Table of Contents
- Introduction to Component Libraries
- The Need for Query Functions
- Types of Query Functions
- Selector-based Queries
- Traversal Queries
- Filtering Queries
- Implementing Query Functions
- Using Built-in Query Functions
- Creating Custom Query Functions
- Best Practices for Query Functions
- Performance Considerations
- Error Handling and Validation
- Documentation and Examples
- Real-World Examples
- Querying Components in React
- Querying Components in Vue
- Querying Components in Angular
- FAQ
- Conclusion
Introduction to Component Libraries
Component libraries have revolutionized the way we build user interfaces. Instead of writing every UI element from scratch, developers can leverage pre-built components that are tested, optimized, and ready to be used in their applications. Some popular component libraries include:
These libraries provide a wide range of components, such as buttons, forms, modals, tables, and more, along with consistent styling and behavior. By using component libraries, developers can focus on building the core functionality of their application while relying on the library to handle the UI aspects.
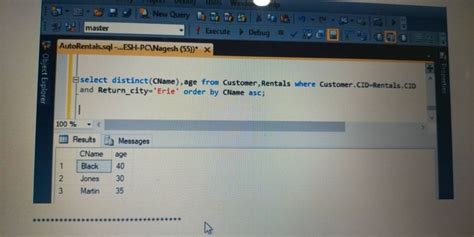
The Need for Query Functions
While component libraries offer a convenient way to build user interfaces, there are scenarios where you need to programmatically interact with the components. This is where query functions come into play. Query functions allow you to search, select, and manipulate components within your application. Some common use cases for query functions include:
- Accessing specific components for testing purposes
- Dynamically modifying component properties or behavior
- Traversing the component tree to find related components
- Filtering components based on certain criteria
Without query functions, you would need to manually traverse the component hierarchy, which can be cumbersome and error-prone. Query functions provide a more efficient and reliable way to interact with your component library.
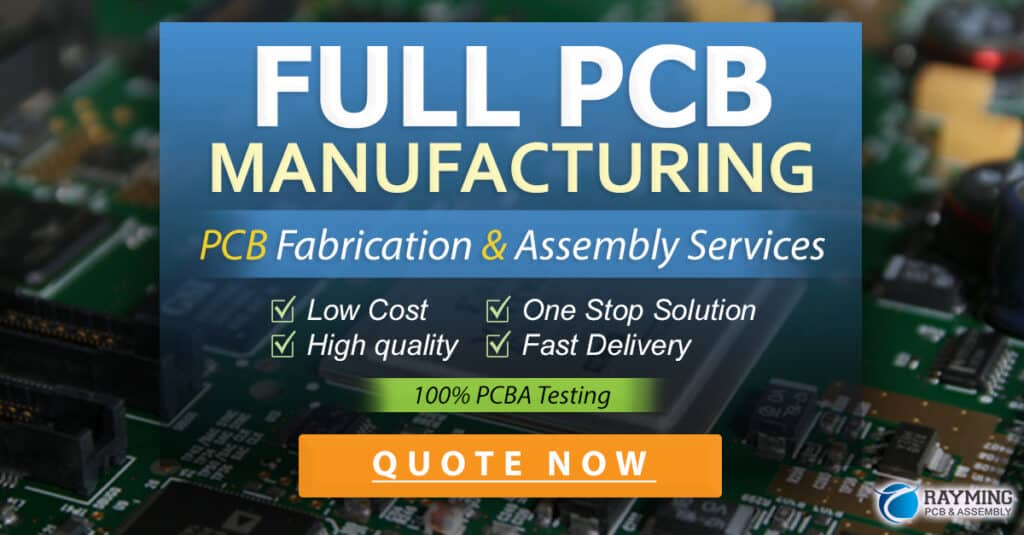
Types of Query Functions
There are different types of query functions available, each serving a specific purpose. Let’s explore some of the most common types:
Selector-based Queries
Selector-based queries allow you to find components based on specific attributes or properties. These queries are similar to CSS selectors, where you can target components using their class names, IDs, or other attributes. Here are a few examples:
Selector | Description |
---|---|
.classname |
Selects components with the specified class name |
#id |
Selects a component with the specified ID |
[attribute] |
Selects components with the specified attribute |
[attribute=value] |
Selects components with the specified attribute and value |
[attribute^=value] |
Selects components whose attribute starts with the value |
[attribute$=value] |
Selects components whose attribute ends with the value |
[attribute*=value] |
Selects components whose attribute contains the value |
Selector-based queries are powerful and flexible, allowing you to target specific components based on their attributes. They are commonly used for testing, debugging, and dynamically modifying component properties.
