What is the DHT22 Sensor?
The DHT22, also known as the AM2302, is a digital temperature and humidity sensor. It utilizes a capacitive humidity sensor and a thermistor to measure the surrounding air and provides a digital signal output. The sensor is known for its high accuracy, stability, and long-term reliability.
Key Features of the DHT22 Sensor
- Temperature range: -40°C to +80°C (-40°F to +176°F)
- Humidity range: 0% to 100% RH
- Temperature accuracy: ±0.5°C (±0.9°F)
- Humidity accuracy: ±2% RH (at 25°C)
- Operating voltage: 3.3V to 5.5V
- Low power consumption
- Long-term stability
DHT22 Pinout and Wiring
The DHT22 sensor has a simple 4-pin interface that makes it easy to connect to microcontrollers such as Arduino or Raspberry Pi. Let’s take a closer look at the DHT22 pinout and how to wire it correctly.
DHT22 Pinout
Pin | Name | Description |
---|---|---|
1 | VCC | Power supply (3.3V to 5.5V) |
2 | DATA | Serial data output |
3 | NC | Not connected (leave unconnected) |
4 | GND | Ground |
Wiring the DHT22 Sensor
To connect the DHT22 sensor to a microcontroller, follow these steps:
- Connect the VCC pin to the power supply (3.3V or 5V, depending on your microcontroller).
- Connect the DATA pin to a digital input/output pin on your microcontroller.
- Connect a 10kΩ pull-up resistor between the DATA pin and VCC pin.
- Connect the GND pin to the ground of your microcontroller.
Here’s an example wiring diagram for connecting the DHT22 sensor to an Arduino:
DHT22
+-------+
VCC ---| 1 |
| 2 |--- DATA
| 3 |
GND ---| 4 |
+-------+
|
|
10kΩ
|
VCC
How the DHT22 Sensor Works
The DHT22 sensor uses a single-wire bi-directional protocol to communicate with the microcontroller. The communication process involves the following steps:
- The microcontroller sends a start signal to the sensor by pulling the DATA line low for at least 18ms.
- The sensor responds by pulling the DATA line low for 80μs, followed by a high pulse for 80μs.
- The sensor then sends 40 bits of data, starting with the humidity data (16 bits), followed by the temperature data (16 bits), and a checksum (8 bits).
- Each bit is transmitted by holding the DATA line low for 50μs, followed by a high pulse. The duration of the high pulse determines the bit value: 26-28μs for a “0” and 70μs for a “1”.
- The microcontroller reads the 40 bits of data and verifies the checksum to ensure the integrity of the received data.
DHT22 Data Format
The DHT22 sensor sends the temperature and humidity data as 16-bit values. The humidity data is sent first, followed by the temperature data.
- Humidity data: 16 bits (integer part: 8 bits, decimal part: 8 bits)
- Temperature data: 16 bits (integer part: 8 bits, decimal part: 8 bits, sign bit: 1 bit)
To calculate the actual humidity and temperature values, use the following formulas:
- Humidity (%) = Humidity data / 10
- Temperature (°C) = Temperature data / 10
If the temperature data has the sign bit set (MSB = 1), it indicates a negative temperature value. In this case, subtract 65536 from the temperature data before dividing by 10.
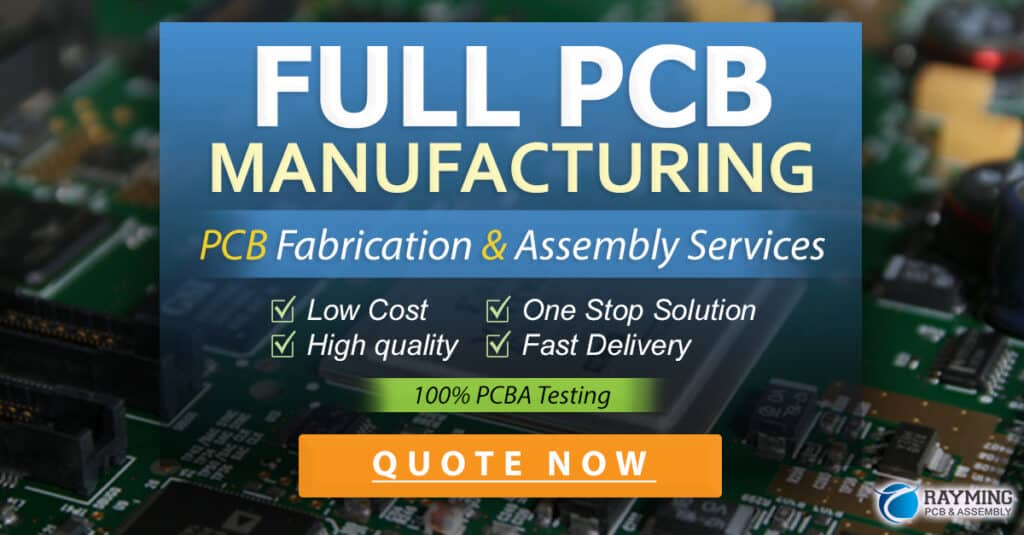
Interfacing the DHT22 Sensor with Arduino
To read data from the DHT22 sensor using an Arduino, you can use the DHT library. This library simplifies the communication process and provides an easy-to-use interface for retrieving temperature and humidity values.
