What is a RuntimeCrash?
A RuntimeCrash is an error that occurs when a program encounters an unexpected problem during its execution, causing it to terminate abruptly. In the context of Ad20 VisualC, a RuntimeCrash refers to a specific type of crash that happens within the Visual C++ runtime environment.
Causes of RuntimeCrash in Ad20 VisualC
There can be several reasons behind a RuntimeCrash in Ad20 VisualC. Some of the most common causes include:
-
Memory corruption: When a program accidentally overwrites memory that doesn’t belong to it or attempts to access memory that has already been freed, it can lead to memory corruption and, consequently, a RuntimeCrash.
-
Null pointer dereference: Trying to access or manipulate a null pointer (a pointer that doesn’t point to a valid memory location) can trigger a RuntimeCrash.
-
Stack overflow: If a program recursively calls functions without proper termination conditions or allocates too much memory on the stack, it can cause a stack overflow, resulting in a RuntimeCrash.
-
Heap corruption: Similar to memory corruption, heap corruption occurs when a program incorrectly manages dynamically allocated memory, leading to issues like buffer overflows or accessing freed memory.
-
Incompatible libraries: Using incompatible or outdated versions of libraries or mixing different versions of the Visual C++ runtime can cause conflicts and lead to RuntimeCrashes.
Debugging a RuntimeCrash
When encountering a RuntimeCrash, it’s essential to gather relevant information to help identify the root cause of the problem. Here are some steps you can take to debug a RuntimeCrash:
-
Enable debugging symbols: Make sure that your project is compiled with debugging symbols. This allows you to obtain more detailed information about the crash, including the exact line of code where the error occurred.
-
Use a debugger: Run your program within a debugger, such as Visual Studio’s built-in debugger or WinDbg. When the crash occurs, the debugger will break at the point of failure, allowing you to examine variables, call stack, and other relevant information.
-
Analyze crash dumps: If you have a crash dump file (
.dmp
), you can load it into a debugger to investigate the crash. Crash dumps contain a snapshot of the program’s state at the time of the crash, including memory contents and thread information. -
Review error messages: Pay attention to any error messages or exceptions thrown during the crash. These messages often provide clues about the nature of the problem and can help narrow down the search for the root cause.
-
Employ debugging techniques: Use debugging techniques such as setting breakpoints, stepping through code, and examining variable values to identify the specific line or section of code where the crash occurs.
Example: Debugging a Null Pointer Dereference
Let’s consider an example of debugging a RuntimeCrash caused by a null pointer dereference. Suppose you have the following code:
#include <iostream>
class MyClass {
public:
void doSomething() {
std::cout << "Doing something" << std::endl;
}
};
int main() {
MyClass* obj = nullptr;
obj->doSomething(); // Null pointer dereference
return 0;
}
When you run this code, it will likely result in a RuntimeCrash due to the null pointer dereference on line 12. To debug this crash:
- Compile the code with debugging symbols enabled.
- Run the program within a debugger.
- When the crash occurs, the debugger will break at line 12.
- Examine the value of the
obj
pointer, which will benullptr
. - Trace back the code to identify where the
obj
pointer was assigned a null value. - Fix the code by properly initializing the
obj
pointer or adding appropriate null checks.
Preventing RuntimeCrashes
To minimize the occurrence of RuntimeCrashes in your Ad20 VisualC programs, consider the following best practices:
-
Proper memory management: Be cautious when allocating and deallocating memory. Always free dynamically allocated memory when it’s no longer needed, and avoid accessing memory that has been freed.
-
Null pointer checks: Before dereferencing a pointer, ensure that it is not null. Add null checks to prevent null pointer dereferences.
-
Bounds checking: When accessing arrays or buffers, make sure to stay within the valid bounds to avoid buffer overflows and out-of-bounds accesses.
-
Exception handling: Implement proper exception handling mechanisms to gracefully handle and recover from errors instead of abruptly terminating the program.
-
Library compatibility: Ensure that you are using compatible versions of libraries and the Visual C++ runtime. Avoid mixing different versions or using outdated libraries.
-
Testing and code review: Thoroughly test your code, including edge cases and error scenarios. Conduct code reviews to identify potential issues and improve code quality.
Example: Preventing Null Pointer Dereference
To prevent the null pointer dereference crash from the previous example, you can modify the code as follows:
#include <iostream>
class MyClass {
public:
void doSomething() {
std::cout << "Doing something" << std::endl;
}
};
int main() {
MyClass* obj = nullptr;
if (obj != nullptr) {
obj->doSomething();
} else {
std::cout << "Object is null. Cannot perform operation." << std::endl;
}
return 0;
}
By adding a null check before dereferencing the obj
pointer, you can prevent the RuntimeCrash and handle the case when the pointer is null gracefully.
FAQ
-
What is a RuntimeCrash in Ad20 VisualC?
A RuntimeCrash in Ad20 VisualC is an error that occurs during the execution of a program, causing it to terminate unexpectedly. It typically happens due to issues like memory corruption, null pointer dereference, or stack overflow. -
How can I debug a RuntimeCrash in Ad20 VisualC?
To debug a RuntimeCrash, you can enable debugging symbols, use a debugger to run the program, analyze crash dumps, review error messages, and employ debugging techniques like setting breakpoints and examining variable values. -
What are some common causes of RuntimeCrashes in Ad20 VisualC?
Common causes of RuntimeCrashes include memory corruption, null pointer dereference, stack overflow, heap corruption, and using incompatible libraries or mixing different versions of the Visual C++ runtime. -
How can I prevent RuntimeCrashes in my Ad20 VisualC programs?
To prevent RuntimeCrashes, follow best practices such as proper memory management, null pointer checks, bounds checking, exception handling, ensuring library compatibility, and conducting thorough testing and code reviews. -
What should I do if I encounter a RuntimeCrash in my Ad20 VisualC program?
If you encounter a RuntimeCrash, gather relevant information like error messages and crash dumps, enable debugging symbols, and use a debugger to investigate the crash. Identify the root cause of the problem and apply appropriate fixes or error handling mechanisms to prevent future occurrences.
Cause | Description |
---|---|
Memory corruption | Accidentally overwriting memory or accessing freed memory |
Null pointer dereference | Attempting to access or manipulate a null pointer |
Stack overflow | Recursive function calls without proper termination or excessive memory allocation on the stack |
Heap corruption | Incorrect management of dynamically allocated memory |
Incompatible libraries | Using incompatible or outdated versions of libraries or mixing different versions of the Visual C++ runtime |
By understanding the causes, debugging techniques, and preventive measures for RuntimeCrashes in Ad20 VisualC, you can write more robust and reliable code. Regular testing, code reviews, and following best practices can go a long way in minimizing the occurrence of RuntimeCrashes and improving the overall stability of your programs.
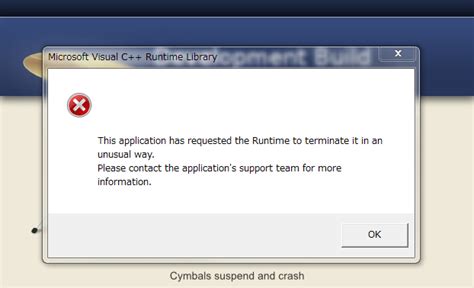
No responses yet