: The device communicates with the Arduino using the I2C protocol, which simplifies wiring and enables easy integration with other I2C devices.
- Programmable Data Rate: The ADS1115 supports data rates from 8 samples per second (SPS) to 860 SPS, allowing for trade-offs between conversion speed and noise reduction.
- Low Power Consumption: With a typical current consumption of only 150μA in continuous conversion mode, the ADS1115 is suitable for low-power applications.
Interfacing ADS1115 with Arduino
To interface the ADS1115 with an Arduino, you will need the following components:
– Arduino board (e.g., Arduino Uno)
– ADS1115 module
– Breadboard
– Jumper wires
Wiring Connections
The ADS1115 communicates with the Arduino using the I2C protocol. The wiring connections are as follows:
ADS1115 Pin | Arduino Pin |
---|---|
VDD | 5V |
GND | GND |
SCL | SCL (A5) |
SDA | SDA (A4) |
ADDR | GND* |
ALRT | Not Connected |
*Note: The ADDR pin is used to set the I2C address of the ADS1115. By connecting it to GND, the address is set to the default value of 0x48. You can connect the ADDR pin to VDD or SCL to change the address if needed.
Arduino Library Installation
To simplify the usage of the ADS1115 with Arduino, you can use the Adafruit ADS1X15 library. Follow these steps to install the library:
- Open the Arduino IDE.
- Go to Sketch -> Include Library -> Manage Libraries.
- Search for “Adafruit ADS1X15” in the search bar.
- Click on the library and select the latest version.
- Click the “Install” button to install the library.
Example Code
Here’s an example code that demonstrates how to read analog values from the ADS1115 using the Arduino:
#include <Wire.h>
#include <Adafruit_ADS1X15.h>
Adafruit_ADS1115 ads;
void setup() {
Serial.begin(9600);
ads.begin();
}
void loop() {
int16_t adc0 = ads.readADC_SingleEnded(0);
float voltage0 = ads.computeVolts(adc0);
Serial.print("ADC Value: ");
Serial.print(adc0);
Serial.print("\tVoltage: ");
Serial.print(voltage0, 3);
Serial.println("V");
delay(1000);
}
This code initializes the ADS1115 and reads the analog value from channel 0 in single-ended mode. The readADC_SingleEnded()
function returns the raw ADC value, which is then converted to voltage using the computeVolts()
function. The voltage value is printed to the serial monitor every second.
Configuring the ADS1115
The ADS1115 offers various configuration options to optimize its performance for specific applications. Here are some of the key configurations:
Input Multiplexer (MUX)
The ADS1115 has four input channels that can be configured as single-ended or differential inputs. The input multiplexer (MUX) settings determine which channels are used and how they are connected.
MUX Setting | Configuration |
---|---|
0x4000 | AIN0 |
0x5000 | AIN1 |
0x6000 | AIN2 |
0x7000 | AIN3 |
0x0000 | AIN0 – AIN1 |
0x1000 | AIN0 – AIN3 |
0x2000 | AIN1 – AIN3 |
0x3000 | AIN2 – AIN3 |
To set the MUX, use the ads.setMultiplexer()
function in the Arduino code.
Programmable Gain Amplifier (PGA)
The ADS1115 has a built-in PGA that can be used to amplify the input signal. The PGA settings determine the gain factor applied to the signal.
PGA Setting | Gain Factor | Full-Scale Range |
---|---|---|
0x0000 | 1x | ±6.144V |
0x0200 | 2x | ±4.096V |
0x0400 | 4x | ±2.048V |
0x0600 | 8x | ±1.024V |
0x0800 | 16x | ±0.512V |
To set the PGA, use the ads.setGain()
function in the Arduino code.
Data Rate
The ADS1115 supports programmable data rates from 8 SPS to 860 SPS. Lower data rates provide better noise reduction but slower conversion speeds, while higher data rates offer faster conversions but with increased noise.
Data Rate Setting | Data Rate (SPS) |
---|---|
0x0000 | 8 |
0x0020 | 16 |
0x0040 | 32 |
0x0060 | 64 |
0x0080 | 128 |
0x00A0 | 250 |
0x00C0 | 475 |
0x00E0 | 860 |
To set the data rate, use the ads.setDataRate()
function in the Arduino code.
