What is a PCB API?
A PCB (Printed Circuit Board) API (Application Programming Interface) is a set of protocols, tools, and libraries that allow developers to interact with and control the design, manufacturing, and assembly processes of PCBs programmatically. It enables the automation of various tasks related to PCB development, such as design rule checks (DRC), bill of materials (BOM) generation, and file format conversions.
Benefits of using a PCB API
- Streamlines the PCB design and manufacturing process
- Reduces human error and improves accuracy
- Enables integration with other software tools and systems
- Facilitates collaboration between team members and stakeholders
- Speeds up time-to-market for PCB-based products
Key features of a PCB API
Design automation
A PCB API can automate various aspects of the PCB design process, such as:
- Applying design rules and constraints
- Generating and placing components
- Routing traces and vias
- Creating and managing layers
- Generating manufacturing files (e.g., Gerber, Excellon, IPC-2581)
Example: Automating design rule checks using a PCB API
from pcb_api import PCBDesign, DesignRuleCheck
# Load the PCB design
design = PCBDesign.load("my_design.pcb")
# Create a design rule check object
drc = DesignRuleCheck(design)
# Define the design rules
drc.add_rule("Minimum trace width", min_width=0.2)
drc.add_rule("Minimum clearance", min_clearance=0.1)
# Run the design rule check
violations = drc.run()
# Print the violations
for violation in violations:
print(violation)
Data management
A PCB API can help manage the data associated with a PCB design, such as:
- Bill of Materials (BOM)
- Component libraries
- Material properties
- Manufacturing specifications
Example: Generating a bill of materials using a PCB API
from pcb_api import PCBDesign, BOM
# Load the PCB design
design = PCBDesign.load("my_design.pcb")
# Create a BOM object
bom = BOM(design)
# Generate the BOM
bom_table = bom.generate()
# Print the BOM
print(bom_table)
Component | Quantity | Manufacturer | Part Number |
---|---|---|---|
Resistor | 10 | ACME | RES-1K-0805 |
Capacitor | 5 | ACME | CAP-10U-1206 |
IC | 1 | ACME | IC-MCU-ATMEGA328P |
File format conversion
A PCB API can convert between different PCB file formats, such as:
- Gerber (RS-274X)
- Excellon
- ODB++
- IPC-2581
- IDX
Example: Converting a PCB design to Gerber format using a PCB API
from pcb_api import PCBDesign, GerberExport
# Load the PCB design
design = PCBDesign.load("my_design.pcb")
# Create a Gerber export object
gerber_export = GerberExport(design)
# Set the Gerber export options
gerber_export.set_option("unit", "mm")
gerber_export.set_option("format", "RS274X")
# Export the PCB design to Gerber format
gerber_export.export("my_design_gerber")
Integration with other tools
A PCB API can integrate with other software tools and systems, such as:
- Electronic Design Automation (EDA) software
- Product Lifecycle Management (PLM) systems
- Enterprise Resource Planning (ERP) systems
- Manufacturing Execution Systems (MES)
Example: Integrating a PCB API with an EDA tool
from pcb_api import PCBDesign
from eda_tool import EDAProject
# Load the PCB design
design = PCBDesign.load("my_design.pcb")
# Create an EDA project
eda_project = EDAProject("my_project")
# Add the PCB design to the EDA project
eda_project.add_design(design)
# Perform EDA-specific tasks
eda_project.run_simulation()
eda_project.generate_schematic()
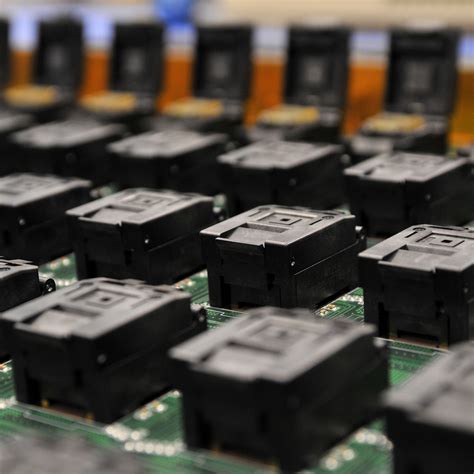
Implementing a PCB API
API architecture
A PCB API typically follows a client-server architecture, where the client (e.g., a design tool or a custom application) communicates with the server (e.g., a PCB manufacturing service) via HTTP requests and responses.
The API server exposes a set of endpoints (URLs) that the client can call to perform various actions, such as uploading a design file, retrieving a bill of materials, or placing an order.
API security
To ensure the security of the PCB API, the following measures can be implemented:
- Authentication: Require clients to authenticate themselves before accessing the API endpoints, using techniques such as API keys, OAuth, or JSON Web Tokens (JWT).
- Encryption: Use HTTPS (SSL/TLS) to encrypt the data transmitted between the client and the server.
- Rate limiting: Limit the number of API requests that a client can make within a given time period to prevent abuse and protect against denial-of-service attacks.
API documentation
To make it easy for developers to use the PCB API, it is important to provide clear and comprehensive documentation that covers the following topics:
- API endpoints: Describe each endpoint, including its URL, HTTP method, request parameters, and response format.
- Authentication: Explain how to authenticate with the API, including the required credentials and the authentication flow.
- Error handling: Describe the possible error codes and messages that the API may return, and how to handle them.
