Introduction to the 28BYJ-48 Stepper Motor
The 28BYJ-48 is a popular and inexpensive stepper motor widely used in DIY projects and Arduino-based applications. This small, compact motor offers precise control and is suitable for a variety of tasks, such as rotating objects, positioning mechanisms, and creating motion in robotics projects. In this comprehensive guide, we will explore the characteristics of the 28BYJ-48 stepper motor, its working principles, and how to effectively use it with an Arduino board.
What is a Stepper Motor?
A stepper motor is a type of brushless DC electric motor that divides a full rotation into a number of equal steps. Unlike conventional DC motors, which rotate continuously when a fixed voltage is applied, stepper motors move in discrete increments, allowing for precise positioning and speed control. Stepper motors are commonly used in applications that require accurate and repeatable motion, such as 3D printers, CNC machines, and robotics.
Key Features of the 28BYJ-48 Stepper Motor
The 28BYJ-48 stepper motor offers several notable features that make it a popular choice among hobbyists and engineers:
-
Compact Size: Measuring approximately 28mm in diameter and 19mm in height, the 28BYJ-48 is a small and lightweight stepper motor that can easily fit into tight spaces.
-
Precision Control: With a step angle of 5.625 degrees, the 28BYJ-48 provides precise incremental movement, allowing for accurate positioning and smooth rotation.
-
Low Cost: Compared to other stepper motors, the 28BYJ-48 is highly affordable, making it accessible to a wide range of users and projects.
-
Compatibility: The motor is designed to work seamlessly with Arduino boards and can be easily controlled using the Arduino IDE and suitable libraries.
Understanding the 28BYJ-48 Stepper Motor
Before diving into the practical aspects of using the 28BYJ-48 stepper motor with Arduino, let’s take a closer look at its internal structure and working principles.
Motor Construction
The 28BYJ-48 stepper motor consists of several key components:
-
Stator: The stationary part of the motor that contains the coils responsible for generating the magnetic fields.
-
Rotor: The rotating part of the motor that is attracted to the magnetic fields generated by the stator coils.
-
Gears: The 28BYJ-48 features a gear reduction system that increases the torque and reduces the speed of the motor.
-
Wires: The motor typically comes with a 5-wire connector, with 4 wires for the coils and 1 wire for the common ground.
Stepper Motor Types
Stepper motors can be classified into three main types based on their winding arrangements and operating principles:
-
Permanent Magnet (PM) Stepper Motors: These motors have a permanent magnet rotor and operate on the attraction and repulsion between the rotor and the electromagnetic stator coils.
-
Variable Reluctance (VR) Stepper Motors: VR motors have a toothed iron rotor and operate on the principle of minimizing the reluctance between the rotor and the energized stator coils.
-
Hybrid Stepper Motors: Hybrid motors combine the features of both PM and VR motors, offering high torque and precision.
The 28BYJ-48 is a permanent magnet stepper motor, which is the most common type used in small-scale applications.
Driving the 28BYJ-48 Stepper Motor
To control the 28BYJ-48 stepper motor, you need a suitable driver circuit that can energize the coils in the correct sequence. The most common driver used with this motor is the ULN2003 driver board, which contains a set of Darlington transistor arrays.
The ULN2003 driver board simplifies the control process by allowing you to connect the motor directly to your Arduino board. It takes care of the necessary current amplification and provides protection against back EMF (electromotive force) generated by the motor coils.
Connecting the 28BYJ-48 Stepper Motor to Arduino
Now that we have a basic understanding of the 28BYJ-48 stepper motor and its driver, let’s explore how to connect it to an Arduino board.
Required Components
To get started, you’ll need the following components:
- 28BYJ-48 stepper motor
- ULN2003 driver board
- Arduino board (e.g., Arduino Uno)
- Jumper wires
- Breadboard (optional)
Wiring Diagram
The wiring diagram for connecting the 28BYJ-48 stepper motor to an Arduino board is as follows:
28BYJ-48 / ULN2003 | Arduino |
---|---|
IN1 | D8 |
IN2 | D9 |
IN3 | D10 |
IN4 | D11 |
VCC | 5V |
GND | GND |
Make sure to connect the wires according to the table above. The 28BYJ-48 motor typically comes with a connector that plugs directly into the ULN2003 driver board, making the wiring process straightforward.
Stepper Motor Libraries
To control the 28BYJ-48 stepper motor with Arduino, you can use one of the available stepper motor libraries. Two popular libraries are:
-
Stepper Library: This is the built-in Arduino library for controlling stepper motors. It provides basic functionality for setting the speed and moving the motor by a specified number of steps.