Installing the DHT Library
- Open the Arduino IDE.
- Go to Sketch -> Include Library -> Manage Libraries.
- Search for “DHT” in the Library Manager.
- Install the “DHT sensor library” by Adafruit.
Example Arduino Code
Here’s an example Arduino sketch that demonstrates how to read temperature and humidity data from the DHT22 sensor:
#include <DHT.h>
#define DHTPIN 2 // Digital pin connected to the DHT sensor
#define DHTTYPE DHT22 // DHT 22 (AM2302)
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
// Wait a few seconds between measurements
delay(2000);
// Reading temperature or humidity takes about 250 milliseconds!
float humidity = dht.readHumidity();
float temperature = dht.readTemperature();
// Check if any reads failed and exit early (to try again)
if (isnan(humidity) || isnan(temperature)) {
Serial.println("Failed to read from DHT sensor!");
return;
}
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.print("% Temperature: ");
Serial.print(temperature);
Serial.println("°C");
}
This code initializes the DHT sensor, reads the humidity and temperature values every 2 seconds, and prints the results to the serial monitor.
Applications of the DHT22 Sensor
The DHT22 sensor’s accuracy and reliability make it suitable for a wide range of applications. Here are some common use cases:
Weather Stations
The DHT22 sensor is often used in weather stations to monitor ambient temperature and humidity. By combining the sensor with other components like a barometric pressure sensor and an anemometer, you can create a comprehensive weather monitoring system.
Home Automation
In home automation systems, the DHT22 sensor can be used to monitor and control the indoor environment. For example, you can use the sensor data to trigger actions like turning on/off the air conditioning or adjusting the humidifier based on the current temperature and humidity levels.
Greenhouse Monitoring
Maintaining optimal temperature and humidity levels is crucial for plant growth in greenhouses. The DHT22 sensor can be used to monitor these parameters and provide real-time data to the greenhouse control system. This allows for automatic adjustment of ventilation, heating, and irrigation to create the ideal growing conditions.
Industrial Monitoring
The DHT22 sensor can be employed in industrial settings to monitor temperature and humidity in manufacturing processes, storage facilities, and laboratories. Accurate monitoring helps ensure product quality, prevent equipment damage, and maintain a safe working environment.
Frequently Asked Questions (FAQ)
-
What is the difference between the DHT22 and DHT11 sensors?
The DHT22 sensor offers higher accuracy and a wider measurement range compared to the DHT11. The DHT22 has a temperature accuracy of ±0.5°C and a humidity accuracy of ±2% RH, while the DHT11 has a temperature accuracy of ±2°C and a humidity accuracy of ±5% RH. -
Can I use the DHT22 sensor with a 3.3V microcontroller?
Yes, the DHT22 sensor is compatible with both 3.3V and 5V microcontrollers. However, when using a 3.3V microcontroller, ensure that the DATA pin is pulled up to 3.3V using a pull-up resistor. -
How often can I read data from the DHT22 sensor?
It is recommended to have a minimum delay of 2 seconds between consecutive readings to ensure the sensor has sufficient time to stabilize and provide accurate measurements. -
What should I do if I get inconsistent or invalid readings from the DHT22 sensor?
If you encounter inconsistent or invalid readings, check the wiring connections, ensure the pull-up resistor is properly connected, and verify that the power supply is stable. Additionally, make sure you are using a reliable library and following the recommended timing constraints for communication. -
Can I use multiple DHT22 sensors with a single microcontroller?
Yes, you can connect multiple DHT22 sensors to a single microcontroller by assigning each sensor a unique digital pin. However, keep in mind that each sensor requires a separate data line and a pull-up resistor.
Conclusion
The DHT22 sensor is a valuable tool for measuring temperature and humidity in various applications. With its high accuracy, wide measurement range, and easy integration with microcontrollers, it offers a reliable solution for environmental monitoring.
By understanding the DHT22 pinout, communication protocol, and interfacing techniques, you can effectively utilize this sensor in your projects. Whether you are building a weather station, automating your home, or monitoring industrial processes, the DHT22 sensor provides the necessary data to make informed decisions and create intelligent systems.
Remember to follow the wiring guidelines, use appropriate libraries, and adhere to the recommended timing constraints to ensure accurate and consistent readings from the DHT22 sensor.
No responses yet