Traversal Queries
Traversal queries enable you to navigate through the component tree and find related components. These queries are useful when you need to access parent, child, or sibling components. Some common traversal queries include:
Query | Description |
---|---|
.parent() |
Selects the immediate parent component |
.children() |
Selects all the direct child components |
.siblings() |
Selects all sibling components (excluding the current one) |
.find() |
Selects descendant components that match a specific selector |
.closest() |
Selects the closest ancestor component that matches a selector |
Traversal queries are particularly handy when you need to access related components based on their hierarchical relationship. For example, you might want to find all the child components of a specific parent component or locate the closest ancestor component that matches a certain criteria.
Filtering Queries
Filtering queries allow you to refine your component selection based on specific conditions. These queries help you narrow down the components based on their properties, state, or other criteria. Some examples of filtering queries include:
Query | Description |
---|---|
.filter() |
Selects components that match a specific condition |
.not() |
Selects components that do not match a specific selector |
.has() |
Selects components that have descendant elements matching a selector |
.is() |
Checks if the selected components match a specific selector |
.eq() |
Selects a component at a specific index |
Filtering queries are useful when you need to select components based on complex conditions or when you want to exclude certain components from your selection. They provide a way to fine-tune your component queries and focus on specific subsets of components.
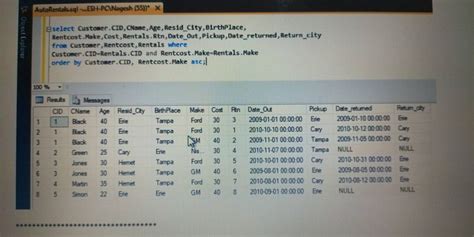
Implementing Query Functions
Now that we understand the different types of query functions, let’s explore how to implement them in your component library.
Using Built-in Query Functions
Many component libraries come with built-in query functions that you can use out of the box. These functions are typically provided as part of the library’s API and can be accessed through the component instances or a dedicated query API.
For example, in React, you can use the ReactDOM.findDOMNode()
function to access the underlying DOM node of a component:
import React from 'react';
import ReactDOM from 'react-dom';
class MyComponent extends React.Component {
componentDidMount() {
const domNode = ReactDOM.findDOMNode(this);
console.log(domNode); // Access the DOM node of the component
}
render() {
return <div>Hello, World!</div>;
}
}
Similarly, in Vue, you can use the $refs
object to access child components:
<template>
<div>
<child-component ref="myChild"></child-component>
</div>
</template>
<script>
export default {
mounted() {
const childComponent = this.$refs.myChild;
console.log(childComponent); // Access the child component instance
}
}
</script>
It’s important to refer to the documentation of your specific component library to understand the available built-in query functions and how to use them effectively.
Creating Custom Query Functions
In addition to using built-in query functions, you can also create your own custom query functions to suit your specific needs. Custom query functions allow you to encapsulate complex querying logic and provide a more convenient way to interact with your components.
Here’s an example of creating a custom query function in React:
import React from 'react';
const findComponentByType = (root, componentType) => {
if (root.type === componentType) {
return root;
}
if (root.props && root.props.children) {
const children = Array.isArray(root.props.children) ? root.props.children : [root.props.children];
for (const child of children) {
if (React.isValidElement(child)) {
const foundComponent = findComponentByType(child, componentType);
if (foundComponent) {
return foundComponent;
}
}
}
}
return null;
};
// Usage
class MyComponent extends React.Component {
componentDidMount() {
const buttonComponent = findComponentByType(this, 'button');
console.log(buttonComponent); // Access the button component instance
}
render() {
return (
<div>
<h1>Hello, World!</h1>
<button>Click me</button>
</div>
);
}
}
In this example, the findComponentByType
function recursively traverses the component tree starting from the root
component and searches for a component with the specified componentType
. If a matching component is found, it is returned; otherwise, null
is returned.
Creating custom query functions allows you to encapsulate complex querying logic and provide a more intuitive way to interact with your components. You can define query functions that are tailored to your specific component library and application requirements.
Best Practices for Query Functions
When working with query functions, it’s important to follow best practices to ensure efficient and maintainable code. Here are a few best practices to keep in mind:
Performance Considerations
Query functions can potentially impact the performance of your application, especially when dealing with large component trees. Here are a few performance considerations to keep in mind:
- Avoid excessive querying: Minimize the number of queries performed and only query for components when necessary.
- Use specific selectors: Use specific selectors to target components precisely, avoiding unnecessary traversals.
- Cache query results: If you need to access the same components multiple times, consider caching the query results to avoid redundant queries.
- Optimize query logic: Ensure that your query functions are optimized for performance, avoiding unnecessary iterations or computations.
Error Handling and Validation
When using query functions, it’s important to handle errors and validate the results to prevent unexpected behavior. Consider the following:
- Null checks: Always check if the query result is null or undefined before accessing its properties or methods.
- Type checks: Verify that the query result is of the expected type before performing operations on it.
- Error handling: Implement proper error handling mechanisms to gracefully handle cases where queries fail or return unexpected results.
- Logging and debugging: Use logging and debugging techniques to troubleshoot and identify issues related to query functions.