-
AccelStepper Library: AccelStepper is a third-party library that offers more advanced features, such as acceleration and deceleration control, multiple simultaneous steppers, and non-blocking operation.
In the following sections, we will provide example code using both libraries to demonstrate how to control the 28BYJ-48 stepper motor.
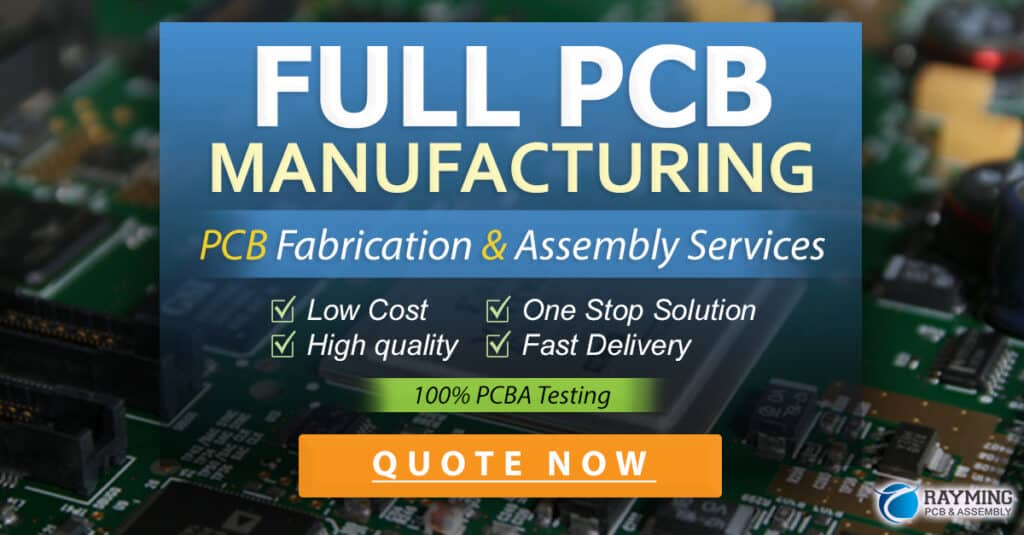
Basic Stepper Motor Control with Arduino
Let’s start with a simple example using the built-in Stepper library to control the 28BYJ-48 stepper motor.
Example 1: Rotating the Motor
Here’s a basic sketch that rotates the motor in both directions:
#include <Stepper.h>
const int stepsPerRevolution = 2048; // Number of steps per revolution for the 28BYJ-48 motor
const int motorSpeed = 15; // Motor speed in RPM
// Initialize the stepper library on pins 8 through 11
Stepper myStepper(stepsPerRevolution, 8, 10, 9, 11);
void setup() {
myStepper.setSpeed(motorSpeed);
}
void loop() {
// Rotate the motor one revolution clockwise
myStepper.step(stepsPerRevolution);
delay(500);
// Rotate the motor one revolution counterclockwise
myStepper.step(-stepsPerRevolution);
delay(500);
}
In this example, we define the number of steps per revolution for the 28BYJ-48 motor (2048) and the desired motor speed in RPM (15). We initialize the Stepper object with the appropriate pins and set the motor speed in the setup()
function.
In the loop()
function, we use the step()
function to rotate the motor one revolution clockwise by passing the number of steps as a positive value. After a short delay, we rotate the motor one revolution counterclockwise by passing a negative value.
Example 2: Controlling the Motor with a Potentiometer
You can also control the speed and direction of the 28BYJ-48 stepper motor using a potentiometer. Here’s an example sketch:
#include <Stepper.h>
const int stepsPerRevolution = 2048; // Number of steps per revolution for the 28BYJ-48 motor
const int potPin = A0; // Analog pin connected to the potentiometer
// Initialize the stepper library on pins 8 through 11
Stepper myStepper(stepsPerRevolution, 8, 10, 9, 11);
void setup() {
// Nothing to do here
}
void loop() {
int potValue = analogRead(potPin); // Read the potentiometer value (0-1023)
int motorSpeed = map(potValue, 0, 1023, 5, 15); // Map the potentiometer value to motor speed (5-15 RPM)
int stepsToMove = map(potValue, 0, 1023, -2048, 2048); // Map the potentiometer value to steps (-2048 to 2048)
myStepper.setSpeed(motorSpeed);
myStepper.step(stepsToMove);
}
In this example, we connect a potentiometer to analog pin A0. The analogRead()
function reads the potentiometer value (0-1023) in the loop()
function.
