Introduction to 4×4 Keypads
A 4×4 keypad is a matrix-style keypad with 16 buttons arranged in a grid of 4 rows and 4 columns. These keypads are commonly used as input devices for microcontroller projects, electronic door locks, digital code locks, and various other embedded applications requiring user input.
The 4×4 keypad offers a compact and efficient way to enter numeric data, passwords, or perform specific actions based on the pressed keys. In this in-depth guide, we will explore the workings of a 4×4 keypad, its interfacing with microcontrollers, and provide code examples to help you integrate a keypad into your projects.
Understanding the 4×4 Keypad Matrix
Keypad Matrix Structure
A 4×4 keypad consists of 16 keys arranged in a matrix formation. The keypad has 8 pins: 4 pins for the rows and 4 pins for the columns. Each key is connected to a unique combination of a row and a column.
Here’s a visual representation of a 4×4 keypad matrix:
Col 1 Col 2 Col 3 Col 4
Row 1 1 2 3 A
Row 2 4 5 6 B
Row 3 7 8 9 C
Row 4 * 0 # D
Keypad Scanning Technique
To detect which key is pressed, the microcontroller uses a scanning technique. The basic idea is to apply a voltage to each row (one at a time) and then read the state of each column. If a key is pressed, it will create a connection between the corresponding row and column, allowing the microcontroller to determine which key was pressed.
The scanning process follows these steps:
- Set all the row pins as outputs and all the column pins as inputs with pull-up resistors enabled.
- Set one row pin to LOW and leave the others at HIGH.
- Check the state of each column pin. If a column pin reads LOW, it means the key at the intersection of the current row and that column is pressed.
- Repeat steps 2 and 3 for each row pin.
By scanning the keypad matrix in this manner, the microcontroller can identify the pressed key based on the row and column combination.
Interfacing 4×4 Keypad with Microcontrollers
Hardware Connections
To interface a 4×4 keypad with a microcontroller, you need to make the following connections:
- Connect the 4 row pins of the keypad to 4 GPIO pins of the microcontroller configured as outputs.
- Connect the 4 column pins of the keypad to 4 GPIO pins of the microcontroller configured as inputs with pull-up resistors enabled.
Here’s an example connection table for a 4×4 keypad and an Arduino Uno:
Keypad Pin | Arduino Pin |
---|---|
Row 1 | D9 |
Row 2 | D8 |
Row 3 | D7 |
Row 4 | D6 |
Column 1 | D5 |
Column 2 | D4 |
Column 3 | D3 |
Column 4 | D2 |
Keypad Library
To simplify the interfacing process, you can use a keypad library specific to your microcontroller platform. These libraries handle the scanning and debouncing of the keypad, making it easier to detect key presses in your code.
For Arduino, a popular library is the “Keypad” library by Mark Stanley and Alexander Brevig. You can install it through the Arduino IDE’s Library Manager or download it from the GitHub repository.