Documentation and Examples
To ensure that your query functions are easily understandable and usable by other developers, it’s crucial to provide clear documentation and examples. Consider the following:
- Function documentation: Document each query function, explaining its purpose, parameters, return value, and any side effects.
- Usage examples: Provide code examples showcasing how to use the query functions in different scenarios.
- Best practices: Include guidelines and best practices for using the query functions effectively and efficiently.
- Versioning and compatibility: Document the versioning and compatibility of your query functions, specifying any breaking changes or dependencies.
By providing comprehensive documentation and examples, you can help other developers understand and utilize your query functions effectively, promoting code reusability and maintainability.
Real-World Examples
To illustrate the practical application of query functions, let’s explore a few real-world examples in popular component libraries.
Querying Components in React
In React, you can use the React.Children
API to traverse and manipulate the children of a component. Here’s an example:
import React from 'react';
class MyComponent extends React.Component {
render() {
const { children } = this.props;
// Count the number of child components
const childCount = React.Children.count(children);
// Map over the child components and modify their props
const modifiedChildren = React.Children.map(children, (child) => {
return React.cloneElement(child, { className: 'modified' });
});
return (
<div>
<h1>My Component</h1>
<p>Number of child components: {childCount}</p>
{modifiedChildren}
</div>
);
}
}
In this example, we use the React.Children.count()
function to count the number of child components and the React.Children.map()
function to iterate over the child components and modify their props using React.cloneElement()
.
Querying Components in Vue
In Vue, you can use the $children
and $parent
properties to access child and parent components respectively. Here’s an example:
<template>
<div>
<child-component ref="myChild"></child-component>
<button @click="accessChildComponent">Access Child Component</button>
</div>
</template>
<script>
export default {
methods: {
accessChildComponent() {
const childComponent = this.$refs.myChild;
console.log(childComponent); // Access the child component instance
const parentComponent = this.$parent;
console.log(parentComponent); // Access the parent component instance
}
}
}
</script>
In this example, we use the $refs
object to access the child component instance and the $parent
property to access the parent component instance.
Querying Components in Angular
In Angular, you can use the @ViewChild
and @ViewChildren
decorators to query child components. Here’s an example:
import { Component, ViewChild, ElementRef } from '@angular/core';
@Component({
selector: 'app-my-component',
template: `
<div>
<child-component #myChild></child-component>
<button (click)="accessChildComponent()">Access Child Component</button>
</div>
`
})
export class MyComponent {
@ViewChild('myChild') childComponent: ElementRef;
accessChildComponent() {
console.log(this.childComponent); // Access the child component instance
}
}
In this example, we use the @ViewChild
decorator to query the child component using a template reference variable (#myChild
) and access its instance in the component class.
These are just a few examples of how query functions can be used in different component libraries. Each library has its own set of query APIs and techniques, so it’s important to refer to the specific documentation of the library you are using.
FAQ
-
What are component library query functions?
Component library query functions are methods or APIs provided by component libraries that allow you to search, select, and manipulate components within your application programmatically. -
Why are query functions important in component libraries?
Query functions are important because they enable developers to interact with components dynamically, access specific components for testing or modification, traverse the component tree, and filter components based on certain criteria. They provide a more efficient and reliable way to work with components compared to manual traversal. -
What are some common types of query functions?
Some common types of query functions include: - Selector-based queries: Allow you to find components based on specific attributes or properties.
- Traversal queries: Enable you to navigate through the component tree and find related components.
-
Filtering queries: Allow you to refine your component selection based on specific conditions.
-
How can I implement query functions in my component library?
You can implement query functions in your component library by: - Using built-in query functions provided by the library’s API.
-
Creating custom query functions to encapsulate complex querying logic and provide a more convenient way to interact with components.
-
What are some best practices for working with query functions?
Some best practices for working with query functions include: - Considering performance by minimizing excessive querying, using specific selectors, caching query results, and optimizing query logic.
- Handling errors and validating query results to prevent unexpected behavior.
- Providing clear documentation and examples to help other developers understand and utilize your query functions effectively.
Conclusion
Component library query functions are a powerful tool for interacting with components programmatically in your application. They allow you to search, select, and manipulate components efficiently, enabling scenarios such as testing, dynamic modification, and traversal of the component tree.
In this article, we explored the concept of component library query functions, including the different types of queries, how to implement them using built-in and custom functions, and best practices for working with them effectively. We also looked at real-world examples in popular component libraries like React, Vue, and Angular.
By leveraging query functions in your component library, you can enhance your development workflow, write more efficient and maintainable code, and create dynamic and interactive user interfaces. Remember to consider performance, error handling, and documentation when working with query functions to ensure a smooth and reliable development experience.
As you continue to work with component libraries, take the time to explore and understand the query functions available in your chosen library. Experiment with different query techniques, create
No responses yet