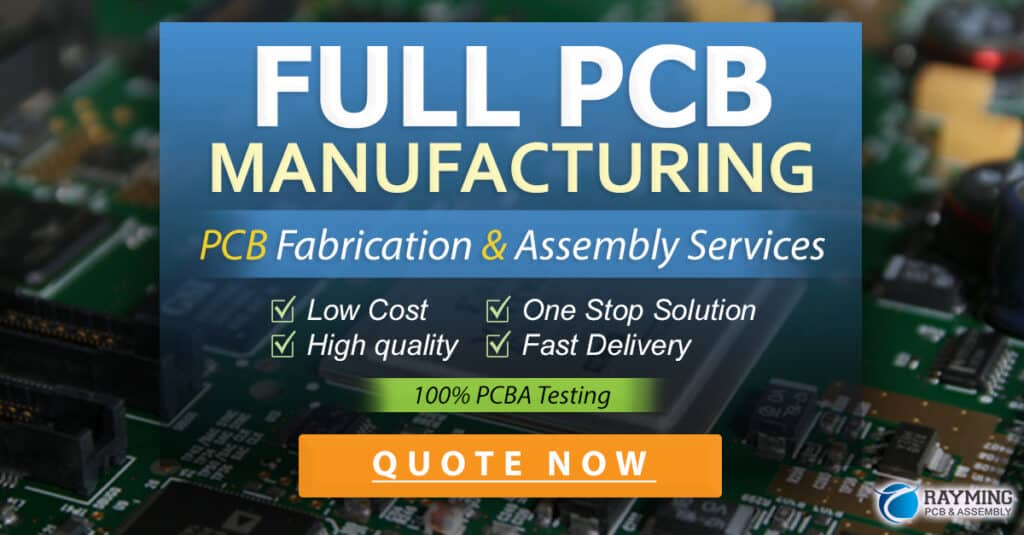
Performing Single-Shot Conversions
In addition to continuous conversion mode, the ADS1115 also supports single-shot conversions. This mode allows you to trigger a single ADC conversion and read the result. Here’s an example code snippet for performing a single-shot conversion:
void setup() {
Serial.begin(9600);
ads.begin();
ads.setGain(GAIN_TWOTHIRDS);
}
void loop() {
ads.startADCConversion(0, false); // Start conversion on channel 0, single-shot mode
while (!ads.conversionComplete()); // Wait for conversion to complete
int16_t adc0 = ads.getLastConversionResults(); // Read the conversion result
float voltage0 = ads.computeVolts(adc0); // Convert to voltage
Serial.print("ADC Value: ");
Serial.print(adc0);
Serial.print("\tVoltage: ");
Serial.print(voltage0, 3);
Serial.println("V");
delay(1000);
}
In this code, the startADCConversion()
function is used to initiate a single-shot conversion on channel 0. The conversionComplete()
function is used to check if the conversion is complete, and the getLastConversionResults()
function retrieves the result.
FAQs
-
What is the resolution of the ADS1115?
The ADS1115 has a 16-bit resolution, providing 65,536 discrete steps for representing the analog input signal. -
Can I use the ADS1115 with 3.3V logic levels?
Yes, the ADS1115 is compatible with both 5V and 3.3V logic levels, making it suitable for use with different Arduino boards and microcontrollers. -
How many input channels does the ADS1115 have?
The ADS1115 has four input channels that can be configured as single-ended or differential inputs. -
What is the maximum sampling rate of the ADS1115?
The ADS1115 supports data rates up to 860 samples per second (SPS). -
Is the ADS1115 suitable for low-power applications?
Yes, the ADS1115 has a low typical current consumption of 150μA in continuous conversion mode, making it suitable for battery-powered and low-power applications.
Conclusion
The ADS1115 is a versatile and high-performance ADC that offers excellent resolution, programmable gain, and multiple input channels. Interfacing the ADS1115 with an Arduino is straightforward using the I2C protocol and the Adafruit ADS1X15 library. By configuring the input multiplexer, programmable gain amplifier, and data rate settings, you can optimize the ADS1115’s performance for your specific application requirements.
Whether you are building a data acquisition system, measuring sensor outputs, or developing industrial control applications, the ADS1115 provides a reliable and accurate solution for analog-to-digital conversion. With its ease of use and extensive features, the ADS1115 is a valuable addition to any Arduino-based project.
No responses yet