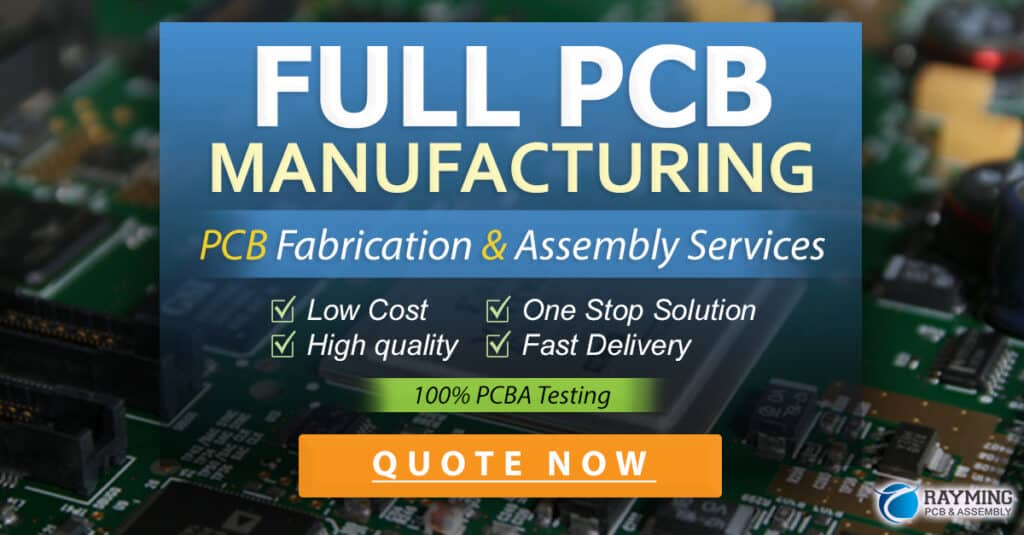
Code Examples
Arduino Code Example
Here’s an example code snippet for interfacing a 4×4 keypad with an Arduino using the Keypad library:
#include <Keypad.h>
const byte ROWS = 4;
const byte COLS = 4;
char keys[ROWS][COLS] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
byte rowPins[ROWS] = {9, 8, 7, 6};
byte colPins[COLS] = {5, 4, 3, 2};
Keypad keypad = Keypad(makeKeymap(keys), rowPins, colPins, ROWS, COLS);
void setup() {
Serial.begin(9600);
}
void loop() {
char key = keypad.getKey();
if (key) {
Serial.println(key);
}
}
This code initializes the Keypad object with the specified key matrix, row pins, and column pins. In the loop()
function, it continuously checks for key presses using the getKey()
method. If a key is pressed, it prints the corresponding character to the serial monitor.
Raspberry Pi Code Example
Here’s an example code snippet for interfacing a 4×4 keypad with a Raspberry Pi using Python:
import RPi.GPIO as GPIO
import time
# Keypad matrix pin configuration
ROW_PINS = [18, 23, 24, 25]
COL_PINS = [17, 27, 22, 10]
# Keypad matrix
KEYPAD = [
['1', '2', '3', 'A'],
['4', '5', '6', 'B'],
['7', '8', '9', 'C'],
['*', '0', '#', 'D']
]
def setup():
GPIO.setmode(GPIO.BCM)
for pin in ROW_PINS:
GPIO.setup(pin, GPIO.OUT)
GPIO.output(pin, GPIO.HIGH)
for pin in COL_PINS:
GPIO.setup(pin, GPIO.IN, pull_up_down=GPIO.PUD_UP)
def scan_keypad():
for row in range(len(ROW_PINS)):
GPIO.output(ROW_PINS[row], GPIO.LOW)
for col in range(len(COL_PINS)):
if GPIO.input(COL_PINS[col]) == GPIO.LOW:
key = KEYPAD[row][col]
while GPIO.input(COL_PINS[col]) == GPIO.LOW:
time.sleep(0.1)
return key
GPIO.output(ROW_PINS[row], GPIO.HIGH)
return None
def main():
setup()
try:
while True:
key = scan_keypad()
if key:
print(key)
except KeyboardInterrupt:
GPIO.cleanup()
if __name__ == '__main__':
main()
This code sets up the GPIO pins for the keypad rows and columns, defines the keypad matrix, and provides functions to scan the keypad for key presses. In the main()
function, it continuously scans the keypad and prints the pressed key to the console.
Applications and Projects
4×4 keypads find numerous applications in embedded systems and microcontroller projects. Here are a few examples:
-
Electronic Door Lock: Use a 4×4 keypad to enter a passcode and control an electronic door lock mechanism.
-
Calculator: Build a simple calculator using a 4×4 keypad for input and a display (e.g., LCD) for output.
-
Menu Navigation: Utilize a 4×4 keypad to navigate through menus and select options in an embedded system.
-
Access Control System: Implement an access control system that requires a user to enter a code using a 4×4 keypad to gain access to a restricted area.