- Code examples: Provide code examples in popular programming languages (e.g., Python, JavaScript) to illustrate how to use the API.
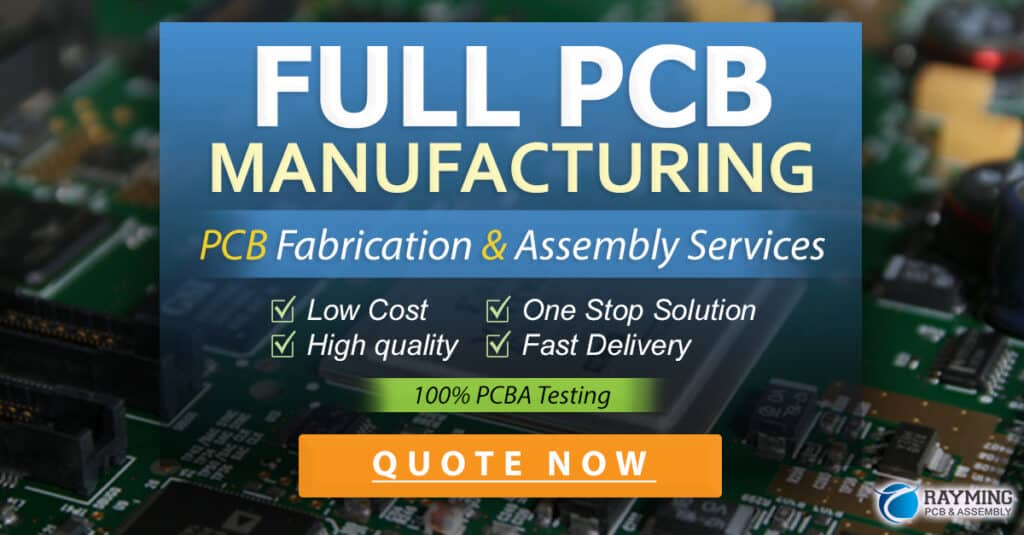
PCB API use cases
Automated PCB design
A PCB API can be used to automate the PCB design process by integrating with EDA tools and custom applications. This allows designers to:
- Apply design rules and constraints automatically
- Generate and place components based on predefined libraries
- Route traces and vias according to specified parameters
- Create and manage layers programmatically
- Generate manufacturing files (e.g., Gerber, Excellon, IPC-2581) with a single click
Rapid prototyping
A PCB API can streamline the rapid prototyping process by enabling developers to:
- Upload PCB design files programmatically
- Retrieve instant quotes for PCB manufacturing
- Place orders for prototypes with a few lines of code
- Track the status of prototype orders in real-time
- Receive notifications when prototypes are ready for shipping
Supply chain integration
A PCB API can integrate with supply chain systems, such as ERP and MES, to:
- Synchronize component and material data between systems
- Automate the procurement of components and materials
- Monitor inventory levels and trigger reorders when necessary
- Track the status of PCB manufacturing and assembly processes
- Generate reports and analytics on supply chain performance
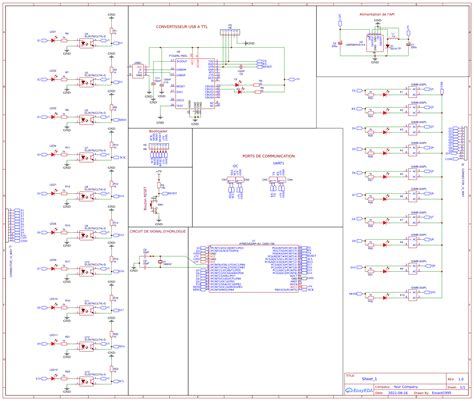
FAQ
What programming languages can I use with a PCB API?
Most PCB APIs support popular programming languages such as Python, JavaScript, C++, and Java. The specific languages supported may vary depending on the API provider.
Do I need to have PCB design experience to use a PCB API?
While having PCB design experience can be helpful, it is not strictly necessary to use a PCB API. Many PCB APIs provide high-level abstractions and helper functions that make it easy for developers to perform common tasks without deep knowledge of PCB design principles.
How much does it cost to use a PCB API?
The cost of using a PCB API varies depending on the provider and the specific features and services used. Some providers offer free tiers for basic functionality, while others charge based on usage or subscription plans.
Can I use a PCB API for commercial projects?
Yes, most PCB APIs can be used for commercial projects, subject to the terms and conditions of the API provider. It is important to review the provider’s licensing agreement and pricing model to ensure compliance and avoid unexpected costs.
How do I get started with a PCB API?
To get started with a PCB API, follow these steps:
- Choose a PCB API provider that meets your requirements and budget.
- Sign up for an account and obtain the necessary credentials (e.g., API key).
- Review the API documentation to understand the available endpoints, request/response formats, and authentication requirements.
- Install any required libraries or SDKs for your programming language of choice.
- Write code to interact with the PCB API, starting with simple tasks like uploading a design file or retrieving a bill of materials.
- Test your code thoroughly and handle any errors or exceptions gracefully.
- Integrate your PCB API code into your larger application or workflow as needed.
By leveraging the power of a PCB API, developers can streamline the PCB design and manufacturing process, reduce errors and costs, and bring innovative products to market faster than ever before.
No responses yet