Arduino Nano Specifications
Before diving into the pinout details, let’s take a look at the key specifications of the Arduino Nano:
Specification | Arduino Nano (3.x) | Arduino Nano (2.x) |
---|---|---|
Microcontroller | ATmega328P | ATmega168 |
Operating Voltage | 5V | 5V |
Input Voltage (recommended) | 7-12V | 7-12V |
Input Voltage (limits) | 6-20V | 6-20V |
Digital I/O Pins | 14 (of which 6 provide PWM output) | 14 (of which 6 provide PWM output) |
Analog Input Pins | 8 | 8 |
DC Current per I/O Pin | 40 mA | 40 mA |
Flash Memory | 32 KB (of which 2 KB used by bootloader) | 16 KB (of which 2 KB used by bootloader) |
SRAM | 2 KB | 1 KB |
EEPROM | 1 KB | 512 bytes |
Clock Speed | 16 MHz | 16 MHz |
Dimensions | 18 x 45 mm | 18 x 45 mm |
These specifications provide an overview of the capabilities and limitations of the Arduino Nano board.
Arduino Nano Pinout
The Arduino Nano features a compact form factor with a well-organized pinout. Let’s explore the different pins and their functions:
Power Pins
The Arduino Nano has the following power-related pins:
VIN
: Input voltage to the Arduino board when using an external power source.5V
: Regulated power supply used to power the microcontroller and other components on the board.3.3V
: 3.3V power supply generated by the on-board Voltage Regulator.GND
: Ground pins.
Digital I/O Pins
The Arduino Nano has 14 digital I/O pins labeled D0 to D13. Each pin can be used as an input or output, and some have additional functionality:
D0
andD1
: Used for serial communication (RX and TX).D2
andD3
: Can be configured to trigger interrupts.D3
,D5
,D6
,D9
,D10
, andD11
: Provide PWM (Pulse Width Modulation) output.D10
,D11
,D12
, andD13
: Used for SPI communication.D13
: Connected to an on-board LED.
Analog Input Pins
The Arduino Nano has 8 analog input pins labeled A0 to A7. These pins can measure analog voltages between 0V and 5V with a resolution of 10 bits (0-1023).
Other Pins
AREF
: Reference voltage for analog inputs.RST
: Reset pin used to restart the microcontroller.
Here’s a visual representation of the Arduino Nano pinout:
+-----+
+------------| USB |------------+
| +-----+ |
B5 | [ ]D13/SCK MISO/D12[ ] | B4
| [ ]3.3V MOSI/D11[ ]~| B3
| [ ]V.ref ___ SS/D10[ ]~| B2
C0 | [ ]A0 / N \ D9[ ]~| B1
C1 | [ ]A1 / A \ D8[ ] | B0
C2 | [ ]A2 \ N / D7[ ] | D7
C3 | [ ]A3 \_0_/ D6[ ]~| D6
C4 | [ ]A4/SDA D5[ ]~| D5
C5 | [ ]A5/SCL D4[ ] | D4
| [ ]A6 INT1/D3[ ]~| D3
| [ ]A7 INT0/D2[ ] | D2
| [ ]5V GND[ ] |
C6 | [ ]RST RST[ ] | C6
| [ ]GND 5V MOSI GND TX1[ ] | D0
| [ ]Vin [ ] [ ] [ ] RX1[ ] | D1
| [ ] [ ] [ ] |
| MISO SCK RST |
| NANO-V3 |
+-------------------------------+
Programming the Arduino Nano
To program the Arduino Nano, you can use the Arduino Integrated Development Environment (IDE). The IDE provides a user-friendly interface for writing, compiling, and uploading sketches (programs) to the board.
Here’s a step-by-step guide to programming the Arduino Nano:
-
Install the Arduino IDE: Download and install the Arduino IDE from the official Arduino website (https://www.arduino.cc/en/software).
-
Connect the Arduino Nano: Connect your Arduino Nano to your computer using a USB cable.
-
Select the Board: In the Arduino IDE, go to “Tools” > “Board” and select “Arduino Nano” from the list.
-
Select the Port: Go to “Tools” > “Port” and select the appropriate serial port for your Arduino Nano.
-
Write your Sketch: In the Arduino IDE, write your sketch (program) using the Arduino programming language, which is based on C++. You can use the built-in examples and libraries to get started.
-
Compile and Upload: Once you have written your sketch, click on the “Verify” button to compile the code. If there are no errors, click on the “Upload” button to upload the sketch to your Arduino Nano.
-
Interact with the Board: After uploading the sketch, you can interact with your Arduino Nano based on the functionality you have programmed.
Here’s a simple example sketch that blinks the on-board LED connected to pin D13:
void setup() {
pinMode(13, OUTPUT);
}
void loop() {
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(13, LOW);
delay(1000);
}
This sketch sets pin D13 as an output, and then alternately turns the LED on and off with a delay of one second in each state.