-
Home Automation: Use a 4×4 keypad as an input device for controlling various home automation functions, such as turning lights on/off or adjusting thermostat settings.
These are just a few examples, and the possibilities are endless. With a 4×4 keypad, you can add user input capabilities to a wide range of projects and applications.
Troubleshooting and Tips
Debouncing
When pressing a key on the keypad, mechanical bouncing can occur, resulting in multiple key presses being registered. To mitigate this issue, you need to implement debouncing techniques in your code.
One common approach is to add a small delay after detecting a key press and wait for the key to stabilize before registering it as a valid press. The Keypad library for Arduino handles debouncing automatically, but if you’re using a different library or writing your own code, you may need to implement debouncing manually.
Pull-up Resistors
When interfacing a 4×4 keypad with a microcontroller, it’s important to use pull-up resistors on the column pins. Pull-up resistors ensure that the column pins are at a high state when no key is pressed, preventing floating inputs and false key press detections.
Most microcontrollers have built-in pull-up resistors that can be enabled through software configuration. If your microcontroller doesn’t have built-in pull-up resistors or if you prefer external resistors, you can connect pull-up resistors (typically 1kΩ to 10kΩ) between each column pin and the positive supply voltage.
Keypad Mapping
When defining the keypad matrix in your code, make sure that the characters in the matrix match the actual labels on your 4×4 keypad. If the labels on your keypad differ from the standard matrix, you may need to modify the matrix in your code accordingly.
Additionally, consider the specific requirements of your project when mapping the keys. For example, if you’re building a numeric keypad, you might want to map the keys to numbers only and handle the non-numeric keys differently.
Frequently Asked Questions (FAQ)
-
Q: Can I use a 4×4 keypad with any microcontroller?
A: Yes, a 4×4 keypad can be interfaced with most microcontrollers that have sufficient GPIO pins available. The interfacing process may vary slightly depending on the specific microcontroller and programming language used. -
Q: How do I connect a 4×4 keypad to a microcontroller?
A: To connect a 4×4 keypad to a microcontroller, you need to identify the row and column pins of the keypad and connect them to the GPIO pins of the microcontroller. The row pins are typically connected to output pins, while the column pins are connected to input pins with pull-up resistors enabled. -
Q: What is the purpose of pull-up resistors in keypad interfacing?
A: Pull-up resistors are used on the column pins of the keypad to ensure that the pins are at a high state when no key is pressed. They prevent floating inputs and false key press detections. Most microcontrollers have built-in pull-up resistors that can be enabled through software configuration. -
Q: How do I handle debouncing when using a 4×4 keypad?
A: Debouncing is necessary to avoid registering multiple key presses due to mechanical bouncing of the keys. One common approach is to add a small delay after detecting a key press and wait for the key to stabilize before registering it as a valid press. Some keypad libraries, such as the Keypad library for Arduino, handle debouncing automatically. -
Q: Can I customize the keypad matrix to match the labels on my 4×4 keypad?
A: Yes, you can modify the keypad matrix in your code to match the labels on your specific 4×4 keypad. If the labels differ from the standard matrix, simply update the matrix definition in your code to reflect the actual key labels.
Conclusion
In this in-depth guide, we explored the workings of a 4×4 keypad, its matrix structure, and the scanning technique used to detect key presses. We also covered the interfacing process with microcontrollers, provided code examples for Arduino and Raspberry Pi, and discussed various applications and projects where 4×4 keypads can be utilized.
By understanding the principles behind 4×4 keypads and following the guidelines provided in this guide, you can effectively integrate keypads into your embedded projects and add user input capabilities to your applications.
Remember to consider factors such as debouncing, pull-up resistors, and keypad mapping when working with 4×4 keypads to ensure reliable and accurate key press detection.
With the knowledge gained from this guide, you’re now equipped to explore the vast possibilities that 4×4 keypads offer and create innovative projects that harness the power of user input.
No responses yet