We use the map()
function to map the potentiometer value to the desired motor speed range (5-15 RPM) and the number of steps to move (-2048 to 2048). This allows us to control both the speed and direction of the motor by turning the potentiometer.
Finally, we set the motor speed using setSpeed()
and move the motor by the specified number of steps using step()
.
Advanced Stepper Motor Control with AccelStepper Library
The AccelStepper library provides more advanced features for controlling stepper motors, including acceleration and deceleration control. Let’s explore an example using this library.
Example 3: Smooth Acceleration and Deceleration
Here’s a sketch that demonstrates smooth acceleration and deceleration with the 28BYJ-48 stepper motor using the AccelStepper library:
#include <AccelStepper.h>
const int stepsPerRevolution = 2048; // Number of steps per revolution for the 28BYJ-48 motor
// Define the AccelStepper interface type; 4 wire motor in half step mode
#define motorInterfaceType 8
// Initialize the AccelStepper library
AccelStepper stepper = AccelStepper(motorInterfaceType, 8, 10, 9, 11);
void setup() {
stepper.setMaxSpeed(1000); // Set the maximum speed in steps per second
stepper.setAcceleration(500); // Set the acceleration in steps per second^2
}
void loop() {
if (stepper.distanceToGo() == 0) {
// If the motor is not moving, start a new movement
stepper.moveTo(2048); // Move 1 revolution forward
delay(1000);
stepper.moveTo(0); // Move back to the original position
delay(1000);
}
stepper.run(); // Run the motor
}
In this example, we define the motor interface type as 8
for a 4-wire motor in half-step mode. We initialize the AccelStepper object with the appropriate pins.
In the setup()
function, we set the maximum speed (setMaxSpeed()
) and acceleration (setAcceleration()
) for the motor.
In the loop()
function, we check if the motor has finished its previous movement using distanceToGo()
. If the motor is not moving, we start a new movement by setting a target position using moveTo()
. We move the motor one revolution forward (2048 steps) and then back to the original position.
The run()
function is called in each iteration of the loop to continuously update the motor’s position and speed based on the acceleration and deceleration settings.
FAQ
1. What is the step angle of the 28BYJ-48 stepper motor?
The 28BYJ-48 stepper motor has a step angle of 5.625 degrees, which means it takes 64 steps to complete one full rotation (360 degrees).
2. Can I use the 28BYJ-48 stepper motor without the ULN2003 driver board?
While it is possible to control the 28BYJ-48 stepper motor without the ULN2003 driver board, it is not recommended. The driver board simplifies the control process and provides the necessary current amplification and protection for the motor.
3. What is the maximum speed of the 28BYJ-48 stepper motor?
The maximum speed of the 28BYJ-48 stepper motor depends on factors such as the voltage supply, driver capabilities, and load. Typically, the motor can achieve speeds up to 15-20 RPM (revolutions per minute) when used with the ULN2003 driver board.
4. Can I control multiple 28BYJ-48 stepper motors simultaneously with Arduino?
Yes, you can control multiple 28BYJ-48 stepper motors simultaneously with Arduino. You’ll need to use separate driver boards for each motor and connect them to different sets of pins on the Arduino board. The AccelStepper library supports controlling multiple stepper motors simultaneously.
5. What is the torque rating of the 28BYJ-48 stepper motor?
The 28BYJ-48 stepper motor has a rated torque of approximately 34.3 mN·m (4.9 oz-in) at a speed of 5 RPM. Keep in mind that the actual torque may vary depending on factors such as voltage supply, speed, and load conditions.
Conclusion
In this comprehensive guide, we explored the 28BYJ-48 stepper motor and how to use it with Arduino. We covered the basic concepts of stepper motors, the construction and working principles of the 28BYJ-48, and the connection process using the ULN2003 driver board.
We provided example code using both the built-in Stepper library and the AccelStepper library, demonstrating basic motor control, speed and direction control with a potentiometer, and smooth acceleration and deceleration.
By following the instructions and examples in this article, you should be able to effectively integrate the 28BYJ-48 stepper motor into your Arduino projects and create precise, controlled motion for a wide range of applications.
Remember to experiment, explore, and have fun with your stepper motor projects. The 28BYJ-48 is a versatile and affordable component that opens up a world of possibilities in the realm of Arduino-based robotics and automation.
Happy tinkering!
No responses yet