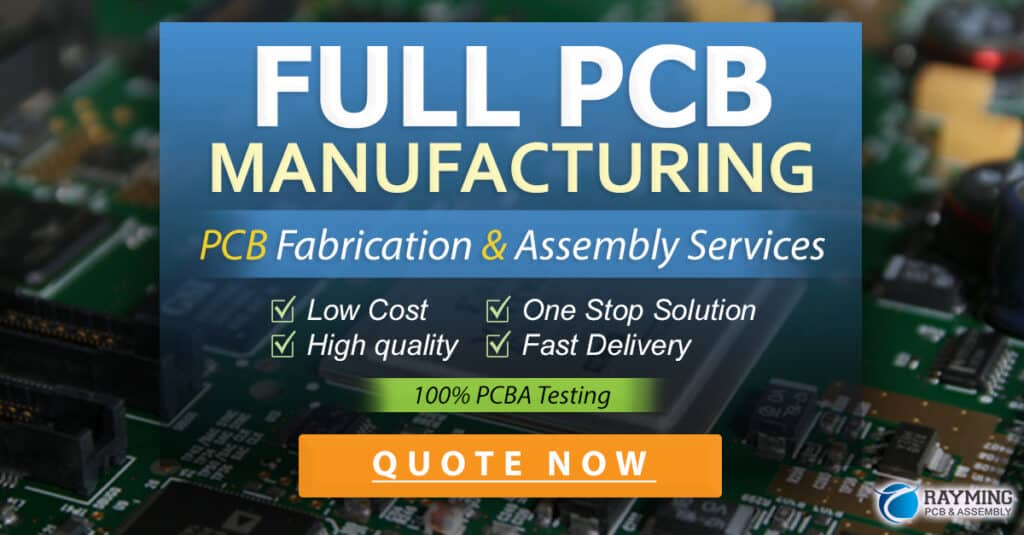
Arduino Nano Libraries and Resources
The Arduino ecosystem offers a wide range of libraries and resources to support your projects. Here are a few notable libraries and resources:
-
Official Arduino Libraries: The Arduino IDE comes with a collection of built-in libraries that provide functionality for various tasks, such as communication protocols, sensor interfacing, and more.
-
Third-Party Libraries: There are numerous third-party libraries available for the Arduino Nano that extend its capabilities. You can find libraries for specific sensors, displays, wireless communication modules, and more on platforms like GitHub and the Arduino Library Manager.
-
Arduino Reference: The official Arduino reference documentation (https://www.arduino.cc/reference/en/) provides detailed information about the Arduino language, functions, and libraries.
-
Arduino Forum: The Arduino forum (https://forum.arduino.cc/) is a community-driven platform where you can ask questions, seek assistance, and share your projects with other Arduino enthusiasts.
-
Project Tutorials and Examples: There are countless tutorials and project examples available online that demonstrate how to use the Arduino Nano for various applications. Websites like Instructables, Hackster.io, and the Arduino Project Hub are great resources for finding inspiration and learning from others.
Frequently Asked Questions (FAQ)
-
What is the difference between the Arduino Nano 3.x and 2.x versions?
The main difference between the Arduino Nano 3.x and 2.x versions lies in the microcontroller. The Arduino Nano 3.x uses the ATmega328P, while the 2.x version uses the ATmega168. The 3.x version has more flash memory, SRAM, and EEPROM compared to the 2.x version. -
Can I power the Arduino Nano using a battery?
Yes, you can power the Arduino Nano using a battery. You can connect the positive terminal of the battery to the VIN pin and the negative terminal to the GND pin. Make sure the battery voltage is within the recommended input voltage range of 7-12V. -
How do I upload a sketch to the Arduino Nano?
To upload a sketch to the Arduino Nano, make sure you have selected the correct board and port in the Arduino IDE. Then, click on the “Upload” button, which will compile the sketch and upload it to the board via the USB connection. -
Can I use the Arduino Nano for real-time applications?
While the Arduino Nano is suitable for many applications, it may not be the best choice for strict real-time applications due to its limited processing power and lack of real-time operating system. For real-time applications, you may need to consider other microcontroller platforms specifically designed for real-time tasks. -
How can I extend the functionality of the Arduino Nano?
You can extend the functionality of the Arduino Nano by connecting external components, such as sensors, actuators, displays, and communication modules. The Arduino Nano’s digital and analog pins allow you to interface with a wide range of devices. Additionally, you can leverage the vast collection of libraries and shields available for the Arduino ecosystem to add new features and capabilities to your projects.
Conclusion
The Arduino Nano is a powerful and compact microcontroller board that offers a wide range of possibilities for electronic projects. With its well-organized pinout, extensive library support, and active community, the Arduino Nano is an excellent choice for beginners and experienced makers alike.
By understanding the Arduino Nano’s specifications, pin descriptions, and programming concepts, you can unlock its potential and create innovative projects. Whether you are building a simple LED blinker or a complex IoT device, the Arduino Nano provides a solid foundation for your electronics endeavors.
Remember to explore the Arduino ecosystem, leverage the available resources, and engage with the community to further enhance your skills and bring your ideas to life. Happy coding and creating with the Arduino Nano!
No responses yet